Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / RightsManagementLicense.cs / 1 / RightsManagementLicense.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // RightsManagementLicense structs. // // History: // 06/13/05 - [....] created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.IO.Packaging; using System.Security.RightsManagement; using System.Security; namespace MS.Internal.Documents { ////// RightsManagementLicense is a structure that describes the RM grants given to a /// user. The data in this class is critical for set, so only Critical code can /// modify the rights granted or the user to whom they are granted. /// internal sealed class RightsManagementLicense { #region Constructors (none) //----------------------------------------------------- // Constructors (none) //----------------------------------------------------- #endregion Constructors (none) #region Internal Methods //------------------------------------------------------ // Internal Methods //----------------------------------------------------- ////// Adds a permission to the list of permissions saved in the license. /// /// The permission to add. ////// Critical /// 1) This is modifying the permissions granted to the holder of this /// license. /// [SecurityCritical] internal void AddPermission(RightsManagementPermissions permission) { _userRights.Value |= permission; } ////// Checks whether the license grants the given permission. /// /// The permission to check for ///Whether or not the license grants the permission internal bool HasPermission(RightsManagementPermissions permission) { return (((_userRights.Value & permission) == permission) && IsLicenseValid); } ////// Converts the permissions granted to a DRX Policy class. /// ///The permissions granted, converted to a policy internal RightsManagementPolicy ConvertToPolicy() { return (RightsManagementPolicy)(_userRights.Value); } #endregion Internal Methods #region Internal Properties //------------------------------------------------------ // Internal Properties //------------------------------------------------------ ////// Checks the validity dates of the license to see if the license is valid. /// ///Whether or not the license is still valid. internal bool IsLicenseValid { get { bool valid = true; // Check both whether the "valid from" date is in the future or the // "valid until" date is in the past. In either case the license is not // valid, and we must return false. if ((ValidFrom.CompareTo(DateTime.UtcNow) > 0) || (ValidUntil.CompareTo(DateTime.UtcNow) < 0)) { valid = false; } return valid; } } ////// Gets or sets the date from when the license is valid, in the UTC /// time zone /// ////// Critical /// 1) The setter is critical because it is setting a /// SecurityCriticalDataForSet field. /// internal DateTime ValidFrom { get { return _validFrom.Value; } [SecurityCritical] set { _validFrom.Value = value; } } ////// Gets or sets the date until when the license is valid, in the UTC /// time zone /// ////// Critical /// 1) The setter is critical because it is setting a /// SecurityCriticalDataForSet field. /// internal DateTime ValidUntil { get { return _validUntil.Value; } [SecurityCritical] set { _validUntil.Value = value; } } ////// Gets or sets the user for whom this license applies /// ////// Critical /// 1) The setter is critical because it is setting a /// SecurityCriticalDataForSet field. /// internal RightsManagementUser LicensedUser { get { return _user.Value; } [SecurityCritical] set { _user.Value = value; } } ////// Gets or sets the permissions granted by this license /// ////// Critical /// 1) The setter is critical because it is setting a /// SecurityCriticalDataForSet field. /// internal RightsManagementPermissions LicensePermissions { get { return _userRights.Value; } [SecurityCritical] set { _userRights.Value = value; } } ////// Gets or sets the name of the person to contact for more rights than /// are currently granted to the user. /// ////// Critical /// 1) This is personally identifiable information. /// internal string ReferralInfoName { [SecurityCritical] get { return _referralInfoName; } [SecurityCritical] set { _referralInfoName = value; } } ////// Gets or sets a URI to contact for more rights than are currently /// granted to the user. /// ////// Critical /// 1) This is personally identifiable information. /// internal Uri ReferralInfoUri { [SecurityCritical] get { return _referralInfoUri; } [SecurityCritical] set { _referralInfoUri = value; } } #endregion Internal Properties #region Private Fields //----------------------------------------------------- // Private Fields //------------------------------------------------------ private SecurityCriticalDataForSet_validFrom; private SecurityCriticalDataForSet _validUntil; private SecurityCriticalDataForSet _user; private SecurityCriticalDataForSet _userRights; /// /// The name of the person to contact for more rights. /// ////// Critical - personally identifiable information /// [SecurityCritical] private string _referralInfoName; ////// The URI to contact for more rights. /// ////// Critical - personally identifiable information /// [SecurityCritical] private Uri _referralInfoUri; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
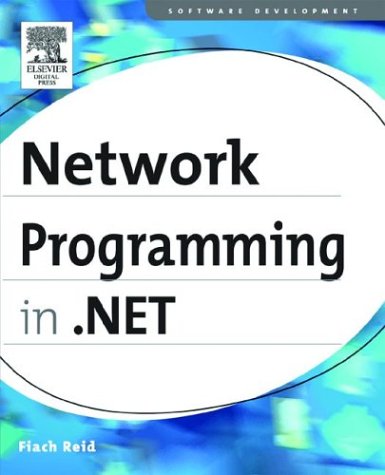
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmtpClient.cs
- UIEndRequest.cs
- SqlProviderManifest.cs
- LinkedList.cs
- CommentGlyph.cs
- TableTextElementCollectionInternal.cs
- PassportAuthenticationEventArgs.cs
- AggregatePushdown.cs
- RegistrySecurity.cs
- TextWriterEngine.cs
- Int32RectValueSerializer.cs
- XmlAttributeProperties.cs
- ToolStripLabel.cs
- XMLSchema.cs
- CodeDomComponentSerializationService.cs
- SinglePhaseEnlistment.cs
- DoubleLink.cs
- SiteMembershipCondition.cs
- ImageConverter.cs
- xml.cs
- Knowncolors.cs
- DataGridAutoFormat.cs
- XmlDomTextWriter.cs
- TcpStreams.cs
- CommonDialog.cs
- SymmetricAlgorithm.cs
- AdministrationHelpers.cs
- GcHandle.cs
- BufferedOutputStream.cs
- BinaryMessageEncoder.cs
- AlignmentXValidation.cs
- Dictionary.cs
- RegexWorker.cs
- ArrayMergeHelper.cs
- PolicyValidationException.cs
- AssemblyInfo.cs
- Cursors.cs
- ClosableStream.cs
- Command.cs
- Region.cs
- oledbmetadatacollectionnames.cs
- RunWorkerCompletedEventArgs.cs
- KeyGesture.cs
- IndentedTextWriter.cs
- DesignerVerb.cs
- Journal.cs
- ImageMap.cs
- TypeSystemProvider.cs
- ConfigurationSectionCollection.cs
- Compilation.cs
- StylusTip.cs
- UrlPath.cs
- PropertyInfoSet.cs
- DrawingContextDrawingContextWalker.cs
- IconHelper.cs
- CustomPopupPlacement.cs
- EntityConnection.cs
- DiscoveryViaBehavior.cs
- ExpandButtonVisibilityConverter.cs
- DataObjectEventArgs.cs
- RectAnimationUsingKeyFrames.cs
- lengthconverter.cs
- LeftCellWrapper.cs
- BitmapScalingModeValidation.cs
- ObjectSet.cs
- ClockController.cs
- VerificationException.cs
- PageCatalogPart.cs
- DataGrid.cs
- OdbcReferenceCollection.cs
- SharedPersonalizationStateInfo.cs
- VirtualizedContainerService.cs
- UnaryNode.cs
- ParseHttpDate.cs
- CodeVariableDeclarationStatement.cs
- DBDataPermission.cs
- WeakReference.cs
- ASCIIEncoding.cs
- ErrorRuntimeConfig.cs
- CommandDevice.cs
- SynchronizedMessageSource.cs
- GeometryConverter.cs
- ModuleConfigurationInfo.cs
- DesignerCommandSet.cs
- BitmapMetadata.cs
- IProducerConsumerCollection.cs
- HtmlInputText.cs
- SmiConnection.cs
- IndicCharClassifier.cs
- XamlTreeBuilder.cs
- PageParserFilter.cs
- DebuggerService.cs
- ResourceReferenceExpression.cs
- DataServiceHost.cs
- DbConnectionStringBuilder.cs
- VersionedStream.cs
- SerializationHelper.cs
- RegexRunnerFactory.cs
- Formatter.cs
- StylusPointProperty.cs