Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / PropertyGridInternal / GridEntryCollection.cs / 1305376 / GridEntryCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.PropertyGridInternal { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Collections; using System.Reflection; using System.Drawing.Design; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms; using System.Windows.Forms.Design; using System.Drawing; using Microsoft.Win32; internal class GridEntryCollection : GridItemCollection { private GridEntry owner; public GridEntryCollection(GridEntry owner, GridEntry[] entries) : base(entries) { this.owner = owner; } public void AddRange(GridEntry[] value) { if (value == null) { throw new ArgumentNullException("value"); } if (entries != null) { GridEntry[] newArray = new GridEntry[entries.Length + value.Length]; entries.CopyTo(newArray, 0); value.CopyTo(newArray, entries.Length); entries = newArray; } else { entries = (GridEntry[])value.Clone(); } } public void Clear() { entries = new GridEntry[0]; } public void CopyTo(Array dest, int index) { entries.CopyTo(dest, index); } internal GridEntry GetEntry(int index) { return (GridEntry)entries[index]; } internal int GetEntry(GridEntry child) { return Array.IndexOf(entries, child); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } protected virtual void Dispose(bool disposing) { if (disposing) { if (owner != null && entries != null) { for (int i = 0; i < entries.Length; i++) { if (entries[i] != null) { ((GridEntry)entries[i]).Dispose(); entries[i] = null; } } entries = new GridEntry[0]; } } } ~GridEntryCollection() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
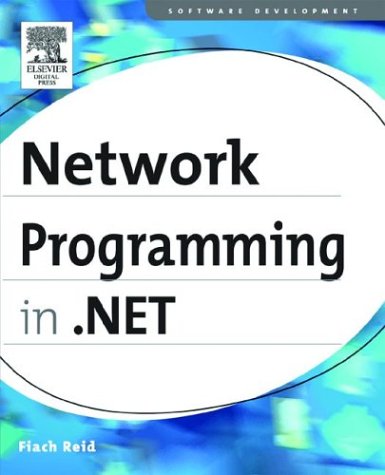
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Config.cs
- TemplateLookupAction.cs
- DocumentPaginator.cs
- UnauthorizedWebPart.cs
- Reference.cs
- PlanCompiler.cs
- StateChangeEvent.cs
- PrintController.cs
- SecurityElement.cs
- EntityTypeBase.cs
- TextParagraphView.cs
- DataGridViewCellStateChangedEventArgs.cs
- Propagator.cs
- EventDescriptorCollection.cs
- ConditionCollection.cs
- PnrpPermission.cs
- ScriptIgnoreAttribute.cs
- HtmlDocument.cs
- ConsoleKeyInfo.cs
- MsmqHostedTransportConfiguration.cs
- MetadataCollection.cs
- PageRanges.cs
- PersistenceTypeAttribute.cs
- SoapSchemaImporter.cs
- UrlParameterReader.cs
- TrimSurroundingWhitespaceAttribute.cs
- MenuTracker.cs
- DescendentsWalkerBase.cs
- IsolatedStorageFilePermission.cs
- WindowsImpersonationContext.cs
- CallbackBehaviorAttribute.cs
- UTF32Encoding.cs
- ApplicationInfo.cs
- WebBaseEventKeyComparer.cs
- DataSourceProvider.cs
- DataGridViewSortCompareEventArgs.cs
- SmtpNegotiateAuthenticationModule.cs
- odbcmetadatafactory.cs
- ElementHost.cs
- ItemContainerGenerator.cs
- PageTrueTypeFont.cs
- VisualStyleElement.cs
- ButtonBase.cs
- SpeakProgressEventArgs.cs
- EmptyEnumerable.cs
- WebReferencesBuildProvider.cs
- XPathSingletonIterator.cs
- KeyValuePair.cs
- DataSourceSelectArguments.cs
- DataViewSettingCollection.cs
- ClientType.cs
- TableLayout.cs
- InputBuffer.cs
- basevalidator.cs
- XPathDocumentBuilder.cs
- UndirectedGraph.cs
- DataBindingCollection.cs
- RuleAttributes.cs
- PropertyValueUIItem.cs
- MeasureItemEvent.cs
- DataGridViewCheckBoxColumn.cs
- NavigateEvent.cs
- WebContext.cs
- DataRelation.cs
- BooleanStorage.cs
- ScriptHandlerFactory.cs
- HostVisual.cs
- SocketConnection.cs
- HasCopySemanticsAttribute.cs
- PersonalizationProvider.cs
- FragmentQueryKB.cs
- WizardStepBase.cs
- CompilerLocalReference.cs
- Main.cs
- TreeView.cs
- FormViewAutoFormat.cs
- WebSysDescriptionAttribute.cs
- NetworkInterface.cs
- DecoderReplacementFallback.cs
- XmlChoiceIdentifierAttribute.cs
- DesignerAdRotatorAdapter.cs
- StringArrayConverter.cs
- WindowsSecurityToken.cs
- WmlTextBoxAdapter.cs
- UnicastIPAddressInformationCollection.cs
- DeviceFiltersSection.cs
- TextPointer.cs
- SrgsGrammarCompiler.cs
- XmlAnyElementAttributes.cs
- TemplatedWizardStep.cs
- DirectoryNotFoundException.cs
- figurelength.cs
- PermissionRequestEvidence.cs
- IsolatedStorageException.cs
- DataSourceProvider.cs
- ParserStreamGeometryContext.cs
- QilCloneVisitor.cs
- ProfileEventArgs.cs
- StatusBarAutomationPeer.cs
- ImmutableCommunicationTimeouts.cs