Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / VSDExceptions.cs / 1 / VSDExceptions.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design { using System; using System.Diagnostics; using System.Windows.Forms; ////// This is the internal exception error constants file /// Define each internal exception error by three constants: int code, string msg, bool showmsg /// format of the const name: /// XXX_YYYYYYY_CODE, (required) /// XXX_YYYYYYY_MSG, (required) where XXX is the area name, e.g. DSD for DataSourceDesigner, YYYY is the exception name /// XXX_YYYYYYY_SHOWMSG, (optional, default to false) /// internal class VSDExceptions{ // private constructor to avoid class being instantiated. private VSDExceptions() { } //###################################################################### // // Error code for Common goes here from 0001 to 9999 // //###################################################################### internal class COMMON{ // private constructor to avoid class being instantiated. private COMMON() { } internal const int START_CODE = 0; internal const string SIMPLENAMESERVICE_NAMEOVERFLOW_MSG = "Failed to create unique name after many attempts"; internal const int SIMPLENAMESERVICE_NAMEOVERFLOW_CODE = START_CODE + 1; internal const string NOT_A_NAMED_OBJECT_MSG = "Named object collection holds something that is not a named object"; internal const int NOT_A_NAMED_OBJECT_CODE = START_CODE + 2; } //###################################################################### // // Error code for DesignTime DataSource goes here from 20001 to 29999 // //###################################################################### internal class DataSource { // private constructor to avoid class being instantiated. private DataSource() { } internal const int START_CODE = 20000; internal const string NO_CONNECTION_NAME_SERVICE_MSG = "Failed to obtain name service for connection collection"; internal const int NO_CONNECTION_NAME_SERVICE_CODE = START_CODE + 1; internal const string TABLE_BELONGS_TO_OTHER_DATA_SOURCE_MSG = "This table belongs to another DataSource already"; internal const int TABLE_BELONGS_TO_OTHER_DATA_SOURCE_CODE = START_CODE + 2; internal const string PARAMETER_NOT_FOUND_MSG = "No parameter named '{0}' found"; internal const int PARAMETER_NOT_FOUND_CODE = START_CODE + 4; internal const string INVALID_PARAMETER_VALUE_MSG = "Invalid parameter value (the object passed in must support IDbDataParameter interface)"; internal const int INVALID_PARAMETER_VALUE_CODE = START_CODE + 5; internal const string INVALID_SOURCE_VALUE_MSG = "SourceCollection can only hold objects of type Source"; internal const int INVALID_SOURCE_VALUE_CODE = START_CODE + 6; internal const string OP_VALID_FOR_RAD_TABLE_ONLY_MSG = "Operation invalid. Table gets data from something else than a database."; internal const int OP_VALID_FOR_RAD_TABLE_ONLY_CODE = START_CODE + 7; internal const string INVALID_COLUMN_VALUE_MSG = "DesignColumnCollection can only hold objects of type DesignColumn"; internal const int INVALID_COLUMN_VALUE_CODE = START_CODE + 8; internal const string DESIGN_COLUMN_NEEDS_DATA_COLUMN_MSG = "DesignColumn object needs a valid DataColumn"; internal const int DESIGN_COLUMN_NEEDS_DATA_COLUMN_CODE = START_CODE + 9; internal const string RELATION_BELONGS_TO_OTHER_DATA_SOURCE_MSG = "This relation belongs to another DataSource already"; internal const int RELATION_BELONGS_TO_OTHER_DATA_SOURCE_CODE = START_CODE + 10; internal const string INVALID_COLUMN_INDEX_MSG = "Index out of range in getting DesignColumn"; internal const int INVALID_COLUMN_INDEX_CODE = START_CODE + 11; internal const string COMMAND_NOT_SET_MSG = "Command not set. Operation cannot be performed"; internal const int COMMAND_NOT_SET_CODE = START_CODE + 12; internal const string CONNECTION_NOT_SET_MSG = "Connection not set. Operation cannot be performed"; internal const int CONNECTION_NOT_SET_CODE = START_CODE + 13; internal const string BAD_QUERY_FOR_GENERATING_IUD_MSG = "Cannot generate updating statements from query that is not a SELECT query"; internal const int BAD_QUERY_FOR_GENERATING_IUD_CODE = START_CODE + 14; internal const string INVALID_DATA_SOURCE_NAME_MSG = "Data source name is empty or invalid"; internal const int INVALID_DATA_SOURCE_NAME_CODE = START_CODE + 15; internal const string INVALID_COLLECTIONTYPE_MSG = "{0} can hold only {1} objects"; internal const int INVALID_COLLECTIONTYPE_CODE = START_CODE + 16; internal const string CANNOT_GET_DATA_CONFIGURATION_CONTEXT_MSG = "Data configuration context could not be obtained"; internal const int CANNOT_GET_DATA_CONFIGURATION_CONTEXT_CODE = START_CODE + 17; internal const string CANNOT_GET_IDBPROVIDER_MSG = "Data provider information could not be obtained"; internal const int CANNOT_GET_IDBPROVIDER_CODE = START_CODE + 18; internal const string DBSOURCECMD_HAS_INVALID_PARENT_MSG = "Parent of the DbSourceCommand is invalid"; internal const int DBSOURCECMD_HAS_INVALID_PARENT_CODE = START_CODE + 19; internal const string DATASOURCECOLLECTIONUNDOUNIT_INVALIDPARA_MSG = "Invalid parameters in DataSourceCollectionUndounit"; internal const int DATASOURCECOLLECTIONUNDOUNIT_INVALIDPARA_CODE = START_CODE + 20; internal const string CANNOT_GET_IDBPROVIDER_FOR_CONNECTION_MSG = "Could not get data provider information for connection of type {0}"; internal const int CANNOT_GET_IDBPROVIDER_FOR_CONNECTION_CODE = START_CODE + 21; internal const string CANNOT_GET_IDBPROVIDER_FOR_CSTRING_MSG = "Could not get data provider information for the following connection string:\r\n{0}\r\n"; internal const int CANNOT_GET_IDBPROVIDER_FOR_CSTRING_CODE = START_CODE + 22; internal const string INVALID_DATA_SOURCE_MSG = "Data source is invalid (null)"; internal const int INVALID_DATA_SOURCE_CODE = START_CODE + 23; internal const string SELECT_COMMAND_TEXT_EMPTY_MSG = "Select command text is null or empty"; internal const int SELECT_COMMAND_TEXT_EMPTY_CODE = START_CODE + 24; internal const string PK_COLUMNS_MISSING_MSG = "Some primary key columns are missing"; internal const int PK_COLUMNS_MISSING_CODE = START_CODE + 25; internal const string CONF_CONTEXT_NULL_MSG = "Data configuration context is null"; internal const int CONF_CONTEXT_NULL_CODE = START_CODE + 26; internal const string COMMAND_OPERATION_INVALID_MSG = "Specified command operation is invalid (can only be INSERT, UPDATE or DELETE)"; internal const int COMMAND_OPERATION_INVALID_CODE = START_CODE + 27; internal const string SPROC_NAME_EMPTY_MSG = "The stored procedure name is null or empty"; internal const int SPROC_NAME_EMPTY_CODE = START_CODE + 28; internal const string NO_SPROC_GENERATOR_MSG = "We have no stored procedure generator for specified backend"; internal const int NO_SPROC_GENERATOR_CODE = START_CODE + 28; internal const string SOURCE_IS_NOT_DBSOURCE_MSG = "The source for this table is not a DbSource"; internal const int SOURCE_IS_NOT_DBSOURCE_CODE = START_CODE + 29; internal const string BINDABLE_CTRL_NOT_FOUND_MSG = "Bindable control could not be found; Visual Studio data binding configuration file might be corrupt"; internal const int BINDABLE_CTRL_NOT_FOUND_CODE = START_CODE + 30; internal const string CANNOT_GET_PROVIDER_FACTORY_MSG = "Could not retrieve the provider factory."; internal const int CANNOT_GET_PROVIDER_FACTORY_CODE = START_CODE + 31; internal