Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / InlinedAggregationOperatorEnumerator.cs / 1305376 / InlinedAggregationOperatorEnumerator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // InlinedAggregationOperatorEnumerator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Threading; namespace System.Linq.Parallel { //---------------------------------------------------------------------------------------- // Inlined aggregate operators for finding the min/max values for primitives (int, long, float, // double, decimal). Versions are also offered for the nullable primitives (int?, long?, float?, // double?, decimal?), which differ slightly in behavior: they return a null value for empty // streams, whereas the ordinary primitive versions throw. // //--------------------------------------------------------------------------------------- // Inlined average operators for primitives (int, long, float, double, decimal), and the // nullable variants. The difference between the nromal and nullable variety is that // nulls are skipped in tallying the count and sum for the average. // ////// A class with some shared implementation between all aggregation enumerators. /// ///internal abstract class InlinedAggregationOperatorEnumerator : QueryOperatorEnumerator { private int m_partitionIndex; // This partition's unique index. protected CancellationToken m_cancellationToken; //--------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal InlinedAggregationOperatorEnumerator(int partitionIndex, CancellationToken cancellationToken) { m_partitionIndex = partitionIndex; m_cancellationToken = cancellationToken; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. There is a boilerplate variant used by callers, // and then one that is used for extensibility by subclasses. // internal sealed override bool MoveNext(ref TIntermediate currentElement, ref int currentKey) { if (MoveNextCore(ref currentElement)) { // A reduction's "index" is the same as its partition number. currentKey = m_partitionIndex; return true; } return false; } protected abstract bool MoveNextCore(ref TIntermediate currentElement); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // InlinedAggregationOperatorEnumerator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Threading; namespace System.Linq.Parallel { //---------------------------------------------------------------------------------------- // Inlined aggregate operators for finding the min/max values for primitives (int, long, float, // double, decimal). Versions are also offered for the nullable primitives (int?, long?, float?, // double?, decimal?), which differ slightly in behavior: they return a null value for empty // streams, whereas the ordinary primitive versions throw. // //--------------------------------------------------------------------------------------- // Inlined average operators for primitives (int, long, float, double, decimal), and the // nullable variants. The difference between the nromal and nullable variety is that // nulls are skipped in tallying the count and sum for the average. // ////// A class with some shared implementation between all aggregation enumerators. /// ///internal abstract class InlinedAggregationOperatorEnumerator : QueryOperatorEnumerator { private int m_partitionIndex; // This partition's unique index. protected CancellationToken m_cancellationToken; //--------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal InlinedAggregationOperatorEnumerator(int partitionIndex, CancellationToken cancellationToken) { m_partitionIndex = partitionIndex; m_cancellationToken = cancellationToken; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. There is a boilerplate variant used by callers, // and then one that is used for extensibility by subclasses. // internal sealed override bool MoveNext(ref TIntermediate currentElement, ref int currentKey) { if (MoveNextCore(ref currentElement)) { // A reduction's "index" is the same as its partition number. currentKey = m_partitionIndex; return true; } return false; } protected abstract bool MoveNextCore(ref TIntermediate currentElement); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
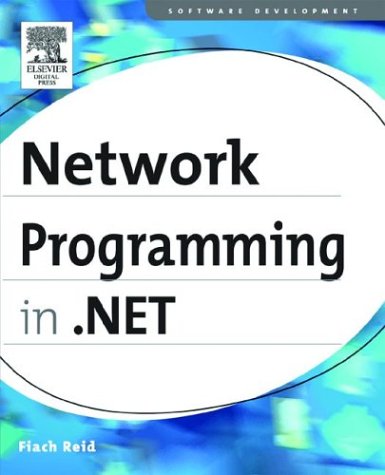
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Relationship.cs
- DataGridViewTextBoxColumn.cs
- GridViewRowEventArgs.cs
- DocumentReference.cs
- EventManager.cs
- ChangeDirector.cs
- HyperlinkAutomationPeer.cs
- DataBindingExpressionBuilder.cs
- TypeConstant.cs
- WinInetCache.cs
- CompareInfo.cs
- _NtlmClient.cs
- RuntimeHandles.cs
- TextEditorParagraphs.cs
- IndicCharClassifier.cs
- WebPartConnectionsCancelVerb.cs
- RemotingAttributes.cs
- BindingWorker.cs
- TextReader.cs
- ErrorTolerantObjectWriter.cs
- GeometryGroup.cs
- HttpConfigurationContext.cs
- Function.cs
- LineProperties.cs
- EntityWithChangeTrackerStrategy.cs
- entitydatasourceentitysetnameconverter.cs
- Model3D.cs
- DbConnectionPoolIdentity.cs
- StatusInfoItem.cs
- ApplicationException.cs
- BooleanKeyFrameCollection.cs
- HtmlHistory.cs
- SystemIPv6InterfaceProperties.cs
- FixedDocument.cs
- ThrowHelper.cs
- TransactionOptions.cs
- GAC.cs
- EpmSyndicationContentSerializer.cs
- BinaryKeyIdentifierClause.cs
- GridSplitter.cs
- smtpconnection.cs
- URL.cs
- IdentityHolder.cs
- PublisherIdentityPermission.cs
- FontCacheLogic.cs
- SQLBinaryStorage.cs
- XmlSerializerObjectSerializer.cs
- WindowsTab.cs
- WorkflowApplicationCompletedEventArgs.cs
- MsmqHostedTransportManager.cs
- ListViewItem.cs
- FilteredSchemaElementLookUpTable.cs
- CqlLexerHelpers.cs
- TextDocumentView.cs
- DocumentViewer.cs
- SerializationBinder.cs
- BufferAllocator.cs
- FormatSettings.cs
- ZoneLinkButton.cs
- FrameworkObject.cs
- XmlName.cs
- UriWriter.cs
- StreamGeometry.cs
- IPHostEntry.cs
- XmlSchemaExporter.cs
- SqlDataSourceQuery.cs
- SqlDataSourceView.cs
- BindingCollectionElement.cs
- RNGCryptoServiceProvider.cs
- SmiGettersStream.cs
- InkCanvasInnerCanvas.cs
- Deserializer.cs
- DiffuseMaterial.cs
- ServiceDescriptions.cs
- SchemaMerger.cs
- MobileUserControlDesigner.cs
- SourceInterpreter.cs
- ItemAutomationPeer.cs
- ServiceModelExtensionCollectionElement.cs
- DuplexChannel.cs
- EventProvider.cs
- CreateUserErrorEventArgs.cs
- OnOperation.cs
- CollectionType.cs
- _SslSessionsCache.cs
- TypeDependencyAttribute.cs
- CodeNamespaceImportCollection.cs
- RelationshipManager.cs
- Control.cs
- FixedSOMTableCell.cs
- NameTable.cs
- NameValueCollection.cs
- WebPartHelpVerb.cs
- XmlUtil.cs
- ThreadStateException.cs
- RelatedCurrencyManager.cs
- TypeSystemProvider.cs
- ApplicationCommands.cs
- PackagingUtilities.cs
- KeyedPriorityQueue.cs