Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / IO / Packaging / PseudoWebRequest.cs / 1 / PseudoWebRequest.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WebRequest class to handle requests for pack-specific URI's that can be satisfied // from the PackageStore. // // History: // 01/25/2006: [....]: Extracted from PackWebRequest file and extended. // //----------------------------------------------------------------------------- #if DEBUG #define TRACE #endif using System; using System.IO; using System.IO.Packaging; using System.Net; using System.Net.Cache; // for RequestCachePolicy using System.Runtime.Serialization; using System.Diagnostics; // For Assert using MS.Utility; // for EventTrace using MS.Internal.IO.Packaging; // for PackageCacheEntry using MS.Internal.PresentationCore; // for SRID exception strings using MS.Internal; namespace MS.Internal.IO.Packaging { ////// pack-specific WebRequest handler for cached packages /// internal class PseudoWebRequest : WebRequest { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Cached instance constructor /// /// uri to resolve /// uri of the package /// uri of the part - may be null /// cache entry to base this response on internal PseudoWebRequest(Uri uri, Uri packageUri, Uri partUri, Package cacheEntry) { Debug.Assert(uri != null, "PackWebRequest uri cannot be null"); Debug.Assert(packageUri != null, "packageUri cannot be null"); Debug.Assert(partUri != null, "partUri cannot be null"); // keep these _uri = uri; _innerUri = packageUri; _partName = partUri; _cacheEntry = cacheEntry; // set defaults SetDefaults(); } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region WebRequest - Sync ////// GetRequestStream /// ///stream ///writing not supported public override Stream GetRequestStream() { throw new NotSupportedException(); } ////// Do not call /// ///null public override WebResponse GetResponse() { Invariant.Assert(false, "PackWebRequest must handle this method."); return null; } #endregion //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Properties ////// CachePolicy for the PackWebRequest /// ///This value is distinct from the CachePolicy of the InnerRequest. ///public override RequestCachePolicy CachePolicy { get { Invariant.Assert(false, "PackWebRequest must handle this method."); return null; } set { Invariant.Assert(false, "PackWebRequest must handle this method."); } } /// /// ConnectionGroupName /// ///String.Empty is the default value public override string ConnectionGroupName { get { return _connectionGroupName; } set { _connectionGroupName = value; } } ////// ContentLength /// ///length of RequestStream public override long ContentLength { get { return _contentLength; } set { throw new NotSupportedException(); } } ////// ContentType /// public override string ContentType { get { return _contentType; } set { // null is explicitly allowed _contentType = value; } } ////// Credentials /// ///Credentials to use when authenticating against the resource ///null is the default value. public override ICredentials Credentials { get { return _credentials; } set { _credentials = value; } } ////// Headers /// ///collection of header name/value pairs associated with the request ///Default is an empty collection. Null is not a valid value. public override WebHeaderCollection Headers { get { // lazy init if (_headers == null) _headers = new WebHeaderCollection(); return _headers; } set { if (value == null) throw new ArgumentNullException("value"); _headers = value; } } ////// Method /// ///protocol method to use in this request ///This value is shared with the InnerRequest. public override string Method { get { return _method; } set { if (value == null) throw new ArgumentNullException("value"); _method = value; } } ////// PreAuthenticate /// ///default is false public override bool PreAuthenticate { get { return _preAuthenticate; } set { _preAuthenticate = value; } } ////// Proxy /// public override IWebProxy Proxy { get { // lazy init if (_proxy == null) _proxy = WebRequest.DefaultWebProxy; return _proxy; } set { _proxy = value; } } ////// Timeout /// ///length of time before the request times out ///This value is shared with the InnerRequest. ///Value must be >= -1 public override int Timeout { get { return _timeout; } set { // negative time that is not -1 (infinite) is an error case if (value < 0 && value != System.Threading.Timeout.Infinite) throw new ArgumentOutOfRangeException("value"); _timeout = value; } } ////// UseDefaultCredentials /// ///This is an odd case where http acts "normally" but ftp throws NotSupportedException. public override