Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DLinq / Dlinq / SqlClient / Common / TypeSystemProvider.cs / 1 / TypeSystemProvider.cs
using System; using System.Collections.Generic; using System.Text; namespace System.Data.Linq.SqlClient { ////// Abstracts the provider side type system. Encapsulates: /// - Mapping from runtime types to provider types. /// - Parsing type strings in the provider's language. /// - Handling application defined (opaque) types. /// - Type coercion precedence rules. /// - Type family organization. /// internal abstract class TypeSystemProvider { internal abstract ProviderType PredictTypeForUnary(SqlNodeType unaryOp, ProviderType operandType); internal abstract ProviderType PredictTypeForBinary(SqlNodeType binaryOp, ProviderType leftType, ProviderType rightType); ////// Return the provider type corresponding to the given clr type. /// internal abstract ProviderType From(Type runtimeType); ////// Return the provider type corresponding to the given object instance. /// internal abstract ProviderType From(object o); ////// Return the provider type corresponding to the given clr type and size. /// internal abstract ProviderType From(Type type, int? size); ////// Return a type by parsing a string. The format of the string is /// provider specific. /// internal abstract ProviderType Parse(string text); ////// Return a type understood only by the application. /// Each call with the same index will return the same ProviderType. /// internal abstract ProviderType GetApplicationType(int index); ////// Returns the most precise type in the family of the type given. /// A family is a group types that serve similar functions. For example, /// in SQL SmallInt and Int are part of one family. /// internal abstract ProviderType MostPreciseTypeInFamily(ProviderType type); ////// For LOB data types that have large type equivalents, this function returns the equivalent large /// data type. If the type is not an LOB or cannot be converted, the function returns the current type. /// For example SqlServer defines the 'Image' LOB type, whose large type equivalent is VarBinary(MAX). /// internal abstract ProviderType GetBestLargeType(ProviderType type); ////// Returns a type that can be used to hold values for both the current /// type and the specified type without data loss. /// internal abstract ProviderType GetBestType(ProviderType typeA, ProviderType typeB); internal abstract ProviderType ReturnTypeOfFunction(SqlFunctionCall functionCall); ////// Get a type that can hold the same information but belongs to a different type family. /// For example, to represent a SQL NChar as an integer type, we need to use the type int. /// (SQL smallint would not be able to contain characters with unicode >32768) /// /// Type of the target type family ///Smallest type of target type family that can hold equivalent information internal abstract ProviderType ChangeTypeFamilyTo(ProviderType type, ProviderType typeWithFamily); internal abstract void InitializeParameter(ProviderType type, System.Data.Common.DbParameter parameter, object value); } ////// Flags control the format of string returned by ToQueryString(). /// [Flags] internal enum QueryFormatOptions { None = 0, SuppressSize = 1 } ////// An abstract type exposed by the TypeSystemProvider. /// internal abstract class ProviderType { ////// True if this type is a Unicode type (eg, ntext, etc). /// internal abstract bool IsUnicodeType { get; } ////// For a unicode type, return it's non-unicode equivalent. /// internal abstract ProviderType GetNonUnicodeEquivalent(); ////// True if this type has only a CLR representation and no provider representation. /// internal abstract bool IsRuntimeOnlyType { get; } ////// True if this type is an application defined type. /// internal abstract bool IsApplicationType { get; } ////// Determine whether this is the given application type. /// internal abstract bool IsApplicationTypeOf(int index); ////// Returns the CLR type which most closely corresponds to this provider type. /// internal abstract Type GetClosestRuntimeType(); ////// Compare implicit type coercion precedence. /// -1 means there is an implicit conversion from this->type. /// 0 means there is a two way implicit conversion from this->type /// 1 means there is an implicit conversion from type->this. /// internal abstract int