Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Tools / comsvcutil / WasAdminWrapper.cs / 1305376 / WasAdminWrapper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.Tools.ServiceModel.ComSvcConfig { using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.DirectoryServices; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.IO; using System.Reflection; using System.Text; using System.Threading; using System.Runtime.InteropServices; using System.Security; using System.ServiceModel; using System.ServiceModel.Configuration; using Microsoft.Tools.ServiceModel; using Microsoft.Tools.ServiceModel.SvcUtil; internal static class WasAdminWrapper { const string webService = "IIS://localhost/w3svc"; const string defaultWebServer = "1"; public static string DefaultWebServer { get { return webService + "/" + defaultWebServer; } } public static bool IsIISInstalled () { try { DirectoryEntry webServiceEntry = new DirectoryEntry(webService); foreach (DirectoryEntry child in webServiceEntry.Children) return true; } catch (COMException) { return false; } return true; } public static string[] GetWebServerNames() { if (!IsIISInstalled ()) return null; try { ListwebServerNames = new List (); DirectoryEntry webServiceEntry = new DirectoryEntry(webService); foreach (DirectoryEntry child in webServiceEntry.Children) { if (child.SchemaClassName.ToUpperInvariant() == "IISWEBSERVER") { webServerNames.Add(webService + "/" + child.Name); // Note, child.Name is a number! the "friendly" name is actually child.Description } } return webServerNames.ToArray(); } catch (COMException ex) { // assume a failure here means that no web servers exist ToolConsole.WriteWarning (SR.GetString(SR.CannotGetWebServersIgnoringWas, ex.ErrorCode, ex.Message)); return null; } } public static string[] GetWebDirectoryNames(string webServer) { if (!IsIISInstalled ()) return null; try { List webDirectoryNames = new List (); DirectoryEntry webServiceEntry = new DirectoryEntry(webServer); foreach (DirectoryEntry child in webServiceEntry.Children) { if (child.SchemaClassName.ToUpperInvariant () == "IISWEBDIRECTORY" || child.SchemaClassName.ToUpperInvariant() == "IISWEBVIRTUALDIR" ) { webDirectoryNames.Add(child.Name); // Must special case the "ROOT" vDir, since most actual vDirs are subchildren of the ROOT vdir of a server. if (child.Name.ToUpperInvariant() == "ROOT") { foreach (DirectoryEntry rootChild in child.Children) { if (rootChild.SchemaClassName.ToUpperInvariant() == "IISWEBDIRECTORY" || rootChild.SchemaClassName.ToUpperInvariant() == "IISWEBVIRTUALDIR") { webDirectoryNames.Add("ROOT" + "/" + rootChild.Name); } } } } } return webDirectoryNames.ToArray(); } catch (COMException ex) { // assume a failure here means that no web directory exist ToolConsole.WriteWarning (SR.GetString(SR.CannotGetWebDirectoryForServer, webServer, ex.ErrorCode, ex.Message)); return null; } } public static bool GetWebDirectoryPath(string webServer, string webDirectory, out string webDirectoryPath) { webDirectoryPath = null; if (!IsIISInstalled ()) return false; if (!webDirectory.ToUpperInvariant().StartsWith("ROOT", StringComparison.Ordinal)) webDirectory = "root/" + webDirectory; string [] webDirectories = GetWebDirectoryNames (webServer ); if (webDirectories == null) return false; bool found = false; foreach (string webDirectoryName in webDirectories ) if (webDirectoryName.ToUpperInvariant () == webDirectory.ToUpperInvariant()) { found = true; break; } if (!found ) return