Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / Point4D.cs / 1305600 / Point4D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 4D point implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/04/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; namespace System.Windows.Media.Media3D { ////// Point4D - 4D point representation. /// Defaults to (0,0,0,0). /// public partial struct Point4D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor that sets point's initial values. /// /// Value of the X coordinate of the new point. /// Value of the Y coordinate of the new point. /// Value of the Z coordinate of the new point. /// Value of the W coordinate of the new point. public Point4D(double x, double y, double z, double w) { _x = x; _y = y; _z = z; _w = w; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Offset - update point position by adding deltaX to X, deltaY to Y, deltaZ to Z, and deltaW to W. /// /// Offset in the X direction. /// Offset in the Y direction. /// Offset in the Z direction. /// Offset in the W direction. public void Offset(double deltaX, double deltaY, double deltaZ, double deltaW) { _x += deltaX; _y += deltaY; _z += deltaZ; _w += deltaW; } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D operator +(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D Add(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D operator -(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D Subtract(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D operator *(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D Multiply(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
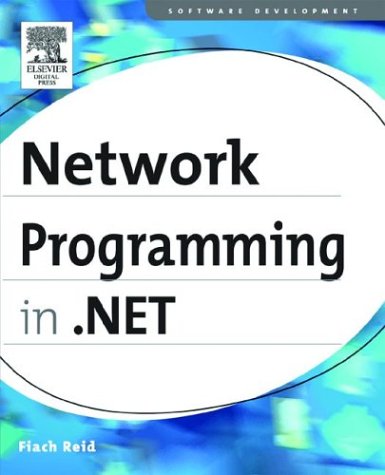
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompleteWizardStep.cs
- WindowsAuthenticationModule.cs
- DefaultParameterValueAttribute.cs
- NavigationPropertyEmitter.cs
- PropertyChangeTracker.cs
- StructuralComparisons.cs
- ValidatedControlConverter.cs
- IntSecurity.cs
- SingleBodyParameterMessageFormatter.cs
- RootBrowserWindowAutomationPeer.cs
- AsymmetricKeyExchangeFormatter.cs
- ConfigurationLocationCollection.cs
- ReferenceEqualityComparer.cs
- DefaultPropertyAttribute.cs
- DiagnosticsConfiguration.cs
- SimpleNameService.cs
- ThicknessAnimationBase.cs
- SqlBooleanizer.cs
- CacheMemory.cs
- RequestTimeoutManager.cs
- SubMenuStyle.cs
- MobileUserControlDesigner.cs
- InvalidPrinterException.cs
- Document.cs
- JsonStringDataContract.cs
- ElementFactory.cs
- QilInvokeEarlyBound.cs
- TraceHelpers.cs
- EndpointNotFoundException.cs
- AtomParser.cs
- ValueUtilsSmi.cs
- HttpModuleAction.cs
- TypedReference.cs
- Int64Animation.cs
- Span.cs
- CachedPathData.cs
- ResXResourceReader.cs
- KeyTime.cs
- WebPartEditorApplyVerb.cs
- CultureSpecificStringDictionary.cs
- SqlUnionizer.cs
- MaterialCollection.cs
- CodeSubDirectory.cs
- AccessorTable.cs
- AssociationProvider.cs
- ImportOptions.cs
- DataSvcMapFileSerializer.cs
- BamlStream.cs
- Html32TextWriter.cs
- StandardToolWindows.cs
- XamlFrame.cs
- InstanceLockQueryResult.cs
- TimelineCollection.cs
- XmlObjectSerializerContext.cs
- FixedElement.cs
- ClockGroup.cs
- Rectangle.cs
- Pair.cs
- WebPartEditorCancelVerb.cs
- Nodes.cs
- TypedTableBaseExtensions.cs
- BamlResourceDeserializer.cs
- TransactionContextValidator.cs
- InputMethod.cs
- UIHelper.cs
- CustomWebEventKey.cs
- SynchronizedDispatch.cs
- LexicalChunk.cs
- ConversionContext.cs
- UpdateCommand.cs
- DiffuseMaterial.cs
- QueryRelOp.cs
- DependencyObjectType.cs
- ResourceProviderFactory.cs
- DrawingContext.cs
- BuildProvidersCompiler.cs
- XamlToRtfParser.cs
- CommandBindingCollection.cs
- DataIdProcessor.cs
- TraceContextEventArgs.cs
- SecurityPolicySection.cs
- RawKeyboardInputReport.cs
- TargetConverter.cs
- StringCollection.cs
- MouseActionConverter.cs
- SafeCloseHandleCritical.cs
- LocalizabilityAttribute.cs
- Debugger.cs
- JobCollate.cs
- Repeater.cs
- AnonymousIdentificationModule.cs
- WindowsEditBoxRange.cs
- WebConvert.cs
- Rectangle.cs
- WindowsScroll.cs
- ToolStripRenderEventArgs.cs
- AddInEnvironment.cs
- invalidudtexception.cs
- XmlWriterSettings.cs
- SQLInt32Storage.cs