Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / mediaeventshelper.cs / 1305600 / mediaeventshelper.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: mediaeventshelper.cs // //----------------------------------------------------------------------------- using System; using System.Text; using System.Diagnostics; using System.ComponentModel; using MS.Internal; using System.Windows.Media.Animation; using System.Windows.Media; using System.Windows.Media.Composition; using System.Windows.Threading; using System.Runtime.InteropServices; using System.IO; using System.Collections; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { #region MediaEventsHelper ////// MediaEventsHelper /// Provides helper methods for media event related tasks /// internal class MediaEventsHelper : IInvokable { #region Constructors and Finalizers ////// Constructor /// internal MediaEventsHelper(MediaPlayer mediaPlayer) { _mediaOpened = new DispatcherOperationCallback(OnMediaOpened); this.DispatcherMediaOpened += _mediaOpened; _mediaFailed = new DispatcherOperationCallback(OnMediaFailed); this.DispatcherMediaFailed += _mediaFailed; _mediaPrerolled = new DispatcherOperationCallback(OnMediaPrerolled); this.DispatcherMediaPrerolled += _mediaPrerolled; _mediaEnded = new DispatcherOperationCallback(OnMediaEnded); this.DispatcherMediaEnded += _mediaEnded; _bufferingStarted = new DispatcherOperationCallback(OnBufferingStarted); this.DispatcherBufferingStarted += _bufferingStarted; _bufferingEnded = new DispatcherOperationCallback(OnBufferingEnded); this.DispatcherBufferingEnded += _bufferingEnded; _scriptCommand = new DispatcherOperationCallback(OnScriptCommand); this.DispatcherScriptCommand += _scriptCommand; _newFrame = new DispatcherOperationCallback(OnNewFrame); this.DispatcherMediaNewFrame += _newFrame; SetSender(mediaPlayer); } #endregion #region Static Methods ////// Create /// ////// Critical: This function hooks up and exposes the unmanaged proxy that /// sinks events from windows media /// [SecurityCritical] internal static void CreateMediaEventsHelper(MediaPlayer mediaPlayer, out MediaEventsHelper eventsHelper, out SafeMILHandle unmanagedProxy) { eventsHelper = new MediaEventsHelper(mediaPlayer); // Created with ref count = 1. Since this object does not hold on // to the unmanaged proxy, the lifetime is now controlled by whoever // called CreateMediaEventsHelper. unmanagedProxy = EventProxyWrapper.CreateEventProxyWrapper(eventsHelper); } #endregion #region Internal Methods / Properties / Events ////// Changes the sender of all events /// internal void SetSender(MediaPlayer sender) { Debug.Assert((sender != null), "Sender is null"); Debug.Assert((sender.Dispatcher != null), "Dispatcher is null"); _sender = sender; _dispatcher = sender.Dispatcher; } ////// Raised when error is encountered. /// internal event EventHandlerMediaFailed { add { _mediaFailedHelper.AddEvent(value); } remove { _mediaFailedHelper.RemoveEvent(value); } } /// /// Raised when media loading is complete /// internal event EventHandler MediaOpened { add { _mediaOpenedHelper.AddEvent(value); } remove { _mediaOpenedHelper.RemoveEvent(value); } } ////// Raised when media prerolling is complete /// internal event EventHandler MediaPrerolled { add { _mediaPrerolledHelper.AddEvent(value); } remove { _mediaPrerolledHelper.RemoveEvent(value); } } ////// Raised when media playback is finished /// internal event EventHandler MediaEnded { add { _mediaEndedHelper.AddEvent(value); } remove { _mediaEndedHelper.RemoveEvent(value); } } ////// Raised when buffering begins /// internal event EventHandler BufferingStarted { add { _bufferingStartedHelper.AddEvent(value); } remove { _bufferingStartedHelper.RemoveEvent(value); } } ////// Raised when buffering is complete /// internal event EventHandler BufferingEnded { add { _bufferingEndedHelper.AddEvent(value); } remove { _bufferingEndedHelper.RemoveEvent(value); } } ////// Raised