Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Advanced / FontFamily.cs / 2 / FontFamily.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * font family object (sdkinc\GDIplusFontFamily.h) */ namespace System.Drawing { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.ComponentModel; using Microsoft.Win32; using System.Globalization; using System.Drawing.Text; using System.Drawing; using System.Drawing.Internal; /** * Represent a FontFamily object */ ////// /// Abstracts a group of type faces having a /// similar basic design but having certain variation in styles. /// public sealed class FontFamily : MarshalByRefObject, IDisposable { private const int LANG_NEUTRAL = 0; private IntPtr nativeFamily; private bool createDefaultOnFail; #if DEBUG private static object lockObj = new object(); private static int idCount = 0; private int id; #endif ////// Sets the GDI+ native family. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2106:SecureAsserts")] private void SetNativeFamily( IntPtr family ) { Debug.Assert( this.nativeFamily == IntPtr.Zero, "Setting GDI+ native font family when already initialized." ); Debug.Assert( family != IntPtr.Zero, "Setting GDI+ native font family to null." ); this.nativeFamily = family; #if DEBUG lock(lockObj){ id = ++idCount; } #endif } ////// Internal constructor to initialize the native GDI+ font to an existing one. /// Used to create generic fonts and by FontCollection class. /// internal FontFamily(IntPtr family) { SetNativeFamily( family ); } ////// /// internal FontFamily(string name, bool createDefaultOnFail) { this.createDefaultOnFail = createDefaultOnFail; CreateFontFamily(name, null); } ////// Initializes a new instance of the ////// class with the specified name. /// /// The parameter determines how errors are /// handled when creating a font based on a font family that does not exist on the /// end user's system at run time. If this parameter is true, then a fall-back font /// will always be used instead. If this parameter is false, an exception will be thrown. /// /// /// public FontFamily(string name) { CreateFontFamily(name, null); } ////// Initializes a new instance of the ////// class with the specified name. /// /// /// Initializes a new instance of the public FontFamily(string name, FontCollection fontCollection) { CreateFontFamily(name, fontCollection); } ////// class in the specified and with the specified name. /// /// Creates the native font family object. /// Note: GDI+ creates singleton font family objects (from the corresponding font file) and reference count them. /// private void CreateFontFamily(string name, FontCollection fontCollection) { IntPtr fontfamily = IntPtr.Zero; IntPtr nativeFontCollection = (fontCollection == null) ? IntPtr.Zero : fontCollection.nativeFontCollection; int status = SafeNativeMethods.Gdip.GdipCreateFontFamilyFromName(name, new HandleRef(fontCollection, nativeFontCollection), out fontfamily); if (status != SafeNativeMethods.Gdip.Ok) { if (createDefaultOnFail) { fontfamily = GetGdipGenericSansSerif(); // This throws if failed. } else { // Special case this incredibly common error message to give more information if (status == SafeNativeMethods.Gdip.FontFamilyNotFound) { throw new ArgumentException(SR.GetString(SR.GdiplusFontFamilyNotFound, name)); } else if (status == SafeNativeMethods.Gdip.NotTrueTypeFont) { throw new ArgumentException(SR.GetString(SR.GdiplusNotTrueTypeFont, name)); } else { throw SafeNativeMethods.Gdip.StatusException(status); } } } SetNativeFamily( fontfamily ); } ////// /// Initializes a new instance of the public FontFamily(GenericFontFamilies genericFamily) { IntPtr fontfamily = IntPtr.Zero; int status; switch (genericFamily) { case GenericFontFamilies.Serif: { status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilySerif(out fontfamily); break; } case GenericFontFamilies.SansSerif: { status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilySansSerif(out fontfamily); break; } case GenericFontFamilies.Monospace: default: { status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilyMonospace(out fontfamily); break; } } if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeFamily( fontfamily ); } ////// class from the specified generic font family. /// /// /// ~FontFamily() { Dispose(false); } ////// Allows an object to free resources before the object is reclaimed by the /// Garbage Collector ( ///). /// /// The GDI+ native font family. It is shared by all FontFamily objects with same family name. /// internal IntPtr NativeFamily { get { //Debug.Assert( this.nativeFamily != IntPtr.Zero, "this.nativeFamily == IntPtr.Zero." ); return this.nativeFamily; } } // The managed wrappers do not expose a Clone method, as it's really nothing more // than AddRef (it doesn't copy the underlying GpFont), and in a garbage collected // world, that's not very useful. ////// /// public override bool Equals(object obj) { if (obj == this) return true; FontFamily ff = obj as FontFamily; if (ff == null) return false; // We can safely use the ptr to the native GDI+ FontFamily because it is common to // all objects of the same family (singleton RO object). return ff.NativeFamily == this.NativeFamily; } ///[To be supplied.] ////// /// Converts this public override string ToString() { return string.Format(CultureInfo.CurrentCulture, "[{0}: Name={1}]", GetType().Name, this.Name); } ///to a /// human-readable string. /// /// /// Gets a hash code for this public override int GetHashCode() { return this.GetName(LANG_NEUTRAL).GetHashCode(); } private static int CurrentLanguage { get { return System.Globalization.CultureInfo.CurrentUICulture.LCID; } } ///. /// /// /// Disposes of this public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } void Dispose(bool disposing) { if (this.nativeFamily != IntPtr.Zero) { try { #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeleteFontFamily(new HandleRef(this, this.nativeFamily)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch (Exception ex) { if (ClientUtils.IsCriticalException(ex)) { throw; } Debug.Fail("Exception thrown during Dispose: " + ex.ToString()); } finally { this.nativeFamily = IntPtr.Zero; } } } ///. /// /// /// Gets the name of this public String Name { get { return GetName(CurrentLanguage); } } ///. /// /// /// public String GetName(int language) { // LF_FACESIZE is 32 StringBuilder name = new StringBuilder(32); int status = SafeNativeMethods.Gdip.GdipGetFamilyName(new HandleRef(this, this.NativeFamily), name, language); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return name.ToString(); } ////// Retuns the name of this ///in /// the specified language. /// /// /// Returns an array that contains all of the /// public static FontFamily[] Families { get { IntPtr screenDC = UnsafeNativeMethods.GetDC(NativeMethods.NullHandleRef); FontFamily[] result = null; try { Graphics graphics = Graphics.FromHdcInternal(screenDC); try { result = GetFamilies(graphics); } finally { graphics.Dispose(); } } finally { UnsafeNativeMethods.ReleaseDC(NativeMethods.NullHandleRef, new HandleRef(null, screenDC)); } return result; } } ///objects associated with the current graphics /// context. /// /// /// public static FontFamily GenericSansSerif { get { return new FontFamily(GetGdipGenericSansSerif()); } } private static IntPtr GetGdipGenericSansSerif() { IntPtr fontfamily = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilySansSerif(out fontfamily); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return fontfamily; } ////// Gets a generic SansSerif ///. /// /// /// Gets a generic Serif public static FontFamily GenericSerif { get { return new FontFamily(GetNativeGenericSerif()); } } private static IntPtr GetNativeGenericSerif() { IntPtr fontfamily = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilySerif(out fontfamily); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return fontfamily; } ///. /// /// /// Gets a generic monospace public static FontFamily GenericMonospace { get { return new FontFamily(GetNativeGenericMonospace()); } } private static IntPtr GetNativeGenericMonospace() { IntPtr fontfamily = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilyMonospace(out fontfamily); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return fontfamily; } // No longer support in FontFamily // Obsolete API and need to be removed later // ///. /// /// /// public static FontFamily[] GetFamilies(Graphics graphics) { if (graphics == null) throw new ArgumentNullException("graphics"); return new InstalledFontCollection().Families; } ////// Returns an array that contains all of the ///objects associated with /// the specified graphics context. /// /// /// Indicates whether the specified public bool IsStyleAvailable(FontStyle style) { int bresult; int status = SafeNativeMethods.Gdip.GdipIsStyleAvailable(new HandleRef(this, this.NativeFamily), style, out bresult); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return bresult != 0; } ///is /// available. /// /// /// Gets the size of the Em square for the /// specified style in font design units. /// public int GetEmHeight(FontStyle style) { int result = 0; int status = SafeNativeMethods.Gdip.GdipGetEmHeight(new