Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / KeyProperty.cs / 1 / KeyProperty.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents PropertyRef Element for Entity keys and referential constraints /// internal sealed class PropertyRefElement : SchemaElement { #region Instance Fields private StructuredProperty _property = null; #endregion #region Public Methods ////// construct a KeyProperty object /// /// public PropertyRefElement(SchemaElement parentElement) : base(parentElement) { } #endregion #region Public Properties ////// property chain from KeyedType to Leaf property /// public StructuredProperty Property { get { return _property; } } #endregion #region Private Methods internal override void ResolveTopLevelNames() { Debug.Assert(false, "This method should never be used. Use other overload instead"); } ////// Since this method can be used in different context, this method does not add any errors /// Please make sure that the caller of this methods handles the error case and add errors /// appropriately /// /// ///internal bool ResolveNames(SchemaEntityType entityType) { if (string.IsNullOrEmpty(this.Name)) { // Don't flag this error. This must already must have flaged as error, while handling name attribute return true; } // Make sure there is a property by this name _property = entityType.FindProperty(this.Name); return (_property != null); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents PropertyRef Element for Entity keys and referential constraints /// internal sealed class PropertyRefElement : SchemaElement { #region Instance Fields private StructuredProperty _property = null; #endregion #region Public Methods ////// construct a KeyProperty object /// /// public PropertyRefElement(SchemaElement parentElement) : base(parentElement) { } #endregion #region Public Properties ////// property chain from KeyedType to Leaf property /// public StructuredProperty Property { get { return _property; } } #endregion #region Private Methods internal override void ResolveTopLevelNames() { Debug.Assert(false, "This method should never be used. Use other overload instead"); } ////// Since this method can be used in different context, this method does not add any errors /// Please make sure that the caller of this methods handles the error case and add errors /// appropriately /// /// ///internal bool ResolveNames(SchemaEntityType entityType) { if (string.IsNullOrEmpty(this.Name)) { // Don't flag this error. This must already must have flaged as error, while handling name attribute return true; } // Make sure there is a property by this name _property = entityType.FindProperty(this.Name); return (_property != null); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
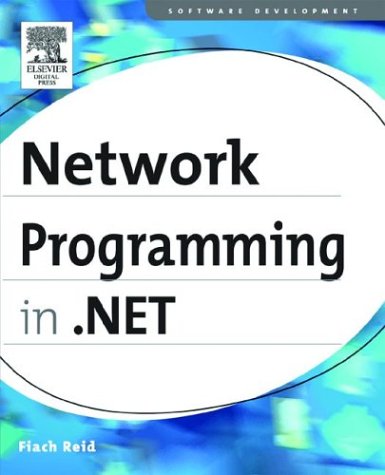
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CursorConverter.cs
- TemplateControlBuildProvider.cs
- FlowDocumentPaginator.cs
- InfoCardSymmetricAlgorithm.cs
- PropertyChangingEventArgs.cs
- XmlSchemaInclude.cs
- DataKeyArray.cs
- StackSpiller.cs
- SiteOfOriginPart.cs
- WebPartAuthorizationEventArgs.cs
- NameValuePair.cs
- WpfGeneratedKnownProperties.cs
- SrgsElementFactoryCompiler.cs
- IncrementalCompileAnalyzer.cs
- WpfWebRequestHelper.cs
- dbenumerator.cs
- NetDataContractSerializer.cs
- ItemChangedEventArgs.cs
- Attribute.cs
- PropertyMetadata.cs
- CheckBoxFlatAdapter.cs
- ContentElement.cs
- BuildManager.cs
- ResourceDictionary.cs
- WorkflowViewService.cs
- ArithmeticException.cs
- ProcessHostMapPath.cs
- EntityAdapter.cs
- ExpressionEditorAttribute.cs
- HScrollProperties.cs
- ParserHooks.cs
- ProfileSettingsCollection.cs
- SimpleTextLine.cs
- TextCollapsingProperties.cs
- ImageSource.cs
- EventListenerClientSide.cs
- BooleanToVisibilityConverter.cs
- JulianCalendar.cs
- ObjRef.cs
- PassportAuthenticationModule.cs
- DesignerCapabilities.cs
- PropertyMap.cs
- ChangePassword.cs
- TextBreakpoint.cs
- CommandHelpers.cs
- PeerObject.cs
- ResourceDisplayNameAttribute.cs
- BasePropertyDescriptor.cs
- CompilerResults.cs
- SerializationTrace.cs
- DataDocumentXPathNavigator.cs
- AssociationSet.cs
- SecurityMessageProperty.cs
- diagnosticsswitches.cs
- SafeMILHandle.cs
- TypeSystemProvider.cs
- PathGeometry.cs
- GridViewSelectEventArgs.cs
- TraceData.cs
- DateTimeFormatInfo.cs
- KeyNameIdentifierClause.cs
- URLMembershipCondition.cs
- CfgRule.cs
- DefaultEvaluationContext.cs
- KeyFrames.cs
- InfoCardKeyedHashAlgorithm.cs
- RuntimeCompatibilityAttribute.cs
- StorageConditionPropertyMapping.cs
- Transform3D.cs
- EmissiveMaterial.cs
- InstanceData.cs
- KeyboardDevice.cs
- MatrixCamera.cs
- Underline.cs
- GlyphRunDrawing.cs
- WhitespaceReader.cs
- LineSegment.cs
- CodeStatement.cs
- XmlText.cs
- QilStrConcatenator.cs
- PolicyChain.cs
- TemplateEditingFrame.cs
- ListViewDeletedEventArgs.cs
- EdmToObjectNamespaceMap.cs
- TableItemProviderWrapper.cs
- WinEventWrap.cs
- PageAsyncTaskManager.cs
- BulletedList.cs
- JsonReaderWriterFactory.cs
- TextMessageEncodingElement.cs
- AndMessageFilter.cs
- TextEditorCharacters.cs
- IncomingWebRequestContext.cs
- PropertyContainer.cs
- SmtpClient.cs
- DiagnosticTrace.cs
- NativeMethods.cs
- CorrelationManager.cs
- ResourceSet.cs
- DataPointer.cs