false; DirectoryEntry webDirectoryEntry = new DirectoryEntry(webServer + "/" + webDirectory); try { if (webDirectoryEntry.Properties.Contains("Path")) { webDirectoryPath = (string)webDirectoryEntry.Properties["Path"].Value; return true; } else { return false; } } catch (COMException ex) { // assume a failure here means the dir does not exist ToolConsole.WriteWarning (SR.GetString(SR.CannotGetWebDirectoryPathOnWebDirOfWebServIgnoring, webServer, webDirectory, ex.ErrorCode, ex.Message)); return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.Tools.ServiceModel.ComSvcConfig { using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.DirectoryServices; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.IO; using System.Reflection; using System.Text; using System.Threading; using System.Runtime.InteropServices; using System.Security; using System.ServiceModel; using System.ServiceModel.Configuration; using Microsoft.Tools.ServiceModel; using Microsoft.Tools.ServiceModel.SvcUtil; internal static class WasAdminWrapper { const string webService = "IIS://localhost/w3svc"; const string defaultWebServer = "1"; public static string DefaultWebServer { get { return webService + "/" + defaultWebServer; } } public static bool IsIISInstalled () { try { DirectoryEntry webServiceEntry = new DirectoryEntry(webService); foreach (DirectoryEntry child in webServiceEntry.Children) return true; } catch (COMException) { return false; } return true; } public static string[] GetWebServerNames() { if (!IsIISInstalled ()) return null; try { List webServerNames = new List (); DirectoryEntry webServiceEntry = new DirectoryEntry(webService); foreach (DirectoryEntry child in webServiceEntry.Children) { if (child.SchemaClassName.ToUpperInvariant() == "IISWEBSERVER") { webServerNames.Add(webService + "/" + child.Name); // Note, child.Name is a number! the "friendly" name is actually child.Description } } return webServerNames.ToArray(); } catch (COMException ex) { // assume a failure here means that no web servers exist ToolConsole.WriteWarning (SR.GetString(SR.CannotGetWebServersIgnoringWas, ex.ErrorCode, ex.Message)); return null; } } public static string[] GetWebDirectoryNames(string webServer) { if (!IsIISInstalled ()) return null; try { List webDirectoryNames = new List (); DirectoryEntry webServiceEntry = new DirectoryEntry(webServer); foreach (DirectoryEntry child in webServiceEntry.Children) { if (child.SchemaClassName.ToUpperInvariant () == "IISWEBDIRECTORY" || child.SchemaClassName.ToUpperInvariant() == "IISWEBVIRTUALDIR" ) { webDirectoryNames.Add(child.Name); // Must special case the "ROOT" vDir, since most actual vDirs are subchildren of the ROOT vdir of a server. if (child.Name.ToUpperInvariant() == "ROOT") { foreach (DirectoryEntry rootChild in child.Children) { if (rootChild.SchemaClassName.ToUpperInvariant() == "IISWEBDIRECTORY" || rootChild.SchemaClassName.ToUpperInvariant() == "IISWEBVIRTUALDIR") { webDirectoryNames.Add("ROOT" + "/" + rootChild.Name); } } } } } return webDirectoryNames.ToArray(); } catch (COMException ex) { // assume a failure here means that no web directory exist ToolConsole.WriteWarning (SR.GetString(SR.CannotGetWebDirectoryForServer, webServer, ex.ErrorCode, ex.Message)); return null; } } public static bool GetWebDirectoryPath(string webServer, string webDirectory, out string webDirectoryPath) { webDirectoryPath = null; if (!IsIISInstalled ()) return false; if (!webDirectory.ToUpperInvariant().StartsWith("ROOT", StringComparison.Ordinal)) webDirectory = "root/" + webDirectory; string [] webDirectories = GetWebDirectoryNames (webServer ); if (webDirectories == null) return false; bool found = false; foreach (string webDirectoryName in webDirectories ) if (webDirectoryName.ToUpperInvariant () == webDirectory.ToUpperInvariant()) { found = true; break; } if (!found ) return false; DirectoryEntry webDirectoryEntry = new DirectoryEntry(webServer + "/" + webDirectory); try { if (webDirectoryEntry.Properties.Contains("Path")) { webDirectoryPath = (string)webDirectoryEntry.Properties["Path"].Value; return true; } else { return false; } } catch (COMException ex) { // assume a failure here means the dir does not exist ToolConsole.WriteWarning (SR.GetString(SR.CannotGetWebDirectoryPathOnWebDirOfWebServIgnoring, webServer, webDirectory, ex.ErrorCode, ex.Message)); return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
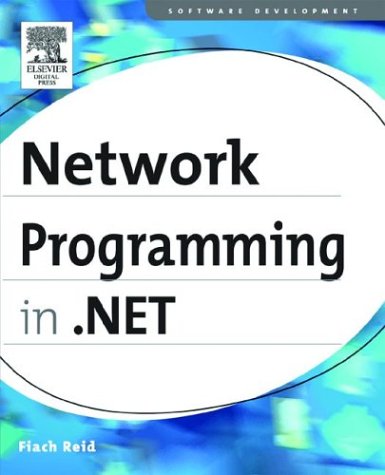
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WeakReference.cs
- AuthorizationContext.cs
- FilterEventArgs.cs
- ToolboxBitmapAttribute.cs
- CodeIterationStatement.cs
- ConstraintManager.cs
- AttachInfo.cs
- ControlParameter.cs
- DbDeleteCommandTree.cs
- Thickness.cs
- UserNameSecurityTokenAuthenticator.cs
- TableLayoutPanel.cs
- DeobfuscatingStream.cs
- SQLDoubleStorage.cs
- FieldTemplateUserControl.cs
- MetadataArtifactLoader.cs
- EdgeProfileValidation.cs
- CodeTypeDelegate.cs
- ListBindingHelper.cs
- WorkflowControlEndpoint.cs
- FixedTextContainer.cs
- StateBag.cs
- GetLastErrorDetailsRequest.cs
- BooleanExpr.cs
- SqlDataSourceConnectionPanel.cs
- ErrorTableItemStyle.cs
- HitTestDrawingContextWalker.cs
- DataGridViewCellValueEventArgs.cs
- HttpModulesSection.cs
- UnsafeNativeMethodsCLR.cs
- PropertyAccessVisitor.cs
- PageAdapter.cs
- RequestCacheValidator.cs
- SectionInformation.cs
- TextBoxLine.cs
- DesignerSelectionListAdapter.cs
- DurableInstanceProvider.cs
- ObjectListCommand.cs
- CompositionCommandSet.cs
- SimpleType.cs
- BitmapMetadataEnumerator.cs
- IsolationInterop.cs
- QueryAsyncResult.cs
- returneventsaver.cs
- DataSpaceManager.cs
- NetSectionGroup.cs
- GridViewEditEventArgs.cs
- LocationSectionRecord.cs
- ExceptionHandlers.cs
- SerializationTrace.cs
- ListViewItem.cs
- PointKeyFrameCollection.cs
- recordstatescratchpad.cs
- WrapperEqualityComparer.cs
- JsonDataContract.cs
- WebConfigurationFileMap.cs
- ProtocolState.cs
- StrongNameMembershipCondition.cs
- DataObjectCopyingEventArgs.cs
- NativeMethods.cs
- PrintDialog.cs
- __FastResourceComparer.cs
- MappingItemCollection.cs
- XmlILIndex.cs
- ConfigurationElementCollection.cs
- CaseInsensitiveHashCodeProvider.cs
- SaveFileDialog.cs
- MetafileHeaderWmf.cs
- ObjectRef.cs
- RemoteHelper.cs
- TextEffectResolver.cs
- OpCodes.cs
- InputScopeConverter.cs
- RepeaterCommandEventArgs.cs
- ExpandCollapseIsCheckedConverter.cs
- SecurityDescriptor.cs
- ExternalFile.cs
- EditorPartChrome.cs
- TableCell.cs
- HtmlShimManager.cs
- SimpleBitVector32.cs
- OutputCacheProfileCollection.cs
- PersonalizationStateQuery.cs
- ACE.cs
- TopClause.cs
- CharacterBuffer.cs
- HttpHeaderCollection.cs
- UpdateTranslator.cs
- VisualBrush.cs
- TrackingDataItemValue.cs
- EventHandlerList.cs
- FloatSumAggregationOperator.cs
- EnumerableRowCollectionExtensions.cs
- wgx_render.cs
- PathFigureCollectionValueSerializer.cs
- HttpClientCertificate.cs
- EventRoute.cs
- ExitEventArgs.cs
- XPathNodeList.cs
- ProjectedSlot.cs