HandleRef(this, this.NativeFamily), style, out result); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return result; } ////// /// public int GetCellAscent(FontStyle style) { int result = 0; int status = SafeNativeMethods.Gdip.GdipGetCellAscent(new HandleRef(this, this.NativeFamily), style, out result); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return result; } ////// Returns the ascender metric for Windows. /// ////// /// public int GetCellDescent(FontStyle style) { int result = 0; int status = SafeNativeMethods.Gdip.GdipGetCellDescent(new HandleRef(this, this.NativeFamily), style, out result); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return result; } ////// Returns the descender metric for Windows. /// ////// /// Returns the distance between two /// consecutive lines of text for this public int GetLineSpacing(FontStyle style) { int result = 0; int status = SafeNativeMethods.Gdip.GdipGetLineSpacing(new HandleRef(this, this.NativeFamily), style, out result); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //with the specified . /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * font family object (sdkinc\GDIplusFontFamily.h) */ namespace System.Drawing { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.ComponentModel; using Microsoft.Win32; using System.Globalization; using System.Drawing.Text; using System.Drawing; using System.Drawing.Internal; /** * Represent a FontFamily object */ ////// /// Abstracts a group of type faces having a /// similar basic design but having certain variation in styles. /// public sealed class FontFamily : MarshalByRefObject, IDisposable { private const int LANG_NEUTRAL = 0; private IntPtr nativeFamily; private bool createDefaultOnFail; #if DEBUG private static object lockObj = new object(); private static int idCount = 0; private int id; #endif ////// Sets the GDI+ native family. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2106:SecureAsserts")] private void SetNativeFamily( IntPtr family ) { Debug.Assert( this.nativeFamily == IntPtr.Zero, "Setting GDI+ native font family when already initialized." ); Debug.Assert( family != IntPtr.Zero, "Setting GDI+ native font family to null." ); this.nativeFamily = family; #if DEBUG lock(lockObj){ id = ++idCount; } #endif } ////// Internal constructor to initialize the native GDI+ font to an existing one. /// Used to create generic fonts and by FontCollection class. /// internal FontFamily(IntPtr family) { SetNativeFamily( family ); } ////// /// internal FontFamily(string name, bool createDefaultOnFail) { this.createDefaultOnFail = createDefaultOnFail; CreateFontFamily(name, null); } ////// Initializes a new instance of the ////// class with the specified name. /// /// The parameter determines how errors are /// handled when creating a font based on a font family that does not exist on the /// end user's system at run time. If this parameter is true, then a fall-back font /// will always be used instead. If this parameter is false, an exception will be thrown. /// /// /// public FontFamily(string name) { CreateFontFamily(name, null); } ////// Initializes a new instance of the ////// class with the specified name. /// /// /// Initializes a new instance of the public FontFamily(string name, FontCollection fontCollection) { CreateFontFamily(name, fontCollection); } ////// class in the specified and with the specified name. /// /// Creates the native font family object. /// Note: GDI+ creates singleton font family objects (from the corresponding font file) and reference count them. /// private void CreateFontFamily(string name, FontCollection fontCollection) { IntPtr fontfamily = IntPtr.Zero; IntPtr nativeFontCollection = (fontCollection == null) ? IntPtr.Zero : fontCollection.nativeFontCollection; int status = SafeNativeMethods.Gdip.GdipCreateFontFamilyFromName(name, new HandleRef(fontCollection, nativeFontCollection), out fontfamily); if (status != SafeNativeMethods.Gdip.Ok) { if (createDefaultOnFail) { fontfamily = GetGdipGenericSansSerif(); // This throws if failed. } else { // Special case this incredibly common error message to give more information if (status == SafeNativeMethods.Gdip.FontFamilyNotFound) { throw new ArgumentException(SR.GetString(SR.GdiplusFontFamilyNotFound, name)); } else if (status == SafeNativeMethods.Gdip.NotTrueTypeFont) { throw new ArgumentException(SR.GetString(SR.GdiplusNotTrueTypeFont, name)); } else { throw SafeNativeMethods.Gdip.StatusException(status); } } } SetNativeFamily( fontfamily ); } ////// /// Initializes a new instance of the public FontFamily(GenericFontFamilies genericFamily) { IntPtr