Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / SecuritySessionFilter.cs / 1 / SecuritySessionFilter.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- using System; using System.ServiceModel.Channels; using System.ServiceModel.Dispatcher; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.ServiceModel; using System.ServiceModel.Security; using System.Xml; using System.Globalization; using System.IdentityModel.Tokens; namespace System.ServiceModel.Security { sealed class SecuritySessionFilter : HeaderFilter { static readonly string SessionContextIdsProperty = String.Format(CultureInfo.InvariantCulture, "{0}/SecuritySessionContextIds", DotNetSecurityStrings.Namespace); UniqueId securityContextTokenId; SecurityStandardsManager standardsManager; string[] excludedActions; bool isStrictMode; public SecuritySessionFilter(UniqueId securityContextTokenId, SecurityStandardsManager standardsManager, bool isStrictMode, params string[] excludedActions) { if (securityContextTokenId == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("securityContextTokenId")); } this.excludedActions = excludedActions; this.securityContextTokenId = securityContextTokenId; this.standardsManager = standardsManager; this.isStrictMode = isStrictMode; } public UniqueId SecurityContextTokenId { get { return this.securityContextTokenId; } } static bool ShouldExcludeMessage(Message message, string[] excludedActions) { string action = message.Headers.Action; if (excludedActions == null || action == null) { return false; } for (int i = 0; i < excludedActions.Length; ++i) { if (String.Equals(action, excludedActions[i], StringComparison.Ordinal)) { return true; } } return false; } internal static bool CanHandleException(Exception e) { return ((e is XmlException) || (e is FormatException) || (e is SecurityTokenException) || (e is MessageSecurityException) || (e is ProtocolException) || (e is InvalidOperationException) || (e is ArgumentException)); } public override bool Match(Message message) { if (ShouldExcludeMessage(message, this.excludedActions)) { return false; } ListcontextIds; object propertyValue; if (!message.Properties.TryGetValue(SessionContextIdsProperty, out propertyValue)) { contextIds = new List (1); try { if (!this.standardsManager.TryGetSecurityContextIds(message, message.Version.Envelope.UltimateDestinationActorValues, this.isStrictMode, contextIds)) { return false; } } #pragma warning suppress 56500 // covered by FxCOP catch (Exception e) { if (!CanHandleException(e)) throw; return false; } message.Properties.Add(SessionContextIdsProperty, contextIds); } else { contextIds = (propertyValue as List ); if (contextIds == null) { return false; } } for (int i = 0; i < contextIds.Count; ++i) { if (contextIds[i] == this.securityContextTokenId) { message.Properties.Remove(SessionContextIdsProperty); return true; } } return false; } public override bool Match(MessageBuffer buffer) { using (Message message = buffer.CreateMessage()) { return Match(message); } } protected internal override IMessageFilterTable CreateFilterTable () { return new SecuritySessionFilterTable (this.standardsManager, this.isStrictMode, this.excludedActions); } class SecuritySessionFilterTable : IMessageFilterTable { Dictionary > contextMappings; Dictionary filterMappings; SecurityStandardsManager standardsManager; string[] excludedActions; bool isStrictMode; public SecuritySessionFilterTable(SecurityStandardsManager standardsManager, bool isStrictMode, string[] excludedActions) { if (standardsManager == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("standardsManager"); } if (excludedActions == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("excludedActions"); } this.standardsManager = standardsManager; this.excludedActions = new string[excludedActions.Length]; excludedActions.CopyTo(this.excludedActions, 0); this.isStrictMode = isStrictMode; contextMappings = new Dictionary >(); filterMappings = new Dictionary (); } public ICollection Keys { get { return this.filterMappings.Keys; } } public