Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / ServiceModel / Configuration / PersistenceProviderElement.cs / 1305376 / PersistenceProviderElement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Configuration { using System; using System.Collections.Specialized; using System.ComponentModel; using System.Configuration; using System.Diagnostics.CodeAnalysis; using System.Reflection; using System.Runtime; using System.ServiceModel.Description; using System.ServiceModel.Persistence; using System.Workflow.Runtime; using System.Xml; public class PersistenceProviderElement : BehaviorExtensionElement { const string persistenceOperationTimeoutParameter = "persistenceOperationTimeout"; const string typeParameter = "type"; int argumentsHash; NameValueCollection persistenceProviderArguments; public PersistenceProviderElement() { this.persistenceProviderArguments = new NameValueCollection(); this.argumentsHash = this.ComputeArgumentsHash(); } // This property is not supposed to be exposed in config. [SuppressMessage("Configuration", "Configuration102:ConfigurationPropertyAttributeRule")] public override Type BehaviorType { get { return typeof(PersistenceProviderBehavior); } } [ConfigurationProperty( persistenceOperationTimeoutParameter, IsRequired = false, DefaultValue = PersistenceProviderBehavior.DefaultPersistenceOperationTimeoutString)] [TypeConverter(typeof(TimeSpanOrInfiniteConverter))] [PositiveTimeSpanValidator] public TimeSpan PersistenceOperationTimeout { get { return (TimeSpan) base[persistenceOperationTimeoutParameter]; } set { base[persistenceOperationTimeoutParameter] = value; } } [SuppressMessage("Configuration", "Configuration102:ConfigurationPropertyAttributeRule")] public NameValueCollection PersistenceProviderArguments { get { return this.persistenceProviderArguments; } } [ConfigurationProperty(typeParameter, IsRequired = true)] [StringValidator(MinLength = 0)] public string Type { get { return (string) base[typeParameter]; } set { base[typeParameter] = value; } } protected internal override object CreateBehavior() { Fx.Assert(this.PersistenceOperationTimeout > TimeSpan.Zero, "This should have been guaranteed by the validator on the setter."); PersistenceProviderFactory providerFactory; Type providerType = System.Type.GetType((string) base[typeParameter]); if (providerType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new InvalidOperationException(SR2.GetString(SR2.PersistenceProviderTypeNotFound))); } ConstructorInfo cInfo = providerType.GetConstructor(new Type[] { typeof(NameValueCollection) }); if (cInfo != null) { providerFactory = (PersistenceProviderFactory) cInfo.Invoke(new object[] { this.persistenceProviderArguments }); } else { cInfo = providerType.GetConstructor(new Type[] { }); Fx.Assert(cInfo != null, "The constructor should have been found - this should have been validated elsewhere."); providerFactory = (PersistenceProviderFactory) cInfo.Invoke(null); } return new PersistenceProviderBehavior(providerFactory, this.PersistenceOperationTimeout); } protected override bool IsModified() { return base.IsModified() || this.argumentsHash != this.ComputeArgumentsHash(); } protected override bool OnDeserializeUnrecognizedAttribute(string name, string value) { persistenceProviderArguments.Add(name, value); return true; } protected override void PostDeserialize() { this.argumentsHash = this.ComputeArgumentsHash(); base.PostDeserialize(); } protected override bool SerializeElement(XmlWriter writer, bool serializeCollectionKey) { bool result; if (writer != null) { foreach (string key in