Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / CommandArguments.cs / 3 / CommandArguments.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.Tools.ServiceModel { using System; using System.DirectoryServices; using System.Globalization; using System.ServiceModel.Install; internal class CommandArguments { bool applyToConfig; bool applyToScriptMaps; bool displayHelp; bool displayLogo; InstallAction installAction; bool installServices; OutputLevel outputLevel; bool recursive; bool requireConfirmation; bool runInstallScript; string scriptMapPath; bool updateScriptMaps; internal CommandArguments(string[] args) { bool installScriptMaps = false; bool removeScriptMaps = false; this.updateScriptMaps = false; this.recursive = false; this.scriptMapPath = null; this.displayHelp = false; this.displayLogo = true; this.requireConfirmation = true; this.outputLevel = OutputLevel.Normal; this.installAction = InstallAction.None; this.applyToConfig = false; this.applyToScriptMaps = false; this.runInstallScript = false; this.installServices = true; if (0 == args.Length) { this.displayHelp = true; return; } foreach (string arg in args) { if (arg.StartsWith("/", StringComparison.Ordinal) || arg.StartsWith("-", StringComparison.Ordinal)) { string key = arg.Substring(1); string value = null; int delim = key.IndexOf(':'); if (-1 != delim) { value = key.Substring(delim + 1); key = key.Substring(0, delim); } switch (key.ToLower(CultureInfo.CurrentCulture)) { case ("i"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Install; this.updateScriptMaps = false; this.applyToConfig = true; // Apply to script maps if not on an IIS7. this.applyToScriptMaps = !IisHelper.ShouldInstallWas; break; case ("ir"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Install; this.updateScriptMaps = false; this.applyToConfig = true; this.applyToScriptMaps = false; break; case ("iru"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Install; this.updateScriptMaps = false; this.applyToConfig = true; // Apply to script maps if not on an IIS7. this.applyToScriptMaps = !IisHelper.ShouldInstallWas; break; case ("u"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Uninstall; this.updateScriptMaps = true; this.recursive = true; this.applyToConfig = true; // Apply to script maps if not on an IIS7. this.applyToScriptMaps = !IisHelper.ShouldInstallWas; break; case ("ua"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Uninstall; this.updateScriptMaps = false; this.recursive = true; this.applyToConfig = true; // Apply to script maps if not on an IIS7. this.applyToScriptMaps = !IisHelper.ShouldInstallWas; break; case ("r"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Reinstall; this.updateScriptMaps = true; this.applyToConfig = true; // Apply to script maps if not on an IIS7. this.applyToScriptMaps = !IisHelper.ShouldInstallWas; break; case ("s"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Install; installScriptMaps = true; this.recursive = true; this.scriptMapPath = value; this.applyToConfig = false; this.applyToScriptMaps = true; break; case ("sn"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Install; installScriptMaps = true; this.recursive = false; this.scriptMapPath = value; this.applyToConfig = false; this.applyToScriptMaps = true; break; case ("k"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Uninstall; removeScriptMaps = true; this.recursive = true; this.scriptMapPath = value; this.applyToConfig = false; this.applyToScriptMaps = true; break; case ("kn"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.Uninstall; removeScriptMaps = true; this.recursive = false; this.scriptMapPath = value; this.applyToConfig = false; this.applyToScriptMaps = true; break; case ("lv"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.ListVersions; this.applyToConfig = true; // Apply to script maps if not on an IIS7. this.applyToScriptMaps = !IisHelper.ShouldInstallWas; this.recursive = true; break; case ("lk"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.ListScriptMaps; this.applyToConfig = false; this.applyToScriptMaps = true; this.recursive = true; break; case ("vi"): if (InstallAction.None != this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOptionError)); } this.installAction = InstallAction.VerifyInstall; this.applyToConfig = true; // Apply to script maps if not on an IIS7. this.applyToScriptMaps = !IisHelper.ShouldInstallWas; break; case("x"): this.runInstallScript = true; break; case ("q"): if (OutputLevel.Normal != this.outputLevel && OutputLevel.Quiet != this.outputLevel) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOutputError)); } this.outputLevel = OutputLevel.Quiet; break; case ("v"): if (OutputLevel.Normal != this.outputLevel && OutputLevel.Verbose != this.outputLevel) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegExclusiveOutputError)); } this.outputLevel = OutputLevel.Verbose; break; case ("y"): this.requireConfirmation = false; break; case ("nologo"): this.displayLogo = false; break; case ("noservices"): this.installServices = false; break; case ("?"): case ("h"): case ("help"): this.displayHelp = true; return; default: throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegUnknownOptionError, key)); } } else { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegUnknownOptionError, arg)); } } if (InstallAction.None == this.installAction) { throw new ApplicationException(SR2.GetString(SR2.ServiceModelRegNoOptionsError)); } if (installScriptMaps || removeScriptMaps) { // If IIS is not installed, let error bubble through. IIS installation will be checked // and handled properly at a later time. if (IisHelper.ShouldInstallIis || IisHelper.ShouldInstallWas) { if (null == this.scriptMapPath) { throw new NullReferenceException(SR2.GetString(SR2.ServiceModelRegNullScriptMapPath)); } } } } internal bool ApplyToConfig { get {return applyToConfig; } } internal bool ApplyToScriptMaps { get {return applyToScriptMaps; } } internal bool DisplayHelp { get {return this.displayHelp; } } internal bool DisplayLogo { get {return this.displayLogo; } } internal InstallAction InstallAction { get {return this.installAction; } } internal bool InstallServices { get {return this.installServices; } } internal OutputLevel OutputLevel { get {return this.outputLevel; } } internal bool Recursive { get {return this.recursive; } } internal bool RequireConfirmation { get {return this.requireConfirmation; } } internal bool RunInstallScript { get { return this.runInstallScript; } } internal string ScriptMapPath { get {return this.scriptMapPath; } } internal bool UpdateScriptMaps { get {return this.updateScriptMaps; } } internal static void DisplayUsage() { Console.WriteLine(SR2.GetString(SR2.HelpDescription)); // ThisAssembly conflicts with one imported from System.ServiceModel.Install.dll (friend assembly) // build uses the correct one (local)... #pragma warning disable 436 Console.WriteLine(SR2.GetString(SR2.HelpUsage, ThisAssembly.Title)); #pragma warning restore 436 