Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities.DurableInstancing / System / Activities / DurableInstancing / InstanceLockTracking.cs / 1305376 / InstanceLockTracking.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.DurableInstancing { using System.Runtime.DurableInstancing; using System.Transactions; sealed class InstanceLockTracking { object synchLock; SqlWorkflowInstanceStore store; public InstanceLockTracking(SqlWorkflowInstanceStore store) { this.InstanceId = Guid.Empty; this.store = store; this.synchLock = new object(); } public Guid InstanceId { get; set; } public bool BoundToLock { get; set; } public long InstanceVersion { get; set; } public bool IsHandleFreed { get; set; } public bool IsSafeToUnlock { get; set; } public void HandleFreed() { lock (this.synchLock) { if (this.BoundToLock && this.IsSafeToUnlock) { this.store.GenerateUnlockCommand(this); } this.IsHandleFreed = true; } } public void TrackStoreLock(Guid instanceId, long instanceVersion, DependentTransaction dependentTransaction) { this.BoundToLock = true; this.InstanceId = instanceId; this.InstanceVersion = instanceVersion; if (dependentTransaction != null) { dependentTransaction.TransactionCompleted += new TransactionCompletedEventHandler(TransactionCompleted); } else { this.IsSafeToUnlock = true; } } public void TrackStoreUnlock(DependentTransaction dependentTransaction) { this.BoundToLock = false; this.IsHandleFreed = true; if (dependentTransaction != null) { dependentTransaction.TransactionCompleted += new TransactionCompletedEventHandler(TransactedUnlockCompleted); } } void TransactionCompleted(object sender, TransactionEventArgs e) { lock (this.synchLock) { if (e.Transaction.TransactionInformation.Status == TransactionStatus.Committed) { if (this.IsHandleFreed) { this.store.GenerateUnlockCommand(this); } else { this.IsSafeToUnlock = true; } } else { this.BoundToLock = false; } } } void TransactedUnlockCompleted(object sender, TransactionEventArgs e) { lock (this.synchLock) { if (e.Transaction.TransactionInformation.Status != TransactionStatus.Committed && this.IsSafeToUnlock) { this.store.GenerateUnlockCommand(this); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
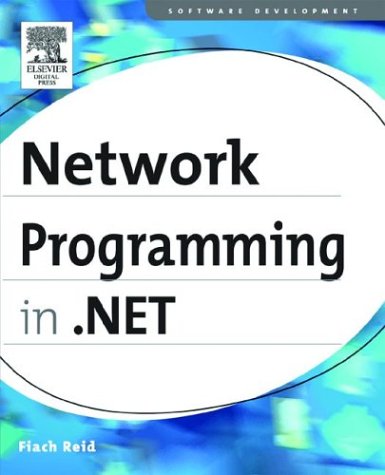
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QualifiedCellIdBoolean.cs
- Vector3DConverter.cs
- NumberFormatInfo.cs
- XmlByteStreamWriter.cs
- HitTestWithGeometryDrawingContextWalker.cs
- OdbcConnectionPoolProviderInfo.cs
- _AcceptOverlappedAsyncResult.cs
- Int32.cs
- KeyValueInternalCollection.cs
- SHA256Managed.cs
- ScriptRegistrationManager.cs
- XPathSingletonIterator.cs
- InvokeAction.cs
- AuthenticationService.cs
- designeractionbehavior.cs
- safesecurityhelperavalon.cs
- ErrorFormatterPage.cs
- webbrowsersite.cs
- TextServicesLoader.cs
- UriScheme.cs
- WebPartEditorCancelVerb.cs
- ConnectivityStatus.cs
- ObjectNavigationPropertyMapping.cs
- StringSource.cs
- NetPeerTcpBindingElement.cs
- Inline.cs
- DataGridViewCellStateChangedEventArgs.cs
- ToolStripSeparator.cs
- Matrix3D.cs
- Int32CollectionValueSerializer.cs
- SystemIPv6InterfaceProperties.cs
- SafeSecurityHandles.cs
- DnsPermission.cs
- RetriableClipboard.cs
- RowUpdatedEventArgs.cs
- ByValueEqualityComparer.cs
- OdbcConnectionStringbuilder.cs
- MemberAccessException.cs
- ResourceBinder.cs
- SignatureHelper.cs
- AuthStoreRoleProvider.cs
- FontStretchConverter.cs
- DataBindingCollectionConverter.cs
- ISAPIWorkerRequest.cs
- DllNotFoundException.cs
- Util.cs
- XmlQueryType.cs
- FileLevelControlBuilderAttribute.cs
- TableMethodGenerator.cs
- DesignBindingConverter.cs
- InputScopeAttribute.cs
- HtmlTableCellCollection.cs
- EmptyReadOnlyDictionaryInternal.cs
- ListControl.cs
- HyperLinkDesigner.cs
- ScriptManagerProxy.cs
- HttpNamespaceReservationInstallComponent.cs
- EventProperty.cs
- TableRowCollection.cs
- PreservationFileWriter.cs
- WindowsListViewGroupSubsetLink.cs
- HMACSHA512.cs
- CatalogPart.cs
- RuleAction.cs
- RectAnimation.cs
- AudienceUriMode.cs
- WindowsFormsSectionHandler.cs
- Vector3DKeyFrameCollection.cs
- ResXFileRef.cs
- HttpHandlerAction.cs
- DelegatedStream.cs
- EventItfInfo.cs
- NCryptNative.cs
- BaseCollection.cs
- SvcMapFileLoader.cs
- Point.cs
- DataGridViewColumnEventArgs.cs
- XmlSchemaSimpleContentExtension.cs
- MarkupWriter.cs
- SecurityCriticalDataForSet.cs
- SHA1.cs
- MobileCategoryAttribute.cs
- ButtonColumn.cs
- BinaryFormatterWriter.cs
- DataDocumentXPathNavigator.cs
- OpCodes.cs
- WinInetCache.cs
- CodeConditionStatement.cs
- DataKeyArray.cs
- StringFreezingAttribute.cs
- XXXInfos.cs
- SynchronizationContext.cs
- IisTraceListener.cs
- RegexRunner.cs
- DynamicVirtualDiscoSearcher.cs
- FilePrompt.cs
- VisualState.cs
- ListMarkerSourceInfo.cs
- RtfToXamlLexer.cs
- WebException.cs