Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Manipulation.cs / 1305600 / Manipulation.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Input.Manipulations; using MS.Internal.PresentationCore; namespace System.Windows.Input { ////// Provides access to various features and settings of manipulation. /// public static class Manipulation { internal static readonly RoutedEvent ManipulationStartingEvent = EventManager.RegisterRoutedEvent("ManipulationStarting", RoutingStrategy.Bubble, typeof(EventHandler), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationStartedEvent = EventManager.RegisterRoutedEvent("ManipulationStarted", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationDeltaEvent = EventManager.RegisterRoutedEvent("ManipulationDelta", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationInertiaStartingEvent = EventManager.RegisterRoutedEvent("ManipulationInertiaStarting", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationBoundaryFeedbackEvent = EventManager.RegisterRoutedEvent("ManipulationBoundaryFeedback", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationCompletedEvent = EventManager.RegisterRoutedEvent("ManipulationCompleted", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); /// /// Determines if a manipulation is currently active on an element. /// /// Specifies the element that may or may not have a manipulation. ///True if there is an active manipulation, false otherwise. public static bool IsManipulationActive(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return GetActiveManipulationDevice(element) != null; } private static ManipulationDevice GetActiveManipulationDevice(UIElement element) { Debug.Assert(element != null, "element should be non-null."); ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if ((device != null) && device.IsManipulationActive) { return device; } return null; } ////// If a manipulation is active, forces the manipulation to proceed to the inertia phase. /// If inertia is already occurring, it will restart inertia. /// /// The element on which there is an active manipulation. public static void StartInertia(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { device.CompleteManipulation(/* withInertia = */ true); } } ////// If a manipulation is active, forces the manipulation to complete. /// /// The element on which there is an active manipulation. public static void CompleteManipulation(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } if (!TryCompleteManipulation(element)) { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } internal static bool TryCompleteManipulation(UIElement element) { ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { device.CompleteManipulation(/* withInertia = */ false); return true; } return false; } ////// Changes the manipulation mode of an active manipulation. /// /// The element on which there is an active manipulation. /// The new manipulation mode. public static void SetManipulationMode(UIElement element, ManipulationModes mode) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = GetActiveManipulationDevice(element); if (device != null) { device.ManipulationMode = mode; } else { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } ////// Retrieves the current manipulation mode of an active manipulation. /// /// The element on which there is an active manipulation. ///The current manipulation mode. public static ManipulationModes GetManipulationMode(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { return device.ManipulationMode; } else { return ManipulationModes.None; } } ////// Changes the container that defines the coordinate space of event data /// for an active manipulation. /// /// The element on which there is an active manipulation. /// The container that defines the coordinate space. public static void SetManipulationContainer(UIElement element, IInputElement container) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = GetActiveManipulationDevice(element); if (device != null) { device.ManipulationContainer = container; } else { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } ////// Retrieves the container that defines the coordinate space of event data /// for an active manipulation. /// /// The element on which there is an active manipulation. ///The container that defines the coordinate space. public static IInputElement GetManipulationContainer(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { return device.ManipulationContainer; } return null; } ////// Changes the pivot for single-finger manipulation on an active manipulation. /// /// The element on which there is an active manipulation. /// The new pivot for single-finger manipulation. public static void SetManipulationPivot(UIElement element, ManipulationPivot pivot) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = GetActiveManipulationDevice(element); if (device != null) { device.ManipulationPivot = pivot; } else { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } ////// Retrieves the pivot for single-finger manipulation on an active manipulation. /// /// The element on which there is an active manipulation. ///The pivot for single-finger manipulation. public static ManipulationPivot GetManipulationPivot(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { return device.ManipulationPivot; } return null; } ////// Associates a manipulator with a UIElement. This will either will start an /// active manipulation or add to an existing one. /// /// The element with which to associate the manipulator. /// The manipulator, such as a TouchDevice. public static void AddManipulator(UIElement element, IManipulator manipulator) { if (element == null) { throw new ArgumentNullException("element"); } if (manipulator == null) { throw new ArgumentNullException("manipulator"); } if (!element.IsManipulationEnabled) { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotEnabled)); } ManipulationDevice device = ManipulationDevice.AddManipulationDevice(element); device.AddManipulator(manipulator); } ////// Disassociates a manipulator with a UIElement. This will remove it from /// an active manipulation, possibly completing it. /// /// The element that the manipulator used to be associated with. /// The manipulator, such as a TouchDevice. public static void RemoveManipulator(UIElement element, IManipulator manipulator) { if (element == null) { throw new ArgumentNullException("element"); } if (manipulator == null) { throw new ArgumentNullException("manipulator"); } if (!TryRemoveManipulator(element, manipulator)) { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } internal static bool TryRemoveManipulator(UIElement element, IManipulator manipulator) { ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { device.RemoveManipulator(manipulator); return true; } return false; } ////// Adds a ManipulationParameters2D object to an active manipulation. /// /// The element on which there is an active manipulation. /// The parameter. [Browsable(false)] public static void SetManipulationParameter(UIElement element, ManipulationParameters2D parameter) { if (element == null) { throw new ArgumentNullException("element"); } if (parameter == null) { throw new ArgumentNullException("parameter"); } ManipulationDevice device = GetActiveManipulationDevice(element); if (device != null) { device.SetManipulationParameters(parameter); } else { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } ////// Searches from the specified visual (inclusively) up the visual tree /// for a UIElement that has a ManipulationMode that desires manipulation events. /// /// /// The starting element. Usually, this is an element that an input device has hit-tested to. /// ////// A UIElement that has ManipulationMode set to Translate, Scale, Rotate, or some combination of the three. /// If no element is found, then null is returned. /// ////// This function reads data that is thread-bound and should be /// called on the same thread that 'visual' is bound to. /// internal static UIElement FindManipulationParent(Visual visual) { while (visual != null) { UIElement element = visual as UIElement; if ((element != null) && element.IsManipulationEnabled) { return element; } else { visual = VisualTreeHelper.GetParent(visual) as Visual; } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Input.Manipulations; using MS.Internal.PresentationCore; namespace System.Windows.Input { ////// Provides access to various features and settings of manipulation. /// public static class Manipulation { internal static readonly RoutedEvent ManipulationStartingEvent = EventManager.RegisterRoutedEvent("ManipulationStarting", RoutingStrategy.Bubble, typeof(EventHandler), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationStartedEvent = EventManager.RegisterRoutedEvent("ManipulationStarted", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationDeltaEvent = EventManager.RegisterRoutedEvent("ManipulationDelta", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationInertiaStartingEvent = EventManager.RegisterRoutedEvent("ManipulationInertiaStarting", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationBoundaryFeedbackEvent = EventManager.RegisterRoutedEvent("ManipulationBoundaryFeedback", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); internal static readonly RoutedEvent ManipulationCompletedEvent = EventManager.RegisterRoutedEvent("ManipulationCompleted", RoutingStrategy.Bubble, typeof(EventHandler ), typeof(ManipulationDevice)); /// /// Determines if a manipulation is currently active on an element. /// /// Specifies the element that may or may not have a manipulation. ///True if there is an active manipulation, false