ComparePrecedenceTo(ProviderType type); ////// Determines whether two types are in the same type family. /// A family is a group types that serve similar functions. For example, /// in SQL SmallInt and Int are part of one family. /// internal abstract bool IsSameTypeFamily(ProviderType type); ////// Used to indicate if the type supports comparison in provider. /// ///Returns true if type supports comparison in provider. internal abstract bool SupportsComparison { get; } ////// Used to indicate if the types supports Length function (LEN in T-SQL). /// ///Returns true if type supports use of length function on the type. internal abstract bool SupportsLength { get; } ////// Returns true if the given values will be equal to eachother for this type. /// internal abstract bool AreValuesEqual(object o1, object o2); ////// Determines whether this type is a LOB (large object) type, or an equivalent type. /// For example, on SqlServer, Image and VarChar(MAX) among others are considered large types. /// ///internal abstract bool IsLargeType { get; } /// /// Convert this type into a string that can be used in a query. /// internal abstract string ToQueryString(); ////// Convert this type into a string that can be used in a query. /// internal abstract string ToQueryString(QueryFormatOptions formatOptions); ////// Whether this type is fixed size or not. /// internal abstract bool IsFixedSize { get; } ////// The type has a size or is large. /// internal abstract bool HasSizeOrIsLarge { get; } ////// The size of this type. /// internal abstract int? Size { get; } ////// True if the type can be ordered. /// internal abstract bool IsOrderable { get; } ////// True if the type can be grouped. /// internal abstract bool IsGroupable { get; } ////// True if the type can appear in a column /// internal abstract bool CanBeColumn { get; } ////// True if the type can appear as a parameter /// internal abstract bool CanBeParameter { get; } ////// True if the type is a single character type. /// internal abstract bool IsChar { get; } ////// True if the type is a multi-character type. /// internal abstract bool IsString { get; } ////// True if the type is a number. /// internal abstract bool IsNumeric { get; } ////// Returns true if the type uses precision and scale. For example, returns true /// for SqlDBTypes Decimal, Float and Real. /// internal abstract bool HasPrecisionAndScale { get; } ////// Determines if it is safe to suppress size specifications for /// the operand of a cast/convert. For example, when casting to string, /// all these types have length less than the default sized used by SqlServer, /// so the length specification can be omitted without fear of truncation. /// internal abstract bool CanSuppressSizeForConversionToString{ get; } public static bool operator ==(ProviderType typeA, ProviderType typeB) { if ((object)typeA == (object)typeB) return true; if ((object)typeA != null) return typeA.Equals(typeB); return false; } public static bool operator != (ProviderType typeA, ProviderType typeB) { if ((object)typeA == (object)typeB) return false; if ((object)typeA != null) return !typeA.Equals(typeB); return true; } public override bool Equals(object obj) { return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Text; namespace System.Data.Linq.SqlClient { ////// Abstracts the provider side type system. Encapsulates: /// - Mapping from runtime types to provider types. /// - Parsing type strings in the provider's language. /// - Handling application defined (opaque) types. /// - Type coercion precedence rules. /// - Type family organization. /// internal abstract class TypeSystemProvider { internal abstract ProviderType PredictTypeForUnary(SqlNodeType unaryOp, ProviderType operandType); internal abstract ProviderType PredictTypeForBinary(SqlNodeType binaryOp, ProviderType leftType, ProviderType rightType); ////// Return the provider type corresponding to the given clr type. /// internal abstract ProviderType From(Type runtimeType); ////// Return the provider type corresponding to the given object instance. /// internal abstract ProviderType From(object o); ////// Return the provider type corresponding to the given clr type and size. /// internal abstract ProviderType From(Type type, int? size); ////// Return a type by parsing a string. The format of the string is /// provider specific. /// internal abstract