Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / TableColumn.cs / 1 / TableColumn.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table column object implementation. // // History: // 06/19/2003 : olego - Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows.Documents { ////// Table column. /// public class TableColumn : FrameworkContentElement { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of a Column /// public TableColumn() { _parentIndex = -1; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Width property. /// public GridLength Width { get { return (GridLength) GetValue(WidthProperty); } set { SetValue(WidthProperty, value); } } ////// Background property. /// public Brush Background { get { return (Brush) GetValue(BackgroundProperty); } set { SetValue(BackgroundProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion Protected Methods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Callback used to notify the Cell about entering model tree. /// internal void OnEnterParentTree() { Table.InvalidateColumns(); } ////// Callback used to notify the Cell about exitting model tree. /// internal void OnExitParentTree() { Table.InvalidateColumns(); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Table owner accessor /// internal Table Table { get { return Parent as Table; } } ////// Column's index in the parents collection. /// internal int Index { get { return (_parentIndex); } set { Debug.Assert (value >= -1 && _parentIndex != value); _parentIndex = value; } } ////// DefaultWidth /// internal static GridLength DefaultWidth { get { return (new GridLength(0, GridUnitType.Auto)); } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// private static bool IsValidWidth(object value) { GridLength gridLength = (GridLength) value; if ((gridLength.GridUnitType == GridUnitType.Pixel || gridLength.GridUnitType == GridUnitType.Star) && (gridLength.Value < 0.0)) { return false; } double maxPixel = Math.Min(1000000, PTS.MaxPageSize); if (gridLength.GridUnitType == GridUnitType.Pixel && (gridLength.Value > maxPixel)) { return false; } return true; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private int _parentIndex; // column's index in parent's children collection #endregion Private Fields //------------------------------------------------------ // // Properties // //----------------------------------------------------- #region Properties ////// /// Width property. /// public static readonly DependencyProperty WidthProperty = DependencyProperty.Register( "Width", typeof(GridLength), typeof(TableColumn), new FrameworkPropertyMetadata( new GridLength(0, GridUnitType.Auto), FrameworkPropertyMetadataOptions.AffectsMeasure, new PropertyChangedCallback(OnWidthChanged)), new ValidateValueCallback(IsValidWidth)); ////// DependencyProperty for public static readonly DependencyProperty BackgroundProperty = Panel.BackgroundProperty.AddOwner( typeof(TableColumn), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsRender, new PropertyChangedCallback(OnBackgroundChanged))); #endregion Properties //----------------------------------------------------- // // Static Initialization // //----------------------------------------------------- #region Static Initialization ///property. /// /// Called when the value of the WidthProperty changes /// private static void OnWidthChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Table table = ((TableColumn) d).Table; if(table != null) { table.InvalidateColumns(); } } ////// Called when the value of the BackgroundProperty changes /// private static void OnBackgroundChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Table table = ((TableColumn) d).Table; if(table != null) { table.InvalidateColumns(); } } #endregion Static Initialization } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table column object implementation. // // History: // 06/19/2003 : olego - Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows.Documents { ////// Table column. /// public class TableColumn : FrameworkContentElement { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of a Column /// public TableColumn() { _parentIndex = -1; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Width property. /// public GridLength Width { get { return (GridLength) GetValue(WidthProperty); } set { SetValue(WidthProperty, value); } } ////// Background property. /// public Brush Background { get { return (Brush) GetValue(BackgroundProperty); } set { SetValue(BackgroundProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion Protected Methods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Callback used to notify the Cell about entering model tree. /// internal void OnEnterParentTree() { Table.InvalidateColumns(); } ////// Callback used to notify the Cell about exitting model tree. /// internal void OnExitParentTree() { Table.InvalidateColumns(); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Table owner accessor /// internal Table Table { get { return Parent as