Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / DrawingContextWalker.cs / 1305600 / DrawingContextWalker.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Contains base class for DrawingContext iterators // // History: // // 2004/04/02 : [....] - Created it. // 2005/06/20 : timothyc - Moved to codegen // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Imaging; using System.Windows.Media.Media3D; using MS.Internal; namespace System.Windows.Media { ////// DrawingContextWalker : The base class for DrawingContext iterators. /// This is *not* thread safe /// internal abstract partial class DrawingContextWalker : DrawingContext { ////// Constructor for DrawingContextWalker /// protected DrawingContextWalker() { // Nothing to do here } ////// DrawingContextWalker implementations are never opened, so they shouldn't be closed. /// public override sealed void Close() { Debug.Assert(false); } #region Protected methods ////// DrawingContextWalker implementations are never opened, so they shouldn't be disposed. /// protected override void DisposeCore() { Debug.Assert(false); } ////// StopWalking - If this called, the current walk will stop. /// protected void StopWalking() { _stopWalking = true; } #endregion Protected methods #region Internal properties ////// ShouldStopWalking Property - internal clients can consult this property to determine /// whether or not the implementer of this DrawingContextWalker has called StopWalking. /// This can also be set by internal callers. /// internal bool ShouldStopWalking { get { return _stopWalking; } set { _stopWalking = value; } } #endregion Internal properties #region Private Members private bool _stopWalking; #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
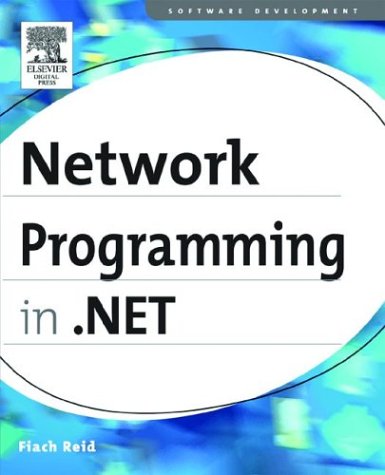
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeNamespaceImportCollection.cs
- Model3D.cs
- BamlRecordReader.cs
- BinaryQueryOperator.cs
- EdmSchemaAttribute.cs
- RequestCachingSection.cs
- CaseStatement.cs
- HMAC.cs
- ISAPIRuntime.cs
- DataGridSortingEventArgs.cs
- BinaryMessageEncodingElement.cs
- TransactionFormatter.cs
- ArraySortHelper.cs
- WinInet.cs
- StylusEditingBehavior.cs
- AttachedAnnotationChangedEventArgs.cs
- SessionIDManager.cs
- DecimalAnimationUsingKeyFrames.cs
- ObjectListField.cs
- GeometryValueSerializer.cs
- DataGridItemEventArgs.cs
- SystemWebCachingSectionGroup.cs
- FileDialog_Vista_Interop.cs
- FreezableOperations.cs
- ProcessHostServerConfig.cs
- Point3DCollectionConverter.cs
- BackgroundFormatInfo.cs
- BufferBuilder.cs
- ReadOnlyNameValueCollection.cs
- InputMethodStateChangeEventArgs.cs
- WebPartTransformerAttribute.cs
- PasswordDeriveBytes.cs
- ThemeableAttribute.cs
- FilterableData.cs
- SqlProfileProvider.cs
- IdentitySection.cs
- Debug.cs
- PasswordTextContainer.cs
- PathFigureCollectionValueSerializer.cs
- SplashScreen.cs
- Command.cs
- Win32MouseDevice.cs
- NetworkStream.cs
- ItemsControlAutomationPeer.cs
- ProcessThread.cs
- RelAssertionDirectKeyIdentifierClause.cs
- Char.cs
- RemotingServices.cs
- EntityDataSourceViewSchema.cs
- SystemIcmpV4Statistics.cs
- AutomationProperties.cs
- Invariant.cs
- FamilyMap.cs
- validationstate.cs
- SplashScreen.cs
- TabControl.cs
- ProcessInfo.cs
- XmlDataSource.cs
- CodeComment.cs
- AttributeProviderAttribute.cs
- WsatConfiguration.cs
- ByteAnimation.cs
- ProcessModuleCollection.cs
- TripleDES.cs
- TreeNodeSelectionProcessor.cs
- WizardStepBase.cs
- FactoryRecord.cs
- BindingsSection.cs
- DataRowComparer.cs
- PolyQuadraticBezierSegment.cs
- DispatcherObject.cs
- DigitalSignature.cs
- MemberHolder.cs
- WindowsScroll.cs
- Italic.cs
- MarkupCompilePass1.cs
- MetadataStore.cs
- TextEndOfLine.cs
- ConnectionStringsSection.cs
- Message.cs
- MarkupWriter.cs
- AddInBase.cs
- ObjectPersistData.cs
- RangeValidator.cs
- PeerContact.cs
- MediaCommands.cs
- XsltLibrary.cs
- XmlQueryContext.cs
- ScriptingProfileServiceSection.cs
- FileIOPermission.cs
- InternalDuplexChannelListener.cs
- LambdaCompiler.Unary.cs
- EventProvider.cs
- DescriptionCreator.cs
- CompiledIdentityConstraint.cs
- ClientScriptManagerWrapper.cs
- ChannelTokenTypeConverter.cs
- ToolBar.cs
- CellTreeNode.cs
- TreeNodeCollection.cs