bool UseDefaultCredentials { get { // ftp throws on this if (IsScheme(Uri.UriSchemeFtp)) throw new NotSupportedException(); return _useDefaultCredentials; } set { // ftp throws on this if (IsScheme(Uri.UriSchemeFtp)) throw new NotSupportedException(); _useDefaultCredentials = value; } } #endregion //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ private bool IsScheme(String schemeName) { return (String.CompareOrdinal(_innerUri.Scheme, schemeName) == 0); } ////// Non-value members are lazy-initialized if possible /// private void SetDefaults() { // set defaults _connectionGroupName = String.Empty; // http default _contentType = null; // default _credentials = null; // actual default _headers = null; // lazy init _preAuthenticate = false; // http default _proxy = null; // lazy init if (IsScheme(Uri.UriSchemeHttp)) { _timeout = 100000; // http default - 100s _method = WebRequestMethods.Http.Get; // http default } else _timeout = System.Threading.Timeout.Infinite; // ftp default and appropriate for cached file if (IsScheme(Uri.UriSchemeFtp)) _method = WebRequestMethods.Ftp.DownloadFile; // ftp default _useDefaultCredentials = false; // http default _contentLength = -1; // we don't support upload } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Uri _uri; // pack uri private Uri _innerUri; // inner uri extracted from the pack uri private Uri _partName; // name of PackagePart (if any) - null for full-container references private Package _cacheEntry; // cached package // local copies of public members private string _connectionGroupName; private string _contentType; // value of [CONTENT-TYPE] in WebHeaderCollection - provided by server private int _contentLength; // length of data to upload - should be -1 private string _method; private ICredentials _credentials; // default is null private WebHeaderCollection _headers; // empty is default private bool _preAuthenticate; // default to false private IWebProxy _proxy; private int _timeout; // timeout private bool _useDefaultCredentials; // default is false for HTTP, exception for FTP } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
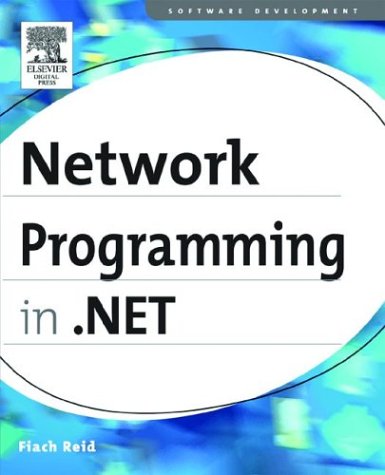
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Tablet.cs
- MSAAWinEventWrap.cs
- SqlErrorCollection.cs
- DataPagerFieldItem.cs
- XmlSchema.cs
- ToolStripButton.cs
- ClrPerspective.cs
- RightsManagementErrorHandler.cs
- TextElementEnumerator.cs
- XmlSchemaAll.cs
- CollectionBase.cs
- XmlQueryCardinality.cs
- Camera.cs
- XmlRawWriterWrapper.cs
- DataError.cs
- DataTableReader.cs
- ApplicationProxyInternal.cs
- InternalMappingException.cs
- CaseInsensitiveHashCodeProvider.cs
- RectangleHotSpot.cs
- CustomAttributeFormatException.cs
- DataBindingCollectionEditor.cs
- GridLength.cs
- HMACRIPEMD160.cs
- AsyncPostBackErrorEventArgs.cs
- RoleManagerModule.cs
- InfoCardSymmetricAlgorithm.cs
- MergeFilterQuery.cs
- DynamicMethod.cs
- SrgsElementFactory.cs
- XmlChoiceIdentifierAttribute.cs
- Quad.cs
- HtmlTitle.cs
- Style.cs
- NavigateEvent.cs
- SQLRoleProvider.cs
- DesignerCalendarAdapter.cs
- XhtmlBasicTextBoxAdapter.cs
- SqlConnection.cs
- JsonClassDataContract.cs
- TimelineClockCollection.cs
- NavigationWindow.cs
- RubberbandSelector.cs
- Size3D.cs
- XamlTemplateSerializer.cs
- DataGridViewRowsRemovedEventArgs.cs
- Button.cs
- ContentElement.cs
- TrustSection.cs
- ToolStripDropDownItem.cs
- Version.cs
- DecoderNLS.cs
- LicenseProviderAttribute.cs
- ListViewItem.cs
- SoapRpcMethodAttribute.cs
- Single.cs
- DispatcherFrame.cs
- ConstrainedDataObject.cs
- DataContractJsonSerializerOperationFormatter.cs
- ControlPropertyNameConverter.cs
- CompatibleComparer.cs
- DataFormat.cs
- ObjRef.cs
- SeverityFilter.cs
- FormatterServices.cs
- TableRowGroup.cs
- SoapTransportImporter.cs
- TextEvent.cs
- ConfigXmlText.cs
- FlagsAttribute.cs
- SchemaEntity.cs
- XmlText.cs
- EdmRelationshipRoleAttribute.cs
- Char.cs
- NavigationService.cs
- PointConverter.cs
- OdbcReferenceCollection.cs
- SortedSet.cs
- RandomNumberGenerator.cs
- BaseAddressPrefixFilterElement.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- ChineseLunisolarCalendar.cs
- PagerSettings.cs
- TableLayoutPanel.cs
- TogglePattern.cs
- FixedSOMImage.cs
- Propagator.JoinPropagator.SubstitutingCloneVisitor.cs
- SequenceDesignerAccessibleObject.cs
- RecognizeCompletedEventArgs.cs
- X509CertificateInitiatorServiceCredential.cs
- SpeechUI.cs
- DecimalConstantAttribute.cs
- ChtmlSelectionListAdapter.cs
- IODescriptionAttribute.cs
- C14NUtil.cs
- CheckStoreFileValidityRequest.cs
- EtwTrace.cs
- PatternMatcher.cs
- GroupedContextMenuStrip.cs
- HtmlLink.cs