otherwise. public static bool IsManipulationActive(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } return GetActiveManipulationDevice(element) != null; } private static ManipulationDevice GetActiveManipulationDevice(UIElement element) { Debug.Assert(element != null, "element should be non-null."); ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if ((device != null) && device.IsManipulationActive) { return device; } return null; } ////// If a manipulation is active, forces the manipulation to proceed to the inertia phase. /// If inertia is already occurring, it will restart inertia. /// /// The element on which there is an active manipulation. public static void StartInertia(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { device.CompleteManipulation(/* withInertia = */ true); } } ////// If a manipulation is active, forces the manipulation to complete. /// /// The element on which there is an active manipulation. public static void CompleteManipulation(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } if (!TryCompleteManipulation(element)) { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } internal static bool TryCompleteManipulation(UIElement element) { ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { device.CompleteManipulation(/* withInertia = */ false); return true; } return false; } ////// Changes the manipulation mode of an active manipulation. /// /// The element on which there is an active manipulation. /// The new manipulation mode. public static void SetManipulationMode(UIElement element, ManipulationModes mode) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = GetActiveManipulationDevice(element); if (device != null) { device.ManipulationMode = mode; } else { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } ////// Retrieves the current manipulation mode of an active manipulation. /// /// The element on which there is an active manipulation. ///The current manipulation mode. public static ManipulationModes GetManipulationMode(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { return device.ManipulationMode; } else { return ManipulationModes.None; } } ////// Changes the container that defines the coordinate space of event data /// for an active manipulation. /// /// The element on which there is an active manipulation. /// The container that defines the coordinate space. public static void SetManipulationContainer(UIElement element, IInputElement container) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = GetActiveManipulationDevice(element); if (device != null) { device.ManipulationContainer = container; } else { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } ////// Retrieves the container that defines the coordinate space of event data /// for an active manipulation. /// /// The element on which there is an active manipulation. ///The container that defines the coordinate space. public static IInputElement GetManipulationContainer(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { return device.ManipulationContainer; } return null; } ////// Changes the pivot for single-finger manipulation on an active manipulation. /// /// The element on which there is an active manipulation. /// The new pivot for single-finger manipulation. public static void SetManipulationPivot(UIElement element, ManipulationPivot pivot) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = GetActiveManipulationDevice(element); if (device != null) { device.ManipulationPivot = pivot; } else { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } ////// Retrieves the pivot for single-finger manipulation on an active manipulation. /// /// The element on which there is an active manipulation. ///The pivot for single-finger manipulation. public static ManipulationPivot GetManipulationPivot(UIElement element) { if (element == null) { throw new ArgumentNullException("element"); } ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { return device.ManipulationPivot; } return null; } ////// Associates a manipulator with a UIElement. This will either will start an /// active manipulation or add to an existing one. /// /// The element with which to associate the manipulator. /// The manipulator, such as a TouchDevice. public static void AddManipulator(UIElement element, IManipulator manipulator) { if (element == null) { throw new ArgumentNullException("element"); } if (manipulator == null) { throw new ArgumentNullException("manipulator"); } if (!element.IsManipulationEnabled) { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotEnabled)); } ManipulationDevice device = ManipulationDevice.AddManipulationDevice(element); device.AddManipulator(manipulator); } ////// Disassociates a manipulator with a UIElement. This will remove it from /// an active manipulation, possibly completing it. /// /// The element that