when a script command that is embedded in the media is reached. /// internal event EventHandlerScriptCommand { add { _scriptCommandHelper.AddEvent(value); } remove { _scriptCommandHelper.RemoveEvent(value); } } /// /// Raised when a requested media frame is reached. /// internal event EventHandler NewFrame { add { _newFrameHelper.AddEvent(value); } remove { _newFrameHelper.RemoveEvent(value); } } internal void RaiseMediaFailed(Exception e) { if (DispatcherMediaFailed != null) { _dispatcher.BeginInvoke( DispatcherPriority.Normal, DispatcherMediaFailed, new ExceptionEventArgs(e)); } } #endregion #region IInvokable ////// Raises an event /// void IInvokable.RaiseEvent(byte[] buffer, int cb) { const int S_OK = 0; AVEvent avEventType = AVEvent.AVMediaNone; int failureHr = S_OK; int size = 0; // // Minumum size is the event enum, the error hresult and two // string lengths (as integers) // size = sizeof(int) * 4; // // The data could be larger, that is benign, in the case that we have // strings appended it will be. // if (cb < size) { Debug.Assert((cb == size), "Invalid event packet"); return; } MemoryStream memStream = new MemoryStream(buffer); using (BinaryReader reader = new BinaryReader(memStream)) { // // Unpack the event and the errorHResult // avEventType = (AVEvent)reader.ReadUInt32(); failureHr = (int)reader.ReadUInt32(); switch(avEventType) { case AVEvent.AVMediaOpened: if (DispatcherMediaOpened != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherMediaOpened, null); } break; case AVEvent.AVMediaFailed: RaiseMediaFailed(HRESULT.ConvertHRToException(failureHr)); break; case AVEvent.AVMediaBufferingStarted: if (DispatcherBufferingStarted != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherBufferingStarted, null); } break; case AVEvent.AVMediaBufferingEnded: if (DispatcherBufferingEnded != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherBufferingEnded, null); } break; case AVEvent.AVMediaEnded: if (DispatcherMediaEnded != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherMediaEnded, null); } break; case AVEvent.AVMediaPrerolled: if (DispatcherMediaPrerolled != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherMediaPrerolled, null); } break; case AVEvent.AVMediaScriptCommand: HandleScriptCommand(reader); break; case AVEvent.AVMediaNewFrame: if (DispatcherMediaNewFrame != null) { // // We set frame updates to background because media is high frequency and bandwidth enough // to interfere dramatically with input. // _dispatcher.BeginInvoke(DispatcherPriority.Background, DispatcherMediaNewFrame, null); } break; default: // // Default case intentionally not handled. // break; } } } #endregion #region Private Methods / Events ////// Handle the parsing of script commands coming up from media that /// supports them. Then binary reader at this point will be positioned /// at the first of the string lengths encoded in the structure. /// private void HandleScriptCommand( BinaryReader reader ) { int parameterTypeLength = (int)reader.ReadUInt32(); int parameterValueLength = (int)reader.ReadUInt32(); if (DispatcherScriptCommand != null) { string parameterType = GetStringFromReader(reader, parameterTypeLength); string parameterValue = GetStringFromReader(reader, parameterValueLength); _dispatcher.BeginInvoke( DispatcherPriority.Normal, DispatcherScriptCommand, new MediaScriptCommandEventArgs( parameterType, parameterValue)); } } ////// Reads in a sequence of unicode characters from a binary /// reader and inserts them into a StringBuilder. From this /// a string is returned. /// private string GetStringFromReader( BinaryReader reader, int stringLength ) { // // Set the initial capacity of the string builder to stringLength // StringBuilder stringBuilder = new StringBuilder(stringLength); // // Also set the actual length, this allows the string to be indexed // to that point. // stringBuilder.Length = stringLength; for(int i = 0; i < stringLength; i++) { stringBuilder[i] = (char)reader.ReadUInt16(); } return stringBuilder.ToString(); } ////// Media Opened event comes through here /// /// Null argument private object OnMediaOpened(object o) { _mediaOpenedHelper.InvokeEvents(_sender, null); return null; } ////// MediaPrerolled event comes through here /// /// Null argument private object OnMediaPrerolled(object o) { _mediaPrerolledHelper.InvokeEvents(_sender, null); return null; } ////// MediaEnded event comes through here /// /// Null argument private object OnMediaEnded(object o) { _mediaEndedHelper.InvokeEvents(_sender, null); return null; } ////// BufferingStarted event comes through here /// /// Null argument private object OnBufferingStarted(object o) { _bufferingStartedHelper.InvokeEvents(_sender, null); return null; } ////// BufferingEnded event comes through here /// /// Null argument private object OnBufferingEnded(object o) { _bufferingEndedHelper.InvokeEvents(_sender, null); return null; } ////// MediaFailed event comes through here /// /// EventArgs private object OnMediaFailed(object o) { ExceptionEventArgs e = (ExceptionEventArgs)o; _mediaFailedHelper.InvokeEvents(_sender, e); return null; } ////// Script commands come through here. /// /// EventArgs private object OnScriptCommand(object o) { MediaScriptCommandEventArgs e = (MediaScriptCommandEventArgs)o; _scriptCommandHelper.InvokeEvents(_sender, e); return null; } ////// New frames come through here. /// private object OnNewFrame(object e) { _newFrameHelper.InvokeEvents(_sender, null); return null; } ////// Raised by a media when it encounters an error. /// ////// Argument passed into the callback is a System.Exception /// private event DispatcherOperationCallback DispatcherMediaFailed; ////// Raised by a media when its done loading. /// private event DispatcherOperationCallback DispatcherMediaOpened; ////// Raised by a media when its done prerolling. /// private event DispatcherOperationCallback DispatcherMediaPrerolled; ////// Raised by a media when its done playback. /// private event DispatcherOperationCallback DispatcherMediaEnded; ////// Raised by a media when buffering begins. /// private event DispatcherOperationCallback DispatcherBufferingStarted; ////// Raised by a media when buffering finishes. /// private event DispatcherOperationCallback DispatcherBufferingEnded; ////// Raised by media when a particular scripting event is received. /// private event DispatcherOperationCallback DispatcherScriptCommand; ////// Raised whenever a new frame is displayed that has been requested. /// This is only required for effects. /// private event DispatcherOperationCallback DispatcherMediaNewFrame; #endregion #region Private Data Members ////// Sender of all events /// private MediaPlayer _sender; ////// Dispatcher of this object /// private Dispatcher _dispatcher; ////// for catching MediaOpened events /// private DispatcherOperationCallback _mediaOpened; ////// for catching MediaFailed events /// private DispatcherOperationCallback _mediaFailed; ////// for catching MediaPrerolled events /// private DispatcherOperationCallback _mediaPrerolled; ////// for catching MediaEnded events /// private DispatcherOperationCallback _mediaEnded; ////// for catching BufferingStarted events /// private DispatcherOperationCallback _bufferingStarted; ////// for catching BufferingEnded events /// private DispatcherOperationCallback _bufferingEnded; ////// for catching script command events. /// private DispatcherOperationCallback _scriptCommand; ////// For catching new frame notifications. /// private DispatcherOperationCallback _newFrame; ////// Helper for MediaFailed events /// private UniqueEventHelper_mediaFailedHelper = new UniqueEventHelper (); /// /// Helper for MediaOpened events /// private UniqueEventHelper _mediaOpenedHelper = new UniqueEventHelper(); ////// Helper for MediaPrerolled