Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CommonUI / System / Drawing / Printing / PrintController.cs / 1 / PrintController.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using Microsoft.Win32; using System; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ////// /// public abstract class PrintController { // DEVMODEs are pretty expensive, so we cache one here and share it with the // Standard and Preview print controllers. If it weren't for all the rules about API changes, // I'd consider making this protected. internal IntPtr modeHandle = IntPtr.Zero; // initialized in StartPrint ///Controls how a document is printed. ////// /// protected PrintController() { IntSecurity.SafePrinting.Demand(); } ////// Initializes a new instance of the ///class. /// /// /// public virtual bool IsPreview { get{ return false; } } // WARNING: if you have nested PrintControllers, this method won't get called on the inner one. // Add initialization code to StartPrint or StartPage instead. internal void Print(PrintDocument document) { IntSecurity.SafePrinting.Demand(); // Most of the printing security is left to the individual print controller // // Get the PrintAction for this event PrintAction printAction; if (IsPreview) { printAction = PrintAction.PrintToPreview; } else { printAction = document.PrinterSettings.PrintToFile ? PrintAction.PrintToFile : PrintAction.PrintToPrinter; } // Check that user has permission to print to this particular printer PrintEventArgs printEvent = new PrintEventArgs(printAction); document._OnBeginPrint(printEvent); if (printEvent.Cancel) { document._OnEndPrint(printEvent); return; } OnStartPrint(document, printEvent); if (printEvent.Cancel) { document._OnEndPrint(printEvent); OnEndPrint(document, printEvent); return; } bool canceled = true; try { canceled = PrintLoop(document); } finally { try { try { document._OnEndPrint(printEvent); printEvent.Cancel = canceled | printEvent.Cancel; } finally { OnEndPrint(document, printEvent); } } finally { if (!IntSecurity.HasPermission(IntSecurity.AllPrinting)) { // Ensure programs with SafePrinting only get to print once for each time they // throw up the PrintDialog. IntSecurity.AllPrinting.Assert(); document.PrinterSettings.PrintDialogDisplayed = false; } } } } // Returns true if print was aborted. // WARNING: if you have nested PrintControllers, this method won't get called on the inner one // Add initialization code to StartPrint or StartPage instead. private bool PrintLoop(PrintDocument document) { QueryPageSettingsEventArgs queryEvent = new QueryPageSettingsEventArgs((PageSettings) document.DefaultPageSettings.Clone()); for (;;) { document._OnQueryPageSettings(queryEvent); if (queryEvent.Cancel) { return true; } PrintPageEventArgs pageEvent = CreatePrintPageEvent(queryEvent.PageSettings); Graphics graphics = OnStartPage(document, pageEvent); pageEvent.SetGraphics(graphics); try { document._OnPrintPage(pageEvent); OnEndPage(document, pageEvent); } finally { pageEvent.Dispose(); } if (pageEvent.Cancel) { return true; } else if (!pageEvent.HasMorePages) { return false; } else { // loop } } } private PrintPageEventArgs CreatePrintPageEvent(PageSettings pageSettings) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); Debug.Assert(modeHandle != IntPtr.Zero, "modeHandle is null. Someone must have forgot to call base.StartPrint"); Rectangle pageBounds = pageSettings.GetBounds(modeHandle); Rectangle marginBounds = new Rectangle(pageSettings.Margins.Left, pageSettings.Margins.Top, pageBounds.Width - (pageSettings.Margins.Left + pageSettings.Margins.Right), pageBounds.Height - (pageSettings.Margins.Top + pageSettings.Margins.Bottom)); PrintPageEventArgs pageEvent = new PrintPageEventArgs(null, marginBounds, pageBounds, pageSettings); return pageEvent; } ////// This is new public property which notifies if this controller is used for PrintPreview. /// ////// /// public virtual void OnStartPrint(PrintDocument document, PrintEventArgs e) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); modeHandle = document.PrinterSettings.GetHdevmode(document.DefaultPageSettings); } ///When overridden in a derived class, begins the control sequence of when and