Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / GrammarBuilding / BuilderElements.cs / 1 / BuilderElements.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System.Collections.ObjectModel; using System.Collections.Generic; using System.Diagnostics; using System.Speech.Recognition; using System.Speech.Internal.SrgsParser; using System.Text; namespace System.Speech.Internal.GrammarBuilding { ////// /// #if VSCOMPILE [DebuggerDisplay ("{DebugSummary}")] #endif internal abstract class BuilderElements : GrammarBuilderBase { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// /// internal BuilderElements () { } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods /// TODOC public override bool Equals (object obj) { BuilderElements refObj = obj as BuilderElements; if (refObj == null) { return false; } // Easy out if the number of elements do not match if (refObj.Count != Count || refObj.Items.Count != Items.Count) { return false; } // Deep recursive search for equality for (int i = 0; i < Items.Count; i++) { if (!Items [i].Equals (refObj.Items [i])) { return false; } } return true; } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.GetHashCode"]/*' /> public override int GetHashCode () { return base.GetHashCode (); } #endregion //******************************************************************** // // Protected Methods // //******************************************************************** #region Internal Methods ////// Optimization for a element tree /// /// protected void Optimize (CollectionnewRules) { // Create an dictionary of [Count of elements, list of elements] SortedDictionary > dict = new SortedDictionary > (); GetDictionaryElements (dict); // The dictionary is sorted from the smallest buckets to the largest. // Revert the order in the keys arrays int [] keys = new int [dict.Keys.Count]; int index = keys.Length - 1; foreach (int key in dict.Keys) { keys [index--] = key; } // Look for each bucket from the largest to the smallest for (int i = 0; i < keys.Length && keys [i] >= 3; i++) { Collection gb = dict [keys [i]]; for (int j = 0; j < gb.Count; j++) { RuleElement newRule = null; RuleRefElement ruleRef = null; for (int k = j + 1; k < gb.Count; k++) { if (gb [j] != null && gb [j].Equals (gb [k])) { BuilderElements current = gb [k]; BuilderElements parent = current.Parent; if (current is SemanticKeyElement) // if current is already a ruleref. There is no need to create a new one { // Simply set the ruleref of the current element to the ruleref of the org element. parent.Items [parent.Items.IndexOf (current)] = gb [j]; } else { // Create a rule to store the common elemts if (newRule == null) { newRule = new RuleElement (current, "_"); newRules.Add (newRule); } // Create a ruleref and attach the if (ruleRef == null) { ruleRef = new RuleRefElement (newRule); gb [j].Parent.Items [gb [j].Parent.Items.IndexOf (gb [j])] = ruleRef; } parent.Items [current.Parent.Items.IndexOf (current)] = ruleRef; } // current.RemoveDictionaryElements (dict); gb [k] = null; } } } } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods /// /// /// /// internal void Add (string phrase) { _items.Add (new GrammarBuilderPhrase (phrase)); } ////// /// /// internal void Add (GrammarBuilder builder) { foreach (GrammarBuilderBase item in builder.InternalBuilder.Items) { _items.Add (item); } } ////// /// /// internal void Add (GrammarBuilderBase item) { _items.Add (item); } ////// /// ////// internal void CloneItems (BuilderElements builders) { foreach (GrammarBuilderBase item in builders.Items) { _items.Add (item); } } /// /// /// /// /// /// ///internal void CreateChildrenElements (IElementFactory elementFactory, IRule parent, IdentifierCollection ruleIds) { foreach (GrammarBuilderBase buider in Items) { IElement element = buider.CreateElement (elementFactory, parent, parent, ruleIds); if (element != null) { element.PostParse (parent); elementFactory.AddElement (parent, element); } } } /// /// /// /// /// /// /// ///internal void CreateChildrenElements (IElementFactory elementFactory, IItem parent, IRule rule, IdentifierCollection ruleIds) { foreach (GrammarBuilderBase buider in Items) { IElement element = buider.CreateElement (elementFactory, parent, rule, ruleIds); if (element != null) { element.PostParse (parent); elementFactory.AddElement (parent, element); } } } /// /// /// ///internal override int CalcCount (BuilderElements parent) { base.CalcCount (parent); int c = 1; foreach (GrammarBuilderBase item in Items) { c += item.CalcCount (this); } Count = c; return c; } #endregion //******************************************************************* // // Internal Properties // //******************************************************************* #region Internal Properties internal List Items { get { return _items; } } override internal string DebugSummary { get { StringBuilder sb = new StringBuilder (); foreach (GrammarBuilderBase item in _items) { if (sb.Length > 0) { sb.Append (" "); } sb.Append (item.DebugSummary); } return sb.ToString (); } } #endregion //******************************************************************* // // Private Method // //******************************************************************** #region Private Method private void GetDictionaryElements (SortedDictionary > dict) { // Recursive search from a matching subtree foreach (GrammarBuilderBase item in Items) { BuilderElements current = item as BuilderElements; // Go deeper if the number of children is greater the element to compare against. if (current != null) { if (!dict.ContainsKey (current.Count)) { dict.Add (current.Count, new Collection ()); } dict [current.Count].Add (current); current.GetDictionaryElements (dict); } } } private void RemoveDictionaryElements (SortedDictionary > dict) { // Recursive search from a matching subtree foreach (GrammarBuilderBase item in Items) { BuilderElements current = item as BuilderElements; // Go deeper if the number of children is greater the element to compare against. if (current != null) { // Recursively remove all elements current.RemoveDictionaryElements (dict); dict [current.Count].Remove (current); } } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields // List of builder elements private readonly List _items = new List (); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System.Collections.ObjectModel; using System.Collections.Generic; using System.Diagnostics; using System.Speech.Recognition; using System.Speech.Internal.SrgsParser; using System.Text; namespace System.Speech.Internal.GrammarBuilding { ////// /// #if VSCOMPILE [DebuggerDisplay ("{DebugSummary}")] #endif internal abstract class BuilderElements : GrammarBuilderBase { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// /// internal BuilderElements () { } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods /// TODOC public override bool Equals (object obj) { BuilderElements refObj = obj as BuilderElements; if (refObj == null) { return false; } // Easy out if the number of elements do not match if (refObj.Count != Count || refObj.Items.Count != Items.Count) { return false; } // Deep recursive search for equality for (int i = 0; i < Items.Count; i++) { if (!Items [i].Equals (refObj.Items [i])) { return false; } } return true; } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.GetHashCode"]/*' /> public override int GetHashCode () { return base.GetHashCode (); } #endregion //******************************************************************** // // Protected Methods // //******************************************************************** #region Internal Methods ////// Optimization for a element tree /// /// protected void Optimize (CollectionnewRules) { // Create an dictionary of [Count of elements, list of elements] SortedDictionary > dict = new SortedDictionary > (); GetDictionaryElements (dict); // The dictionary is sorted from the smallest buckets to the largest. // Revert the order in the keys arrays int [] keys = new int [dict.Keys.Count]; int index = keys.Length - 1; foreach (int key in dict.Keys) { keys [index--] = key; } // Look for each bucket from the largest to the smallest for (int i = 0; i < keys.Length && keys [i] >= 3; i++) { Collection gb = dict [keys [i]]; for (int j = 0; j < gb.Count; j++) { RuleElement newRule = null; RuleRefElement ruleRef = null; for (int k = j + 1; k < gb.Count; k++) { if (gb [j] != null && gb [j].Equals (gb [k])) { BuilderElements current = gb [k]; BuilderElements parent = current.Parent; if (current is SemanticKeyElement) // if current is already a ruleref. There is no need to create a new one { // Simply set the ruleref of the current element to the ruleref of the org element. parent.Items [parent.Items.IndexOf (current)] = gb [j]; } else { // Create a rule to store the common elemts if (newRule == null) { newRule = new RuleElement (current, "_"); newRules.Add (newRule); } // Create a ruleref and attach the if (ruleRef == null) { ruleRef = new RuleRefElement (newRule); gb [j].Parent.Items [gb [j].Parent.Items.IndexOf (gb [j])] = ruleRef; } parent.Items [current.Parent.Items.IndexOf (current)] = ruleRef; } // current.RemoveDictionaryElements (dict); gb [k] = null; } } } } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods /// /// /// /// internal void Add (string phrase) { _items.Add (new GrammarBuilderPhrase (phrase)); } ////// /// /// internal void Add (GrammarBuilder builder) { foreach (GrammarBuilderBase item in builder.InternalBuilder.Items) { _items.Add (item); } } ////// /// /// internal void Add (GrammarBuilderBase item) { _items.Add (item); } ////// /// ////// internal void CloneItems (BuilderElements builders) { foreach (GrammarBuilderBase item in builders.Items) { _items.Add (item); } } /// /// /// /// /// /// ///internal void CreateChildrenElements (IElementFactory elementFactory, IRule parent, IdentifierCollection ruleIds) { foreach (GrammarBuilderBase buider in Items) { IElement element = buider.CreateElement (elementFactory, parent, parent, ruleIds); if (element != null) { element.PostParse (parent); elementFactory.AddElement (parent, element); } } } /// /// /// /// /// /// /// ///internal void CreateChildrenElements (IElementFactory elementFactory, IItem parent, IRule rule, IdentifierCollection ruleIds) { foreach (GrammarBuilderBase buider in Items) { IElement element = buider.CreateElement (elementFactory, parent, rule, ruleIds); if (element != null) { element.PostParse (parent); elementFactory.AddElement (parent, element); } } } /// /// /// ///internal override int CalcCount (BuilderElements parent) { base.CalcCount (parent); int c = 1; foreach (GrammarBuilderBase item in Items) { c += item.CalcCount (this); } Count = c; return c; } #endregion //******************************************************************* // // Internal Properties // //******************************************************************* #region Internal Properties internal List Items { get { return _items; } } override internal string DebugSummary { get { StringBuilder sb = new StringBuilder (); foreach (GrammarBuilderBase item in _items) { if (sb.Length > 0) { sb.Append (" "); } sb.Append (item.DebugSummary); } return sb.ToString (); } } #endregion //******************************************************************* // // Private Method // //******************************************************************** #region Private Method private void GetDictionaryElements (SortedDictionary > dict) { // Recursive search from a matching subtree foreach (GrammarBuilderBase item in Items) { BuilderElements current = item as BuilderElements; // Go deeper if the number of children is greater the element to compare against. if (current != null) { if (!dict.ContainsKey (current.Count)) { dict.Add (current.Count, new Collection ()); } dict [current.Count].Add (current); current.GetDictionaryElements (dict); } } } private void RemoveDictionaryElements (SortedDictionary > dict) { // Recursive search from a matching subtree foreach (GrammarBuilderBase item in Items) { BuilderElements current = item as BuilderElements; // Go deeper if the number of children is greater the element to compare against. if (current != null) { // Recursively remove all elements current.RemoveDictionaryElements (dict); dict [current.Count].Remove (current); } } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields // List of builder elements private readonly List _items = new List (); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
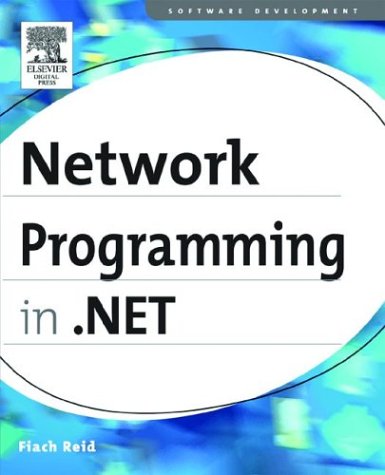
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NgenServicingAttributes.cs
- _Win32.cs
- Nullable.cs
- SystemDropShadowChrome.cs
- XmlAnyElementAttributes.cs
- ConcurrencyBehavior.cs
- VectorValueSerializer.cs
- DataServiceConfiguration.cs
- RootAction.cs
- GetPageCompletedEventArgs.cs
- SingleAnimationUsingKeyFrames.cs
- EventArgs.cs
- PersonalizableAttribute.cs
- KeyProperty.cs
- RepeaterItem.cs
- HWStack.cs
- TemplateBamlRecordReader.cs
- FlowLayout.cs
- NamedPipeHostedTransportConfiguration.cs
- SqlConnectionHelper.cs
- AssemblyBuilder.cs
- SmtpException.cs
- Metafile.cs
- RuntimeIdentifierPropertyAttribute.cs
- Pen.cs
- XamlSerializationHelper.cs
- LiteralLink.cs
- InstanceDataCollectionCollection.cs
- CollectionBase.cs
- HttpHeaderCollection.cs
- AttributeSetAction.cs
- AnnotationAdorner.cs
- CodeGenerator.cs
- KeyValueConfigurationCollection.cs
- AuthenticationConfig.cs
- StylusCaptureWithinProperty.cs
- TransformerConfigurationWizardBase.cs
- UnsafeNativeMethods.cs
- GradientBrush.cs
- VectorKeyFrameCollection.cs
- ImmutableClientRuntime.cs
- StrongNameMembershipCondition.cs
- XmlMtomWriter.cs
- DesignerActionGlyph.cs
- SerializerDescriptor.cs
- ViewStateException.cs
- TabItemWrapperAutomationPeer.cs
- RenderDataDrawingContext.cs
- TimeIntervalCollection.cs
- WebPartEditorApplyVerb.cs
- WorkflowExecutor.cs
- DrawingGroup.cs
- XmlAutoDetectWriter.cs
- WsatProxy.cs
- DateTimeHelper.cs
- StrokeSerializer.cs
- EnumValAlphaComparer.cs
- SecurityTokenRequirement.cs
- TextBox.cs
- DecimalStorage.cs
- XmlEncoding.cs
- LocatorGroup.cs
- CodeConditionStatement.cs
- ConfigXmlCDataSection.cs
- ApplicationFileCodeDomTreeGenerator.cs
- WsiProfilesElementCollection.cs
- UnsafeNativeMethods.cs
- TraceSwitch.cs
- StoreContentChangedEventArgs.cs
- odbcmetadatacollectionnames.cs
- XmlSerializer.cs
- InputReferenceExpression.cs
- SecurityResources.cs
- KeyFrames.cs
- FlowLayoutSettings.cs
- NetworkInformationPermission.cs
- FactoryId.cs
- CircleHotSpot.cs
- HttpInputStream.cs
- CodeTryCatchFinallyStatement.cs
- VirtualizingStackPanel.cs
- NativeMethodsOther.cs
- BaseCollection.cs
- PlainXmlDeserializer.cs
- XamlContextStack.cs
- Configuration.cs
- CorrelationService.cs
- PolicyChain.cs
- MissingManifestResourceException.cs
- DataControlCommands.cs
- MediaScriptCommandRoutedEventArgs.cs
- CssClassPropertyAttribute.cs
- CurrencyManager.cs
- XmlPropertyBag.cs
- ObjectDataSourceView.cs
- MobileRedirect.cs
- ApplicationBuildProvider.cs
- DataGridViewImageCell.cs
- WindowsFormsHostPropertyMap.cs
- IntegerValidatorAttribute.cs