Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / HttpModuleAction.cs / 3 / HttpModuleAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Configuration; using System.Web.Configuration.Common; using System.Web.Util; using System.Globalization; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpModuleAction : ConfigurationElement { private static readonly ConfigurationElementProperty s_elemProperty = new ConfigurationElementProperty(new CallbackValidator(typeof(HttpModuleAction), Validate)); private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), String.Empty, ConfigurationPropertyOptions.IsRequired); private ModulesEntry _modualEntry; static HttpModuleAction() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propName); _properties.Add(_propType); } internal HttpModuleAction() { } public HttpModuleAction(String name, String type) : this() { Name = name; Type = type; _modualEntry = null; } internal string Key { get { return Name; } } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Name { get { return (string)base[_propName]; } set { base[_propName] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal string FileName { get { return ElementInformation.Properties["name"].Source; } } internal int LineNumber { get { return ElementInformation.Properties["name"].LineNumber; } } internal ModulesEntry Entry { get { try { if (_modualEntry == null) { _modualEntry = new ModulesEntry(Name, Type, _propType.Name, this); } return _modualEntry; } catch (Exception ex) { throw new ConfigurationErrorsException(ex.Message, ElementInformation.Properties[_propType.Name].Source, ElementInformation.Properties[_propType.Name].LineNumber); } } } internal static bool IsSpecialModule(String className) { return ModulesEntry.IsTypeMatch(typeof(System.Web.Security.DefaultAuthenticationModule), className); } internal static bool IsSpecialModuleName(String name) { return (StringUtil.EqualsIgnoreCase(name, "DefaultAuthentication")); } protected override ConfigurationElementProperty ElementProperty { get { return s_elemProperty; } } private static void Validate(object value) { if (value == null) { throw new ArgumentNullException("httpModule"); } HttpModuleAction elem = (HttpModuleAction)value; if (HttpModuleAction.IsSpecialModule(elem.Type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Special_module_cannot_be_added_manually, elem.Type), elem.ElementInformation.Properties["type"].Source, elem.ElementInformation.Properties["type"].LineNumber); } if (HttpModuleAction.IsSpecialModuleName(elem.Name)) { throw new ConfigurationErrorsException( SR.GetString(SR.Special_module_cannot_be_added_manually, elem.Name), elem.ElementInformation.Properties["name"].Source, elem.ElementInformation.Properties["name"].LineNumber); } } } // class HttpModule } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Configuration; using System.Web.Configuration.Common; using System.Web.Util; using System.Globalization; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpModuleAction : ConfigurationElement { private static readonly ConfigurationElementProperty s_elemProperty = new ConfigurationElementProperty(new CallbackValidator(typeof(HttpModuleAction), Validate)); private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), String.Empty, ConfigurationPropertyOptions.IsRequired); private ModulesEntry _modualEntry; static HttpModuleAction() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propName); _properties.Add(_propType); } internal HttpModuleAction() { } public HttpModuleAction(String name, String type) : this() { Name = name; Type = type; _modualEntry = null; } internal string Key { get { return Name; } } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Name { get { return (string)base[_propName]; } set { base[_propName] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal string FileName { get { return ElementInformation.Properties["name"].Source; } } internal int LineNumber { get { return ElementInformation.Properties["name"].LineNumber; } } internal