Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Principal / WindowsImpersonationContext.cs / 1 / WindowsImpersonationContext.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // WindowsImpersonationContext.cs // // Representation of an impersonation context. // namespace System.Security.Principal { using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Runtime.ConstrainedExecution; [System.Runtime.InteropServices.ComVisible(true)] public class WindowsImpersonationContext : IDisposable { private SafeTokenHandle m_safeTokenHandle = SafeTokenHandle.InvalidHandle; private WindowsIdentity m_wi; private FrameSecurityDescriptor m_fsd; private WindowsImpersonationContext () {} internal WindowsImpersonationContext (SafeTokenHandle safeTokenHandle, WindowsIdentity wi, bool isImpersonating, FrameSecurityDescriptor fsd) { // make this a no-op on Win98 so calling code does not have to special case down-level platforms. if (WindowsIdentity.RunningOnWin2K) { if (safeTokenHandle.IsInvalid) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidImpersonationToken")); if (isImpersonating) { if (!Win32Native.DuplicateHandle(Win32Native.GetCurrentProcess(), safeTokenHandle, Win32Native.GetCurrentProcess(), ref m_safeTokenHandle, 0, true, Win32Native.DUPLICATE_SAME_ACCESS)) throw new SecurityException(Win32Native.GetMessage(Marshal.GetLastWin32Error())); m_wi = wi; } m_fsd = fsd; } } // Revert to previous impersonation (the only public method). public void Undo () { // make this a no-op on Win98 so calling code does not have to special case down-level platforms. if (!WindowsIdentity.RunningOnWin2K) return; int hr = 0; if (m_safeTokenHandle.IsInvalid) { // the thread was not initially impersonating hr = Win32.RevertToSelf(); if (hr < 0) throw new SecurityException(Win32Native.GetMessage(hr)); } else { hr = Win32.RevertToSelf(); if (hr < 0) throw new SecurityException(Win32Native.GetMessage(hr)); hr = Win32.ImpersonateLoggedOnUser(m_safeTokenHandle); if (hr < 0) throw new SecurityException(Win32Native.GetMessage(hr)); } WindowsIdentity.UpdateThreadWI(m_wi); if (m_fsd != null) m_fsd.SetTokenHandles(null, null); } // Non-throwing version that does not new any exception objects. To be called when reliability matters [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] internal bool UndoNoThrow() { bool bRet = false; try{ // make this a no-op on Win98 so calling code does not have to special case down-level platforms. if (!WindowsIdentity.RunningOnWin2K) return true; int hr = 0; if (m_safeTokenHandle.IsInvalid) { // the thread was not initially impersonating hr = Win32.RevertToSelf(); } else { hr = Win32.RevertToSelf(); if (hr >= 0) hr = Win32.ImpersonateLoggedOnUser(m_safeTokenHandle); } bRet = (hr >= 0); if (m_fsd != null) m_fsd.SetTokenHandles(null,null); } catch { bRet = false; } return bRet; } // // IDisposable interface. // [ComVisible(false)] protected virtual void Dispose(bool disposing) { if (disposing) { if (m_safeTokenHandle != null && !m_safeTokenHandle.IsClosed) { Undo(); m_safeTokenHandle.Dispose(); } } } [ComVisible(false)] public void Dispose () { Dispose(true); } } }
Link Menu
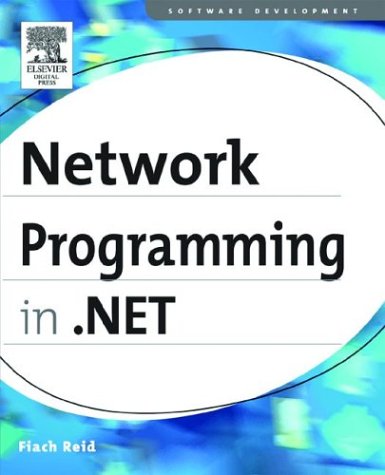
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PLINQETWProvider.cs
- AudioException.cs
- XPathMessageContext.cs
- TimeSpanConverter.cs
- NativeMethods.cs
- ToolStripItemGlyph.cs
- PropertyChangedEventManager.cs
- MaskedTextBoxDesigner.cs
- PaintValueEventArgs.cs
- TCEAdapterGenerator.cs
- sapiproxy.cs
- EntityDescriptor.cs
- _PooledStream.cs
- ReferencedAssembly.cs
- DbParameterHelper.cs
- ExpanderAutomationPeer.cs
- Context.cs
- Workspace.cs
- ServiceRoute.cs
- wgx_sdk_version.cs
- XmlParserContext.cs
- ListViewGroupConverter.cs
- DirectionalLight.cs
- PasswordRecovery.cs
- PathTooLongException.cs
- URLEditor.cs
- PersonalizationDictionary.cs
- RuntimeCompatibilityAttribute.cs
- List.cs
- LineGeometry.cs
- GroupAggregateExpr.cs
- ExpressionHelper.cs
- RoutedCommand.cs
- DescendentsWalkerBase.cs
- FactoryGenerator.cs
- COAUTHIDENTITY.cs
- XmlWrappingWriter.cs
- NamespaceInfo.cs
- RSAPKCS1KeyExchangeFormatter.cs
- TypedTableBaseExtensions.cs
- SimpleRecyclingCache.cs
- UnlockCardRequest.cs
- AuthenticationService.cs
- TabPage.cs
- AspNetCompatibilityRequirementsAttribute.cs
- QilIterator.cs
- DataGridViewUtilities.cs
- ArrayWithOffset.cs
- SplitterCancelEvent.cs
- CompiledRegexRunnerFactory.cs
- DescendentsWalkerBase.cs
- safelink.cs
- GenericXmlSecurityToken.cs
- CornerRadiusConverter.cs
- EntityContainerAssociationSet.cs
- XsltLibrary.cs
- TypeDefinition.cs
- GridViewItemAutomationPeer.cs
- Int32Storage.cs
- RecognizerInfo.cs
- FormClosingEvent.cs
- OleDbMetaDataFactory.cs
- PauseStoryboard.cs
- SqlCachedBuffer.cs
- MethodAccessException.cs
- WmpBitmapEncoder.cs
- SimpleWorkerRequest.cs
- HttpDictionary.cs
- HitTestFilterBehavior.cs
- _UriSyntax.cs
- ContainerControlDesigner.cs
- XmlQualifiedNameTest.cs
- HotCommands.cs
- ProgressBar.cs
- TransformConverter.cs
- CheckableControlBaseAdapter.cs
- HttpWriter.cs
- Zone.cs
- BufferModeSettings.cs
- KeyboardNavigation.cs
- WebEvents.cs
- BlockExpression.cs
- querybuilder.cs
- MethodBody.cs
- HttpCacheVary.cs
- EmptyStringExpandableObjectConverter.cs
- ResolveDuplexAsyncResult.cs
- XmlComplianceUtil.cs
- DataServiceClientException.cs
- DurableMessageDispatchInspector.cs
- Vector3DAnimationBase.cs
- ColorPalette.cs
- StylusPoint.cs
- BigInt.cs
- StreamDocument.cs
- FontWeightConverter.cs
- ProfileSettings.cs
- TreeViewImageKeyConverter.cs
- ArraySegment.cs
- BlurBitmapEffect.cs