Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / Serialization / CodeDomSerializationProvider.cs / 1 / CodeDomSerializationProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design.Serialization { using System.Design; using System; using System.CodeDom; using System.CodeDom.Compiler; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Resources; using System.Runtime.Serialization; using System.Globalization; ////// /// This is the serialization provider for all code dom serializers. /// internal sealed class CodeDomSerializationProvider : IDesignerSerializationProvider { ////// Returns a code dom serializer /// private object GetCodeDomSerializer(IDesignerSerializationManager manager, object currentSerializer, Type objectType, Type serializerType) { // If this isn't a serializer type we recognize, do nothing. Also, if metadata specified // a custom serializer, then use it. if (currentSerializer != null) { return null; } // Null is a valid value that can be passed into GetSerializer. It indicates // that the value we need to serialize is null, in which case we handle it // through the PrimitiveCodeDomSerializer. // if (objectType == null) { return PrimitiveCodeDomSerializer.Default; } // Support for components. // if (typeof(IComponent).IsAssignableFrom(objectType)) { return ComponentCodeDomSerializer.Default; } // We special case enums. They do have instance descriptors, but we want // better looking code than the instance descriptor can provide for flags, // so we do it ourselves. // if (typeof(Enum).IsAssignableFrom(objectType)) { return EnumCodeDomSerializer.Default; } // We will provide a serializer for any intrinsic types. // if (objectType.IsPrimitive || objectType.IsEnum || objectType == typeof(string)) { return PrimitiveCodeDomSerializer.Default; } // And one for collections. // if (typeof(ICollection).IsAssignableFrom(objectType)) { return CollectionCodeDomSerializer.Default; } // And one for IContainer if (typeof(IContainer).IsAssignableFrom(objectType)) { return ContainerCodeDomSerializer.Default; } // And one for resources // if (typeof(ResourceManager).IsAssignableFrom(objectType)) { return ResourceCodeDomSerializer.Default; } // The default serializer can do any object including those with instance descriptors. return CodeDomSerializer.Default; } ////// Returns a code dom serializer for members /// private object GetMemberCodeDomSerializer(IDesignerSerializationManager manager, object currentSerializer, Type objectType, Type serializerType) { // Don't provide our serializer if someone else already had one if (currentSerializer != null) { return null; } if (typeof(PropertyDescriptor).IsAssignableFrom(objectType)) { return PropertyMemberCodeDomSerializer.Default; } if (typeof(EventDescriptor).IsAssignableFrom(objectType)) { return EventMemberCodeDomSerializer.Default; } return null; } ////// Returns a code dom serializer for types /// private object GetTypeCodeDomSerializer(IDesignerSerializationManager manager, object currentSerializer, Type objectType, Type serializerType) { // Don't provide our serializer if someone else already had one if (currentSerializer != null) { return null; } if (typeof(IComponent).IsAssignableFrom(objectType)) { return ComponentTypeCodeDomSerializer.Default; } return TypeCodeDomSerializer.Default; } ////// /// This will be called by the serialization manager when it /// is trying to locate a serialzer for an object