Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / ExpressionBindingCollection.cs / 2 / ExpressionBindingCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Web.Util; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class ExpressionBindingCollection : ICollection { private EventHandler changedEvent; private Hashtable bindings; private Hashtable removedBindings; ////// public ExpressionBindingCollection() { this.bindings = new Hashtable(StringComparer.OrdinalIgnoreCase); } ////// public int Count { get { return bindings.Count; } } ////// public bool IsReadOnly { get { return false; } } ////// public bool IsSynchronized { get { return false; } } ////// public ICollection RemovedBindings { get { int bindingCount = 0; ICollection keys = null; if (removedBindings != null) { keys = removedBindings.Keys; bindingCount = keys.Count; string[] removedNames = new string[bindingCount]; int i = 0; foreach (string s in keys) { removedNames[i++] = s; } removedBindings.Clear(); return removedNames; } else { return new string[0]; } } } ////// private Hashtable RemovedBindingsTable { get { if (removedBindings == null) { removedBindings = new Hashtable(StringComparer.OrdinalIgnoreCase); } return removedBindings; } } ////// public object SyncRoot { get { return this; } } ////// public ExpressionBinding this[string propertyName] { get { object o = bindings[propertyName]; if (o != null) return(ExpressionBinding)o; return null; } } public event EventHandler Changed { add { changedEvent = (EventHandler)Delegate.Combine(changedEvent, value); } remove { changedEvent = (EventHandler)Delegate.Remove(changedEvent, value); } } ////// public void Add(ExpressionBinding binding) { bindings[binding.PropertyName] = binding; RemovedBindingsTable.Remove(binding.PropertyName); OnChanged(); } ////// public bool Contains(string propName) { return bindings.Contains(propName); } ////// public void Clear() { ICollection keys = bindings.Keys; if ((keys.Count != 0) && (removedBindings == null)) { // ensure the removedBindings hashtable is created Hashtable h = RemovedBindingsTable; } foreach (string s in keys) { removedBindings[s] = String.Empty; } bindings.Clear(); OnChanged(); } ////// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ////// public void CopyTo(ExpressionBinding[] array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ////// public IEnumerator GetEnumerator() { return bindings.Values.GetEnumerator(); } private void OnChanged() { if (changedEvent != null) { changedEvent(this, EventArgs.Empty); } } ////// public void Remove(string propertyName) { Remove(propertyName, true); } ////// public void Remove(ExpressionBinding binding) { Remove(binding.PropertyName, true); } ////// public void Remove(string propertyName, bool addToRemovedList) { if (Contains(propertyName)) { if (addToRemovedList && bindings.Contains(propertyName)) { RemovedBindingsTable[propertyName] = String.Empty; } bindings.Remove(propertyName); OnChanged(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
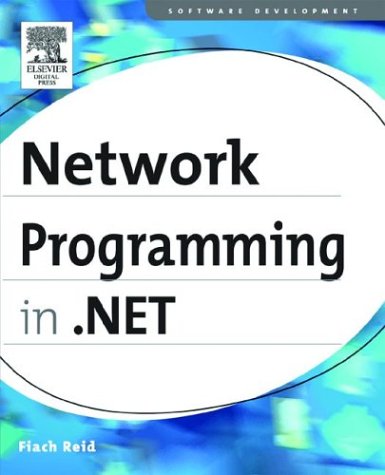
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RightsManagementInformation.cs
- HotSpotCollection.cs
- SerialStream.cs
- ImageList.cs
- BitmapFrame.cs
- SHA1Cng.cs
- Context.cs
- ListSourceHelper.cs
- MetadataArtifactLoaderCompositeResource.cs
- DefaultDiscoveryServiceExtension.cs
- EnumMemberAttribute.cs
- XmlILConstructAnalyzer.cs
- SQLDecimalStorage.cs
- MimeTypeAttribute.cs
- HtmlInputImage.cs
- BaseDataBoundControl.cs
- SafeHandles.cs
- AssemblyInfo.cs
- MultiAsyncResult.cs
- InheritanceContextChangedEventManager.cs
- DesignBindingConverter.cs
- Environment.cs
- controlskin.cs
- ComboBox.cs
- StateElement.cs
- NativeMethods.cs
- _ShellExpression.cs
- ObjectPersistData.cs
- ThreadExceptionDialog.cs
- XPathPatternParser.cs
- StatusBar.cs
- PrintControllerWithStatusDialog.cs
- Models.cs
- PartitionResolver.cs
- RIPEMD160Managed.cs
- WizardPanel.cs
- ScrollChangedEventArgs.cs
- TreeView.cs
- ServiceOperationDetailViewControl.cs
- HtmlMeta.cs
- RotateTransform.cs
- LocatorGroup.cs
- KeyboardInputProviderAcquireFocusEventArgs.cs
- MarkupCompiler.cs
- Dictionary.cs
- DataGridState.cs
- MexTcpBindingElement.cs
- XmlSchemaObjectTable.cs
- ArrayTypeMismatchException.cs
- Command.cs
- DelegatingConfigHost.cs
- BamlVersionHeader.cs
- CompositeClientFormatter.cs
- CookieHandler.cs
- ZipIOExtraFieldZip64Element.cs
- BuilderInfo.cs
- BadImageFormatException.cs
- WebCategoryAttribute.cs
- EdmProviderManifest.cs
- DataGridViewCellConverter.cs
- COM2PropertyPageUITypeConverter.cs
- SoapSchemaImporter.cs
- cryptoapiTransform.cs
- SystemGatewayIPAddressInformation.cs
- TableCellAutomationPeer.cs
- RulePatternOps.cs
- LinkedResourceCollection.cs
- SqlDataSourceCustomCommandEditor.cs
- ConfigXmlElement.cs
- UniqueIdentifierService.cs
- ComponentRenameEvent.cs
- AlternateView.cs
- AuditLevel.cs
- InternalTypeHelper.cs
- AuthenticationConfig.cs
- ServicesExceptionNotHandledEventArgs.cs
- FieldNameLookup.cs
- EngineSiteSapi.cs
- ParameterCollection.cs
- VisualBrush.cs
- DeploymentExceptionMapper.cs
- DecimalConstantAttribute.cs
- CqlIdentifiers.cs
- ServiceNameElement.cs
- ImageBrush.cs
- GestureRecognizer.cs
- LinkUtilities.cs
- RSACryptoServiceProvider.cs
- ZipIOCentralDirectoryBlock.cs
- ConfigDefinitionUpdates.cs
- DBDataPermission.cs
- _AutoWebProxyScriptWrapper.cs
- WebBrowserHelper.cs
- Int32Storage.cs
- ValueSerializer.cs
- SplitterEvent.cs
- JavaScriptString.cs
- NotFiniteNumberException.cs
- AttributeParameterInfo.cs
- Int16AnimationUsingKeyFrames.cs