Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Configuration / TransformerInfoCollection.cs / 1 / TransformerInfoCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Security.Principal; using System.Web; using System.Web.Compilation; using System.Web.Configuration; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.Util; using System.Xml; using System.Security.Permissions; [ConfigurationCollection(typeof(TransformerInfo))] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TransformerInfoCollection : ConfigurationElementCollection { private static ConfigurationPropertyCollection _properties; private Hashtable _transformerEntries; static TransformerInfoCollection() { _properties = new ConfigurationPropertyCollection(); } ///protected override ConfigurationPropertyCollection Properties { get { return _properties; } } public TransformerInfo this[int index] { get { return (TransformerInfo)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index, value); } } public void Add(TransformerInfo transformerInfo) { BaseAdd(transformerInfo); } public void Clear() { BaseClear(); } /// protected override ConfigurationElement CreateNewElement() { return new TransformerInfo(); } public void Remove(string s) { BaseRemove(s); } public void RemoveAt(int index) { BaseRemoveAt(index); } /// protected override object GetElementKey(ConfigurationElement element) { return ((TransformerInfo)element).Name; } internal Hashtable GetTransformerEntries() { if (_transformerEntries == null) { lock (this) { if (_transformerEntries == null) { _transformerEntries = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach (TransformerInfo ti in this) { Type transformerType = ConfigUtil.GetType(ti.Type, "type", ti); if (transformerType.IsSubclassOf(typeof(WebPartTransformer)) == false) { throw new ConfigurationErrorsException( SR.GetString( SR.Type_doesnt_inherit_from_type, ti.Type, typeof(WebPartTransformer).FullName), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } Type consumerType; Type providerType; try { consumerType = WebPartTransformerAttribute.GetConsumerType(transformerType); providerType = WebPartTransformerAttribute.GetProviderType(transformerType); } catch (Exception e) { throw new ConfigurationErrorsException( SR.GetString(SR.Transformer_attribute_error, e.Message), e, ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } if (_transformerEntries.Count != 0) { foreach (DictionaryEntry entry in _transformerEntries) { Type existingTransformerType = (Type)entry.Value; // We know these methods will not throw, because for the type to be in the transformers // collection, we must have successfully gotten the types previously without an exception. Type existingConsumerType = WebPartTransformerAttribute.GetConsumerType(existingTransformerType); Type existingProviderType = WebPartTransformerAttribute.GetProviderType(existingTransformerType); if ((consumerType == existingConsumerType) && (providerType == existingProviderType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Transformer_types_already_added, (string)entry.Key, ti.Name), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } } } _transformerEntries[ti.Name] = transformerType; } } } } // return _transformerEntries; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Security.Principal; using System.Web; using System.Web.Compilation; using System.Web.Configuration; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.Util; using System.Xml; using System.Security.Permissions; [ConfigurationCollection(typeof(TransformerInfo))] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TransformerInfoCollection : ConfigurationElementCollection { private static ConfigurationPropertyCollection _properties; private Hashtable _transformerEntries; static TransformerInfoCollection() { _properties = new ConfigurationPropertyCollection(); } ///protected override ConfigurationPropertyCollection Properties { get { return _properties; } } public TransformerInfo this[int index] { get { return (TransformerInfo)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index, value); } } public void Add(TransformerInfo transformerInfo) { BaseAdd(transformerInfo); } public void Clear() { BaseClear(); } /// protected override ConfigurationElement CreateNewElement() { return new TransformerInfo(); } public void Remove(string s) { BaseRemove(s); } public void RemoveAt(int index) { BaseRemoveAt(index); } /// protected