Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / CheckStoreFileValidityRequest.cs / 1 / CheckStoreFileValidityRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.IO; using System.Xml; using System.Security.Cryptography; using System.Xml.Schema; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Opens a store file and checks the first element to make sure that this is // a valid infocard store file. // class CheckStoreFileValidityRequest :UIAgentRequest { string m_filename; bool m_valid = true; public CheckStoreFileValidityRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : base( rpcHandle, inArgs, outArgs, parent ) { } protected override void OnInitializeAsSystem() { base.OnInitializeAsSystem(); } // // Summary // Read the marshalled arguments // protected override void OnMarshalInArgs() { BinaryReader reader = new InfoCardBinaryReader( InArgs, System.Text.Encoding.Unicode ); m_filename = Utility.DeserializeString( reader ); } // // Summary // Read the first element of the file // protected override void OnProcess() { try { try { using ( FileStream file = File.OpenRead( m_filename ) ) { // // Use a stream that validates the xml against internally stored schemas. // XmlReaderSettings settings = InfoCardSchemas.CreateDefaultReaderSettings(); settings.IgnoreWhitespace = false; using( XmlReader reader = InfoCardSchemas.CreateReader( file, settings ) ) { IDT.TraceDebug( " Roaming: Check if the store file is valid" ); if( !reader.IsStartElement( XmlNames.WSIdentity.EncryptedStoreElement, XmlNames.WSIdentity.Namespace ) ) { m_valid = false; } } } } catch ( XmlSchemaValidationException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.SchemaValidationFailed) , e ) ); } catch ( UnauthorizedAccessException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.ImportInaccesibleFile ), e ) ); } catch ( IOException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ), e ) ); } catch ( XmlException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFile ), e ) ); } } catch ( ImportException ) { // // Translate ImportException to the boolean value indicating an invalid file // m_valid = false; } } // // Summary // Write the boolean value to be returned // protected override void OnMarshalOutArgs() { Stream stream = OutArgs; BinaryWriter writer = new BinaryWriter( stream ); writer.Write( m_valid ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
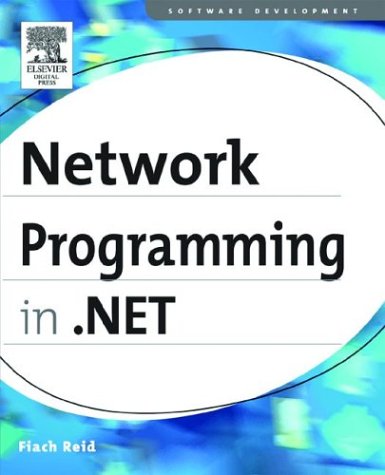
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BigInt.cs
- ExtentJoinTreeNode.cs
- TextEditorCopyPaste.cs
- RegistrationServices.cs
- SoapCodeExporter.cs
- BasicExpressionVisitor.cs
- XmlSchemaAnnotated.cs
- ClientApiGenerator.cs
- KnownAssemblyEntry.cs
- EditorBrowsableAttribute.cs
- KoreanCalendar.cs
- Exceptions.cs
- ProcessDesigner.cs
- WindowVisualStateTracker.cs
- RemotingServices.cs
- documentsequencetextview.cs
- ComPlusInstanceContextInitializer.cs
- NetTcpBindingElement.cs
- ListDictionaryInternal.cs
- Visual.cs
- IgnoreFlushAndCloseStream.cs
- HtmlInputRadioButton.cs
- ItemsPanelTemplate.cs
- DataGridViewToolTip.cs
- StoryFragments.cs
- ZipIOModeEnforcingStream.cs
- ConnectionPoint.cs
- ComponentDispatcherThread.cs
- IUnknownConstantAttribute.cs
- SHA384Managed.cs
- FastPropertyAccessor.cs
- TraceFilter.cs
- coordinatorfactory.cs
- GACMembershipCondition.cs
- CodeChecksumPragma.cs
- TextViewSelectionProcessor.cs
- NetworkCredential.cs
- SiteIdentityPermission.cs
- NavigationProperty.cs
- FilteredAttributeCollection.cs
- PerfCounters.cs
- SymLanguageType.cs
- ActivitySurrogateSelector.cs
- TypeDescriptionProvider.cs
- BindableAttribute.cs
- BaseProcessor.cs
- Content.cs
- ResXResourceSet.cs
- ProfilePropertyNameValidator.cs
- XmlIterators.cs
- MenuItemAutomationPeer.cs
- IISUnsafeMethods.cs
- SplineKeyFrames.cs
- PropertyMappingExceptionEventArgs.cs
- ObjectDisposedException.cs
- XmlSerializationReader.cs
- XmlDataImplementation.cs
- ConsoleTraceListener.cs
- CaseInsensitiveComparer.cs
- TransformProviderWrapper.cs
- FontFaceLayoutInfo.cs
- ErrorFormatter.cs
- XMLDiffLoader.cs
- MergeFailedEvent.cs
- PropertyDescriptor.cs
- DataColumn.cs
- LockCookie.cs
- _IPv6Address.cs
- MemberInfoSerializationHolder.cs
- EncoderParameter.cs
- ValidatingReaderNodeData.cs
- LinkButton.cs
- CodeGen.cs
- MemoryFailPoint.cs
- SaveFileDialog.cs
- XmlToDatasetMap.cs
- EventWaitHandleSecurity.cs
- DataGridBoolColumn.cs
- MenuTracker.cs
- Identity.cs
- OpenFileDialog.cs
- SchemaCreator.cs
- Activity.cs
- DataException.cs
- SingleQueryOperator.cs
- AdministrationHelpers.cs
- TaskDesigner.cs
- AspNetHostingPermission.cs
- AssemblyNameProxy.cs
- _OSSOCK.cs
- ToolboxItemAttribute.cs
- HandlerBase.cs
- Error.cs
- PriorityChain.cs
- Win32MouseDevice.cs
- StorageAssociationTypeMapping.cs
- SplashScreen.cs
- WebSysDisplayNameAttribute.cs
- DocumentPageView.cs
- Literal.cs