ProviderType Parse(string text); ////// Return a type understood only by the application. /// Each call with the same index will return the same ProviderType. /// internal abstract ProviderType GetApplicationType(int index); ////// Returns the most precise type in the family of the type given. /// A family is a group types that serve similar functions. For example, /// in SQL SmallInt and Int are part of one family. /// internal abstract ProviderType MostPreciseTypeInFamily(ProviderType type); ////// For LOB data types that have large type equivalents, this function returns the equivalent large /// data type. If the type is not an LOB or cannot be converted, the function returns the current type. /// For example SqlServer defines the 'Image' LOB type, whose large type equivalent is VarBinary(MAX). /// internal abstract ProviderType GetBestLargeType(ProviderType type); ////// Returns a type that can be used to hold values for both the current /// type and the specified type without data loss. /// internal abstract ProviderType GetBestType(ProviderType typeA, ProviderType typeB); internal abstract ProviderType ReturnTypeOfFunction(SqlFunctionCall functionCall); ////// Get a type that can hold the same information but belongs to a different type family. /// For example, to represent a SQL NChar as an integer type, we need to use the type int. /// (SQL smallint would not be able to contain characters with unicode >32768) /// /// Type of the target type family ///Smallest type of target type family that can hold equivalent information internal abstract ProviderType ChangeTypeFamilyTo(ProviderType type, ProviderType typeWithFamily); internal abstract void InitializeParameter(ProviderType type, System.Data.Common.DbParameter parameter, object value); } ////// Flags control the format of string returned by ToQueryString(). /// [Flags] internal enum QueryFormatOptions { None = 0, SuppressSize = 1 } ////// An abstract type exposed by the TypeSystemProvider. /// internal abstract class ProviderType { ////// True if this type is a Unicode type (eg, ntext, etc). /// internal abstract bool IsUnicodeType { get; } ////// For a unicode type, return it's non-unicode equivalent. /// internal abstract ProviderType GetNonUnicodeEquivalent(); ////// True if this type has only a CLR representation and no provider representation. /// internal abstract bool IsRuntimeOnlyType { get; } ////// True if this type is an application defined type. /// internal abstract bool IsApplicationType { get; } ////// Determine whether this is the given application type. /// internal abstract bool IsApplicationTypeOf(int index); ////// Returns the CLR type which most closely corresponds to this provider type. /// internal abstract Type GetClosestRuntimeType(); ////// Compare implicit type coercion precedence. /// -1 means there is an implicit conversion from this->type. /// 0 means there is a two way implicit conversion from this->type /// 1 means there is an implicit conversion from type->this. /// internal abstract int ComparePrecedenceTo(ProviderType type); ////// Determines whether two types are in the same type family. /// A family is a group types that serve similar functions. For example, /// in SQL SmallInt and Int are part of one family. /// internal abstract bool IsSameTypeFamily(ProviderType type); ////// Used to indicate if the type supports comparison in provider. /// ///Returns true if type supports comparison in provider. internal abstract bool SupportsComparison { get; } ////// Used to indicate if the types supports Length function (LEN in T-SQL). /// ///Returns true if type supports use of length function on the type. internal abstract bool SupportsLength { get; } ////// Returns true if the given values will be equal to eachother for this type. /// internal abstract bool AreValuesEqual(object o1, object o2); ////// Determines whether this type is a LOB (large object) type, or an equivalent type. /// For example, on SqlServer, Image and VarChar(MAX) among others are considered large types. /// ///internal abstract bool IsLargeType { get; } /// /// Convert this type into a string that can be used in a query. /// internal abstract string ToQueryString(); ////// Convert this type into a string that can be used in a query. /// internal abstract string ToQueryString(QueryFormatOptions formatOptions); ////// Whether