Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / nulltextcontainer.cs / 1 / nulltextcontainer.cs
//---------------------------------------------------------------------------- //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // Description: // NullTextContainer is an immutable empty TextContainer that contains // single collapsed Start/End position. This is primarily used internally // so parameter check is mostly replaced with debug assert. // // History: // 07/14/2004 - Zhenbin Xu (ZhenbinX) - Created. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Diagnostics; using System.Windows.Threading; using System.Windows; // DependencyID etc. using MS.Internal.Documents; using MS.Internal; //===================================================================== ////// NullTextContainer is an immutable empty TextContainer that contains /// single collapsed Start/End position. This is primarily used internally /// so parameter check is mostly replaced with debug assert. /// internal sealed class NullTextContainer : ITextContainer { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors internal NullTextContainer() { _start = new NullTextPointer(this, LogicalDirection.Backward); _end = new NullTextPointer(this, LogicalDirection.Forward); } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods // // This is readonly Text OM. All modification methods returns false // void ITextContainer.BeginChange() { } ////// void ITextContainer.BeginChangeNoUndo() { // We don't support undo, so follow the BeginChange codepath. ((ITextContainer)this).BeginChange(); } ////// /// void ITextContainer.EndChange() { ((ITextContainer)this).EndChange(false /* skipEvents */); } ////// void ITextContainer.EndChange(bool skipEvents) { } // Allocate a new ITextPointer at the specified offset. // Equivalent to this.Start.CreatePointer(offset), but does not // necessarily allocate this.Start. ITextPointer ITextContainer.CreatePointerAtOffset(int offset, LogicalDirection direction) { return ((ITextContainer)this).Start.CreatePointer(offset, direction); } // Not Implemented. ITextPointer ITextContainer.CreatePointerAtCharOffset(int charOffset, LogicalDirection direction) { throw new NotImplementedException(); } ITextPointer ITextContainer.CreateDynamicTextPointer(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).CreatePointer(direction); } StaticTextPointer ITextContainer.CreateStaticPointerAtOffset(int offset) { return new StaticTextPointer(this, ((ITextContainer)this).CreatePointerAtOffset(offset, LogicalDirection.Forward)); } TextPointerContext ITextContainer.GetPointerContext(StaticTextPointer pointer, LogicalDirection direction) { return ((ITextPointer)pointer.Handle0).GetPointerContext(direction); } int ITextContainer.GetOffsetToPosition(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).GetOffsetToPosition((ITextPointer)position2.Handle0); } int ITextContainer.GetTextInRun(StaticTextPointer position, LogicalDirection direction, char[] textBuffer, int startIndex, int count) { return ((ITextPointer)position.Handle0).GetTextInRun(direction, textBuffer, startIndex, count); } object ITextContainer.GetAdjacentElement(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).GetAdjacentElement(direction); } DependencyObject ITextContainer.GetParent(StaticTextPointer position) { return null; } StaticTextPointer ITextContainer.CreatePointer(StaticTextPointer position, int offset) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).CreatePointer(offset)); } StaticTextPointer ITextContainer.GetNextContextPosition(StaticTextPointer position, LogicalDirection direction) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).GetNextContextPosition(direction)); } int ITextContainer.CompareTo(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo((ITextPointer)position2.Handle0); } int ITextContainer.CompareTo(StaticTextPointer position1, ITextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo(position2); } object ITextContainer.GetValue(StaticTextPointer position, DependencyProperty formattingProperty) { return ((ITextPointer)position.Handle0).GetValue(formattingProperty); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties ////// bool ITextContainer.IsReadOnly { get { return true; } } ////// /// ITextPointer ITextContainer.Start { get { Debug.Assert(_start != null); return _start; } } ////// /// ITextPointer ITextContainer.End { get { Debug.Assert(_end != null); return _end; } } ////// /// Autoincremented counter of content changes in this TextContainer /// uint ITextContainer.Generation { get { // For read-only content, return some constant value. return 0; } } ////// Collection of highlights applied to TextContainer content. /// Highlights ITextContainer.Highlights { get { //agurcan: The following line makes it hard to use debugger on FDS code so I'm commenting it out //Debug.Assert(false, "Unexpected Highlights access on NullTextContainer!"); return null; } } ////// DependencyObject ITextContainer.Parent { get { return null; } } // Optional text selection, always null for this ITextContainer. ITextSelection ITextContainer.TextSelection { get { return null; } set { Invariant.Assert(false, "NullTextContainer is never associated with a TextEditor/TextSelection!"); } } // Optional undo manager, always null for this ITextContainer. UndoManager ITextContainer.UndoManager { get { return null; } } ///// // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // Description: // NullTextContainer is an immutable empty TextContainer that contains // single collapsed Start/End position. This is primarily used internally // so parameter check is mostly replaced with debug assert. // // History: // 07/14/2004 - Zhenbin Xu (ZhenbinX) - Created. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Diagnostics; using System.Windows.Threading; using System.Windows; // DependencyID etc. using MS.Internal.Documents; using MS.Internal; //===================================================================== /// /// NullTextContainer is an immutable empty TextContainer that contains /// single collapsed Start/End position. This is primarily used internally /// so parameter check is mostly replaced with debug assert. /// internal sealed class NullTextContainer : ITextContainer { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors internal NullTextContainer() { _start = new NullTextPointer(this, LogicalDirection.Backward); _end = new NullTextPointer(this, LogicalDirection.Forward); } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods // // This is readonly Text OM. All modification methods returns false // void ITextContainer.BeginChange() { } ////// void ITextContainer.BeginChangeNoUndo() { // We don't support undo, so follow the BeginChange codepath. ((ITextContainer)this).BeginChange(); } ////// /// void ITextContainer.EndChange() { ((ITextContainer)this).EndChange(false /* skipEvents */); } ////// void ITextContainer.EndChange(bool skipEvents) { } // Allocate a new ITextPointer at the specified offset. // Equivalent to this.Start.CreatePointer(offset), but does not // necessarily allocate this.Start. ITextPointer ITextContainer.CreatePointerAtOffset(int offset, LogicalDirection direction) { return ((ITextContainer)this).Start.CreatePointer(offset, direction); } // Not Implemented. ITextPointer ITextContainer.CreatePointerAtCharOffset(int charOffset, LogicalDirection direction) { throw new NotImplementedException(); } ITextPointer ITextContainer.CreateDynamicTextPointer(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).CreatePointer(direction); } StaticTextPointer ITextContainer.CreateStaticPointerAtOffset(int offset) { return new StaticTextPointer(this, ((ITextContainer)this).CreatePointerAtOffset(offset, LogicalDirection.Forward)); } TextPointerContext ITextContainer.GetPointerContext(StaticTextPointer pointer, LogicalDirection direction) { return ((ITextPointer)pointer.Handle0).GetPointerContext(direction); } int ITextContainer.GetOffsetToPosition(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).GetOffsetToPosition((ITextPointer)position2.Handle0); } int ITextContainer.GetTextInRun(StaticTextPointer position, LogicalDirection direction, char[] textBuffer, int startIndex, int count) { return ((ITextPointer)position.Handle0).GetTextInRun(direction, textBuffer, startIndex, count); } object ITextContainer.GetAdjacentElement(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).GetAdjacentElement(direction); } DependencyObject ITextContainer.GetParent(StaticTextPointer position) { return null; } StaticTextPointer ITextContainer.CreatePointer(StaticTextPointer position, int offset) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).CreatePointer(offset)); } StaticTextPointer ITextContainer.GetNextContextPosition(StaticTextPointer