Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusPointProperty.cs / 1305600 / StylusPointProperty.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Globalization; using System.Windows.Media; using System.Collections.Generic; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// StylusPointProperty /// public class StylusPointProperty { ////// Instance data /// private Guid _id; private bool _isButton; ////// StylusPointProperty /// /// identifier /// isButton public StylusPointProperty(Guid identifier, bool isButton) { Initialize(identifier, isButton); } ////// StylusPointProperty /// /// ///Protected - used by the StylusPointPropertyInfo ctor protected StylusPointProperty(StylusPointProperty stylusPointProperty) { if (null == stylusPointProperty) { throw new ArgumentNullException("stylusPointProperty"); } Initialize(stylusPointProperty.Id, stylusPointProperty.IsButton); } ////// Common ctor helper /// /// identifier /// isButton private void Initialize(Guid identifier, bool isButton) { // // validate isButton for known guids // if (StylusPointPropertyIds.IsKnownButton(identifier)) { if (!isButton) { //error, this is a known button throw new ArgumentException(SR.Get(SRID.InvalidIsButtonForId), "isButton"); } } else { if (StylusPointPropertyIds.IsKnownId(identifier) && isButton) { //error, this is a known guid that is NOT a button throw new ArgumentException(SR.Get(SRID.InvalidIsButtonForId2), "isButton"); } } _id = identifier; _isButton = isButton; } ////// Id /// public Guid Id { get { return _id; } } ////// IsButton /// public bool IsButton { get { return _isButton; } } ////// Returns a human readable string representation /// public override string ToString() { return "{Id=" + StylusPointPropertyIds.GetStringRepresentation(_id) + ", IsButton=" + _isButton.ToString(CultureInfo.InvariantCulture) + "}"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
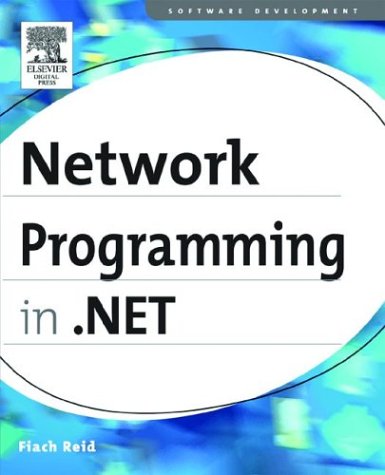
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SystemGatewayIPAddressInformation.cs
- InvalidFilterCriteriaException.cs
- DataGridTextBox.cs
- DeploymentExceptionMapper.cs
- DiscoveryMessageSequence11.cs
- FormClosingEvent.cs
- DebugView.cs
- DataStorage.cs
- SqlCharStream.cs
- TraceSection.cs
- SessionSwitchEventArgs.cs
- CalendarButton.cs
- WindowHideOrCloseTracker.cs
- NamespaceEmitter.cs
- DataBoundControlAdapter.cs
- TraceRecords.cs
- ObjectDataSourceMethodEventArgs.cs
- LinqDataSourceHelper.cs
- SourceSwitch.cs
- ValidatorCompatibilityHelper.cs
- ClientTargetCollection.cs
- SignatureDescription.cs
- AnimationClockResource.cs
- VerificationException.cs
- PaginationProgressEventArgs.cs
- AssemblyResourceLoader.cs
- HtmlTableRow.cs
- WebMessageFormatHelper.cs
- XmlSerializationWriter.cs
- RegistryConfigurationProvider.cs
- MsmqOutputChannel.cs
- RoutedEventHandlerInfo.cs
- ManagementScope.cs
- SoapMessage.cs
- Win32MouseDevice.cs
- ComponentChangingEvent.cs
- dataobject.cs
- HTMLTextWriter.cs
- HttpRuntimeSection.cs
- ClientBuildManagerCallback.cs
- InvalidEnumArgumentException.cs
- ArgIterator.cs
- indexingfiltermarshaler.cs
- MarginsConverter.cs
- DataGridColumnDropSeparator.cs
- InvalidComObjectException.cs
- MemoryStream.cs
- SocketPermission.cs
- RowSpanVector.cs
- IODescriptionAttribute.cs
- FontUnit.cs
- ResponseStream.cs
- Enum.cs
- ServiceModelSectionGroup.cs
- SymmetricAlgorithm.cs
- Substitution.cs
- GraphicsPath.cs
- TransformedBitmap.cs
- MsmqInputChannelBase.cs
- _LocalDataStore.cs
- QuaternionIndependentAnimationStorage.cs
- ToolboxBitmapAttribute.cs
- DataGrid.cs
- MimeObjectFactory.cs
- TypeForwardedToAttribute.cs
- ClientFormsAuthenticationCredentials.cs
- FragmentQuery.cs
- ExecutedRoutedEventArgs.cs
- NumericExpr.cs
- SiteMapPath.cs
- KerberosReceiverSecurityToken.cs
- ExceptionHelpers.cs
- ParameterModifier.cs
- KeyManager.cs
- ZoneMembershipCondition.cs
- CompositeKey.cs
- OrthographicCamera.cs
- TableLayout.cs
- ReflectionPermission.cs
- BaseDataList.cs
- TileBrush.cs
- InputReport.cs
- LinqExpressionNormalizer.cs
- DocumentGridContextMenu.cs
- ExtendedPropertyInfo.cs
- MenuBase.cs
- _NegoStream.cs
- connectionpool.cs
- DriveInfo.cs
- HatchBrush.cs
- FacetValueContainer.cs
- AccessorTable.cs
- XmlSchemaFacet.cs
- GroupJoinQueryOperator.cs
- WeakRefEnumerator.cs
- DecoderFallbackWithFailureFlag.cs
- TemplateEditingService.cs
- DesignTimeTemplateParser.cs
- ExpressionEvaluator.cs
- ScaleTransform3D.cs