events /// private UniqueEventHelper _mediaPrerolledHelper = new UniqueEventHelper(); ////// Helper for MediaEnded events /// private UniqueEventHelper _mediaEndedHelper = new UniqueEventHelper(); ////// Helper for BufferingStarted events /// private UniqueEventHelper _bufferingStartedHelper = new UniqueEventHelper(); ////// Helper for BufferingEnded events /// private UniqueEventHelper _bufferingEndedHelper = new UniqueEventHelper(); ////// Helper for the script command events /// private UniqueEventHelper_scriptCommandHelper = new UniqueEventHelper (); /// /// Helper for the NewFrame events. /// private UniqueEventHelper _newFrameHelper = new UniqueEventHelper(); #endregion } #endregion }; // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: mediaeventshelper.cs // //----------------------------------------------------------------------------- using System; using System.Text; using System.Diagnostics; using System.ComponentModel; using MS.Internal; using System.Windows.Media.Animation; using System.Windows.Media; using System.Windows.Media.Composition; using System.Windows.Threading; using System.Runtime.InteropServices; using System.IO; using System.Collections; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { #region MediaEventsHelper ////// MediaEventsHelper /// Provides helper methods for media event related tasks /// internal class MediaEventsHelper : IInvokable { #region Constructors and Finalizers ////// Constructor /// internal MediaEventsHelper(MediaPlayer mediaPlayer) { _mediaOpened = new DispatcherOperationCallback(OnMediaOpened); this.DispatcherMediaOpened += _mediaOpened; _mediaFailed = new DispatcherOperationCallback(OnMediaFailed); this.DispatcherMediaFailed += _mediaFailed; _mediaPrerolled = new DispatcherOperationCallback(OnMediaPrerolled); this.DispatcherMediaPrerolled += _mediaPrerolled; _mediaEnded = new DispatcherOperationCallback(OnMediaEnded); this.DispatcherMediaEnded += _mediaEnded; _bufferingStarted = new DispatcherOperationCallback(OnBufferingStarted); this.DispatcherBufferingStarted += _bufferingStarted; _bufferingEnded = new DispatcherOperationCallback(OnBufferingEnded); this.DispatcherBufferingEnded += _bufferingEnded; _scriptCommand = new DispatcherOperationCallback(OnScriptCommand); this.DispatcherScriptCommand += _scriptCommand; _newFrame = new DispatcherOperationCallback(OnNewFrame); this.DispatcherMediaNewFrame += _newFrame; SetSender(mediaPlayer); } #endregion #region Static Methods ////// Create /// ////// Critical: This function hooks up and exposes the unmanaged proxy that /// sinks events from windows media /// [SecurityCritical] internal static void CreateMediaEventsHelper(MediaPlayer mediaPlayer, out MediaEventsHelper eventsHelper, out SafeMILHandle unmanagedProxy) { eventsHelper = new MediaEventsHelper(mediaPlayer); // Created with ref count = 1. Since this object does not hold on // to the unmanaged proxy, the lifetime is now controlled by whoever // called CreateMediaEventsHelper. unmanagedProxy = EventProxyWrapper.CreateEventProxyWrapper(eventsHelper); } #endregion #region Internal Methods / Properties / Events ////// Changes the sender of all events /// internal void SetSender(MediaPlayer sender) { Debug.Assert((sender != null), "Sender is null"); Debug.Assert((sender.Dispatcher != null), "Dispatcher is null"); _sender = sender; _dispatcher = sender.Dispatcher; } ////// Raised when error is encountered. /// internal event EventHandlerMediaFailed { add { _mediaFailedHelper.AddEvent(value); } remove { _mediaFailedHelper.RemoveEvent(value); } } /// /// Raised when media loading is complete /// internal event EventHandler MediaOpened { add { _mediaOpenedHelper.AddEvent(value); } remove { _mediaOpenedHelper.RemoveEvent(value); } } ////// Raised when media prerolling is complete /// internal event EventHandler MediaPrerolled { add { _mediaPrerolledHelper.AddEvent(value); } remove { _mediaPrerolledHelper.RemoveEvent(value); } } ////// Raised when media playback is finished /// internal event EventHandler MediaEnded { add { _mediaEndedHelper.AddEvent(value); } remove { _mediaEndedHelper.RemoveEvent(value); } } ////// Raised when buffering begins /// internal event EventHandler BufferingStarted { add { _bufferingStartedHelper.AddEvent(value); } remove { _bufferingStartedHelper.RemoveEvent(value); } } ////// Raised when buffering is complete /// internal event EventHandler BufferingEnded { add { _bufferingEndedHelper.AddEvent(value); } remove { _bufferingEndedHelper.RemoveEvent(value); } } ////// Raised when a script command that is embedded in the media is reached. /// internal event EventHandlerScriptCommand { add { _scriptCommandHelper.AddEvent(value); } remove { _scriptCommandHelper.RemoveEvent(value); } } /// /// Raised when a requested media frame is reached. /// internal event EventHandler NewFrame { add { _newFrameHelper.AddEvent(value); } remove { _newFrameHelper.RemoveEvent(value); } } internal void RaiseMediaFailed(Exception e) { if (DispatcherMediaFailed != null) { _dispatcher.BeginInvoke( DispatcherPriority.Normal, DispatcherMediaFailed, new ExceptionEventArgs(e)); } } #endregion #region IInvokable ////// Raises an event /// void IInvokable.RaiseEvent(byte[] buffer, int cb) { const int S_OK = 0; AVEvent avEventType = AVEvent.AVMediaNone; int failureHr = S_OK; int size = 0; // // Minumum size is the event enum, the error hresult and two // string lengths (as integers) // size = sizeof(int) * 4; // // The data could be larger, that is benign, in the case that we have // strings appended it will be. // if (cb < size) { Debug.Assert((cb == size), "Invalid event packet"); return; } MemoryStream memStream = new MemoryStream(buffer); using (BinaryReader reader = new BinaryReader(memStream)) { // // Unpack the event and the errorHResult // avEventType = (AVEvent)reader.ReadUInt32(); failureHr = (int)reader.ReadUInt32(); switch(avEventType) { case AVEvent.AVMediaOpened: if (DispatcherMediaOpened != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherMediaOpened, null); } break; case AVEvent.AVMediaFailed: RaiseMediaFailed(HRESULT.ConvertHRToException(failureHr)); break; case AVEvent.AVMediaBufferingStarted: if (DispatcherBufferingStarted != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherBufferingStarted, null); } break; case AVEvent.AVMediaBufferingEnded: if (DispatcherBufferingEnded != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherBufferingEnded, null); } break; case AVEvent.AVMediaEnded: if (DispatcherMediaEnded != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherMediaEnded, null); } break; case AVEvent.AVMediaPrerolled: if (DispatcherMediaPrerolled != null) { _dispatcher.BeginInvoke(DispatcherPriority.Normal, DispatcherMediaPrerolled, null); } break; case AVEvent.AVMediaScriptCommand: HandleScriptCommand(reader); break; case AVEvent.AVMediaNewFrame: if (DispatcherMediaNewFrame != null) { // // We set frame updates to background because media is high frequency and bandwidth enough // to interfere dramatically with input. // _dispatcher.BeginInvoke(DispatcherPriority.Background, DispatcherMediaNewFrame, null); } break; default: // // Default case intentionally not handled. // break; } } } #endregion #region Private Methods / Events ////// Handle the parsing of script commands coming up from media that /// supports them. Then binary reader at this point will be positioned /// at the first of the string lengths encoded in the structure. /// private void HandleScriptCommand( BinaryReader reader ) { int parameterTypeLength = (int)reader.ReadUInt32(); int parameterValueLength = (int)reader.ReadUInt32(); if (DispatcherScriptCommand != null) { string parameterType = GetStringFromReader(reader, parameterTypeLength); string parameterValue = GetStringFromReader(reader, parameterValueLength); _dispatcher.BeginInvoke( DispatcherPriority.Normal, DispatcherScriptCommand, new MediaScriptCommandEventArgs( parameterType, parameterValue)); } } ////// Reads in a sequence of unicode characters from a binary /// reader and inserts them into a StringBuilder. From this /// a string is returned. /// private string GetStringFromReader( BinaryReader reader, int stringLength ) { // // Set the initial capacity of the string builder to stringLength // StringBuilder stringBuilder = new StringBuilder(stringLength); // // Also set the actual length, this allows the string to be indexed // to that point. // stringBuilder.Length = stringLength; for(int i = 0; i < stringLength; i++) { stringBuilder[i] = (char)reader.ReadUInt16(); } return stringBuilder.ToString(); } ////// Media Opened event comes through here /// /// Null argument private object OnMediaOpened(object o) { _mediaOpenedHelper.InvokeEvents(_sender, null); return null; } ////// MediaPrerolled event comes through here /// /// Null argument private object OnMediaPrerolled(object o) { _mediaPrerolledHelper.InvokeEvents(_sender, null); return null; } ////// MediaEnded event comes through here /// /// Null argument private object OnMediaEnded(object o) { _mediaEndedHelper.InvokeEvents(_sender, null); return null; } ////// BufferingStarted event comes through here /// /// Null argument private object OnBufferingStarted(object o) { _bufferingStartedHelper.InvokeEvents(_sender, null); return null; } ////// BufferingEnded event comes through here /// /// Null argument private object OnBufferingEnded(object o) { _bufferingEndedHelper.InvokeEvents(_sender, null); return null; } ////// MediaFailed event comes through here /// /// EventArgs private object OnMediaFailed(object o) { ExceptionEventArgs e = (ExceptionEventArgs)o; _mediaFailedHelper.InvokeEvents(_sender, e); return null; } ////// Script commands come through here. /// /// EventArgs private object OnScriptCommand(object o) { MediaScriptCommandEventArgs e = (MediaScriptCommandEventArgs)o; _scriptCommandHelper.InvokeEvents(_sender, e); return null; } ////// New frames come through here. /// private object OnNewFrame(object e) { _newFrameHelper.InvokeEvents(_sender, null); return null; } ////// Raised by a media when it encounters an error. /// ////// Argument passed into the callback is a System.Exception /// private event DispatcherOperationCallback DispatcherMediaFailed; ////// Raised by a media when its done loading. /// private event DispatcherOperationCallback DispatcherMediaOpened; ////// Raised by a media when its done prerolling. /// private event DispatcherOperationCallback DispatcherMediaPrerolled; ////// Raised by a media when its done playback. /// private event DispatcherOperationCallback DispatcherMediaEnded; ////// Raised by a media when buffering begins. /// private event DispatcherOperationCallback DispatcherBufferingStarted; ////// Raised by a media when buffering finishes. /// private event DispatcherOperationCallback DispatcherBufferingEnded; ////// Raised by media when a particular scripting event is received. /// private event DispatcherOperationCallback DispatcherScriptCommand; ////// Raised whenever a new frame is displayed that has been requested. /// This is only required for effects. /// private event DispatcherOperationCallback DispatcherMediaNewFrame; #endregion #region Private Data Members ////// Sender of all events /// private MediaPlayer _sender; ////// Dispatcher of this object /// private Dispatcher _dispatcher; ////// for catching MediaOpened events /// private DispatcherOperationCallback _mediaOpened; ////// for catching MediaFailed events /// private DispatcherOperationCallback _mediaFailed; ////// for catching MediaPrerolled events /// private DispatcherOperationCallback _mediaPrerolled; ////// for catching MediaEnded events /// private DispatcherOperationCallback _mediaEnded; ////// for catching BufferingStarted