ICollection Values { get { return this.filterMappings.Values; } } public FilterData this[MessageFilter filter] { get { return this.filterMappings[filter]; } set { if (this.filterMappings.ContainsKey(filter)) { this.Remove(filter); } this.Add(filter, value); } } public int Count { get { return this.filterMappings.Count; } } public bool IsReadOnly { get { return false; } } public void Add(KeyValuePair item) { this.Add(item.Key, item.Value); } public void Clear() { this.filterMappings.Clear(); this.contextMappings.Clear(); } public bool Contains(KeyValuePair item) { return this.ContainsKey(item.Key); } public void CopyTo(KeyValuePair [] array, int arrayIndex) { int pos = arrayIndex; foreach (KeyValuePair entry in this.contextMappings.Values) { array[pos] = entry; ++pos; } } public bool Remove(KeyValuePair item) { return this.Remove(item.Key); } IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } public IEnumerator > GetEnumerator() { return ((ICollection >)this.contextMappings.Values).GetEnumerator(); } public void Add(MessageFilter filter, FilterData data) { SecuritySessionFilter sessionFilter = filter as SecuritySessionFilter; if (sessionFilter == null) { DiagnosticUtility.DebugAssert(String.Format(CultureInfo.InvariantCulture, "Unknown filter type {0}", filter.GetType())); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR.GetString(SR.UnknownFilterType, filter.GetType()))); } if (sessionFilter.standardsManager != this.standardsManager) { DiagnosticUtility.DebugAssert("Standards manager of filter does not match that of filter table"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR.GetString(SR.StandardsManagerDoesNotMatch))); } if (sessionFilter.isStrictMode != this.isStrictMode) { DiagnosticUtility.DebugAssert("Session filter's isStrictMode differs from filter table's isStrictMode"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR.GetString(SR.FilterStrictModeDifferent))); } if (this.contextMappings.ContainsKey(sessionFilter.SecurityContextTokenId)) { DiagnosticUtility.DebugAssert(SR.GetString(SR.SecuritySessionIdAlreadyPresentInFilterTable, sessionFilter.SecurityContextTokenId)); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.SecuritySessionIdAlreadyPresentInFilterTable, sessionFilter.SecurityContextTokenId))); } this.filterMappings.Add(filter, data); this.contextMappings.Add(sessionFilter.SecurityContextTokenId, new KeyValuePair (filter, data)); } public bool ContainsKey(MessageFilter filter) { return this.filterMappings.ContainsKey(filter); } public bool Remove(MessageFilter filter) { SecuritySessionFilter sessionFilter = filter as SecuritySessionFilter; if (sessionFilter == null) { return false; } bool result = this.filterMappings.Remove(filter); if (result) { this.contextMappings.Remove(sessionFilter.SecurityContextTokenId); } return result; } public bool TryGetValue(MessageFilter filter, out FilterData data) { return this.filterMappings.TryGetValue(filter, out data); } bool TryGetContextIds(Message message, out List contextIds) { object propertyValue; if (!message.Properties.TryGetValue(SessionContextIdsProperty, out propertyValue)) { contextIds = new List (1); return this.standardsManager.TryGetSecurityContextIds(message, message.Version.Envelope.UltimateDestinationActorValues, isStrictMode, contextIds); } else { contextIds = propertyValue as List ; return (contextIds != null); } } bool TryMatchCore(Message message, out KeyValuePair match) { match = default(KeyValuePair ); if (ShouldExcludeMessage(message, this.excludedActions)) { return false; } List contextIds; try { if (!TryGetContextIds(message, out contextIds)) { return false; } } #pragma warning suppress 56500 // covered by FxCOP catch (Exception e) { if (!SecuritySessionFilter.CanHandleException(e)) throw; return false; } for (int i = 0; i < contextIds.Count; ++i) { if (this.contextMappings.TryGetValue(contextIds[i], out match)) { message.Properties.Remove(SessionContextIdsProperty); return true; } } return false; } public bool GetMatchingValue(Message message, out FilterData