Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / StandardCommandToolStripMenuItem.cs / 1 / StandardCommandToolStripMenuItem.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Design; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using System.Windows.Forms.Design.Behavior; using System.Runtime.InteropServices; using System.Drawing.Drawing2D; ////// /// Associates standard command with ToolStripMenuItem. /// ///internal class StandardCommandToolStripMenuItem : ToolStripMenuItem { private bool _cachedImage = false; private Image _image = null; private CommandID menuID; private IMenuCommandService menuCommandService; private IServiceProvider serviceProvider; private string name; private MenuCommand menuCommand; // Ok to call MenuService.FindComand to find the menuCommand mapping to the appropriated menuID. [SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes")] [SuppressMessage("Microsoft.Security", "CA2102:CatchNonClsCompliantExceptionsInGeneralHandlers")] public StandardCommandToolStripMenuItem(CommandID menuID, string text, string imageName, IServiceProvider serviceProvider) { this.menuID = menuID; this.serviceProvider = serviceProvider; // Findcommand can throw; so we need to catch and disable the command. try { menuCommand = MenuService.FindCommand(menuID); } catch { this.Enabled = false; } this.Text = text; this.name = imageName; RefreshItem(); } public void RefreshItem() { if (menuCommand != null) { this.Visible = menuCommand.Visible; this.Enabled = menuCommand.Enabled; this.Checked = menuCommand.Checked; } } /// /// /// Retrieves the menu editor service, which we cache for speed. /// public IMenuCommandService MenuService { get { if (menuCommandService == null) { menuCommandService = (IMenuCommandService)serviceProvider.GetService(typeof(IMenuCommandService)); } return menuCommandService; } } public override Image Image { // Standard 'catch all - rethrow critical' exception pattern [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes")] get { // Defer loading the image until we're sure we need it if (!_cachedImage) { _cachedImage = true; try { if (name != null) { _image = new Bitmap(typeof(ToolStripMenuItem), name + ".bmp"); } this.ImageTransparentColor = Color.Magenta; } catch (Exception ex) { if (ClientUtils.IsCriticalException(ex)) { throw; } } catch { } } return _image; } set { _image = value; _cachedImage = true; } } protected override void OnClick(System.EventArgs e) { if (menuCommand != null) { menuCommand.Invoke(); } else if (MenuService != null) { if (MenuService.GlobalInvoke(menuID)) { return; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
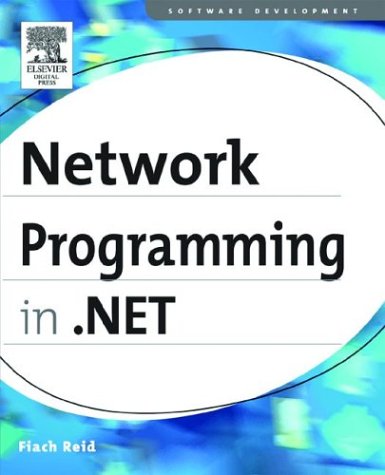
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SpellerError.cs
- MemberCollection.cs
- RefreshEventArgs.cs
- ExternalFile.cs
- Visual.cs
- TransactionProxy.cs
- PointAnimationBase.cs
- ToolBarOverflowPanel.cs
- LicFileLicenseProvider.cs
- UIElementParaClient.cs
- CollectionViewSource.cs
- EncoderBestFitFallback.cs
- MouseActionConverter.cs
- Baml6Assembly.cs
- MetadataCollection.cs
- AsymmetricSecurityProtocol.cs
- ChangesetResponse.cs
- counter.cs
- UInt16.cs
- MessagePropertyVariants.cs
- MenuItemBindingCollection.cs
- TransformerConfigurationWizardBase.cs
- TypeEnumerableViewSchema.cs
- StylusTip.cs
- DateTime.cs
- Button.cs
- WebPartDisplayMode.cs
- FontNamesConverter.cs
- ObjectCloneHelper.cs
- FormsAuthenticationCredentials.cs
- SapiGrammar.cs
- ScrollContentPresenter.cs
- CacheAxisQuery.cs
- ValidatedControlConverter.cs
- PageMediaSize.cs
- TextParaLineResult.cs
- SystemResourceHost.cs
- SubtreeProcessor.cs
- HotSpot.cs
- UdpChannelListener.cs
- DataServiceHost.cs
- GroupByQueryOperator.cs
- WasEndpointConfigContainer.cs
- TrackingProfileSerializer.cs
- TextServicesManager.cs
- EventBuilder.cs
- ValidationHelper.cs
- ApplicationFileParser.cs
- MultitargetUtil.cs
- EntityConnectionStringBuilder.cs
- TextRunTypographyProperties.cs
- OleDbCommandBuilder.cs
- FileDialogCustomPlace.cs
- _ScatterGatherBuffers.cs
- LocalizabilityAttribute.cs
- HideDisabledControlAdapter.cs
- KeyConstraint.cs
- InkCanvasInnerCanvas.cs
- DateTimePicker.cs
- EUCJPEncoding.cs
- BitmapImage.cs
- SiteMapPath.cs
- ReachSerializer.cs
- ReferencedType.cs
- FrameworkElementAutomationPeer.cs
- Hex.cs
- DbXmlEnabledProviderManifest.cs
- ListenerAdapterBase.cs
- AssociationSet.cs
- _AutoWebProxyScriptHelper.cs
- PropertyFilterAttribute.cs
- DataServiceSaveChangesEventArgs.cs
- UpdateCompiler.cs
- DataBindingList.cs
- CachedBitmap.cs
- MediaSystem.cs
- ThreadPool.cs
- BaseUriHelper.cs
- remotingproxy.cs
- XmlSchemaFacet.cs
- NonSerializedAttribute.cs
- DataContractSet.cs
- Content.cs
- InheritanceUI.cs
- CodeRegionDirective.cs
- TableParaClient.cs
- EventLogPermission.cs
- PeerObject.cs
- AlphaSortedEnumConverter.cs
- coordinatorscratchpad.cs
- DesignerTransaction.cs
- FontResourceCache.cs
- SafeProcessHandle.cs
- Pen.cs
- PropertyChange.cs
- WebServiceClientProxyGenerator.cs
- EncoderExceptionFallback.cs
- DBPropSet.cs
- ForwardPositionQuery.cs
- NativeMethods.cs