Console.WriteLine(SR2.GetString(SR2.HelpInstall, "-i")); Console.WriteLine(SR2.GetString(SR2.HelpInstallRegister, "-ir")); Console.WriteLine(SR2.GetString(SR2.HelpInstallNoUpdate, "-iru")); Console.WriteLine(SR2.GetString(SR2.HelpUninstall, "-u")); Console.WriteLine(SR2.GetString(SR2.HelpUninstallAll, "-ua")); Console.WriteLine(SR2.GetString(SR2.HelpReinstall, "-r")); Console.WriteLine(SR2.GetString(SR2.HelpInstallRunWCFInstaller, "-x")); Console.WriteLine(SR2.GetString(SR2.HelpInstallScriptMapsRecursively, "-s")); Console.WriteLine(SR2.GetString(SR2.HelpInstallScriptMapsNonRecursively, "-sn")); Console.WriteLine(SR2.GetString(SR2.HelpRemoveScriptMapsRecursively, "-k")); Console.WriteLine(SR2.GetString(SR2.HelpRemoveScriptMapsNonRecursively, "-kn")); Console.WriteLine(SR2.GetString(SR2.HelpListVersions, "-lv")); Console.WriteLine(SR2.GetString(SR2.HelpListScriptMaps, "-lk")); Console.WriteLine(SR2.GetString(SR2.HelpVerify, "-vi")); Console.WriteLine(SR2.GetString(SR2.HelpConfirmation, "-y")); Console.WriteLine(SR2.GetString(SR2.HelpQuiet, "-q")); Console.WriteLine(SR2.GetString(SR2.HelpVerbose, "-v")); Console.WriteLine(SR2.GetString(SR2.HelpNoLogo, "-nologo")); Console.WriteLine(SR2.GetString(SR2.HelpDisplayHelp, "-?")); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
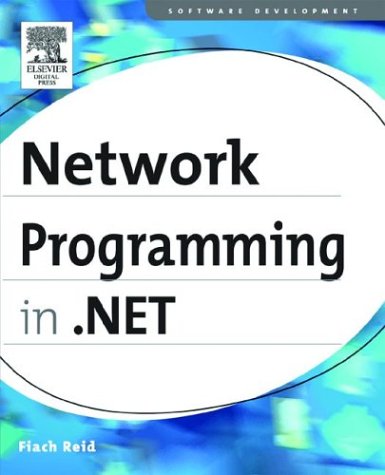
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ZoneButton.cs
- SubqueryRules.cs
- XamlReaderHelper.cs
- SecurityContext.cs
- DocumentXmlWriter.cs
- BoolExpr.cs
- XmlDownloadManager.cs
- RawTextInputReport.cs
- RetrieveVirtualItemEventArgs.cs
- ChannelBuilder.cs
- LinkConverter.cs
- StyleTypedPropertyAttribute.cs
- APCustomTypeDescriptor.cs
- TableColumn.cs
- OleDbError.cs
- WebPartMinimizeVerb.cs
- ImageListStreamer.cs
- DataGridViewCellCollection.cs
- AdornerHitTestResult.cs
- WebEvents.cs
- NativeMethods.cs
- Subordinate.cs
- AsymmetricKeyExchangeDeformatter.cs
- DesignerActionGlyph.cs
- keycontainerpermission.cs
- DataGridViewHeaderCell.cs
- GCHandleCookieTable.cs
- NetworkAddressChange.cs
- CommonXSendMessage.cs
- WindowsListViewGroupHelper.cs
- ContentElement.cs
- SizeAnimation.cs
- DataSourceHelper.cs
- RectangleHotSpot.cs
- BezierSegment.cs
- MsmqActivation.cs
- FacetValueContainer.cs
- ExpressionBuilderCollection.cs
- RectAnimation.cs
- FtpRequestCacheValidator.cs
- ColorConvertedBitmapExtension.cs
- Event.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- AsyncOperation.cs
- LayoutSettings.cs
- LabelAutomationPeer.cs
- GeometryModel3D.cs
- NamespaceQuery.cs
- XmlSchemaSet.cs
- SqlConnectionStringBuilder.cs
- Matrix.cs
- PatternMatcher.cs
- PtsHost.cs
- PrintPreviewControl.cs
- ConfigurationLoader.cs
- _ListenerRequestStream.cs
- COM2ExtendedTypeConverter.cs
- SQLMoney.cs
- WizardStepBase.cs
- DataServiceHost.cs
- ping.cs
- TextEffect.cs
- PartialTrustVisibleAssembliesSection.cs
- DataRowView.cs
- SevenBitStream.cs
- RecommendedAsConfigurableAttribute.cs
- RightsManagementInformation.cs
- ProtocolProfile.cs
- SelectedDatesCollection.cs
- EventProviderBase.cs
- DataBinding.cs
- TrackingValidationObjectDictionary.cs
- FlowPosition.cs
- ShaderRenderModeValidation.cs
- CodeFieldReferenceExpression.cs
- PersonalizableTypeEntry.cs
- DrawingGroupDrawingContext.cs
- SByte.cs
- EnvironmentPermission.cs
- BasicExpressionVisitor.cs
- SqlNode.cs
- ConfigurationSchemaErrors.cs
- Knowncolors.cs
- MembershipPasswordException.cs
- BuildManager.cs
- CharUnicodeInfo.cs
- XmlTextReader.cs
- DelegateSerializationHolder.cs
- SecurityUtils.cs
- SmtpMail.cs
- MediaPlayer.cs
- PropertyGroupDescription.cs
- TemplateColumn.cs
- DropShadowBitmapEffect.cs
- EditorZoneBase.cs
- XmlConvert.cs
- DataServiceEntityAttribute.cs
- HostingEnvironmentException.cs
- NetworkCredential.cs
- ConnectionStringSettings.cs