Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / TemplateEditingService.cs / 1 / TemplateEditingService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Web.UI; using System.Web.UI.WebControls; ////// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] [Obsolete("Use of this type is not recommended because template editing is handled in ControlDesigner. To support template editing expose template data in the TemplateGroups property and call SetViewFlags(ViewFlags.TemplateEditing, true). http://go.microsoft.com/fwlink/?linkid=14202")] public sealed class TemplateEditingService : ITemplateEditingService, IDisposable { private IDesignerHost designerHost; /// public TemplateEditingService(IDesignerHost designerHost) { if (designerHost == null) { throw new ArgumentNullException("designerHost"); } this.designerHost = designerHost; } /// public bool SupportsNestedTemplateEditing { get { return false; } } /// public ITemplateEditingFrame CreateFrame(TemplatedControlDesigner designer, string frameName, string[] templateNames) { return CreateFrame(designer, frameName, templateNames, null, null); } /// public ITemplateEditingFrame CreateFrame(TemplatedControlDesigner designer, string frameName, string[] templateNames, Style controlStyle, Style[] templateStyles) { if (designer == null) { throw new ArgumentNullException("designer"); } if ((frameName == null) || (frameName.Length == 0)) { throw new ArgumentNullException("frameName"); } if ((templateNames == null) || (templateNames.Length == 0)) { throw new ArgumentException("templateNames"); } if ((templateStyles != null) && (templateStyles.Length != templateNames.Length)) { throw new ArgumentException("templateStyles"); } frameName = CreateFrameName(frameName); return new TemplateEditingFrame(designer, frameName, templateNames, controlStyle, templateStyles); } private string CreateFrameName(string frameName) { Debug.Assert((frameName != null) && (frameName.Length != 0)); // Strips out the ampersand typically used for menu mnemonics int index = frameName.IndexOf('&'); if (index < 0) { return frameName; } else if (index == 0) { return frameName.Substring(index + 1); } else { return frameName.Substring(0, index) + frameName.Substring(index + 1); } } /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } /// ~TemplateEditingService() { Dispose(false); } private void Dispose(bool disposing) { if (disposing) { designerHost = null; } } /// public string GetContainingTemplateName(Control control) { string containingTemplateName = String.Empty; HtmlControlDesigner designer = (HtmlControlDesigner)designerHost.GetDesigner(control); if (designer != null) { IHtmlControlDesignerBehavior behavior = designer.BehaviorInternal; NativeMethods.IHTMLElement htmlElement = (NativeMethods.IHTMLElement)behavior.DesignTimeElement; if (htmlElement != null) { object[] varTemplateName = new Object[1]; NativeMethods.IHTMLElement htmlelemParentNext; NativeMethods.IHTMLElement htmlelemParentCur = htmlElement.GetParentElement(); while (htmlelemParentCur != null) { htmlelemParentCur.GetAttribute("templatename", /*lFlags*/ 0, varTemplateName); if (varTemplateName[0] != null && varTemplateName[0].GetType() == typeof(string)) { containingTemplateName = varTemplateName[0].ToString(); break; } htmlelemParentNext = htmlelemParentCur.GetParentElement(); htmlelemParentCur = htmlelemParentNext; } } } return containingTemplateName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
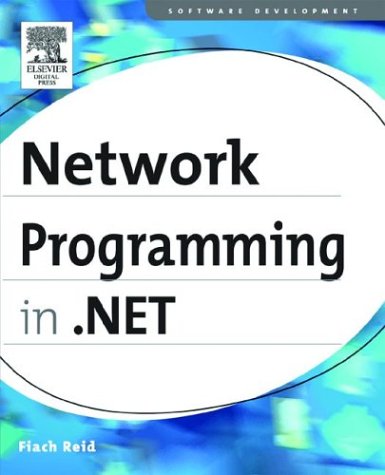
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MaterialGroup.cs
- DataGridCaption.cs
- Logging.cs
- XmlComplianceUtil.cs
- Utils.cs
- ComponentGlyph.cs
- StateWorkerRequest.cs
- BuildManagerHost.cs
- OleDbFactory.cs
- FunctionNode.cs
- ControlCollection.cs
- OutputScope.cs
- PointAnimationUsingKeyFrames.cs
- DiscoveryClientDocuments.cs
- Message.cs
- Quad.cs
- UnsafeNativeMethodsCLR.cs
- Perspective.cs
- KeyConstraint.cs
- UncommonField.cs
- VBIdentifierTrimConverter.cs
- ActivitiesCollection.cs
- MappingItemCollection.cs
- IdentifierService.cs
- XsltContext.cs
- DataGridLengthConverter.cs
- StringWriter.cs
- Rect.cs
- CodeSubDirectory.cs
- TypeSystemProvider.cs
- ToolBarButton.cs
- HttpModuleActionCollection.cs
- AttributeEmitter.cs
- Utils.cs
- PlainXmlWriter.cs
- UpdatePanelControlTrigger.cs
- ReadWriteSpinLock.cs
- Errors.cs
- PopOutPanel.cs
- ResourceManagerWrapper.cs
- TreeViewImageKeyConverter.cs
- StylusEventArgs.cs
- IdnElement.cs
- ProjectionCamera.cs
- EdmSchemaAttribute.cs
- KeyedHashAlgorithm.cs
- XmlSchemaParticle.cs
- WindowCollection.cs
- ConvertTextFrag.cs
- FontFamily.cs
- xmlfixedPageInfo.cs
- TableLayoutRowStyleCollection.cs
- RtType.cs
- SystemWebSectionGroup.cs
- UnsafeCollabNativeMethods.cs
- Font.cs
- TrustVersion.cs
- DataSourceControlBuilder.cs
- DataGridViewComboBoxCell.cs
- FilterableAttribute.cs
- Hash.cs
- ListViewInsertEventArgs.cs
- Subtree.cs
- ExitEventArgs.cs
- SubpageParaClient.cs
- InheritanceUI.cs
- TransformerConfigurationWizardBase.cs
- SocketAddress.cs
- ContextStack.cs
- InputDevice.cs
- ThousandthOfEmRealDoubles.cs
- ExpandButtonVisibilityConverter.cs
- WebColorConverter.cs
- ResourceReferenceExpressionConverter.cs
- WindowsListViewGroupSubsetLink.cs
- InternalMappingException.cs
- XmlSchemaElement.cs
- TextEvent.cs
- PropertyToken.cs
- SqlResolver.cs
- CultureNotFoundException.cs
- FamilyCollection.cs
- IsolatedStorage.cs
- ScrollItemPattern.cs
- SafeNativeMethods.cs
- ButtonPopupAdapter.cs
- UpdatePanel.cs
- XmlSchemaObject.cs
- HttpProcessUtility.cs
- TextUtf8RawTextWriter.cs
- UrlMappingsSection.cs
- ToolStripDropDownClosingEventArgs.cs
- DependencyPropertyKind.cs
- CompositionAdorner.cs
- XmlObjectSerializerReadContext.cs
- FtpWebResponse.cs
- CatalogZoneBase.cs
- DataGridViewCellValueEventArgs.cs
- ModelUIElement3D.cs
- RewritingValidator.cs