how to print a document. ////// /// public virtual Graphics OnStartPage(PrintDocument document, PrintPageEventArgs e) { return null; } ///When overridden in a derived class, begins the control /// sequence of when and how to print a page in a document. ////// /// public virtual void OnEndPage(PrintDocument document, PrintPageEventArgs e) { } ///When overridden in a derived class, completes the control sequence of when and how /// to print a page in a document. ////// /// public virtual void OnEndPrint(PrintDocument document, PrintEventArgs e) { IntSecurity.UnmanagedCode.Assert(); Debug.Assert(modeHandle != IntPtr.Zero, "modeHandle is null. Someone must have forgot to call base.StartPrint"); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); modeHandle = IntPtr.Zero; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //When overridden in a derived class, completes the /// control sequence of when and how to print a document. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using Microsoft.Win32; using System; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ////// /// public abstract class PrintController { // DEVMODEs are pretty expensive, so we cache one here and share it with the // Standard and Preview print controllers. If it weren't for all the rules about API changes, // I'd consider making this protected. internal IntPtr modeHandle = IntPtr.Zero; // initialized in StartPrint ///Controls how a document is printed. ////// /// protected PrintController() { IntSecurity.SafePrinting.Demand(); } ////// Initializes a new instance of the ///class. /// /// /// public virtual bool IsPreview { get{ return false; } } // WARNING: if you have nested PrintControllers, this method won't get called on the inner one. // Add initialization code to StartPrint or StartPage instead. internal void Print(PrintDocument document) { IntSecurity.SafePrinting.Demand(); // Most of the printing security is left to the individual print controller // // Get the PrintAction for this event PrintAction printAction; if (IsPreview) { printAction = PrintAction.PrintToPreview; } else { printAction = document.PrinterSettings.PrintToFile ? PrintAction.PrintToFile : PrintAction.PrintToPrinter; } // Check that user has permission to print to this particular printer PrintEventArgs printEvent = new PrintEventArgs(printAction); document._OnBeginPrint(printEvent); if (printEvent.Cancel) { document._OnEndPrint(printEvent); return; } OnStartPrint(document, printEvent); if (printEvent.Cancel) { document._OnEndPrint(printEvent); OnEndPrint(document, printEvent); return; } bool canceled = true; try { canceled = PrintLoop(document); } finally { try { try { document._OnEndPrint(printEvent); printEvent.Cancel = canceled | printEvent.Cancel; } finally { OnEndPrint(document, printEvent); } } finally { if (!IntSecurity.HasPermission(IntSecurity.AllPrinting)) { // Ensure programs with SafePrinting only get to print once for each time they // throw up the PrintDialog. IntSecurity.AllPrinting.Assert(); document.PrinterSettings.PrintDialogDisplayed = false; } } } } // Returns true if print was aborted. // WARNING: if you have nested PrintControllers, this method won't get called on the inner one // Add initialization code to StartPrint or StartPage instead. private bool PrintLoop(PrintDocument document) { QueryPageSettingsEventArgs queryEvent = new QueryPageSettingsEventArgs((PageSettings) document.DefaultPageSettings.Clone()); for (;;) { document._OnQueryPageSettings(queryEvent); if (queryEvent.Cancel) { return true; } PrintPageEventArgs pageEvent = CreatePrintPageEvent(queryEvent.PageSettings); Graphics graphics = OnStartPage(document, pageEvent); pageEvent.SetGraphics(graphics); try { document._OnPrintPage(pageEvent); OnEndPage(document, pageEvent); } finally { pageEvent.Dispose(); } if (pageEvent.Cancel) { return true; } else if (!pageEvent.HasMorePages) { return false; } else { // loop } } } private PrintPageEventArgs CreatePrintPageEvent(PageSettings pageSettings) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); Debug.Assert(modeHandle != IntPtr.Zero, "modeHandle is null. Someone must have forgot to call base.StartPrint"); Rectangle pageBounds = pageSettings.GetBounds(modeHandle); Rectangle marginBounds = new Rectangle(pageSettings.Margins.Left, pageSettings.Margins.Top, pageBounds.Width - (pageSettings.Margins.Left + pageSettings.Margins.Right), pageBounds.Height - (pageSettings.Margins.Top + pageSettings.Margins.Bottom)); PrintPageEventArgs pageEvent = new PrintPageEventArgs(null, marginBounds, pageBounds, pageSettings); return pageEvent; } ////// This is new public property which notifies if this controller is used for PrintPreview. /// ////// /// public virtual void OnStartPrint(PrintDocument document, PrintEventArgs e) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); modeHandle = document.PrinterSettings.GetHdevmode(document.DefaultPageSettings); } ///When overridden in a derived class, begins the control sequence of when and how to print a document. ////// /// public virtual Graphics OnStartPage(PrintDocument document, PrintPageEventArgs e) { return null; } ///When overridden in a derived class, begins the control /// sequence of when and how to print a page in a document. ////// /// public virtual void OnEndPage(PrintDocument document, PrintPageEventArgs e) { } ///When overridden in a derived class, completes the control sequence of when and how /// to print a page in a document. ////// /// public virtual void OnEndPrint(PrintDocument document, PrintEventArgs e) { IntSecurity.UnmanagedCode.Assert(); Debug.Assert(modeHandle != IntPtr.Zero, "modeHandle is null. Someone must have forgot to call base.StartPrint"); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); modeHandle = IntPtr.Zero; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.When overridden in a derived class, completes the /// control sequence of when and how to print a document. ///
Link Menu
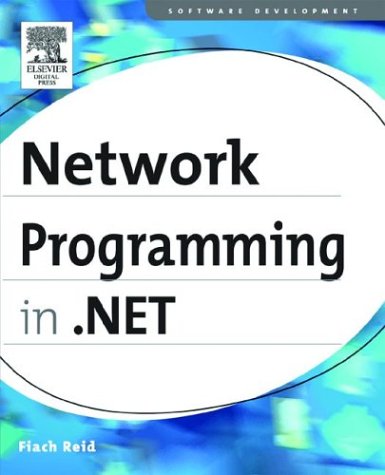
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaImporter.cs
- DbConnectionClosed.cs
- Rfc2898DeriveBytes.cs
- BinaryWriter.cs
- WebServiceEnumData.cs
- MetadataWorkspace.cs
- RefExpr.cs
- MenuItemStyleCollection.cs
- WebConfigurationFileMap.cs
- TextRangeEdit.cs
- ListSourceHelper.cs
- AttributeAction.cs
- Wildcard.cs
- Label.cs
- DataGridViewBand.cs
- BinaryWriter.cs
- JsonMessageEncoderFactory.cs
- SafeFileMappingHandle.cs
- ReferenceEqualityComparer.cs
- DebugView.cs
- LinkedResourceCollection.cs
- XhtmlBasicListAdapter.cs
- RightsManagementLicense.cs
- XmlLoader.cs
- PopOutPanel.cs
- ReliabilityContractAttribute.cs
- GroupDescription.cs
- SecurityDescriptor.cs
- HeaderCollection.cs
- ImageAutomationPeer.cs
- DataBindingCollection.cs
- CollectionEditor.cs
- ItemContainerGenerator.cs
- _AuthenticationState.cs
- ListBoxItem.cs
- BindingElementCollection.cs
- WeakReferenceEnumerator.cs
- coordinator.cs
- EdmToObjectNamespaceMap.cs
- ComplexPropertyEntry.cs
- SafeRightsManagementPubHandle.cs
- WindowsHyperlink.cs
- ContravarianceAdapter.cs
- OpenFileDialog.cs
- ImportedNamespaceContextItem.cs
- LoginUtil.cs
- CharUnicodeInfo.cs
- VarRemapper.cs
- PriorityBindingExpression.cs
- RtfControlWordInfo.cs
- CompilerScope.cs
- AvTraceFormat.cs
- TextProperties.cs
- SqlGatherProducedAliases.cs
- ButtonFieldBase.cs
- PageTheme.cs
- ExecutionContext.cs
- ListItemCollection.cs
- BinaryParser.cs
- PageHandlerFactory.cs
- MulticastOption.cs
- ExternalException.cs
- TextEditorCopyPaste.cs
- ColorTransform.cs
- DataContractAttribute.cs
- TypeHelper.cs
- GenericTypeParameterBuilder.cs
- PasswordRecoveryAutoFormat.cs
- WorkflowServiceHost.cs
- ToolboxSnapDragDropEventArgs.cs
- StatusBar.cs
- ScrollEventArgs.cs
- UnionExpr.cs
- XmlWriterSettings.cs
- EventToken.cs
- RepeatBehaviorConverter.cs
- EnvelopedSignatureTransform.cs
- MailAddressCollection.cs
- HtmlInputText.cs
- WebPartCancelEventArgs.cs
- EncodingTable.cs
- MetabaseReader.cs
- BezierSegment.cs
- ReferenceService.cs
- ErrorEventArgs.cs
- TableChangeProcessor.cs
- _FixedSizeReader.cs
- XmlText.cs
- XmlElementAttributes.cs
- mactripleDES.cs
- ReaderWriterLock.cs
- DurableRuntimeValidator.cs
- DataDesignUtil.cs
- ValueSerializer.cs
- StringTraceRecord.cs
- SamlAssertionKeyIdentifierClause.cs
- CacheHelper.cs
- SqlBulkCopyColumnMappingCollection.cs
- CompiledXpathExpr.cs
- PersonalizableAttribute.cs