Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / Ticks.cs / 1 / Ticks.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- using System.Security; namespace System.ServiceModel.Channels { static class Ticks { public static long Now { ////// Critical - makes a native method call /// Safe - SafeNativeMethod /// [SecurityCritical, SecurityTreatAsSafe] get { long time; #pragma warning suppress 56523 // [....], function has no error return value SafeNativeMethods.GetSystemTimeAsFileTime(out time); return time; } } public static long FromMilliseconds(int milliseconds) { return checked ((long)milliseconds * TimeSpan.TicksPerMillisecond); } public static int ToMilliseconds(long ticks) { return checked((int) (ticks / TimeSpan.TicksPerMillisecond)); } public static long FromTimeSpan(TimeSpan duration) { return duration.Ticks; } public static TimeSpan ToTimeSpan(long ticks) { return new TimeSpan(ticks); } public static long Add(long firstTicks, long secondTicks) { if (firstTicks == long.MaxValue || firstTicks == long.MinValue) { return firstTicks; } if (secondTicks == long.MaxValue || secondTicks == long.MinValue) { return secondTicks; } if (firstTicks >= 0 && long.MaxValue - firstTicks <= secondTicks) { return long.MaxValue - 1; } if (firstTicks <= 0 && long.MinValue - firstTicks >= secondTicks) { return long.MinValue + 1; } return checked(firstTicks + secondTicks); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
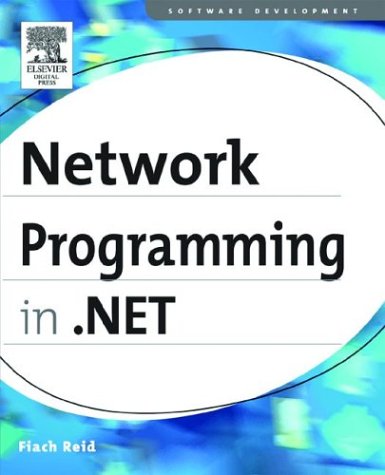
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MessageHeaderException.cs
- ExpandedWrapper.cs
- RightsManagementPermission.cs
- SqlSelectClauseBuilder.cs
- XPathDocumentNavigator.cs
- SchemaMapping.cs
- ExpressionBuilderCollection.cs
- XmlSignatureManifest.cs
- TypeUtils.cs
- IxmlLineInfo.cs
- EmptyEnumerable.cs
- TreeNodeStyle.cs
- DataTableNameHandler.cs
- CreatingCookieEventArgs.cs
- AuthenticationModulesSection.cs
- DataGridViewTopLeftHeaderCell.cs
- XmlSchemaAttribute.cs
- URLIdentityPermission.cs
- ModelItemCollection.cs
- BinaryUtilClasses.cs
- SqlDataSourceConfigureSelectPanel.cs
- ProfileBuildProvider.cs
- DigitShape.cs
- Nullable.cs
- RectAnimationUsingKeyFrames.cs
- Suspend.cs
- DefaultMergeHelper.cs
- Duration.cs
- ComponentEvent.cs
- EncryptedKey.cs
- Visual.cs
- SoapCodeExporter.cs
- PerformanceCounterPermissionEntry.cs
- FontFamily.cs
- ProcessThreadCollection.cs
- Region.cs
- SoapExtensionReflector.cs
- SessionSwitchEventArgs.cs
- Panel.cs
- MenuItemAutomationPeer.cs
- WmlLiteralTextAdapter.cs
- CompModSwitches.cs
- WpfWebRequestHelper.cs
- ComboBox.cs
- SemanticAnalyzer.cs
- DataBindEngine.cs
- ConfigXmlComment.cs
- ServiceParser.cs
- SmuggledIUnknown.cs
- BuildResultCache.cs
- ApplicationServiceHelper.cs
- ListViewUpdatedEventArgs.cs
- SpellerHighlightLayer.cs
- HwndAppCommandInputProvider.cs
- BStrWrapper.cs
- SafeNativeMethods.cs
- WorkflowServiceHostFactory.cs
- DefaultShape.cs
- RuleRefElement.cs
- PrintControllerWithStatusDialog.cs
- DataControlImageButton.cs
- base64Transforms.cs
- PassportAuthenticationModule.cs
- AddressHeaderCollection.cs
- ListBindableAttribute.cs
- ProxyWebPart.cs
- Int32KeyFrameCollection.cs
- ArglessEventHandlerProxy.cs
- ZipIOCentralDirectoryBlock.cs
- TextEditorCharacters.cs
- CheckBox.cs
- BooleanFunctions.cs
- RijndaelManagedTransform.cs
- CollectionViewGroupRoot.cs
- HandleCollector.cs
- InfoCardMasterKey.cs
- TreeViewHitTestInfo.cs
- CodeDomSerializationProvider.cs
- HtmlWindow.cs
- MembershipUser.cs
- FocusTracker.cs
- EditingScopeUndoUnit.cs
- EncryptedType.cs
- DataListItemCollection.cs
- PropertyRecord.cs
- WebPartEditorOkVerb.cs
- Matrix3DConverter.cs
- SystemResources.cs
- EdmComplexPropertyAttribute.cs
- RankException.cs
- MissingSatelliteAssemblyException.cs
- ConsoleTraceListener.cs
- DbConnectionOptions.cs
- Tuple.cs
- ScrollBar.cs
- ColumnHeaderConverter.cs
- SmtpTransport.cs
- WebPartConnection.cs
- LinkedResourceCollection.cs
- ViewCellRelation.cs