fontfamily = IntPtr.Zero; int status; switch (genericFamily) { case GenericFontFamilies.Serif: { status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilySerif(out fontfamily); break; } case GenericFontFamilies.SansSerif: { status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilySansSerif(out fontfamily); break; } case GenericFontFamilies.Monospace: default: { status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilyMonospace(out fontfamily); break; } } if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeFamily( fontfamily ); } ////// class from the specified generic font family. /// /// /// ~FontFamily() { Dispose(false); } ////// Allows an object to free resources before the object is reclaimed by the /// Garbage Collector ( ///). /// /// The GDI+ native font family. It is shared by all FontFamily objects with same family name. /// internal IntPtr NativeFamily { get { //Debug.Assert( this.nativeFamily != IntPtr.Zero, "this.nativeFamily == IntPtr.Zero." ); return this.nativeFamily; } } // The managed wrappers do not expose a Clone method, as it's really nothing more // than AddRef (it doesn't copy the underlying GpFont), and in a garbage collected // world, that's not very useful. ////// /// public override bool Equals(object obj) { if (obj == this) return true; FontFamily ff = obj as FontFamily; if (ff == null) return false; // We can safely use the ptr to the native GDI+ FontFamily because it is common to // all objects of the same family (singleton RO object). return ff.NativeFamily == this.NativeFamily; } ///[To be supplied.] ////// /// Converts this public override string ToString() { return string.Format(CultureInfo.CurrentCulture, "[{0}: Name={1}]", GetType().Name, this.Name); } ///to a /// human-readable string. /// /// /// Gets a hash code for this public override int GetHashCode() { return this.GetName(LANG_NEUTRAL).GetHashCode(); } private static int CurrentLanguage { get { return System.Globalization.CultureInfo.CurrentUICulture.LCID; } } ///. /// /// /// Disposes of this public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } void Dispose(bool disposing) { if (this.nativeFamily != IntPtr.Zero) { try { #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeleteFontFamily(new HandleRef(this, this.nativeFamily)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch (Exception ex) { if (ClientUtils.IsCriticalException(ex)) { throw; } Debug.Fail("Exception thrown during Dispose: " + ex.ToString()); } finally { this.nativeFamily = IntPtr.Zero; } } } ///. /// /// /// Gets the name of this public String Name { get { return GetName(CurrentLanguage); } } ///. /// /// /// public String GetName(int language) { // LF_FACESIZE is 32 StringBuilder name = new StringBuilder(32); int status = SafeNativeMethods.Gdip.GdipGetFamilyName(new HandleRef(this, this.NativeFamily), name, language); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return name.ToString(); } ////// Retuns the name of this ///in /// the specified language. /// /// /// Returns an array that contains all of the /// public static FontFamily[] Families { get { IntPtr screenDC = UnsafeNativeMethods.GetDC(NativeMethods.NullHandleRef); FontFamily[] result = null; try { Graphics graphics = Graphics.FromHdcInternal(screenDC); try { result = GetFamilies(graphics); } finally { graphics.Dispose(); } } finally { UnsafeNativeMethods.ReleaseDC(NativeMethods.NullHandleRef, new HandleRef(null, screenDC)); } return result; } } ///objects associated with the current graphics /// context. /// /// /// public static FontFamily GenericSansSerif { get { return new FontFamily(GetGdipGenericSansSerif()); } } private static IntPtr GetGdipGenericSansSerif() { IntPtr fontfamily = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilySansSerif(out fontfamily); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return fontfamily; } ////// Gets a generic SansSerif ///. /// /// /// Gets a generic Serif public static FontFamily GenericSerif { get { return new FontFamily(GetNativeGenericSerif()); } } private static IntPtr GetNativeGenericSerif() { IntPtr fontfamily = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilySerif(out fontfamily); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return fontfamily; } ///. /// /// /// Gets a generic monospace public static FontFamily GenericMonospace { get { return new FontFamily(GetNativeGenericMonospace()); } } private static IntPtr GetNativeGenericMonospace() { IntPtr fontfamily = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipGetGenericFontFamilyMonospace(out fontfamily); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return