ModulesEntry Entry { get { try { if (_modualEntry == null) { _modualEntry = new ModulesEntry(Name, Type, _propType.Name, this); } return _modualEntry; } catch (Exception ex) { throw new ConfigurationErrorsException(ex.Message, ElementInformation.Properties[_propType.Name].Source, ElementInformation.Properties[_propType.Name].LineNumber); } } } internal static bool IsSpecialModule(String className) { return ModulesEntry.IsTypeMatch(typeof(System.Web.Security.DefaultAuthenticationModule), className); } internal static bool IsSpecialModuleName(String name) { return (StringUtil.EqualsIgnoreCase(name, "DefaultAuthentication")); } protected override ConfigurationElementProperty ElementProperty { get { return s_elemProperty; } } private static void Validate(object value) { if (value == null) { throw new ArgumentNullException("httpModule"); } HttpModuleAction elem = (HttpModuleAction)value; if (HttpModuleAction.IsSpecialModule(elem.Type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Special_module_cannot_be_added_manually, elem.Type), elem.ElementInformation.Properties["type"].Source, elem.ElementInformation.Properties["type"].LineNumber); } if (HttpModuleAction.IsSpecialModuleName(elem.Name)) { throw new ConfigurationErrorsException( SR.GetString(SR.Special_module_cannot_be_added_manually, elem.Name), elem.ElementInformation.Properties["name"].Source, elem.ElementInformation.Properties["name"].LineNumber); } } } // class HttpModule } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
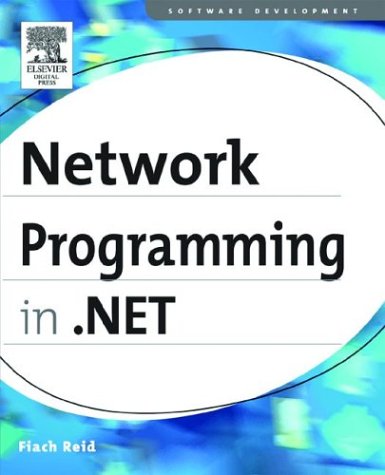
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IPHostEntry.cs
- XmlSchemaInferenceException.cs
- ToolStripInSituService.cs
- XomlCompilerResults.cs
- Message.cs
- Literal.cs
- ConvertBinder.cs
- RotateTransform3D.cs
- DataGridRelationshipRow.cs
- KeyToListMap.cs
- BitmapEffectGroup.cs
- ScrollBar.cs
- SerializationHelper.cs
- DeflateEmulationStream.cs
- StylusCollection.cs
- XmlChildEnumerator.cs
- FamilyTypeface.cs
- HandleRef.cs
- TagMapInfo.cs
- PlanCompiler.cs
- ColumnCollection.cs
- WindowsListView.cs
- MenuAdapter.cs
- XPathSelectionIterator.cs
- entityreference_tresulttype.cs
- RawStylusInput.cs
- JsonDeserializer.cs
- WebPageTraceListener.cs
- AccessDataSourceView.cs
- ContentOperations.cs
- DataSourceCache.cs
- CompilationSection.cs
- RSACryptoServiceProvider.cs
- __Error.cs
- RemoteWebConfigurationHostServer.cs
- Cursor.cs
- ImageSource.cs
- KerberosReceiverSecurityToken.cs
- XmlUTF8TextReader.cs
- AffineTransform3D.cs
- QilInvokeLateBound.cs
- DetailsViewInsertEventArgs.cs
- TableLayoutPanel.cs
- CodeTypeConstructor.cs
- TraceContextEventArgs.cs
- MethodToken.cs
- MessageContractExporter.cs
- BitmapPalette.cs
- XPathArrayIterator.cs
- StackSpiller.Bindings.cs
- Opcode.cs
- QuadraticBezierSegment.cs
- EmbeddedMailObjectsCollection.cs
- HandlerMappingMemo.cs
- MarkupCompilePass2.cs
- FormsAuthenticationConfiguration.cs
- Assert.cs
- WebConfigurationHostFileChange.cs
- DataGridViewButtonCell.cs
- OdbcDataAdapter.cs
- InputProcessorProfilesLoader.cs
- DataControlFieldHeaderCell.cs
- OperationValidationEventArgs.cs
- DateTimeConverter.cs
- NullReferenceException.cs
- NonValidatingSecurityTokenAuthenticator.cs
- ToolConsole.cs
- MatrixKeyFrameCollection.cs
- XmlSchemaNotation.cs
- RefExpr.cs
- ResetableIterator.cs
- XmlDownloadManager.cs
- ZipIOLocalFileHeader.cs
- ArraySubsetEnumerator.cs
- arclist.cs
- assertwrapper.cs
- DbConnectionClosed.cs
- MD5CryptoServiceProvider.cs
- ISAPIRuntime.cs
- CodeTypeReference.cs
- HttpResponseHeader.cs
- XamlPointCollectionSerializer.cs
- Margins.cs
- TypeConverter.cs
- TdsRecordBufferSetter.cs
- TraceHwndHost.cs
- WebAdminConfigurationHelper.cs
- bidPrivateBase.cs
- SynchronizedPool.cs
- NetworkAddressChange.cs
- AxWrapperGen.cs
- CommonDialog.cs
- HostingPreferredMapPath.cs
- sqlinternaltransaction.cs
- BindingGroup.cs
- WindowsListBox.cs
- AxisAngleRotation3D.cs
- Semaphore.cs
- EndpointInfo.cs
- AuthenticationException.cs