const string FACTORY_CANNOT_CREATE_ADAPTERS_MSG = "The provider factory does not support creating adapters."; internal const int FACTORY_CANNOT_CREATE_ADAPTERS_CODE = START_CODE + 32; internal const string FACTORY_CANNOT_CREATE_COMMAND_BUILDERS_MSG = "The provider factory does not support creating command builders."; internal const int FACTORY_CANNOT_CREATE_COMMAND_BUILDERS_CODE = START_CODE + 33; internal const string FACTORY_COULD_NOT_CREATE_ADAPTER_MSG = "The provider factory failed to create an adapter."; internal const int FACTORY_COULD_NOT_CREATE_ADAPTER_CODE = START_CODE + 34; internal const string FACTORY_COULD_NOT_CREATE_COMMAND_BUILDER_MSG = "The provider factory failed to create a command builder."; internal const int FACTORY_COULD_NOT_CREATE_COMMAND_BUILDER_CODE = START_CODE + 35; internal const string CANNOT_GET_COMMAND_BUILDER_FROM_DBSOURCE_MSG = "Failed to get a command builder for the DbSource."; internal const int CANNOT_GET_COMMAND_BUILDER_FROM_DBSOURCE_CODE = START_CODE + 36; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
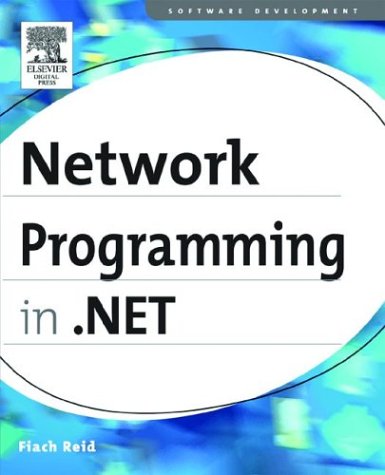
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RoutedUICommand.cs
- DynamicContractTypeBuilder.cs
- CacheOutputQuery.cs
- ChangesetResponse.cs
- InProcStateClientManager.cs
- ReadOnlyDataSourceView.cs
- ProviderConnectionPointCollection.cs
- ConfigXmlComment.cs
- CellConstantDomain.cs
- AddInDeploymentState.cs
- PropagatorResult.cs
- SafeHandles.cs
- KnownBoxes.cs
- StorageAssociationSetMapping.cs
- WebBrowserSiteBase.cs
- WindowsIdentity.cs
- WebEvents.cs
- Attributes.cs
- Hashtable.cs
- Compiler.cs
- DataServiceExpressionVisitor.cs
- PeerPresenceInfo.cs
- Geometry.cs
- clipboard.cs
- ButtonBase.cs
- MethodExpr.cs
- StaticContext.cs
- CheckBox.cs
- AddingNewEventArgs.cs
- ProxyWebPartConnectionCollection.cs
- PrivilegeNotHeldException.cs
- StringArrayEditor.cs
- Models.cs
- XmlSchemaChoice.cs
- DBDataPermissionAttribute.cs
- CustomExpression.cs
- MetadataCache.cs
- ScrollItemProviderWrapper.cs
- OracleParameterCollection.cs
- OptimalBreakSession.cs
- PostBackOptions.cs
- ConnectionManagementElement.cs
- AtomServiceDocumentSerializer.cs
- ContentDisposition.cs
- TableRowCollection.cs
- IntegerCollectionEditor.cs
- DecimalAverageAggregationOperator.cs
- UriParserTemplates.cs
- UITypeEditor.cs
- WorkflowServiceBehavior.cs
- HtmlElementCollection.cs
- XsltInput.cs
- DockingAttribute.cs
- ActiveDocumentEvent.cs
- mediaeventshelper.cs
- CloseCollectionAsyncResult.cs
- CounterCreationData.cs
- AttachInfo.cs
- SynchronizationContext.cs
- Blend.cs
- CombinedTcpChannel.cs
- OperationInfo.cs
- SerializerDescriptor.cs
- InfoCardClaim.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- BaseTreeIterator.cs
- SettingsPropertyValueCollection.cs
- SecurityNegotiationException.cs
- FixedPosition.cs
- RealizationDrawingContextWalker.cs
- Attributes.cs
- ModifiableIteratorCollection.cs
- EntityViewGenerator.cs
- GridViewRowEventArgs.cs
- CodeSubDirectoriesCollection.cs
- EntityModelSchemaGenerator.cs
- ExclusiveCanonicalizationTransform.cs
- SettingsPropertyNotFoundException.cs
- RetrieveVirtualItemEventArgs.cs
- SerializationSectionGroup.cs
- StackBuilderSink.cs
- TableItemStyle.cs
- RtfControlWordInfo.cs
- ThemeInfoAttribute.cs
- RedirectionProxy.cs
- ResourcesBuildProvider.cs
- FrameworkObject.cs
- PropertyCondition.cs
- ParallelEnumerableWrapper.cs
- RedistVersionInfo.cs
- NonSerializedAttribute.cs
- ListControlStringCollectionEditor.cs
- BooleanToVisibilityConverter.cs
- TransactionChannelFactory.cs
- SoapException.cs
- DataSourceListEditor.cs
- XamlValidatingReader.cs
- EntitySetBase.cs
- XmlComment.cs
- TextEffectCollection.cs