Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / AssociationSet.cs / 1305376 / AssociationSet.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Class for representing an Association set /// public sealed class AssociationSet : RelationshipSet { #region Constructors ////// Initializes a new instance of AssocationSet with the given name and the association type /// /// The name of the Assocation set /// The association type of the entities that this associationship set type contains internal AssociationSet(string name, AssociationType associationType) : base(name, null, null, null, associationType) { } #endregion #region Fields private readonly ReadOnlyMetadataCollection_associationSetEnds = new ReadOnlyMetadataCollection (new MetadataCollection ()); #endregion #region Properties /// /// Returns the association type associated with this association set /// public new AssociationType ElementType { get { return (AssociationType)base.ElementType; } } ////// Returns the ends of the association set /// [MetadataProperty(BuiltInTypeKind.AssociationSetEnd, true)] public ReadOnlyMetadataCollectionAssociationSetEnds { get { return _associationSetEnds; } } /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.AssociationSet; } } #endregion #region Methods ////// Sets this item to be readonly, once this is set, the item will never be writable again. /// internal override void SetReadOnly() { if (!this.IsReadOnly) { base.SetReadOnly(); this.AssociationSetEnds.Source.SetReadOnly(); } } ////// Adds the given end to the collection of ends /// /// internal void AddAssociationSetEnd(AssociationSetEnd associationSetEnd) { this.AssociationSetEnds.Source.Add(associationSetEnd); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Class for representing an Association set /// public sealed class AssociationSet : RelationshipSet { #region Constructors ////// Initializes a new instance of AssocationSet with the given name and the association type /// /// The name of the Assocation set /// The association type of the entities that this associationship set type contains internal AssociationSet(string name, AssociationType associationType) : base(name, null, null, null, associationType) { } #endregion #region Fields private readonly ReadOnlyMetadataCollection_associationSetEnds = new ReadOnlyMetadataCollection (new MetadataCollection ()); #endregion #region Properties /// /// Returns the association type associated with this association set /// public new AssociationType ElementType { get { return (AssociationType)base.ElementType; } } ////// Returns the ends of the association set /// [MetadataProperty(BuiltInTypeKind.AssociationSetEnd, true)] public ReadOnlyMetadataCollectionAssociationSetEnds { get { return _associationSetEnds; } } /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.AssociationSet; } } #endregion #region Methods ////// Sets this item to be readonly, once this is set, the item will never be writable again. /// internal override void SetReadOnly() { if (!this.IsReadOnly) { base.SetReadOnly(); this.AssociationSetEnds.Source.SetReadOnly(); } } ////// Adds the given end to the collection of ends /// /// internal void AddAssociationSetEnd(AssociationSetEnd associationSetEnd) { this.AssociationSetEnds.Source.Add(associationSetEnd); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
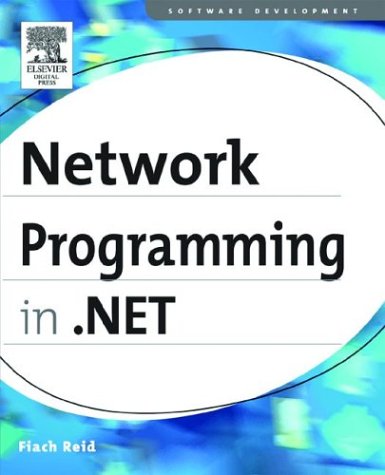
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectToIdCache.cs
- XmlAnyElementAttribute.cs
- Int64.cs
- WebPartManagerInternals.cs
- IsolatedStorageFilePermission.cs
- VScrollBar.cs
- CurrentTimeZone.cs
- SettingsProviderCollection.cs
- QilScopedVisitor.cs
- EncodingNLS.cs
- ToolZone.cs
- peersecuritysettings.cs
- HandlerMappingMemo.cs
- CryptoConfig.cs
- DataGridViewTextBoxEditingControl.cs
- XPathNode.cs
- WindowsListView.cs
- SignatureDescription.cs
- PageAsyncTaskManager.cs
- QueueProcessor.cs
- OdbcConnectionStringbuilder.cs
- StateManagedCollection.cs
- BitmapInitialize.cs
- HybridDictionary.cs
- UndirectedGraph.cs
- SEHException.cs
- FieldNameLookup.cs
- InvokePattern.cs
- TypeElement.cs
- ToolStripContainerActionList.cs
- ActiveXContainer.cs
- ColumnProvider.cs
- CLSCompliantAttribute.cs
- EditorResources.cs
- FileDialog.cs
- isolationinterop.cs
- EditorZoneBase.cs
- EmptyStringExpandableObjectConverter.cs
- SmiMetaData.cs
- NotifyInputEventArgs.cs
- RegexMatchCollection.cs
- MachineKeyConverter.cs
- OperationResponse.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- Directory.cs
- MetadataCacheItem.cs
- DBPropSet.cs
- ReliableOutputSessionChannel.cs
- HtmlInputHidden.cs
- TypeLibraryHelper.cs
- QueryProcessor.cs
- TemplatePartAttribute.cs
- XamlInt32CollectionSerializer.cs
- SimpleTextLine.cs
- SqlCacheDependency.cs
- Int32Storage.cs
- TemplatePropertyEntry.cs
- InputMethodStateChangeEventArgs.cs
- TransactionInterop.cs
- LassoSelectionBehavior.cs
- AstTree.cs
- TransformGroup.cs
- SqlProcedureAttribute.cs
- ConditionalAttribute.cs
- TextRangeAdaptor.cs
- WindowsToolbar.cs
- ExtendedPropertyCollection.cs
- ApplicationBuildProvider.cs
- SqlUtil.cs
- TextEmbeddedObject.cs
- ItemCheckedEvent.cs
- SecurityPermission.cs
- TextBoxDesigner.cs
- ReceiveMessageRecord.cs
- SchemaCollectionCompiler.cs
- SystemInformation.cs
- CodeSubDirectoriesCollection.cs
- IEnumerable.cs
- ResourceDescriptionAttribute.cs
- OleDbConnection.cs
- BamlReader.cs
- CryptoStream.cs
- PropertyInfo.cs
- MasterPage.cs
- Thread.cs
- BoundField.cs
- ACL.cs
- HitTestFilterBehavior.cs
- MemberDescriptor.cs
- HandoffBehavior.cs
- EventProviderBase.cs
- Manipulation.cs
- LoginCancelEventArgs.cs
- TabletDevice.cs
- DrawTreeNodeEventArgs.cs
- IpcChannelHelper.cs
- RectangleHotSpot.cs
- CallInfo.cs
- HttpCachePolicy.cs
- DateTimeOffsetConverter.cs