fontfamily; } // No longer support in FontFamily // Obsolete API and need to be removed later // ///. /// /// /// public static FontFamily[] GetFamilies(Graphics graphics) { if (graphics == null) throw new ArgumentNullException("graphics"); return new InstalledFontCollection().Families; } ////// Returns an array that contains all of the ///objects associated with /// the specified graphics context. /// /// /// Indicates whether the specified public bool IsStyleAvailable(FontStyle style) { int bresult; int status = SafeNativeMethods.Gdip.GdipIsStyleAvailable(new HandleRef(this, this.NativeFamily), style, out bresult); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return bresult != 0; } ///is /// available. /// /// /// Gets the size of the Em square for the /// specified style in font design units. /// public int GetEmHeight(FontStyle style) { int result = 0; int status = SafeNativeMethods.Gdip.GdipGetEmHeight(new HandleRef(this, this.NativeFamily), style, out result); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return result; } ////// /// public int GetCellAscent(FontStyle style) { int result = 0; int status = SafeNativeMethods.Gdip.GdipGetCellAscent(new HandleRef(this, this.NativeFamily), style, out result); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return result; } ////// Returns the ascender metric for Windows. /// ////// /// public int GetCellDescent(FontStyle style) { int result = 0; int status = SafeNativeMethods.Gdip.GdipGetCellDescent(new HandleRef(this, this.NativeFamily), style, out result); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return result; } ////// Returns the descender metric for Windows. /// ////// /// Returns the distance between two /// consecutive lines of text for this public int GetLineSpacing(FontStyle style) { int result = 0; int status = SafeNativeMethods.Gdip.GdipGetLineSpacing(new HandleRef(this, this.NativeFamily), style, out result); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.with the specified . ///
Link Menu
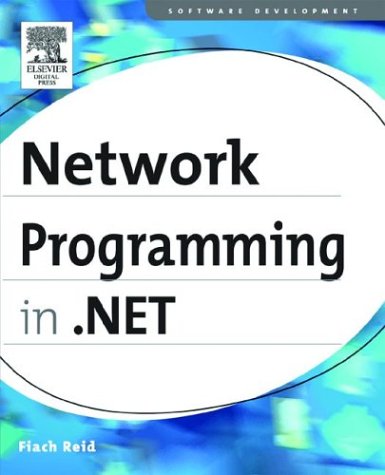
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListView.cs
- SetStateDesigner.cs
- CustomAttributeBuilder.cs
- HyperLinkStyle.cs
- FormClosingEvent.cs
- ApplicationManager.cs
- SqlLiftIndependentRowExpressions.cs
- DbProviderFactory.cs
- Clipboard.cs
- SqlColumnizer.cs
- TextBoxAutoCompleteSourceConverter.cs
- BlockCollection.cs
- ResourceWriter.cs
- Binding.cs
- ControlBuilder.cs
- FigureHelper.cs
- ZipIOCentralDirectoryFileHeader.cs
- XPathExpr.cs
- EdmRelationshipRoleAttribute.cs
- Popup.cs
- XmlTextAttribute.cs
- InternalPermissions.cs
- ComboBox.cs
- WindowsListViewItemCheckBox.cs
- RSAPKCS1KeyExchangeFormatter.cs
- DragEvent.cs
- ListenerAdapter.cs
- ExportOptions.cs
- DecimalKeyFrameCollection.cs
- PersonalizationStateQuery.cs
- ListViewSortEventArgs.cs
- ViewStateException.cs
- XmlSchemas.cs
- Argument.cs
- TreeNodeEventArgs.cs
- SiteMapPathDesigner.cs
- ProcessModuleDesigner.cs
- EventListenerClientSide.cs
- ToolStripContentPanel.cs
- CollectionViewGroupInternal.cs
- ComplexBindingPropertiesAttribute.cs
- MetadataProperty.cs
- ResourceDisplayNameAttribute.cs
- InvariantComparer.cs
- ScriptReference.cs
- EntityDataSourceWrapper.cs
- ExpressionPrefixAttribute.cs
- SqlFunctionAttribute.cs
- MiniLockedBorderGlyph.cs
- CommandArguments.cs
- SqlRowUpdatingEvent.cs
- Switch.cs
- ListViewItemSelectionChangedEvent.cs
- ScriptModule.cs
- ObjectView.cs
- TextBoxBaseDesigner.cs
- DataGridViewBand.cs
- WorkflowDesigner.cs
- TaskFileService.cs
- FileResponseElement.cs
- WizardPanelChangingEventArgs.cs
- NotFiniteNumberException.cs
- UnmanagedBitmapWrapper.cs
- TreeBuilderXamlTranslator.cs
- CheckableControlBaseAdapter.cs
- IteratorDescriptor.cs
- CharacterBuffer.cs
- PageVisual.cs
- DomainUpDown.cs
- StringResourceManager.cs
- XmlTextReaderImplHelpers.cs
- ISAPIApplicationHost.cs
- Int64KeyFrameCollection.cs
- XappLauncher.cs
- ConnectionManagementElementCollection.cs
- AnimatedTypeHelpers.cs
- _CommandStream.cs
- SmtpFailedRecipientException.cs
- UnsafeNativeMethods.cs
- ConfigXmlDocument.cs
- RSAOAEPKeyExchangeFormatter.cs
- IPipelineRuntime.cs
- WebPartConnectionsDisconnectVerb.cs
- SimpleModelProvider.cs
- WebPartExportVerb.cs
- DetailsViewUpdateEventArgs.cs
- XmlDictionaryReaderQuotasElement.cs
- Span.cs
- HeaderLabel.cs
- DefaultEventAttribute.cs
- PropertyEntry.cs
- TextSelection.cs
- ComNativeDescriptor.cs
- IdnElement.cs
- InvalidPrinterException.cs
- EntityDataSourceEntitySetNameItem.cs
- Normalizer.cs
- StringValidator.cs
- RegexGroup.cs
- PasswordRecoveryAutoFormat.cs