Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Configuration / System / Configuration / ConfigurationPropertyAttribute.cs / 1 / ConfigurationPropertyAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration.Internal; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.IO; using System.Reflection; using System.Security.Permissions; using System.Xml; using System.Globalization; using System.ComponentModel; using System.Security; using System.Text; namespace System.Configuration { [AttributeUsage(AttributeTargets.Property)] public sealed class ConfigurationPropertyAttribute : Attribute { internal static readonly String DefaultCollectionPropertyName = ""; private String _Name; private object _DefaultValue = ConfigurationElement.s_nullPropertyValue; private ConfigurationPropertyOptions _Flags = ConfigurationPropertyOptions.None; public ConfigurationPropertyAttribute(String name) { _Name = name; } public String Name { get { return _Name; } } public object DefaultValue { get { return _DefaultValue; } set { _DefaultValue = value; } } public ConfigurationPropertyOptions Options { get { return _Flags; } set { _Flags = value; } } public bool IsDefaultCollection { get { return ((Options & ConfigurationPropertyOptions.IsDefaultCollection) != 0); } set { if (value == true) { Options |= ConfigurationPropertyOptions.IsDefaultCollection; } else Options &= ~ConfigurationPropertyOptions.IsDefaultCollection; } } public bool IsRequired { get { return ((Options & ConfigurationPropertyOptions.IsRequired) != 0); } set { if (value == true) { Options |= ConfigurationPropertyOptions.IsRequired; } else { Options &= ~ConfigurationPropertyOptions.IsRequired; } } } public bool IsKey { get { return ((Options & ConfigurationPropertyOptions.IsKey) != 0); } set { if (value == true) { Options |= ConfigurationPropertyOptions.IsKey; } else { Options &= ~ConfigurationPropertyOptions.IsKey; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration.Internal; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.IO; using System.Reflection; using System.Security.Permissions; using System.Xml; using System.Globalization; using System.ComponentModel; using System.Security; using System.Text; namespace System.Configuration { [AttributeUsage(AttributeTargets.Property)] public sealed class ConfigurationPropertyAttribute : Attribute { internal static readonly String DefaultCollectionPropertyName = ""; private String _Name; private object _DefaultValue = ConfigurationElement.s_nullPropertyValue; private ConfigurationPropertyOptions _Flags = ConfigurationPropertyOptions.None; public ConfigurationPropertyAttribute(String name) { _Name = name; } public String Name { get { return _Name; } } public object DefaultValue { get { return _DefaultValue; } set { _DefaultValue = value; } } public ConfigurationPropertyOptions Options { get { return _Flags; } set { _Flags = value; } } public bool IsDefaultCollection { get { return ((Options & ConfigurationPropertyOptions.IsDefaultCollection) != 0); } set { if (value == true) { Options |= ConfigurationPropertyOptions.IsDefaultCollection; } else Options &= ~ConfigurationPropertyOptions.IsDefaultCollection; } } public bool IsRequired { get { return ((Options & ConfigurationPropertyOptions.IsRequired) != 0); } set { if (value == true) { Options |= ConfigurationPropertyOptions.IsRequired; } else { Options &= ~ConfigurationPropertyOptions.IsRequired; } } } public bool IsKey { get { return ((Options & ConfigurationPropertyOptions.IsKey) != 0); } set { if (value == true) { Options |= ConfigurationPropertyOptions.IsKey; } else { Options &= ~ConfigurationPropertyOptions.IsKey; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
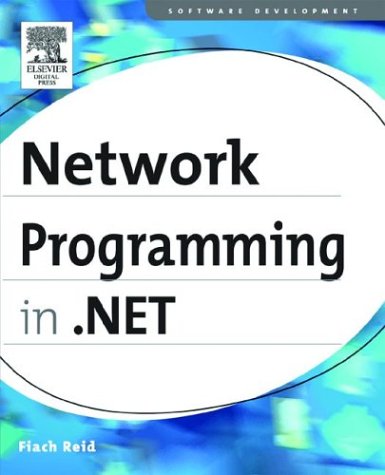
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeEventReferenceExpression.cs
- DbCommandDefinition.cs
- Help.cs
- AdCreatedEventArgs.cs
- XmlSchemaAppInfo.cs
- CustomValidator.cs
- XXXInfos.cs
- SourceInterpreter.cs
- StylusButtonCollection.cs
- Base64Encoding.cs
- CodeAttributeArgument.cs
- BindingWorker.cs
- ServiceModelSecurityTokenRequirement.cs
- CriticalExceptions.cs
- EditorAttribute.cs
- BitSet.cs
- RequestCache.cs
- ConfigurationElementProperty.cs
- CssStyleCollection.cs
- AnnotationMap.cs
- ThreadAttributes.cs
- EffectiveValueEntry.cs
- ImageUrlEditor.cs
- ScriptingWebServicesSectionGroup.cs
- ExpressionBindingCollection.cs
- GifBitmapEncoder.cs
- HttpApplicationFactory.cs
- BulletedList.cs
- BindingMemberInfo.cs
- DocobjHost.cs
- ThicknessAnimation.cs
- EditorZoneBase.cs
- SoapInteropTypes.cs
- CodeArrayCreateExpression.cs
- QueryableFilterRepeater.cs
- FileIOPermission.cs
- Padding.cs
- TextTrailingWordEllipsis.cs
- XmlDsigSep2000.cs
- Sequence.cs
- Scripts.cs
- TriggerBase.cs
- PolicyValidationException.cs
- ParsedAttributeCollection.cs
- InspectionWorker.cs
- WindowsScrollBarBits.cs
- HtmlShim.cs
- ActivationArguments.cs
- ProviderConnectionPointCollection.cs
- DbProviderSpecificTypePropertyAttribute.cs
- AuthenticationService.cs
- TreeWalkHelper.cs
- WebScriptEndpoint.cs
- BitmapEffectGeneralTransform.cs
- xmlglyphRunInfo.cs
- PathFigureCollection.cs
- ResolveDuplexCD1AsyncResult.cs
- TreeNodeStyleCollection.cs
- Tag.cs
- ListControl.cs
- TemporaryBitmapFile.cs
- SourceFilter.cs
- DataTablePropertyDescriptor.cs
- TextSerializer.cs
- SecureConversationSecurityTokenParameters.cs
- SystemDiagnosticsSection.cs
- DispatchWrapper.cs
- Util.cs
- SizeLimitedCache.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- IndexedString.cs
- EditorZone.cs
- DPTypeDescriptorContext.cs
- DataGridViewCellStyleConverter.cs
- KerberosReceiverSecurityToken.cs
- XmlAggregates.cs
- AdornerPresentationContext.cs
- BinaryQueryOperator.cs
- CharConverter.cs
- ValueChangedEventManager.cs
- nulltextcontainer.cs
- ListView.cs
- DataTableCollection.cs
- QuaternionAnimation.cs
- Events.cs
- ExpressionBindingCollection.cs
- FlowDocumentPage.cs
- DataColumnChangeEvent.cs
- XmlSerializationGeneratedCode.cs
- WebResourceUtil.cs
- BlockUIContainer.cs
- PartitionResolver.cs
- IDQuery.cs
- FixedSOMPage.cs
- DocumentViewer.cs
- XmlNodeChangedEventManager.cs
- XamlWrappingReader.cs
- ChannelSinkStacks.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- SupportsEventValidationAttribute.cs