type. /// If this serialization provider can provide a serializer /// that is of the correct type, it should return it. /// Otherwise, it should return null. /// object IDesignerSerializationProvider.GetSerializer(IDesignerSerializationManager manager, object currentSerializer, Type objectType, Type serializerType) { if (serializerType == typeof(CodeDomSerializer)) { return GetCodeDomSerializer(manager, currentSerializer, objectType, serializerType); } else if (serializerType == typeof(MemberCodeDomSerializer)) { return GetMemberCodeDomSerializer(manager, currentSerializer, objectType, serializerType); } else if (serializerType == typeof(TypeCodeDomSerializer)) { return GetTypeCodeDomSerializer(manager, currentSerializer, objectType, serializerType); } return null; // don't understand this type of serializer. } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
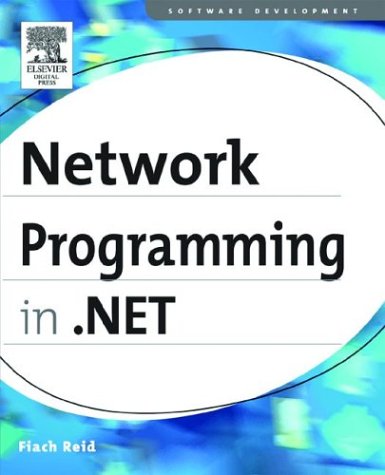
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmiEventStream.cs
- XmlRootAttribute.cs
- Types.cs
- DiagnosticTrace.cs
- XmlWriterTraceListener.cs
- dbdatarecord.cs
- TimeoutConverter.cs
- JapaneseCalendar.cs
- DesignerTransactionCloseEvent.cs
- ListViewGroupItemCollection.cs
- FullTrustAssembliesSection.cs
- DocumentationServerProtocol.cs
- entitydatasourceentitysetnameconverter.cs
- OptionalRstParameters.cs
- MailMessage.cs
- __Filters.cs
- XMLSchema.cs
- TargetInvocationException.cs
- HandleCollector.cs
- GradientBrush.cs
- CuspData.cs
- MSAAEventDispatcher.cs
- NativeMethods.cs
- CollectionEditorDialog.cs
- BoundPropertyEntry.cs
- ApplicationDirectory.cs
- FixedSOMPageConstructor.cs
- QilTypeChecker.cs
- SecurityCriticalDataForSet.cs
- ScriptResourceHandler.cs
- OutOfProcStateClientManager.cs
- Attributes.cs
- EntityStoreSchemaFilterEntry.cs
- TemplateEditingVerb.cs
- SafeHandles.cs
- CommandManager.cs
- DesignerDataParameter.cs
- Graphics.cs
- DataGridItemCollection.cs
- TerminatorSinks.cs
- HostingEnvironmentSection.cs
- ListBoxItemAutomationPeer.cs
- TriggerActionCollection.cs
- QueryCacheKey.cs
- Opcode.cs
- RbTree.cs
- CodeGotoStatement.cs
- SynchronizationScope.cs
- FormCollection.cs
- Package.cs
- CultureInfo.cs
- HashCoreRequest.cs
- TextTreeDeleteContentUndoUnit.cs
- ToolStripStatusLabel.cs
- WebPartVerb.cs
- XmlSchemaAny.cs
- SplitContainer.cs
- DocumentEventArgs.cs
- System.Data_BID.cs
- LambdaCompiler.Generated.cs
- JsonDataContract.cs
- SchemaImporterExtensionElementCollection.cs
- ImmComposition.cs
- DelayedRegex.cs
- HeaderLabel.cs
- DataRelationCollection.cs
- EditorServiceContext.cs
- DocumentPropertiesDialog.cs
- DBBindings.cs
- ConstructorArgumentAttribute.cs
- FileFormatException.cs
- RotationValidation.cs
- EdgeModeValidation.cs
- SqlMultiplexer.cs
- HTMLTagNameToTypeMapper.cs
- NativeWindow.cs
- VectorAnimationBase.cs
- CredentialManagerDialog.cs
- InstanceContextMode.cs
- AssemblyInfo.cs
- SystemUnicastIPAddressInformation.cs
- PrefixQName.cs
- StringAttributeCollection.cs
- DynamicQueryableWrapper.cs
- TemplateControl.cs
- AppSettingsExpressionBuilder.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- TranslateTransform.cs
- SecurityElement.cs
- QilGenerator.cs
- CodeEntryPointMethod.cs
- SiteMapHierarchicalDataSourceView.cs
- StaticSiteMapProvider.cs
- NumberFormatInfo.cs
- TextPenaltyModule.cs
- SystemIPGlobalProperties.cs
- BooleanAnimationUsingKeyFrames.cs
- QueuePropertyVariants.cs
- HashMembershipCondition.cs
- BindingGroup.cs