override object GetElementKey(ConfigurationElement element) { return ((TransformerInfo)element).Name; } internal Hashtable GetTransformerEntries() { if (_transformerEntries == null) { lock (this) { if (_transformerEntries == null) { _transformerEntries = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach (TransformerInfo ti in this) { Type transformerType = ConfigUtil.GetType(ti.Type, "type", ti); if (transformerType.IsSubclassOf(typeof(WebPartTransformer)) == false) { throw new ConfigurationErrorsException( SR.GetString( SR.Type_doesnt_inherit_from_type, ti.Type, typeof(WebPartTransformer).FullName), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } Type consumerType; Type providerType; try { consumerType = WebPartTransformerAttribute.GetConsumerType(transformerType); providerType = WebPartTransformerAttribute.GetProviderType(transformerType); } catch (Exception e) { throw new ConfigurationErrorsException( SR.GetString(SR.Transformer_attribute_error, e.Message), e, ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } if (_transformerEntries.Count != 0) { foreach (DictionaryEntry entry in _transformerEntries) { Type existingTransformerType = (Type)entry.Value; // We know these methods will not throw, because for the type to be in the transformers // collection, we must have successfully gotten the types previously without an exception. Type existingConsumerType = WebPartTransformerAttribute.GetConsumerType(existingTransformerType); Type existingProviderType = WebPartTransformerAttribute.GetProviderType(existingTransformerType); if ((consumerType == existingConsumerType) && (providerType == existingProviderType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Transformer_types_already_added, (string)entry.Key, ti.Name), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } } } _transformerEntries[ti.Name] = transformerType; } } } } // return _transformerEntries; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
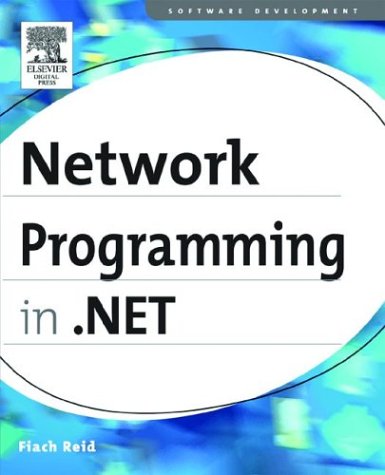
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPageTraceListener.cs
- ColumnResizeUndoUnit.cs
- ScriptComponentDescriptor.cs
- ISAPIRuntime.cs
- DataGridToolTip.cs
- HitTestParameters.cs
- TableLayoutSettingsTypeConverter.cs
- NetCodeGroup.cs
- SelectedDatesCollection.cs
- DocumentViewerHelper.cs
- XmlSchemaExporter.cs
- RolePrincipal.cs
- DataGridColumnDropSeparator.cs
- GenericWebPart.cs
- COM2ColorConverter.cs
- SecondaryIndexDefinition.cs
- StickyNote.cs
- Selector.cs
- Utils.cs
- OleDbParameter.cs
- PeerInvitationResponse.cs
- RegexTypeEditor.cs
- GeometryDrawing.cs
- OAVariantLib.cs
- SynchronizationLockException.cs
- IntellisenseTextBox.cs
- DocumentViewerBaseAutomationPeer.cs
- OdbcUtils.cs
- Transactions.cs
- SignedPkcs7.cs
- ExtendedPropertyInfo.cs
- unsafenativemethodsother.cs
- FastEncoder.cs
- TextPointerBase.cs
- ConsoleKeyInfo.cs
- EntityDataSourceView.cs
- PackageController.cs
- LowerCaseStringConverter.cs
- StylusCollection.cs
- _SSPISessionCache.cs
- SecurityUtils.cs
- GradientBrush.cs
- MultilineStringConverter.cs
- TextParaClient.cs
- ContractMapping.cs
- _ChunkParse.cs
- Operand.cs
- AppDomainGrammarProxy.cs
- ManipulationDelta.cs
- FileUtil.cs
- ImageFormatConverter.cs
- RowType.cs
- DataGridCommandEventArgs.cs
- HwndStylusInputProvider.cs
- DataViewSetting.cs
- HashCryptoHandle.cs
- Ticks.cs
- CommandHelper.cs
- SqlParameterizer.cs
- ConfigurationProperty.cs
- DataBoundLiteralControl.cs
- BooleanStorage.cs
- DecimalAnimation.cs
- DataGridViewCellStateChangedEventArgs.cs
- infer.cs
- SendActivityValidator.cs
- ContextMenuService.cs
- ThreadInterruptedException.cs
- EntityDataSourceDataSelection.cs
- DataKey.cs
- RelationshipEndCollection.cs
- ErrorFormatter.cs
- HostTimeoutsElement.cs
- IDReferencePropertyAttribute.cs
- HtmlElementEventArgs.cs
- DesignColumnCollection.cs
- LinqDataSourceDisposeEventArgs.cs
- GregorianCalendarHelper.cs
- GridViewRowEventArgs.cs
- ClientSettingsStore.cs
- ControlDesigner.cs
- MarkupCompiler.cs
- PolyQuadraticBezierSegment.cs
- ReflectionUtil.cs
- WebPartConnectionCollection.cs
- SqlDataSourceCommandParser.cs
- PointCollection.cs
- NetStream.cs
- PngBitmapDecoder.cs
- FrameworkTemplate.cs
- SqlDataSource.cs
- LayoutEditorPart.cs
- ProfessionalColors.cs
- SHA1.cs
- ClassicBorderDecorator.cs
- TimeoutException.cs
- DefaultPrintController.cs
- ComponentDispatcherThread.cs
- VisualBrush.cs
- XmlCharCheckingReader.cs