this.persistenceProviderArguments.AllKeys) { writer.WriteAttributeString(key, this.persistenceProviderArguments[key]); } result = base.SerializeElement(writer, serializeCollectionKey); result |= this.persistenceProviderArguments.Count > 0; this.argumentsHash = this.ComputeArgumentsHash(); } else { result = base.SerializeElement(writer, serializeCollectionKey); } return result; } protected override void Unmerge(ConfigurationElement sourceElement, ConfigurationElement parentElement, ConfigurationSaveMode saveMode) { PersistenceProviderElement persistenceProviderElement = (PersistenceProviderElement) sourceElement; this.persistenceProviderArguments = new NameValueCollection(persistenceProviderElement.persistenceProviderArguments); this.argumentsHash = persistenceProviderElement.argumentsHash; base.Unmerge(sourceElement, parentElement, saveMode); } int ComputeArgumentsHash() { int result = 0; foreach (string key in this.persistenceProviderArguments.AllKeys) { result ^= key.GetHashCode() ^ this.persistenceProviderArguments[key].GetHashCode(); } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
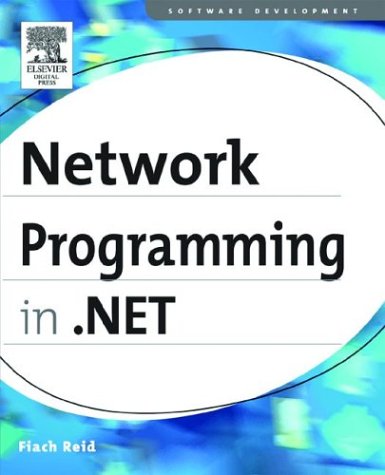
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResXBuildProvider.cs
- UnionCodeGroup.cs
- AssertSection.cs
- BaseUriHelper.cs
- SafeProcessHandle.cs
- TypeInfo.cs
- WebSysDisplayNameAttribute.cs
- ComponentConverter.cs
- TypeConverter.cs
- HtmlSelectionListAdapter.cs
- ImplicitInputBrush.cs
- SafeProcessHandle.cs
- Visual3D.cs
- SectionUpdates.cs
- IndicShape.cs
- ElementMarkupObject.cs
- EventLog.cs
- ButtonStandardAdapter.cs
- XpsColorContext.cs
- ServiceHostFactory.cs
- UnauthorizedWebPart.cs
- ExpressionBuilderCollection.cs
- DefaultValueTypeConverter.cs
- TreeViewAutomationPeer.cs
- LinqDataSourceValidationException.cs
- FontFaceLayoutInfo.cs
- ManagementOperationWatcher.cs
- UnsafeNativeMethods.cs
- ThreadPool.cs
- VisualBasicImportReference.cs
- EventProviderTraceListener.cs
- CmsUtils.cs
- ElementUtil.cs
- OpenTypeLayout.cs
- AQNBuilder.cs
- X509UI.cs
- AppDomainProtocolHandler.cs
- DataSourceCache.cs
- HeaderUtility.cs
- UrlMappingCollection.cs
- TemplatePropertyEntry.cs
- SqlCachedBuffer.cs
- BoundPropertyEntry.cs
- Activator.cs
- ValueProviderWrapper.cs
- SqlReorderer.cs
- ProtocolsConfiguration.cs
- XmlWrappingReader.cs
- UserPreferenceChangingEventArgs.cs
- PipelineModuleStepContainer.cs
- SafeThemeHandle.cs
- CompiledRegexRunnerFactory.cs
- DataServiceConfiguration.cs
- DataServiceProviderMethods.cs
- QueueSurrogate.cs
- _RequestCacheProtocol.cs
- UserControl.cs
- InputReport.cs
- MobileComponentEditorPage.cs
- MimeMultiPart.cs
- CacheMemory.cs
- SQLInt64.cs
- TreeNodeCollection.cs
- TextDecorationLocationValidation.cs
- IssuedTokenServiceCredential.cs
- ColumnPropertiesGroup.cs
- TextEditorMouse.cs
- MsmqChannelListenerBase.cs
- AbsoluteQuery.cs
- NameValuePermission.cs
- CookieProtection.cs
- StackSpiller.Bindings.cs
- MatrixAnimationUsingPath.cs
- StructuredTypeInfo.cs
- OdbcCommand.cs
- SignatureHelper.cs
- MenuItemStyleCollection.cs
- MetadataItemCollectionFactory.cs
- MessagePropertyDescriptionCollection.cs
- Glyph.cs
- XPathNavigator.cs
- PixelFormatConverter.cs
- TagMapCollection.cs
- ImpersonateTokenRef.cs
- CqlIdentifiers.cs
- HttpConfigurationContext.cs
- BoundsDrawingContextWalker.cs
- Slider.cs
- Environment.cs
- Span.cs
- ProcessHost.cs
- ParsedAttributeCollection.cs
- XmlJsonWriter.cs
- DoubleCollection.cs
- DesignerTextBoxAdapter.cs
- SamlAttribute.cs
- TypeRestriction.cs
- QuotedPrintableStream.cs
- WebBrowserNavigatedEventHandler.cs
- XmlSchemaElement.cs