this type is fixed size or not. /// internal abstract bool IsFixedSize { get; } ////// The type has a size or is large. /// internal abstract bool HasSizeOrIsLarge { get; } ////// The size of this type. /// internal abstract int? Size { get; } ////// True if the type can be ordered. /// internal abstract bool IsOrderable { get; } ////// True if the type can be grouped. /// internal abstract bool IsGroupable { get; } ////// True if the type can appear in a column /// internal abstract bool CanBeColumn { get; } ////// True if the type can appear as a parameter /// internal abstract bool CanBeParameter { get; } ////// True if the type is a single character type. /// internal abstract bool IsChar { get; } ////// True if the type is a multi-character type. /// internal abstract bool IsString { get; } ////// True if the type is a number. /// internal abstract bool IsNumeric { get; } ////// Returns true if the type uses precision and scale. For example, returns true /// for SqlDBTypes Decimal, Float and Real. /// internal abstract bool HasPrecisionAndScale { get; } ////// Determines if it is safe to suppress size specifications for /// the operand of a cast/convert. For example, when casting to string, /// all these types have length less than the default sized used by SqlServer, /// so the length specification can be omitted without fear of truncation. /// internal abstract bool CanSuppressSizeForConversionToString{ get; } public static bool operator ==(ProviderType typeA, ProviderType typeB) { if ((object)typeA == (object)typeB) return true; if ((object)typeA != null) return typeA.Equals(typeB); return false; } public static bool operator != (ProviderType typeA, ProviderType typeB) { if ((object)typeA == (object)typeB) return false; if ((object)typeA != null) return !typeA.Equals(typeB); return true; } public override bool Equals(object obj) { return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
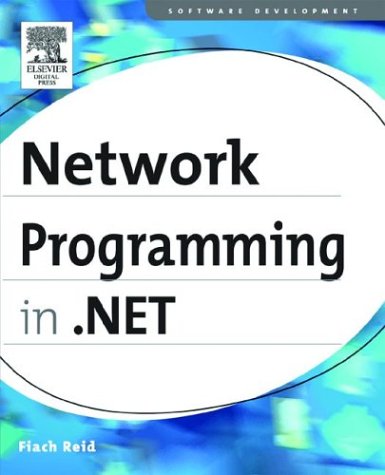
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedNode.cs
- HtmlElement.cs
- IIS7WorkerRequest.cs
- FamilyCollection.cs
- PerspectiveCamera.cs
- SystemInformation.cs
- Imaging.cs
- QilReplaceVisitor.cs
- LinkConverter.cs
- NativeStructs.cs
- PagerSettings.cs
- ApplicationCommands.cs
- DataGridViewCheckBoxColumn.cs
- EntityTypeEmitter.cs
- DynamicUpdateCommand.cs
- StandardCommandToolStripMenuItem.cs
- QueryExecutionOption.cs
- ZipIOCentralDirectoryFileHeader.cs
- ToggleButtonAutomationPeer.cs
- MethodBuilderInstantiation.cs
- Tag.cs
- IdentityManager.cs
- SelectionItemProviderWrapper.cs
- ApplicationBuildProvider.cs
- Rect.cs
- SecurityPolicySection.cs
- TrustLevelCollection.cs
- ReferencedType.cs
- StringValidator.cs
- SwitchAttribute.cs
- HttpHostedTransportConfiguration.cs
- SqlMethodTransformer.cs
- InputScopeConverter.cs
- CompositeDuplexElement.cs
- Pair.cs
- VariableAction.cs
- Vector3DAnimation.cs
- CompilerErrorCollection.cs
- TextEditorTables.cs
- TypeConstant.cs
- Math.cs
- FixedSOMFixedBlock.cs
- OleDbFactory.cs
- MenuItemCollectionEditor.cs
- XmlHierarchyData.cs
- ObjectView.cs
- ReadOnlyDictionary.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- MemberCollection.cs
- Matrix.cs
- DataRecordInternal.cs
- ProfilePropertySettingsCollection.cs
- PointLightBase.cs
- HttpCachePolicy.cs
- XamlStream.cs
- AggregateNode.cs
- InstanceDescriptor.cs
- UmAlQuraCalendar.cs
- BamlBinaryReader.cs
- DataRowCollection.cs
- DataObjectMethodAttribute.cs
- SequentialOutput.cs
- RawUIStateInputReport.cs
- X509ClientCertificateAuthenticationElement.cs
- EventLogInternal.cs
- XamlInterfaces.cs
- UTF7Encoding.cs
- UrlPath.cs
- PropertyChangedEventArgs.cs
- TextTabProperties.cs
- IteratorFilter.cs
- HttpCapabilitiesEvaluator.cs
- CompoundFileStorageReference.cs
- ParenExpr.cs
- MemberMaps.cs
- SplitterPanel.cs
- BitStack.cs
- MobileControlPersister.cs
- Rfc2898DeriveBytes.cs
- Grant.cs
- Transaction.cs
- AddInPipelineAttributes.cs
- TableDetailsRow.cs
- LogReserveAndAppendState.cs
- SoapProtocolImporter.cs
- SendDesigner.xaml.cs
- ControlCollection.cs
- FaultCallbackWrapper.cs
- TreeNodeEventArgs.cs
- Misc.cs
- CharacterHit.cs
- VoiceInfo.cs
- CodeSnippetStatement.cs
- CommonDialog.cs
- shaperfactoryquerycachekey.cs
- TableAutomationPeer.cs
- ObjectListTitleAttribute.cs
- ModuleBuilderData.cs
- ConfigurationException.cs
- ClientSettingsProvider.cs