Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / GDI / TextRenderer.cs / 1 / TextRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Internal; using System; using System.Drawing; using System.Windows.Forms.Internal; using System.Diagnostics; ////// /// public sealed class TextRenderer { //cannot instantiate private TextRenderer() { } ////// This class provides API for drawing GDI text. /// ///public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText( text, wf, bounds, foreColor, GetIntTextFormatFlags( flags ) ); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText(text, wf, bounds, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } private static IntTextFormatFlags GetIntTextFormatFlags(TextFormatFlags flags) { if( ((uint)flags & WindowsGraphics.GdiUnsupportedFlagMask) == 0 ) { return (IntTextFormatFlags) flags; } // Clear TextRenderer custom flags. IntTextFormatFlags windowsGraphicsSupportedFlags = (IntTextFormatFlags) ( ((uint)flags) & ~WindowsGraphics.GdiUnsupportedFlagMask ); return windowsGraphicsSupportedFlags; } /// MeasureText wrappers. public static Size MeasureText(string text, Font font ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf); } } public static Size MeasureText(string text, Font font, Size proposedSize ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, WindowsGraphicsCacheManager.GetWindowsFont(font), proposedSize); } } public static Size MeasureText(string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } public static Size MeasureText(IDeviceContext dc, string text, Font font) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf, proposedSize); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using (WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper(dc, flags)) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wgr.WindowsGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } } internal static Color DisabledTextColor(Color backColor) { //Theme specs -- if the backcolor is darker than Control, we use // ControlPaint.Dark(backcolor). Otherwise we use ControlDark. // see VS#357226 Color disabledTextForeColor = SystemColors.ControlDark; if (ControlPaint.IsDarker(backColor, SystemColors.Control)) { disabledTextForeColor = ControlPaint.Dark(backColor); } return disabledTextForeColor; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Internal; using System; using System.Drawing; using System.Windows.Forms.Internal; using System.Diagnostics; ////// /// public sealed class TextRenderer { //cannot instantiate private TextRenderer() { } ////// This class provides API for drawing GDI text. /// ///public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText( text, wf, bounds, foreColor, GetIntTextFormatFlags( flags ) ); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText(text, wf, bounds, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } private static IntTextFormatFlags GetIntTextFormatFlags(TextFormatFlags flags) { if( ((uint)flags & WindowsGraphics.GdiUnsupportedFlagMask) == 0 ) { return (IntTextFormatFlags) flags; } // Clear TextRenderer custom flags. IntTextFormatFlags windowsGraphicsSupportedFlags = (IntTextFormatFlags) ( ((uint)flags) & ~WindowsGraphics.GdiUnsupportedFlagMask ); return windowsGraphicsSupportedFlags; } /// MeasureText wrappers. public static Size MeasureText(string text, Font font ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf); } } public static Size MeasureText(string text, Font font, Size proposedSize ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, WindowsGraphicsCacheManager.GetWindowsFont(font), proposedSize); } } public static Size MeasureText(string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } public static Size MeasureText(IDeviceContext dc, string text, Font font) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf, proposedSize); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using (WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper(dc, flags)) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wgr.WindowsGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } } internal static Color DisabledTextColor(Color backColor) { //Theme specs -- if the backcolor is darker than Control, we use // ControlPaint.Dark(backcolor). Otherwise we use ControlDark. // see VS#357226 Color disabledTextForeColor = SystemColors.ControlDark; if (ControlPaint.IsDarker(backColor, SystemColors.Control)) { disabledTextForeColor = ControlPaint.Dark(backColor); } return disabledTextForeColor; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
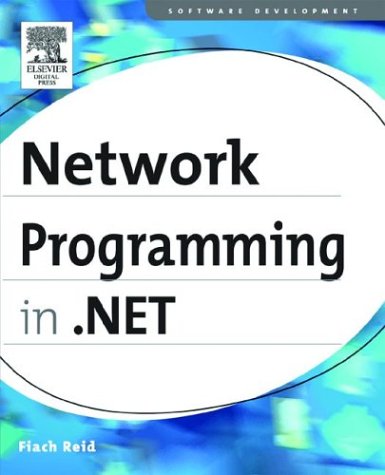
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThumbAutomationPeer.cs
- TextTreeRootTextBlock.cs
- WindowsImpersonationContext.cs
- DependencySource.cs
- Root.cs
- Viewport3DVisual.cs
- ConfigXmlElement.cs
- WorkflowServiceBehavior.cs
- SingletonInstanceContextProvider.cs
- IdnElement.cs
- PageClientProxyGenerator.cs
- VisualTreeHelper.cs
- ReferenceEqualityComparer.cs
- LabelEditEvent.cs
- XPathDocumentBuilder.cs
- CharUnicodeInfo.cs
- SynchronizingStream.cs
- Logging.cs
- WebPartDisplayModeCollection.cs
- HttpRequestBase.cs
- Point4DConverter.cs
- XDRSchema.cs
- x509utils.cs
- AssignDesigner.xaml.cs
- LinkTarget.cs
- PerformanceCounterPermissionEntry.cs
- SkipStoryboardToFill.cs
- XmlEntityReference.cs
- UnknownWrapper.cs
- Serialization.cs
- ContentTypeSettingClientMessageFormatter.cs
- XmlLoader.cs
- SmtpReplyReaderFactory.cs
- SiteMapDataSourceView.cs
- AdRotator.cs
- SecureUICommand.cs
- XsltCompileContext.cs
- WebPartsPersonalizationAuthorization.cs
- QuadraticBezierSegment.cs
- PEFileReader.cs
- Win32.cs
- FacetChecker.cs
- RadioButtonAutomationPeer.cs
- ImpersonationContext.cs
- Normalizer.cs
- TreeView.cs
- CompensableActivity.cs
- ClientBuildManagerCallback.cs
- PreProcessor.cs
- Variable.cs
- CodeDomSerializationProvider.cs
- XmlNullResolver.cs
- PasswordRecovery.cs
- TabControl.cs
- CircleHotSpot.cs
- SQLDouble.cs
- RepeatInfo.cs
- FixedNode.cs
- EditorPartChrome.cs
- PeerCustomResolverElement.cs
- WriteTimeStream.cs
- ServiceReference.cs
- SmtpReplyReaderFactory.cs
- BoundPropertyEntry.cs
- TracedNativeMethods.cs
- ModelPropertyCollectionImpl.cs
- Typography.cs
- QueryOperatorEnumerator.cs
- AdornerLayer.cs
- MdiWindowListStrip.cs
- PageCodeDomTreeGenerator.cs
- SyndicationDeserializer.cs
- RemotingAttributes.cs
- Documentation.cs
- Trigger.cs
- Menu.cs
- HostedNamedPipeTransportManager.cs
- CodeValidator.cs
- MimeFormatExtensions.cs
- DataGridRow.cs
- BitmapImage.cs
- TrackingConditionCollection.cs
- EntityViewGenerationAttribute.cs
- QueueProcessor.cs
- RepeaterItem.cs
- DCSafeHandle.cs
- XPathDocument.cs
- TargetParameterCountException.cs
- FileSystemInfo.cs
- TextEditorTables.cs
- ConnectionOrientedTransportChannelFactory.cs
- TabControlCancelEvent.cs
- DesignerForm.cs
- WebPartConnectionsConnectVerb.cs
- MdiWindowListStrip.cs
- RoleGroupCollection.cs
- CultureTable.cs
- ThemeInfoAttribute.cs
- SeparatorAutomationPeer.cs
- SHA1Managed.cs