data) { KeyValuePair matchingPair; if (!TryMatchCore(message, out matchingPair)) { data = default(FilterData); return false; } data = matchingPair.Value; return true; } public bool GetMatchingValue(MessageBuffer buffer, out FilterData data) { using (Message message = buffer.CreateMessage()) { return GetMatchingValue(message, out data); } } public bool GetMatchingValues(Message message, ICollection results) { FilterData matchingData; if (!GetMatchingValue(message, out matchingData)) { return false; } results.Add(matchingData); return true; } public bool GetMatchingValues(MessageBuffer buffer, ICollection results) { using (Message message = buffer.CreateMessage()) { return GetMatchingValues(message, results); } } public bool GetMatchingFilter(Message message, out MessageFilter filter) { KeyValuePair matchingPair; if (!TryMatchCore(message, out matchingPair)) { filter = null; return false; } filter = matchingPair.Key; return true; } public bool GetMatchingFilter(MessageBuffer buffer, out MessageFilter filter) { using (Message message = buffer.CreateMessage()) { return GetMatchingFilter(message, out filter); } } public bool GetMatchingFilters(Message message, ICollection results) { MessageFilter match; if (GetMatchingFilter(message, out match)) { results.Add(match); return true; } return false; } public bool GetMatchingFilters(MessageBuffer buffer, ICollection results) { using (Message message = buffer.CreateMessage()) { return GetMatchingFilters(message, results); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
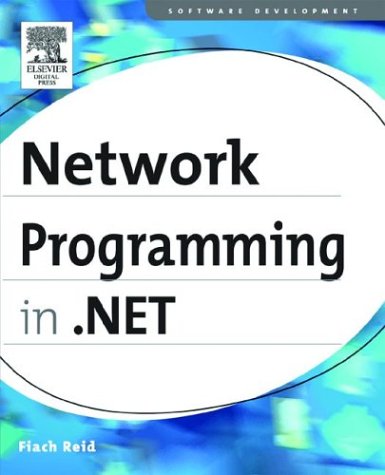
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextRangeBase.cs
- BitmapVisualManager.cs
- ComboBoxRenderer.cs
- RoleManagerEventArgs.cs
- MSG.cs
- exports.cs
- ChineseLunisolarCalendar.cs
- XmlAutoDetectWriter.cs
- NativeActivityMetadata.cs
- WinFormsSecurity.cs
- webeventbuffer.cs
- PolicyDesigner.cs
- KeyboardDevice.cs
- DesignerOptionService.cs
- DataTableMapping.cs
- OdbcConnection.cs
- BlurBitmapEffect.cs
- While.cs
- ArgumentsParser.cs
- SoapHttpTransportImporter.cs
- Simplifier.cs
- InheritedPropertyChangedEventArgs.cs
- CommonDialog.cs
- GenericTypeParameterBuilder.cs
- Int64Storage.cs
- HostExecutionContextManager.cs
- CellPartitioner.cs
- NaturalLanguageHyphenator.cs
- CellParaClient.cs
- AnnotationAuthorChangedEventArgs.cs
- exports.cs
- MiniConstructorInfo.cs
- TraceContext.cs
- DataViewManagerListItemTypeDescriptor.cs
- DataGridViewRowCancelEventArgs.cs
- Guid.cs
- DynamicUpdateCommand.cs
- MemberDomainMap.cs
- SerialPort.cs
- TranslateTransform3D.cs
- KeyboardDevice.cs
- MenuItemStyleCollection.cs
- DataQuery.cs
- ResourceManager.cs
- XmlSchemaComplexContentExtension.cs
- XmlMembersMapping.cs
- RectAnimation.cs
- AutomationEvent.cs
- CodeTypeReferenceExpression.cs
- SelectiveScrollingGrid.cs
- ParameterCollectionEditor.cs
- XPathParser.cs
- VSWCFServiceContractGenerator.cs
- SamlAssertion.cs
- BindingValueChangedEventArgs.cs
- ImmutablePropertyDescriptorGridEntry.cs
- HtmlValidationSummaryAdapter.cs
- TextModifierScope.cs
- recordstatefactory.cs
- Point.cs
- UriWriter.cs
- SqlDataSourceCommandEventArgs.cs
- CodeCompiler.cs
- WebPartManagerInternals.cs
- CardSpaceShim.cs
- TriggerCollection.cs
- GeneralTransform3D.cs
- LinkUtilities.cs
- DecimalKeyFrameCollection.cs
- DivideByZeroException.cs
- AndCondition.cs
- DecryptedHeader.cs
- BitStream.cs
- AnnotationService.cs
- Bitmap.cs
- PlatformNotSupportedException.cs
- PackageFilter.cs
- UnmanagedHandle.cs
- EventLogConfiguration.cs
- StoreContentChangedEventArgs.cs
- DbDataSourceEnumerator.cs
- SystemNetworkInterface.cs
- SecureStringHasher.cs
- FontInfo.cs
- MemberDescriptor.cs
- EncoderExceptionFallback.cs
- _AcceptOverlappedAsyncResult.cs
- DependencyPropertyKind.cs
- PerformanceCounter.cs
- XhtmlBasicObjectListAdapter.cs
- PersonalizationStateQuery.cs
- InputScopeManager.cs
- TextViewBase.cs
- ShapingWorkspace.cs
- externdll.cs
- OrderByQueryOptionExpression.cs
- Polyline.cs
- FilterEventArgs.cs
- TextCharacters.cs
- NavigateEvent.cs