Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / TableRowCollection.cs / 1 / TableRowCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ Editor("System.Web.UI.Design.WebControls.TableRowsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TableRowCollection : IList { ///Encapsulates the collection of ///objects within a control. /// A protected field of type private Table owner; ///. Represents the collection internally. /// /// internal TableRowCollection(Table owner) { this.owner = owner; } ////// Gets the /// count of public int Count { get { if (owner.HasControls()) { return owner.Controls.Count; } return 0; } } ///in the collection. /// /// public TableRow this[int index] { get { return(TableRow)owner.Controls[index]; } } ////// Gets a ///referenced by the /// specified ordinal index value. /// /// public int Add(TableRow row) { AddAt(-1, row); return owner.Controls.Count - 1; } ////// Adds the specified ///to the end of the collection. /// /// public void AddAt(int index, TableRow row) { owner.Controls.AddAt(index, row); if (row.TableSection != TableRowSection.TableBody) { owner.HasRowSections = true; } } ////// Adds the specified ///to the collection at the specified /// index location. /// /// public void AddRange(TableRow[] rows) { if (rows == null) { throw new ArgumentNullException("rows"); } foreach(TableRow row in rows) { Add(row); } } ////// public void Clear() { if (owner.HasControls()) { owner.Controls.Clear(); owner.HasRowSections = false; } } ///Removes all ///controls from the collection. /// public int GetRowIndex(TableRow row) { if (owner.HasControls()) { return owner.Controls.IndexOf(row); } return -1; } ///Returns an ordinal index value that denotes the position of the specified /// ///within the collection. /// public IEnumerator GetEnumerator() { return owner.Controls.GetEnumerator(); } ////// Returns an enumerator of all ///controls within the /// collection. /// /// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies contents from the collection to the specified ///with the /// specified starting index. /// public Object SyncRoot { get { return this;} } ////// Gets the object that can be used to synchronize access to the collection. In /// this case, it is the collection itself. /// ////// public bool IsReadOnly { get { return false;} } ////// Gets a value indicating whether the collection is read-only. /// ////// public bool IsSynchronized { get { return false;} } ////// Gets a value indicating whether access to the collection is synchronized /// (thread-safe). /// ////// public void Remove(TableRow row) { owner.Controls.Remove(row); } ///Removes the specified ///from the collection. /// public void RemoveAt(int index) { owner.Controls.RemoveAt(index); } // IList implementation, required by collection editor ///Removes the ///from the collection at the specified /// index location. object IList.this[int index] { get { return owner.Controls[index]; } set { RemoveAt(index); AddAt(index, (TableRow)value); } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object o) { return Add((TableRow) o); } /// bool IList.Contains(object o) { return owner.Controls.Contains((TableRow)o); } /// int IList.IndexOf(object o) { return owner.Controls.IndexOf((TableRow)o); } /// void IList.Insert(int index, object o) { AddAt(index, (TableRow)o); } /// void IList.Remove(object o) { Remove((TableRow)o); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ Editor("System.Web.UI.Design.WebControls.TableRowsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TableRowCollection : IList { ///Encapsulates the collection of ///objects within a control. /// A protected field of type private Table owner; ///. Represents the collection internally. /// /// internal TableRowCollection(Table owner) { this.owner = owner; } ////// Gets the /// count of public int Count { get { if (owner.HasControls()) { return owner.Controls.Count; } return 0; } } ///in the collection. /// /// public TableRow this[int index] { get { return(TableRow)owner.Controls[index]; } } ////// Gets a ///referenced by the /// specified ordinal index value. /// /// public int Add(TableRow row) { AddAt(-1, row); return owner.Controls.Count - 1; } ////// Adds the specified ///to the end of the collection. /// /// public void AddAt(int index, TableRow row) { owner.Controls.AddAt(index, row); if (row.TableSection != TableRowSection.TableBody) { owner.HasRowSections = true; } } ////// Adds the specified ///to the collection at the specified /// index location. /// /// public void AddRange(TableRow[] rows) { if (rows == null) { throw new ArgumentNullException("rows"); } foreach(TableRow row in rows) { Add(row); } } ////// public void Clear() { if (owner.HasControls()) { owner.Controls.Clear(); owner.HasRowSections = false; } } ///Removes all ///controls from the collection. /// public int GetRowIndex(TableRow row) { if (owner.HasControls()) { return owner.Controls.IndexOf(row); } return -1; } ///Returns an ordinal index value that denotes the position of the specified /// ///within the collection. /// public IEnumerator GetEnumerator() { return owner.Controls.GetEnumerator(); } ////// Returns an enumerator of all ///controls within the /// collection. /// /// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies contents from the collection to the specified ///with the /// specified starting index. /// public Object SyncRoot { get { return this;} } ////// Gets the object that can be used to synchronize access to the collection. In /// this case, it is the collection itself. /// ////// public bool IsReadOnly { get { return false;} } ////// Gets a value indicating whether the collection is read-only. /// ////// public bool IsSynchronized { get { return false;} } ////// Gets a value indicating whether access to the collection is synchronized /// (thread-safe). /// ////// public void Remove(TableRow row) { owner.Controls.Remove(row); } ///Removes the specified ///from the collection. /// public void RemoveAt(int index) { owner.Controls.RemoveAt(index); } // IList implementation, required by collection editor ///Removes the ///from the collection at the specified /// index location. object IList.this[int index] { get { return owner.Controls[index]; } set { RemoveAt(index); AddAt(index, (TableRow)value); } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object o) { return Add((TableRow) o); } /// bool IList.Contains(object o) { return owner.Controls.Contains((TableRow)o); } /// int IList.IndexOf(object o) { return owner.Controls.IndexOf((TableRow)o); } /// void IList.Insert(int index, object o) { AddAt(index, (TableRow)o); } /// void IList.Remove(object o) { Remove((TableRow)o); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
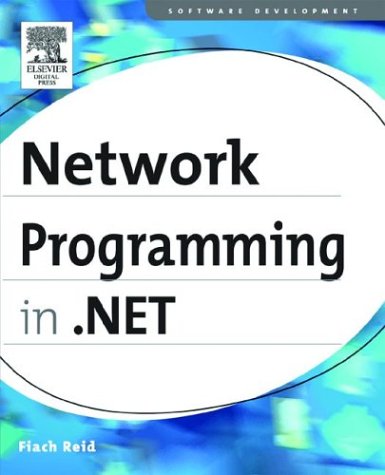
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridItemEventArgs.cs
- ActivityWithResult.cs
- IncrementalReadDecoders.cs
- Globals.cs
- TripleDES.cs
- IndexerHelper.cs
- WinInetCache.cs
- ThreadBehavior.cs
- DocumentPageTextView.cs
- ModelEditingScope.cs
- FieldInfo.cs
- SettingsBase.cs
- HandlerMappingMemo.cs
- JpegBitmapDecoder.cs
- OutputCacheSettings.cs
- StandardToolWindows.cs
- CodeMemberEvent.cs
- VerificationException.cs
- ConvertEvent.cs
- SplitterEvent.cs
- PartialTrustHelpers.cs
- LabelEditEvent.cs
- TextEndOfSegment.cs
- HttpBufferlessInputStream.cs
- RC2CryptoServiceProvider.cs
- XmlElementAttribute.cs
- DesignerTextViewAdapter.cs
- BatchParser.cs
- NativeMethods.cs
- ConfigurationPropertyCollection.cs
- NullRuntimeConfig.cs
- TemplateXamlParser.cs
- DBConnection.cs
- GroupBox.cs
- ErrorProvider.cs
- SourceSwitch.cs
- SourceFilter.cs
- DerivedKeyCachingSecurityTokenSerializer.cs
- DataGridViewRowEventArgs.cs
- FileVersionInfo.cs
- DefaultExpressionVisitor.cs
- StorageEntityContainerMapping.cs
- RepeaterDesigner.cs
- DataGridColumnFloatingHeader.cs
- WriteTimeStream.cs
- Type.cs
- BypassElementCollection.cs
- ConnectionConsumerAttribute.cs
- HtmlImage.cs
- BitmapPalette.cs
- InstanceNotFoundException.cs
- ConfigurationValues.cs
- CompleteWizardStep.cs
- mediaclock.cs
- WebPartDescriptionCollection.cs
- FormatException.cs
- PenCursorManager.cs
- PriorityRange.cs
- ModifierKeysConverter.cs
- PerformanceCountersElement.cs
- DataSetUtil.cs
- BitmapImage.cs
- SelectionProcessor.cs
- ValidationHelper.cs
- RequestCacheValidator.cs
- TimeZone.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- SequentialUshortCollection.cs
- ServerIdentity.cs
- TransformationRules.cs
- HandlerBase.cs
- StickyNote.cs
- RegexCompiler.cs
- IdentityModelDictionary.cs
- SingleConverter.cs
- OracleDataAdapter.cs
- PackUriHelper.cs
- GeometryConverter.cs
- ProfileGroupSettings.cs
- MetafileHeader.cs
- CompilerGeneratedAttribute.cs
- CodeArrayCreateExpression.cs
- XPathBinder.cs
- TransformFinalBlockRequest.cs
- TextWriterEngine.cs
- Geometry.cs
- ProcessHostServerConfig.cs
- FontFamily.cs
- TypeElement.cs
- ModelServiceImpl.cs
- DbConnectionFactory.cs
- PEFileReader.cs
- SkipStoryboardToFill.cs
- CacheRequest.cs
- BamlLocalizableResourceKey.cs
- KeyMatchBuilder.cs
- ImageClickEventArgs.cs
- FixedStringLookup.cs
- ColorMatrix.cs
- DocumentReferenceCollection.cs