Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / FamilyTypeface.cs / 1305600 / FamilyTypeface.cs
//+------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: FamilyTypeface.cs // // Contents: FamilyTypeface implementation // // Spec: http://team/sites/Avalon/Specs/Fonts.htm // // Created: 11-11-2003 Tarek Mahmoud Sayed ([....]) // //----------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.ComponentModel; using System.Windows.Markup; // for XmlLanguage using MS.Internal.FontFace; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// The FamilyTypeface object specifies the details of a single typeface supported by a /// FontFamily. There are as many FamilyTypeface objects as there are typefaces supported. /// public class FamilyTypeface : IDeviceFont, ITypefaceMetrics { ////// Construct a default family typeface /// public FamilyTypeface() {} ////// Construct a read-only FamilyTypeface from a Typeface. /// internal FamilyTypeface(Typeface face) { _style = face.Style; _weight = face.Weight; _stretch = face.Stretch; _underlinePosition = face.UnderlinePosition; _underlineThickness = face.UnderlineThickness; _strikeThroughPosition = face.StrikethroughPosition; _strikeThroughThickness = face.StrikethroughThickness; _capsHeight = face.CapsHeight; _xHeight = face.XHeight; _readOnly = true; } ////// Typeface style /// public FontStyle Style { get { return _style; } set { VerifyChangeable(); _style = value; } } ////// Typeface weight /// public FontWeight Weight { get { return _weight; } set { VerifyChangeable(); _weight = value; } } ////// Typeface stretch /// public FontStretch Stretch { get { return _stretch; } set { VerifyChangeable(); _stretch = value; } } ////// Typeface underline position in EM relative to baseline /// public double UnderlinePosition { get { return _underlinePosition ; } set { CompositeFontParser.VerifyMultiplierOfEm("UnderlinePosition", ref value); VerifyChangeable(); _underlinePosition = value; } } ////// Typeface underline thickness in EM /// public double UnderlineThickness { get { return _underlineThickness; } set { CompositeFontParser.VerifyPositiveMultiplierOfEm("UnderlineThickness", ref value); VerifyChangeable(); _underlineThickness = value; } } ////// Typeface strikethrough position in EM relative to baseline /// public double StrikethroughPosition { get { return _strikeThroughPosition; } set { CompositeFontParser.VerifyMultiplierOfEm("StrikethroughPosition", ref value); VerifyChangeable(); _strikeThroughPosition = value; } } ////// Typeface strikethrough thickness in EM /// public double StrikethroughThickness { get { return _strikeThroughThickness; } set { CompositeFontParser.VerifyPositiveMultiplierOfEm("StrikethroughThickness", ref value); VerifyChangeable(); _strikeThroughThickness = value; } } ////// Typeface caps height in EM /// public double CapsHeight { get { return _capsHeight; } set { CompositeFontParser.VerifyPositiveMultiplierOfEm("CapsHeight", ref value); VerifyChangeable(); _capsHeight = value; } } ////// Typeface X-height in EM /// public double XHeight { get { return _xHeight; } set { CompositeFontParser.VerifyPositiveMultiplierOfEm("XHeight", ref value); VerifyChangeable(); _xHeight = value; } } ////// Flag indicate whether this is symbol typeface; always false for FamilyTypeface. /// bool ITypefaceMetrics.Symbol { get { return false; } } ////// Style simulation flags for this typeface. /// StyleSimulations ITypefaceMetrics.StyleSimulations { get { return StyleSimulations.None; } } ////// Collection of localized face names adjusted by the font differentiator. /// public IDictionaryAdjustedFaceNames { get { return FontDifferentiator.ConstructFaceNamesByStyleWeightStretch(_style, _weight, _stretch); } } /// /// Compares two typefaces for equality; returns true if the specified typeface /// is not null and has the same properties as this typeface. /// public bool Equals(FamilyTypeface typeface) { if (typeface == null) return false; return ( Style == typeface.Style && Weight == typeface.Weight && Stretch == typeface.Stretch ); } ////// Name or unique identifier of a device font. /// public string DeviceFontName { get { return _deviceFontName; } set { VerifyChangeable(); _deviceFontName = value; } } ////// Collection of character metrics for a device font. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public CharacterMetricsDictionary DeviceFontCharacterMetrics { get { if (_characterMetrics == null) { _characterMetrics = new CharacterMetricsDictionary(); } return _characterMetrics; } } ////// public override bool Equals(object o) { return Equals(o as FamilyTypeface); } ////// /// public override int GetHashCode() { return _style.GetHashCode() ^ _weight.GetHashCode() ^ _stretch.GetHashCode(); } private void VerifyChangeable() { if (_readOnly) throw new NotSupportedException(SR.Get(SRID.General_ObjectIsReadOnly)); } string IDeviceFont.Name { get { return _deviceFontName; } } bool IDeviceFont.ContainsCharacter(int unicodeScalar) { return _characterMetrics != null && _characterMetrics.GetValue(unicodeScalar) != null; } ////// /// Critical - As it uses raw pointers. /// [SecurityCritical] unsafe void IDeviceFont.GetAdvanceWidths( char* characterString, int characterLength, double emSize, int* pAdvances ) { unsafe { for (int i = 0; i < characterLength; ++i) { CharacterMetrics metrics = _characterMetrics.GetValue(characterString[i]); if (metrics != null) { // Side bearings are included in the advance width but are not used as offsets for glyph positioning. pAdvances[i] = Math.Max(0, (int)((metrics.BlackBoxWidth + metrics.LeftSideBearing + metrics.RightSideBearing) * emSize)); } else { pAdvances[i] = 0; } } } } private bool _readOnly; private FontStyle _style; private FontWeight _weight; private FontStretch _stretch; private double _underlinePosition; private double _underlineThickness; private double _strikeThroughPosition; private double _strikeThroughThickness; private double _capsHeight; private double _xHeight; private string _deviceFontName; private CharacterMetricsDictionary _characterMetrics; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
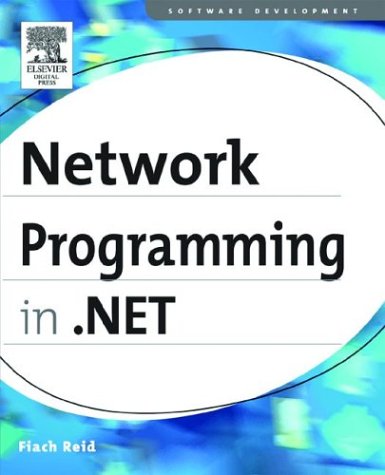
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnsignedPublishLicense.cs
- UserControl.cs
- DataGridViewBindingCompleteEventArgs.cs
- ComplexObject.cs
- XPathSingletonIterator.cs
- FlowLayoutSettings.cs
- XmlEntity.cs
- AssemblyCacheEntry.cs
- SqlStream.cs
- BindingExpression.cs
- LogLogRecordHeader.cs
- WebExceptionStatus.cs
- XmlSchemaAnnotation.cs
- _AutoWebProxyScriptEngine.cs
- XmlDataFileEditor.cs
- UnknownBitmapEncoder.cs
- SiteMapNode.cs
- OutputScopeManager.cs
- DataAccessor.cs
- InvokePatternIdentifiers.cs
- SynchronizingStream.cs
- DataGridViewHeaderCell.cs
- SystemFonts.cs
- AsymmetricKeyExchangeFormatter.cs
- RadioButton.cs
- WindowsMenu.cs
- ClientSession.cs
- ListViewInsertionMark.cs
- MorphHelpers.cs
- ResourceReader.cs
- SelectionRange.cs
- HasCopySemanticsAttribute.cs
- SqlCacheDependencySection.cs
- PageCatalogPart.cs
- PagerSettings.cs
- CookieParameter.cs
- FormViewCommandEventArgs.cs
- HtmlDocument.cs
- TempEnvironment.cs
- StreamWriter.cs
- ConfigXmlCDataSection.cs
- ExcCanonicalXml.cs
- StringHandle.cs
- RectangleF.cs
- EventPrivateKey.cs
- GridViewUpdatedEventArgs.cs
- Point3D.cs
- WebPartConnectionsCancelEventArgs.cs
- DataGridViewBindingCompleteEventArgs.cs
- TextFormatterImp.cs
- ThousandthOfEmRealDoubles.cs
- ServicePointManager.cs
- ProfessionalColors.cs
- PersistenceProvider.cs
- TextRenderer.cs
- returneventsaver.cs
- XmlObjectSerializerWriteContext.cs
- FormViewUpdateEventArgs.cs
- DictionaryItemsCollection.cs
- ArcSegment.cs
- DeclarationUpdate.cs
- ItemAutomationPeer.cs
- MetadataCollection.cs
- PingReply.cs
- OrthographicCamera.cs
- BitmapEffectOutputConnector.cs
- ConstraintStruct.cs
- StringFunctions.cs
- GridView.cs
- KerberosReceiverSecurityToken.cs
- ClassicBorderDecorator.cs
- ZipIOExtraFieldPaddingElement.cs
- SystemIPv6InterfaceProperties.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- WindowsToolbarItemAsMenuItem.cs
- ApplicationBuildProvider.cs
- ToolStripCollectionEditor.cs
- ProxyGenerator.cs
- MostlySingletonList.cs
- TdsRecordBufferSetter.cs
- ServiceModelSectionGroup.cs
- ModifierKeysValueSerializer.cs
- UTF7Encoding.cs
- OleAutBinder.cs
- CodeNamespaceCollection.cs
- TextHidden.cs
- RegexTree.cs
- SlipBehavior.cs
- ReversePositionQuery.cs
- UserControlParser.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- WebPageTraceListener.cs
- CustomAttribute.cs
- Point4DConverter.cs
- HttpCookiesSection.cs
- CodeChecksumPragma.cs
- SEHException.cs
- CustomAttributeSerializer.cs
- IPEndPointCollection.cs
- DropDownList.cs