Table; } } ////// Column's index in the parents collection. /// internal int Index { get { return (_parentIndex); } set { Debug.Assert (value >= -1 && _parentIndex != value); _parentIndex = value; } } ////// DefaultWidth /// internal static GridLength DefaultWidth { get { return (new GridLength(0, GridUnitType.Auto)); } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// private static bool IsValidWidth(object value) { GridLength gridLength = (GridLength) value; if ((gridLength.GridUnitType == GridUnitType.Pixel || gridLength.GridUnitType == GridUnitType.Star) && (gridLength.Value < 0.0)) { return false; } double maxPixel = Math.Min(1000000, PTS.MaxPageSize); if (gridLength.GridUnitType == GridUnitType.Pixel && (gridLength.Value > maxPixel)) { return false; } return true; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private int _parentIndex; // column's index in parent's children collection #endregion Private Fields //------------------------------------------------------ // // Properties // //----------------------------------------------------- #region Properties ////// /// Width property. /// public static readonly DependencyProperty WidthProperty = DependencyProperty.Register( "Width", typeof(GridLength), typeof(TableColumn), new FrameworkPropertyMetadata( new GridLength(0, GridUnitType.Auto), FrameworkPropertyMetadataOptions.AffectsMeasure, new PropertyChangedCallback(OnWidthChanged)), new ValidateValueCallback(IsValidWidth)); ////// DependencyProperty for public static readonly DependencyProperty BackgroundProperty = Panel.BackgroundProperty.AddOwner( typeof(TableColumn), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsRender, new PropertyChangedCallback(OnBackgroundChanged))); #endregion Properties //----------------------------------------------------- // // Static Initialization // //----------------------------------------------------- #region Static Initialization ///property. /// /// Called when the value of the WidthProperty changes /// private static void OnWidthChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Table table = ((TableColumn) d).Table; if(table != null) { table.InvalidateColumns(); } } ////// Called when the value of the BackgroundProperty changes /// private static void OnBackgroundChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Table table = ((TableColumn) d).Table; if(table != null) { table.InvalidateColumns(); } } #endregion Static Initialization } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
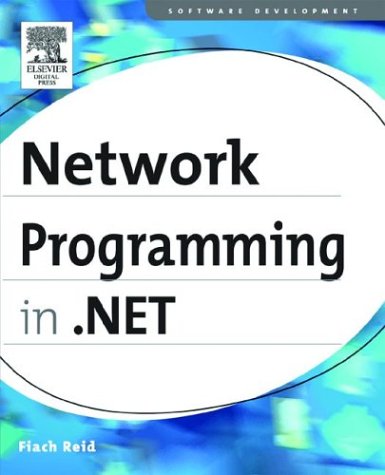
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CurrencyManager.cs
- KeyValueInternalCollection.cs
- ResourceDictionaryCollection.cs
- NonSerializedAttribute.cs
- MaterialCollection.cs
- VisualCollection.cs
- AttachedPropertyDescriptor.cs
- NetMsmqSecurity.cs
- ChildTable.cs
- ExceptQueryOperator.cs
- updatecommandorderer.cs
- PaperSource.cs
- BuildManagerHost.cs
- DispatcherHooks.cs
- ColumnHeaderCollectionEditor.cs
- MembershipSection.cs
- Ipv6Element.cs
- FileDialogPermission.cs
- _Semaphore.cs
- TextControlDesigner.cs
- SafeRegistryKey.cs
- Figure.cs
- SmtpAuthenticationManager.cs
- RetrieveVirtualItemEventArgs.cs
- PageFunction.cs
- TraceHandler.cs
- PropertyValueUIItem.cs
- WeakReferenceList.cs
- DLinqTableProvider.cs
- DaylightTime.cs
- Switch.cs
- CollectionBase.cs
- BridgeDataReader.cs
- SafeNativeMethods.cs
- OdbcInfoMessageEvent.cs
- RootBuilder.cs
- SQLDateTimeStorage.cs
- String.cs
- RSAPKCS1SignatureFormatter.cs
- RewritingSimplifier.cs
- DoubleAnimationUsingPath.cs
- ItemDragEvent.cs
- ExtensionDataReader.cs
- UpdateCompiler.cs
- GenericParameterDataContract.cs
- path.cs
- HttpHeaderCollection.cs
- SocketException.cs
- ListItemViewControl.cs
- ContainerControl.cs
- StylusPointPropertyId.cs
- PassportIdentity.cs
- WebPartTransformerAttribute.cs
- AsymmetricSignatureFormatter.cs
- NumberEdit.cs
- AuthStoreRoleProvider.cs
- DefaultParameterValueAttribute.cs
- DataGridViewToolTip.cs
- DataGridItemCollection.cs
- LineBreak.cs
- BitmapEffectRenderDataResource.cs
- XhtmlBasicCommandAdapter.cs
- ConfigurationPermission.cs
- MessageRpc.cs
- GetRecipientListRequest.cs
- DataGridViewRowsAddedEventArgs.cs
- SqlUnionizer.cs
- PropertiesTab.cs
- NumberFormatInfo.cs
- DataRowCollection.cs
- Html32TextWriter.cs
- RefreshResponseInfo.cs
- Encoder.cs
- RequiredAttributeAttribute.cs
- DataTableExtensions.cs
- GenericAuthenticationEventArgs.cs
- JoinTreeNode.cs
- RectangleHotSpot.cs
- _emptywebproxy.cs
- IUnknownConstantAttribute.cs
- EditorZone.cs
- VectorCollectionValueSerializer.cs
- FlowLayout.cs
- HwndMouseInputProvider.cs
- WsdlWriter.cs
- ValidatorUtils.cs
- PackageDocument.cs
- MasterPage.cs
- ISCIIEncoding.cs
- MetadataException.cs
- WindowsListView.cs
- ProjectionPathBuilder.cs
- ContextMarshalException.cs
- RSAOAEPKeyExchangeFormatter.cs
- HttpModuleCollection.cs
- NameValueFileSectionHandler.cs
- ZipIOCentralDirectoryBlock.cs
- HealthMonitoringSectionHelper.cs
- DbParameterCollectionHelper.cs
- PerfCounterSection.cs