Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / System / Windows / Interop / ComponentDispatcherThread.cs / 1 / ComponentDispatcherThread.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using MS.Win32; using System.Globalization; using System.Security; using System.Security.Permissions; using MS.Internal.WindowsBase; namespace System.Windows.Interop { ////// This is a class used to implement per-thread instance of the ComponentDispatcher. /// internal class ComponentDispatcherThread { ////// Returns true if one or more components has gone modal. /// Although once one component is modal a 2nd shouldn't. /// public bool IsThreadModal { get { return (_modalCount > 0); } } ////// Returns "current" message. More exactly the last MSG Raised. /// ////// Critical: This is blocked off as defense in depth to avoid leaking message information /// public MSG CurrentKeyboardMessage { [SecurityCritical] get { return _currentKeyboardMSG; } [SecurityCritical] set { _currentKeyboardMSG = value; } } ////// A component calls this to go modal. Current thread wide only. /// ////// Critical: This is blocked off as defense in depth /// [SecurityCritical] public void PushModal() { _modalCount += 1; if(1 == _modalCount) { if(null != _enterThreadModal) _enterThreadModal(null, EventArgs.Empty); } } ////// A component calls this to end being modal. /// ////// Critical: This is blocked off as defense in depth to avoid tampering with input /// [SecurityCritical] public void PopModal() { _modalCount -= 1; if(0 == _modalCount) { if(null != _leaveThreadModal) _leaveThreadModal(null, EventArgs.Empty); } if(_modalCount < 0) _modalCount = 0; // Throwing is also good } ////// The message loop pumper calls this when it is time to do idle processing. /// ////// Critical: This is blocked off as defense in depth /// [SecurityCritical] public void RaiseIdle() { if(null != _threadIdle) _threadIdle(null, EventArgs.Empty); } ////// The message loop pumper calls this for every keyboard message. /// ////// Critical: This is blocked off as defense in depth to prevent message leakage /// [SecurityCritical] public bool RaiseThreadMessage(ref MSG msg) { bool handled=false; if(null != _threadFilterMessage) _threadFilterMessage(ref msg, ref handled); if(handled) return handled; if(null != _threadPreprocessMessage) _threadPreprocessMessage(ref msg, ref handled); return handled; } ////// Components register delegates with this event to handle /// thread idle processing. /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event EventHandler ThreadIdle { [SecurityCritical] add { _threadIdle += value; } [SecurityCritical] remove { _threadIdle -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [SecurityCritical] private event EventHandler _threadIdle; ////// Components register delegates with this event to handle /// Keyboard Messages (first chance processing). /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event ThreadMessageEventHandler ThreadFilterMessage { [SecurityCritical] add { _threadFilterMessage += value; } [SecurityCritical] remove { _threadFilterMessage -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [SecurityCritical] private event ThreadMessageEventHandler _threadFilterMessage; ////// Components register delegates with this event to handle /// Keyboard Messages (second chance processing). /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event ThreadMessageEventHandler ThreadPreprocessMessage { [SecurityCritical] add { _threadPreprocessMessage += value; } [SecurityCritical] remove { _threadPreprocessMessage -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [SecurityCritical] private