the manipulator used to be associated with. /// The manipulator, such as a TouchDevice. public static void RemoveManipulator(UIElement element, IManipulator manipulator) { if (element == null) { throw new ArgumentNullException("element"); } if (manipulator == null) { throw new ArgumentNullException("manipulator"); } if (!TryRemoveManipulator(element, manipulator)) { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } internal static bool TryRemoveManipulator(UIElement element, IManipulator manipulator) { ManipulationDevice device = ManipulationDevice.GetManipulationDevice(element); if (device != null) { device.RemoveManipulator(manipulator); return true; } return false; } ////// Adds a ManipulationParameters2D object to an active manipulation. /// /// The element on which there is an active manipulation. /// The parameter. [Browsable(false)] public static void SetManipulationParameter(UIElement element, ManipulationParameters2D parameter) { if (element == null) { throw new ArgumentNullException("element"); } if (parameter == null) { throw new ArgumentNullException("parameter"); } ManipulationDevice device = GetActiveManipulationDevice(element); if (device != null) { device.SetManipulationParameters(parameter); } else { throw new InvalidOperationException(SR.Get(SRID.Manipulation_ManipulationNotActive)); } } ////// Searches from the specified visual (inclusively) up the visual tree /// for a UIElement that has a ManipulationMode that desires manipulation events. /// /// /// The starting element. Usually, this is an element that an input device has hit-tested to. /// ////// A UIElement that has ManipulationMode set to Translate, Scale, Rotate, or some combination of the three. /// If no element is found, then null is returned. /// ////// This function reads data that is thread-bound and should be /// called on the same thread that 'visual' is bound to. /// internal static UIElement FindManipulationParent(Visual visual) { while (visual != null) { UIElement element = visual as UIElement; if ((element != null) && element.IsManipulationEnabled) { return element; } else { visual = VisualTreeHelper.GetParent(visual) as Visual; } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
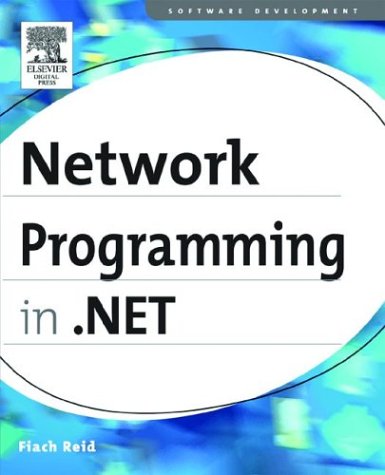
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridEntryCollection.cs
- InstanceDataCollectionCollection.cs
- NonPrimarySelectionGlyph.cs
- GenericTextProperties.cs
- RichTextBox.cs
- ReadOnlyHierarchicalDataSourceView.cs
- Byte.cs
- JavaScriptSerializer.cs
- XamlPointCollectionSerializer.cs
- RemotingConfiguration.cs
- _SingleItemRequestCache.cs
- CounterSampleCalculator.cs
- WebBodyFormatMessageProperty.cs
- HostSecurityManager.cs
- FunctionDetailsReader.cs
- AdapterSwitches.cs
- SQLConvert.cs
- FixedTextContainer.cs
- SqlCacheDependency.cs
- DependencyPropertyDescriptor.cs
- OutputScopeManager.cs
- InkCanvasSelection.cs
- AsymmetricKeyExchangeFormatter.cs
- CodeIdentifiers.cs
- XmlEncodedRawTextWriter.cs
- Crypto.cs
- MethodAccessException.cs
- DomainConstraint.cs
- PrinterUnitConvert.cs
- ShaderRenderModeValidation.cs
- TableHeaderCell.cs
- SmtpSection.cs
- IMembershipProvider.cs
- DataTemplateSelector.cs
- HostingPreferredMapPath.cs
- MeasurementDCInfo.cs
- ObjectTokenCategory.cs
- ConfigurationManager.cs
- EncryptedData.cs
- LogConverter.cs
- PlanCompilerUtil.cs
- RectKeyFrameCollection.cs
- EventLogEntryCollection.cs
- FormsAuthenticationTicket.cs
- RequestSecurityTokenForGetBrowserToken.cs
- TextRangeEdit.cs
- AttributeCollection.cs
- Focus.cs
- CalendarTable.cs
- EventMappingSettings.cs
- CompensatableSequenceActivity.cs
- GridViewSortEventArgs.cs
- DataBindingCollection.cs
- Html32TextWriter.cs
- UInt16.cs
- FormatterConverter.cs
- Point3DKeyFrameCollection.cs
- SubpageParagraph.cs
- BaseProcessor.cs
- ClickablePoint.cs
- CompositeActivityTypeDescriptorProvider.cs
- ConfigurationException.cs
- OdbcEnvironmentHandle.cs
- PersonalizationStateInfoCollection.cs
- ToolStripPanel.cs
- BinHexDecoder.cs
- FontCacheUtil.cs
- SortQueryOperator.cs
- SessionStateSection.cs
- EventWaitHandleSecurity.cs
- CellTreeSimplifier.cs
- SchemaLookupTable.cs
- TextBlockAutomationPeer.cs
- HttpClientCertificate.cs
- WorkerRequest.cs
- TextWriterTraceListener.cs
- WCFServiceClientProxyGenerator.cs
- TypeConverterAttribute.cs
- Message.cs
- GeometryGroup.cs
- CodeStatementCollection.cs
- CodeSnippetStatement.cs
- xsdvalidator.cs
- EndPoint.cs
- PartialTrustVisibleAssemblyCollection.cs
- Fx.cs
- KeyedCollection.cs
- peernodestatemanager.cs
- TextShapeableCharacters.cs
- DiagnosticTraceSource.cs
- Query.cs
- UnaryNode.cs
- SQLInt16Storage.cs
- ComponentResourceKeyConverter.cs
- GeometryModel3D.cs
- TextParaLineResult.cs
- DbParameterCollectionHelper.cs
- ColumnHeaderConverter.cs
- PartitionedStreamMerger.cs
- EntityCommandCompilationException.cs