Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / TypeRestriction.cs / 1305376 / TypeRestriction.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Common.Utils.Boolean; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration.Structures { using DomainBoolExpr = BoolExpr>; using CellConstantSet = Set ; // A class that denotes the boolean expression: "varType in values" // See the comments in OneOfConst for partially and fully-done objects internal class TypeRestriction : MemberRestriction { #region Constructors // effects: Creates a partial OneOfTypeConst of the form "node in values" internal TypeRestriction(MemberPath node, IEnumerable values) : base(new MemberProjectedSlot(node), CreateTypeConstants(values)) { } // effects: Creates a partial OneOfTypeConst of the form "node = values" internal TypeRestriction(MemberPath node, Constant value) : base(new MemberProjectedSlot(node), value) { Debug.Assert(value is TypeConstant || value.IsNull(), "Type or NULL expected"); } // effects: Creates an expression of the form "type of node in type constants // corresponding to values", i.e., a boolean expression of the form "TypeOf(slot) in values" // possibleValues are all the values that node's type can take internal TypeRestriction(MemberPath node, IEnumerable values, IEnumerable possibleValues) : base(new MemberProjectedSlot(node), CreateTypeConstants(values), CreateTypeConstants(possibleValues)) { } // effects: Creates a "fully-done" OneOfTypeConst that represents // "node in values". possibleValues are all the values that node's type can take internal TypeRestriction(MemberPath node, IEnumerable values, IEnumerable possibleValues) : base(new MemberProjectedSlot(node), values, possibleValues) { } // effects: Creates an expression of the form "slot = value" // possibleValues are all the values that slot can take internal TypeRestriction(MemberPath node, Constant value, IEnumerable possibleValues) : base(new MemberProjectedSlot(node), value, possibleValues) { Debug.Assert(value is TypeConstant || value.IsNull(), "Type or NULL expected"); } // effects: Creates an expression of the form "slot in domain" internal TypeRestriction(MemberProjectedSlot slot, Domain domain) : base(slot, domain) { } #endregion #region Helper static methods // effects: Given a list of types (which can contain nulls), // returns a corresponding list of Type Constants for those types private static IEnumerable CreateTypeConstants(IEnumerable types) { foreach (EdmType type in types) { if (type == null) { yield return Constant.Null; } else { yield return new TypeConstant(type); } } } #endregion #region Methods // requires: IsFullyDone is true // effects: Fixes the range of this in accordance with range internal override DomainBoolExpr FixRange(Set range, MemberDomainMap memberDomainMap) { Debug.Assert(IsFullyDone, "Ranges are fixed only for fully done OneOfConsts"); IEnumerable possibleValues = memberDomainMap.GetDomain(RestrictedMemberSlot.MemberPath); BoolLiteral newLiteral = new TypeRestriction(RestrictedMemberSlot, new Domain(range, possibleValues)); return newLiteral.GetDomainBoolExpression(memberDomainMap); } internal override StringBuilder AsCql(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { // type comparison: make a big OR // Important to enclose all the OR statements in () ! if (Domain.Count > 1) { builder.Append('('); } bool isFirst = true; // Add Cql of the form "(T.A IS OF (ONLY Person) OR .....)" foreach (Constant constant in Domain.Values) { TypeConstant typeConstant = constant as TypeConstant; Debug.Assert(typeConstant != null || constant.IsNull(), "Constants for type checks must be type constants or NULLs"); if (isFirst == false) { builder.Append(" OR "); } isFirst = false; if (RestrictedMemberSlot.MemberPath.EdmType is RefType) { builder.Append("Deref("); RestrictedMemberSlot.MemberPath.AsCql(builder, blockAlias); builder.Append(')'); } else { // non-reference type RestrictedMemberSlot.MemberPath.AsCql(builder, blockAlias); } if (constant.IsNull()) { builder.Append(" IS NULL"); } else { // type constant builder.Append(" IS OF (ONLY "); CqlWriter.AppendEscapedTypeName(builder, typeConstant.CdmType); builder.Append(')'); } } if (Domain.Count > 1) { builder.Append(')'); } return builder; } internal override StringBuilder AsUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { // Add user readable string of the form "(T.A IS OF (ONLY Person) OR .....)" if (RestrictedMemberSlot.MemberPath.EdmType is RefType) { builder.Append("Deref("); RestrictedMemberSlot.MemberPath.AsCql(builder, blockAlias); builder.Append(')'); } else { // non-reference type RestrictedMemberSlot.MemberPath.AsCql(builder, blockAlias); } if (Domain.Count > 1) { builder.Append(" is a ("); } else { builder.Append(" is type "); } bool isFirst = true; foreach (Constant constant in Domain.Values) { TypeConstant typeConstant = constant as TypeConstant; Debug.Assert(typeConstant != null || constant.IsNull(), "Constants for type checks must be type constants or NULLs"); if (isFirst == false) { builder.Append(" OR "); } if (constant.IsNull()) { builder.Append(" NULL"); } else { CqlWriter.AppendEscapedTypeName(builder, typeConstant.CdmType); } isFirst = false; } if (Domain.Count > 1) { builder.Append(')'); } return builder; } #endregion #region String methods internal override void ToCompactString(StringBuilder builder) { builder.Append("type("); RestrictedMemberSlot.ToCompactString(builder); builder.Append(") IN ("); StringUtil.ToCommaSeparatedStringSorted(builder, Domain.Values); builder.Append(")"); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
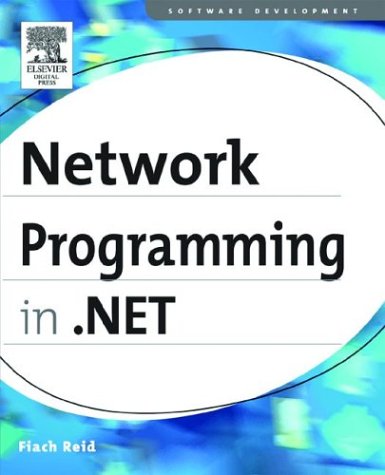
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataProtection.cs
- AttachedPropertyMethodSelector.cs
- XmlQualifiedNameTest.cs
- NullRuntimeConfig.cs
- RuleSettings.cs
- MessageBodyDescription.cs
- SuppressMergeCheckAttribute.cs
- KeyEvent.cs
- DocumentPageHost.cs
- XPathMessageContext.cs
- TypeConstant.cs
- SecurityElement.cs
- Environment.cs
- MetroSerializationManager.cs
- CatalogPartCollection.cs
- Sequence.cs
- OdbcPermission.cs
- Crypto.cs
- ControlCommandSet.cs
- ConnectionPoint.cs
- ToggleProviderWrapper.cs
- DnsEndPoint.cs
- TransformationRules.cs
- ExtentCqlBlock.cs
- ToolStripScrollButton.cs
- PromptEventArgs.cs
- SchemaConstraints.cs
- KeyedQueue.cs
- ProtocolsConfiguration.cs
- DropShadowBitmapEffect.cs
- StylusCaptureWithinProperty.cs
- WebConfigurationManager.cs
- AssociationSetMetadata.cs
- ParallelDesigner.cs
- SerializationStore.cs
- UserControl.cs
- WebPartHelpVerb.cs
- SignatureToken.cs
- LinkTarget.cs
- ExpressionNode.cs
- WebPartVerbCollection.cs
- SspiNegotiationTokenAuthenticatorState.cs
- DataSysAttribute.cs
- CorrelationToken.cs
- PropertyCollection.cs
- ErrorWebPart.cs
- DataGridViewCheckBoxColumn.cs
- QuinticEase.cs
- HyperLinkStyle.cs
- WebBrowserNavigatingEventHandler.cs
- TextContainerChangeEventArgs.cs
- Number.cs
- SortedList.cs
- MsmqOutputChannel.cs
- TreeNode.cs
- latinshape.cs
- URI.cs
- DynamicUpdateCommand.cs
- BufferAllocator.cs
- MarkerProperties.cs
- DataControlButton.cs
- ListBoxItemAutomationPeer.cs
- KeyBinding.cs
- XmlSerializerFactory.cs
- MediaContextNotificationWindow.cs
- DataPagerCommandEventArgs.cs
- DataGridColumnHeadersPresenter.cs
- ConfigurationStrings.cs
- SystemIcmpV4Statistics.cs
- MembershipValidatePasswordEventArgs.cs
- AudioFormatConverter.cs
- StylusPointPropertyInfo.cs
- TextOutput.cs
- TemplatedMailWebEventProvider.cs
- CategoryList.cs
- XpsFixedDocumentSequenceReaderWriter.cs
- Handle.cs
- InvokeWebServiceDesigner.cs
- XmlComment.cs
- selecteditemcollection.cs
- ScriptReferenceEventArgs.cs
- SpellCheck.cs
- ProfileInfo.cs
- CompositionDesigner.cs
- XmlArrayItemAttributes.cs
- PerformanceCounterPermissionEntry.cs
- AssemblyHash.cs
- TextElementEnumerator.cs
- CodeTryCatchFinallyStatement.cs
- ToolStripTemplateNode.cs
- DataControlField.cs
- DoubleLinkList.cs
- BuiltInExpr.cs
- PageBuildProvider.cs
- ActivityWithResultValueSerializer.cs
- Util.cs
- Paragraph.cs
- PropertyChangingEventArgs.cs
- HashAlgorithm.cs
- SchemaCollectionCompiler.cs