event ThreadMessageEventHandler _threadPreprocessMessage; ////// Components register delegates with this event to handle /// a component on this thread has "gone modal", when previously none were. /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event EventHandler EnterThreadModal { [SecurityCritical] add { _enterThreadModal += value; } [SecurityCritical] remove { _enterThreadModal -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [SecurityCritical] private event EventHandler _enterThreadModal; ////// Components register delegates with this event to handle /// all components on this thread are done being modal. /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event EventHandler LeaveThreadModal { [SecurityCritical] add { _leaveThreadModal += value; } [SecurityCritical] remove { _leaveThreadModal -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [SecurityCritical] private event EventHandler _leaveThreadModal; private int _modalCount; ////// Critical: This holds the last message that was recieved /// [SecurityCritical] private MSG _currentKeyboardMSG; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
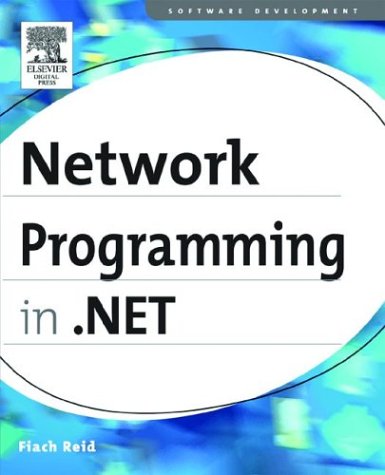
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MissingManifestResourceException.cs
- ProfilePropertyNameValidator.cs
- ConnectionStringSettingsCollection.cs
- CardSpacePolicyElement.cs
- ColorConvertedBitmapExtension.cs
- IUnknownConstantAttribute.cs
- SqlHelper.cs
- MultipleViewProviderWrapper.cs
- CqlGenerator.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- BuildProvidersCompiler.cs
- WebPartDescription.cs
- GenericTextProperties.cs
- ToolStripDropDown.cs
- XmlNodeReader.cs
- XmlWrappingReader.cs
- Crc32Helper.cs
- UpdateCommandGenerator.cs
- DataGridViewRowsRemovedEventArgs.cs
- DataControlField.cs
- ScrollContentPresenter.cs
- ResourceExpressionEditorSheet.cs
- LocatorPartList.cs
- ObjectToken.cs
- RayHitTestParameters.cs
- PackageFilter.cs
- WebBrowserEvent.cs
- GcHandle.cs
- ListViewItem.cs
- AncestorChangedEventArgs.cs
- AppDomainAttributes.cs
- TypeCodeDomSerializer.cs
- WorkflowTimerService.cs
- MeasurementDCInfo.cs
- CustomAttributeFormatException.cs
- TrackingMemoryStream.cs
- StyleCollection.cs
- BoundColumn.cs
- GridEntry.cs
- DependencyPropertyKind.cs
- MetadataCacheItem.cs
- TypeGeneratedEventArgs.cs
- ObjectViewListener.cs
- CommentEmitter.cs
- AsnEncodedData.cs
- SharedStatics.cs
- FormViewUpdateEventArgs.cs
- ClientSideQueueItem.cs
- XmlNodeChangedEventArgs.cs
- PolygonHotSpot.cs
- TemplateBamlTreeBuilder.cs
- AsyncStreamReader.cs
- MultiTargetingUtil.cs
- DrawingContextWalker.cs
- Metadata.cs
- XmlWriter.cs
- DecoderBestFitFallback.cs
- DirectoryNotFoundException.cs
- ExitEventArgs.cs
- ApplicationContext.cs
- TimeStampChecker.cs
- InvokeMemberBinder.cs
- FrameworkElementFactory.cs
- ScrollViewer.cs
- BuildProviderCollection.cs
- StringTraceRecord.cs
- LabelDesigner.cs
- WpfPayload.cs
- ProjectionCamera.cs
- Message.cs
- XmlUrlResolver.cs
- _Events.cs
- RawAppCommandInputReport.cs
- XmlSchemaFacet.cs
- RuleValidation.cs
- ArraySet.cs
- AppDomain.cs
- XMLSyntaxException.cs
- PropertyReferenceSerializer.cs
- CommandBinding.cs
- EnumBuilder.cs
- RowToFieldTransformer.cs
- CodeMemberEvent.cs
- InputScopeNameConverter.cs
- ConfigurationStrings.cs
- SettingsPropertyValue.cs
- OdbcParameter.cs
- PkcsUtils.cs
- ValueTypeFixupInfo.cs
- BaseTreeIterator.cs
- TypeConverterAttribute.cs
- BindableTemplateBuilder.cs
- TextEditorTyping.cs
- WebPart.cs
- TextEffectResolver.cs
- FieldAccessException.cs
- HandlerFactoryCache.cs
- MultiSelectRootGridEntry.cs
- LabelDesigner.cs
- DaylightTime.cs