events /// private DispatcherOperationCallback _bufferingStarted; ////// for catching BufferingEnded events /// private DispatcherOperationCallback _bufferingEnded; ////// for catching script command events. /// private DispatcherOperationCallback _scriptCommand; ////// For catching new frame notifications. /// private DispatcherOperationCallback _newFrame; ////// Helper for MediaFailed events /// private UniqueEventHelper_mediaFailedHelper = new UniqueEventHelper (); /// /// Helper for MediaOpened events /// private UniqueEventHelper _mediaOpenedHelper = new UniqueEventHelper(); ////// Helper for MediaPrerolled events /// private UniqueEventHelper _mediaPrerolledHelper = new UniqueEventHelper(); ////// Helper for MediaEnded events /// private UniqueEventHelper _mediaEndedHelper = new UniqueEventHelper(); ////// Helper for BufferingStarted events /// private UniqueEventHelper _bufferingStartedHelper = new UniqueEventHelper(); ////// Helper for BufferingEnded events /// private UniqueEventHelper _bufferingEndedHelper = new UniqueEventHelper(); ////// Helper for the script command events /// private UniqueEventHelper_scriptCommandHelper = new UniqueEventHelper (); /// /// Helper for the NewFrame events. /// private UniqueEventHelper _newFrameHelper = new UniqueEventHelper(); #endregion } #endregion }; // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
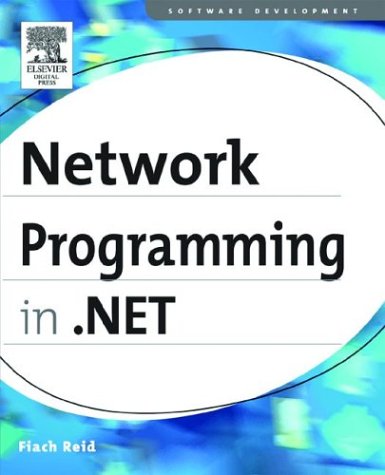
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AdCreatedEventArgs.cs
- CodeExporter.cs
- QuadraticBezierSegment.cs
- DataControlFieldCell.cs
- MetadataPropertyAttribute.cs
- DbConnectionClosed.cs
- UITypeEditors.cs
- OleDbException.cs
- XmlIncludeAttribute.cs
- PaperSource.cs
- ConfigurationValidatorAttribute.cs
- DictionaryEntry.cs
- InputScope.cs
- SymDocumentType.cs
- ReflectionUtil.cs
- XPathParser.cs
- ExpandCollapseProviderWrapper.cs
- SortedDictionary.cs
- FeatureManager.cs
- ToolStripItemCollection.cs
- ASCIIEncoding.cs
- BitmapSourceSafeMILHandle.cs
- ClientScriptManager.cs
- RightNameExpirationInfoPair.cs
- HtmlTernaryTree.cs
- HttpListenerContext.cs
- Transform.cs
- WindowsToolbarItemAsMenuItem.cs
- DataGridViewComboBoxEditingControl.cs
- SchemaTableOptionalColumn.cs
- SchemaConstraints.cs
- HashHelper.cs
- Vector3DIndependentAnimationStorage.cs
- MethodCallConverter.cs
- ControlType.cs
- SpotLight.cs
- SystemIPInterfaceStatistics.cs
- SqlConnectionPoolGroupProviderInfo.cs
- CodeTypeOfExpression.cs
- NavigationProperty.cs
- RelationalExpressions.cs
- WsdlBuildProvider.cs
- ElementMarkupObject.cs
- LocationSectionRecord.cs
- SafeBitVector32.cs
- SafeViewOfFileHandle.cs
- RenderContext.cs
- MatrixStack.cs
- XmlILAnnotation.cs
- OneOfElement.cs
- AppSecurityManager.cs
- BuildProvidersCompiler.cs
- AuthorizationRule.cs
- Brush.cs
- wgx_sdk_version.cs
- EditorZone.cs
- SafeHandle.cs
- JournalEntry.cs
- ConfigXmlElement.cs
- ServiceDescriptionData.cs
- TextWriterTraceListener.cs
- Soap.cs
- ModelUIElement3D.cs
- XPathParser.cs
- TextBox.cs
- WorkflowViewManager.cs
- GridSplitter.cs
- CompressedStack.cs
- DiagnosticsConfigurationHandler.cs
- PropertyNames.cs
- ToolBarButtonClickEvent.cs
- Currency.cs
- httpstaticobjectscollection.cs
- SqlTypesSchemaImporter.cs
- relpropertyhelper.cs
- MembershipPasswordException.cs
- SyndicationDeserializer.cs
- MediaSystem.cs
- UriTemplatePathSegment.cs
- GetWinFXPath.cs
- ExpandSegmentCollection.cs
- CriticalExceptions.cs
- DragEventArgs.cs
- EntityProxyTypeInfo.cs
- PartialClassGenerationTaskInternal.cs
- PreviousTrackingServiceAttribute.cs
- InstanceDataCollectionCollection.cs
- MultilineStringConverter.cs
- BitmapFrameEncode.cs
- OutputBuffer.cs
- ListViewCommandEventArgs.cs
- BinaryFormatter.cs
- AsyncOperation.cs
- UserCancellationException.cs
- Filter.cs
- DynamicRendererThreadManager.cs
- RevocationPoint.cs
- ScriptManagerProxy.cs
- AddInSegmentDirectoryNotFoundException.cs
- PolicyStatement.cs