Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ColumnHeaderCollectionEditor.cs / 1 / ColumnHeaderCollectionEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.IO; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms; using System.Drawing; using System.Design; using System.Drawing.Design; using System.Windows.Forms.ComponentModel; using System.Diagnostics.CodeAnalysis; ////// /// internal class ColumnHeaderCollectionEditor : CollectionEditor { ////// Provides an editor for an image collection. ////// /// //Called through reflection [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] public ColumnHeaderCollectionEditor(Type type) : base(type){ } ///Initializes a new instance of the ///class. /// /// protected override string HelpTopic { get { return "net.ComponentModel.ColumnHeaderCollectionEditor"; } } ///Gets the help topic to display for the dialog help button or pressing F1. Override to /// display a different help topic. ////// /// protected override object SetItems(object editValue, object[] value) { if (editValue != null) { Array oldValue = (Array)GetItems(editValue); bool valueSame = (oldValue.Length == value.Length); // We look to see if the value implements IList, and if it does, // we set through that. // Debug.Assert(editValue is System.Collections.IList, "editValue is not an IList"); System.Windows.Forms.ListView.ColumnHeaderCollection list = editValue as System.Windows.Forms.ListView.ColumnHeaderCollection; if (editValue != null) { list.Clear(); System.Windows.Forms.ColumnHeader[] colHeaders = new System.Windows.Forms.ColumnHeader[value.Length]; Array.Copy(value, 0, colHeaders, 0, value.Length); list.AddRange( colHeaders ); } } return editValue; } ////// Sets /// the specified collection to have the specified array of items. /// ////// /// internal override void OnItemRemoving(object item) { ListView listview = this.Context.Instance as ListView; if (listview == null) { return; } System.Windows.Forms.ColumnHeader column = item as System.Windows.Forms.ColumnHeader; if (column != null) { IComponentChangeService cs = GetService(typeof(IComponentChangeService)) as IComponentChangeService; PropertyDescriptor itemsProp = null; if (cs != null) { itemsProp = TypeDescriptor.GetProperties(this.Context.Instance)["Columns"]; cs.OnComponentChanging(this.Context.Instance, itemsProp); } listview.Columns.Remove( column ); if (cs != null && itemsProp != null) { cs.OnComponentChanged(this.Context.Instance, itemsProp, null, null); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Removes the item from listview column header collection /// ///
Link Menu
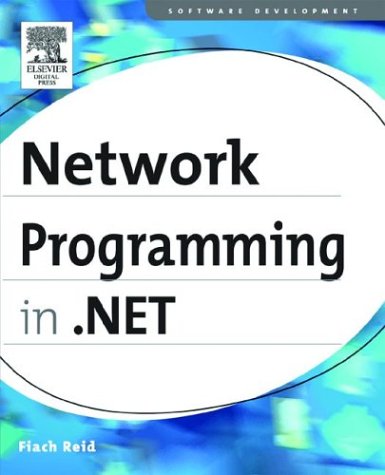
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InvalidWMPVersionException.cs
- GroupDescription.cs
- AQNBuilder.cs
- HostProtectionPermission.cs
- SimplePropertyEntry.cs
- Int32CollectionConverter.cs
- QuaternionKeyFrameCollection.cs
- SecuritySessionFilter.cs
- XamlParser.cs
- Queue.cs
- TCPClient.cs
- AuthorizationRule.cs
- FormsIdentity.cs
- ObjectViewEntityCollectionData.cs
- CachedPathData.cs
- ObjectConverter.cs
- RelationshipSet.cs
- TypeUtil.cs
- Pkcs7Recipient.cs
- CodeArrayCreateExpression.cs
- CachedCompositeFamily.cs
- ShaderRenderModeValidation.cs
- PolicyUnit.cs
- EntityDataSourceMemberPath.cs
- WebCategoryAttribute.cs
- SpecularMaterial.cs
- WSIdentityFaultException.cs
- CompareValidator.cs
- DataGridView.cs
- XamlHostingConfiguration.cs
- ConstructorNeedsTagAttribute.cs
- RelationHandler.cs
- FormsIdentity.cs
- XmlDataDocument.cs
- WindowsUpDown.cs
- PermissionSet.cs
- OptimalBreakSession.cs
- FontConverter.cs
- RegexRunner.cs
- StringValidator.cs
- ReturnEventArgs.cs
- UrlAuthorizationModule.cs
- FillBehavior.cs
- TaiwanCalendar.cs
- Mappings.cs
- Query.cs
- DocumentEventArgs.cs
- ComNativeDescriptor.cs
- DesignerProperties.cs
- RepeatButton.cs
- StateChangeEvent.cs
- CodeExpressionCollection.cs
- DataControlLinkButton.cs
- KeyValuePairs.cs
- BufferBuilder.cs
- CompensatableSequenceActivity.cs
- IPAddressCollection.cs
- StandardOleMarshalObject.cs
- DataGridViewToolTip.cs
- Vector3DConverter.cs
- ShapeTypeface.cs
- ISFClipboardData.cs
- HtmlDocument.cs
- TreeNodeCollection.cs
- BitmapPalette.cs
- mediaclock.cs
- StandardCommands.cs
- ApplicationGesture.cs
- LongValidatorAttribute.cs
- AdornerDecorator.cs
- DefaultBinder.cs
- NodeLabelEditEvent.cs
- DataGridCommandEventArgs.cs
- Win32SafeHandles.cs
- WebPartMinimizeVerb.cs
- TPLETWProvider.cs
- RelationshipWrapper.cs
- TemplateInstanceAttribute.cs
- TemplateXamlParser.cs
- UriPrefixTable.cs
- ActivityStateRecord.cs
- Error.cs
- UnmanagedMemoryStream.cs
- JulianCalendar.cs
- TreeNode.cs
- OrderPreservingMergeHelper.cs
- _ConnectionGroup.cs
- JoinTreeNode.cs
- TreeViewAutomationPeer.cs
- SQLRoleProvider.cs
- MetadataCache.cs
- AttributeEmitter.cs
- OdbcFactory.cs
- X509ChainElement.cs
- HttpWebResponse.cs
- Msmq3PoisonHandler.cs
- SafeViewOfFileHandle.cs
- CssStyleCollection.cs
- XPathDocumentNavigator.cs
- NavigationWindow.cs