Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / DragEventArgs.cs / 1 / DragEventArgs.cs
//---------------------------------------------------------------------------- // // File: DragEventArgs.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: DragEventArgs for drag-and-drop operation. // // // History: // 08/19/2004 : [....] Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// The DragEventArgs class represents a type of RoutedEventArgs that /// are relevant to all drag events(DragEnter/DragOver/DragLeave/DragDrop). /// public sealed class DragEventArgs : RoutedEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructs a DragEventArgs instance. /// /// /// The data object used in this drag drop operation. /// /// /// The current state of the mouse button and modifier keys. /// /// /// Allowed effects of a drag-and-drop operation. /// /// /// The target of the event. /// /// /// The current mouse position of the target. /// internal DragEventArgs(IDataObject data, DragDropKeyStates dragDropKeyStates, DragDropEffects allowedEffects, DependencyObject target, Point point) { if (!DragDrop.IsValidDragDropKeyStates(dragDropKeyStates)) { Debug.Assert(false, "Invalid dragDropKeyStates"); } if (!DragDrop.IsValidDragDropEffects(allowedEffects)) { Debug.Assert(false, "Invalid allowedEffects"); } if (target == null) { Debug.Assert(false, "Invalid target"); } this._data = data; this._dragDropKeyStates = dragDropKeyStates; this._allowedEffects = allowedEffects; this._target = target; this._dropPoint = point; this._effects = allowedEffects; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// The point of drop operation that based on relativeTo. /// public Point GetPosition(IInputElement relativeTo) { Point dropPoint; if (relativeTo == null) { throw new ArgumentNullException("relativeTo"); } dropPoint = new Point(0, 0); if (_target != null) { // Translate the drop point from the drop target to the relative element. dropPoint = InputElement.TranslatePoint(_dropPoint, _target, (DependencyObject)relativeTo); } return dropPoint; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// The data object of drop operation /// public IDataObject Data { get { return _data; } } ////// The DragDropKeyStates that indicates the current states for /// physical keyboard keys and mouse buttons. /// public DragDropKeyStates KeyStates { get { return _dragDropKeyStates; } } ////// The allowed effects of drag and drop operation /// public DragDropEffects AllowedEffects { get { return _allowedEffects; } } ////// The effects of drag and drop operation /// public DragDropEffects Effects { get { return _effects; } set { if (!DragDrop.IsValidDragDropEffects(value)) { throw new ArgumentException(SR.Get(SRID.DragDrop_DragDropEffectsInvalid, "value")); } _effects = value; } } #endregion Public Properties #region Protected Methods //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { DragEventHandler handler = (DragEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private IDataObject _data; private DragDropKeyStates _dragDropKeyStates; private DragDropEffects _allowedEffects; private DragDropEffects _effects; private DependencyObject _target; private Point _dropPoint; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
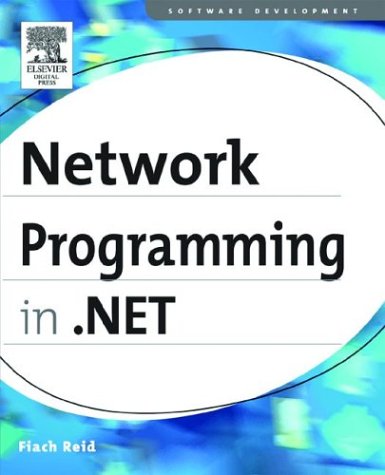
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaAttributeGroup.cs
- TableCell.cs
- WsdlServiceChannelBuilder.cs
- CodeTypeParameterCollection.cs
- XmlSubtreeReader.cs
- Point3DConverter.cs
- AnimatedTypeHelpers.cs
- SimpleMailWebEventProvider.cs
- CodeThrowExceptionStatement.cs
- TextBlock.cs
- XmlTypeMapping.cs
- Wildcard.cs
- DefaultTraceListener.cs
- WebPartEventArgs.cs
- GenerateTemporaryTargetAssembly.cs
- XmlSchemaObjectCollection.cs
- CommentAction.cs
- HttpFileCollection.cs
- SqlPersonalizationProvider.cs
- CommonObjectSecurity.cs
- SqlFormatter.cs
- DataGridViewHitTestInfo.cs
- WhiteSpaceTrimStringConverter.cs
- ImageField.cs
- ListViewItemSelectionChangedEvent.cs
- PieceNameHelper.cs
- CloudCollection.cs
- Dictionary.cs
- DbDataAdapter.cs
- FormViewPagerRow.cs
- DataSourceUtil.cs
- CompilerErrorCollection.cs
- ClientScriptManager.cs
- SessionState.cs
- TemplateControl.cs
- ObjectAnimationUsingKeyFrames.cs
- MatrixStack.cs
- AsymmetricSignatureFormatter.cs
- CharEntityEncoderFallback.cs
- SerializationSectionGroup.cs
- HtmlTitle.cs
- CompositeFontFamily.cs
- ListSortDescription.cs
- ApplicationActivator.cs
- XmlWrappingReader.cs
- Size.cs
- Label.cs
- Point4D.cs
- CodeArrayIndexerExpression.cs
- ProviderConnectionPointCollection.cs
- AttachedPropertiesService.cs
- TcpServerChannel.cs
- HttpModuleAction.cs
- DesignerView.xaml.cs
- ManagementInstaller.cs
- SoapTypeAttribute.cs
- PrintPreviewDialog.cs
- TagMapCollection.cs
- BindingNavigator.cs
- FileCodeGroup.cs
- BoolExpressionVisitors.cs
- SQLInt64.cs
- DetailsViewRow.cs
- CancellationTokenRegistration.cs
- ToolStrip.cs
- ServiceContractDetailViewControl.cs
- SqlFactory.cs
- TextServicesProperty.cs
- ObservableCollection.cs
- PackageFilter.cs
- Script.cs
- SapiAttributeParser.cs
- HttpTransportSecurityElement.cs
- StorageInfo.cs
- HtmlCalendarAdapter.cs
- Vector3dCollection.cs
- ItemsControl.cs
- RuntimeHelpers.cs
- ErrorRuntimeConfig.cs
- ValidatorUtils.cs
- MultilineStringConverter.cs
- Rules.cs
- SplitterCancelEvent.cs
- odbcmetadatacollectionnames.cs
- ExcludeFromCodeCoverageAttribute.cs
- CompModSwitches.cs
- WebRequestModuleElementCollection.cs
- LoadedEvent.cs
- ImportCatalogPart.cs
- UntypedNullExpression.cs
- srgsitem.cs
- BinaryFormatterWriter.cs
- ManifestSignedXml.cs
- CompoundFileStorageReference.cs
- XmlSchemaAppInfo.cs
- SymLanguageVendor.cs
- PrintDialogException.cs
- DataServiceContext.cs
- AutoSizeToolBoxItem.cs
- SetStoryboardSpeedRatio.cs