position, LogicalDirection direction) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).GetNextContextPosition(direction)); } int ITextContainer.CompareTo(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo((ITextPointer)position2.Handle0); } int ITextContainer.CompareTo(StaticTextPointer position1, ITextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo(position2); } object ITextContainer.GetValue(StaticTextPointer position, DependencyProperty formattingProperty) { return ((ITextPointer)position.Handle0).GetValue(formattingProperty); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties ////// bool ITextContainer.IsReadOnly { get { return true; } } ////// /// ITextPointer ITextContainer.Start { get { Debug.Assert(_start != null); return _start; } } ////// /// ITextPointer ITextContainer.End { get { Debug.Assert(_end != null); return _end; } } ////// /// Autoincremented counter of content changes in this TextContainer /// uint ITextContainer.Generation { get { // For read-only content, return some constant value. return 0; } } ////// Collection of highlights applied to TextContainer content. /// Highlights ITextContainer.Highlights { get { //agurcan: The following line makes it hard to use debugger on FDS code so I'm commenting it out //Debug.Assert(false, "Unexpected Highlights access on NullTextContainer!"); return null; } } ////// DependencyObject ITextContainer.Parent { get { return null; } } // Optional text selection, always null for this ITextContainer. ITextSelection ITextContainer.TextSelection { get { return null; } set { Invariant.Assert(false, "NullTextContainer is never associated with a TextEditor/TextSelection!"); } } // Optional undo manager, always null for this ITextContainer. UndoManager ITextContainer.UndoManager { get { return null; } } /////
Link Menu
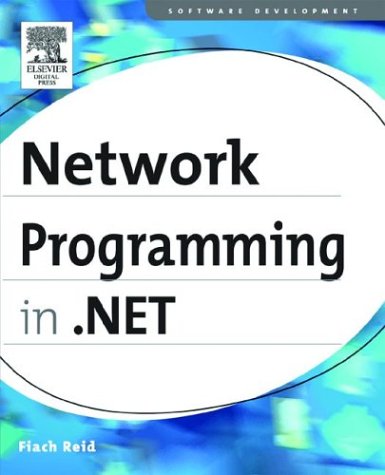
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- odbcmetadatacollectionnames.cs
- MethodImplAttribute.cs
- TemplateField.cs
- TypeResolver.cs
- ScriptResourceAttribute.cs
- SourceFileBuildProvider.cs
- CompletionCallbackWrapper.cs
- ServiceKnownTypeAttribute.cs
- ReceiveActivity.cs
- ObjectSecurity.cs
- ConfigPathUtility.cs
- TdsParameterSetter.cs
- MainMenu.cs
- ObjectDataSourceSelectingEventArgs.cs
- DataChangedEventManager.cs
- TextLine.cs
- SchemaSetCompiler.cs
- ExtendedPropertyDescriptor.cs
- InputScopeConverter.cs
- Ticks.cs
- VirtualPathUtility.cs
- ButtonBaseAdapter.cs
- CalculatedColumn.cs
- MULTI_QI.cs
- ListDictionary.cs
- TreeNodeStyleCollectionEditor.cs
- PointConverter.cs
- DataGridViewCellEventArgs.cs
- RenderData.cs
- LogConverter.cs
- IisTraceWebEventProvider.cs
- DataGridItemCollection.cs
- TypeLoadException.cs
- BaseDataList.cs
- LocationReference.cs
- PseudoWebRequest.cs
- Visitor.cs
- XXXOnTypeBuilderInstantiation.cs
- WebResourceAttribute.cs
- Point3DKeyFrameCollection.cs
- UrlPropertyAttribute.cs
- UpdateExpressionVisitor.cs
- DesignerSerializationOptionsAttribute.cs
- TemplateBindingExtension.cs
- TemplateBindingExtensionConverter.cs
- StreamGeometry.cs
- DataExpression.cs
- OperatorExpressions.cs
- ThreadAttributes.cs
- SqlNodeAnnotations.cs
- DrawingGroup.cs
- CmsUtils.cs
- MarkedHighlightComponent.cs
- StyleXamlParser.cs
- CustomWebEventKey.cs
- CommandBinding.cs
- EntityDesignerDataSourceView.cs
- NavigationProgressEventArgs.cs
- FlowLayoutPanel.cs
- SplitterEvent.cs
- FileAuthorizationModule.cs
- FlowDocumentReaderAutomationPeer.cs
- ResourceReferenceExpressionConverter.cs
- SystemUnicastIPAddressInformation.cs
- Timer.cs
- SchemaElementDecl.cs
- AbstractDataSvcMapFileLoader.cs
- WebBrowserEvent.cs
- WMICapabilities.cs
- BookmarkScopeManager.cs
- CategoryNameCollection.cs
- EdmRelationshipRoleAttribute.cs
- ComNativeDescriptor.cs
- FastPropertyAccessor.cs
- Int32Collection.cs
- InternalTransaction.cs
- RawAppCommandInputReport.cs
- CompensatableTransactionScopeActivity.cs
- URL.cs
- SplashScreenNativeMethods.cs
- LongValidatorAttribute.cs
- XamlToRtfWriter.cs
- ComponentDesigner.cs
- ServiceBehaviorElement.cs
- ThreadPool.cs
- CriticalFinalizerObject.cs
- Configuration.cs
- CommonServiceBehaviorElement.cs
- HttpListener.cs
- PauseStoryboard.cs
- ConfigDefinitionUpdates.cs
- XMLSchema.cs
- FullTrustAssembly.cs
- XmlBaseReader.cs
- XmlStringTable.cs
- LabelTarget.cs
- QilPatternVisitor.cs
- Properties.cs
- ZipIOModeEnforcingStream.cs
- BindingListCollectionView.cs