Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Mapping / StorageAssociationTypeMapping.cs / 1 / StorageAssociationTypeMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; namespace System.Data.Mapping { ////// Represents the Mapping metadata for an association type map in CS space. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ComplexTypeMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EndPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --EndPropertyMap /// --ScalarPropertyMap /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// --TableMappingFragment /// --ParentEntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ComplexTypeMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// This class represents the metadata for all association Type map elements in the /// above example. Users can access the table mapping fragments under the /// association type mapping through this class. /// internal class StorageAssociationTypeMapping : StorageTypeMapping { #region Constructors ////// Construct the new AssociationTypeMapping object. /// /// Represents the Association Type metadata object /// Set Mapping that contains this Type mapping internal StorageAssociationTypeMapping(AssociationType relation, StorageSetMapping setMapping) : base(setMapping) { this.m_relation = relation; } #endregion #region Fields AssociationType m_relation; //Type for which the mapping is represented #endregion #region Properties ////// The AssociationTypeType Metadata object for which the mapping is represented. /// internal AssociationType AssociationType { get { return this.m_relation; } } ////// a list of TypeMetadata that this mapping holds true for. /// Since Association types dont participate in Inheritance, This can only /// be one type. /// internal override ReadOnlyCollectionTypes { get { return new ReadOnlyCollection (new AssociationType[] { m_relation }); } } /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// Since Association types dont participate in Inheritance, an Empty list /// is returned here. /// internal override ReadOnlyCollectionIsOfTypes { get { return new List ().AsReadOnly(); } } #endregion #region Methods /// /// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal override void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("AssociationTypeMapping"); sb.Append(" "); sb.Append("Type Name:"); sb.Append(this.m_relation.Name); sb.Append(" "); Console.WriteLine(sb.ToString()); foreach (StorageMappingFragment fragment in MappingFragments) { fragment.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; namespace System.Data.Mapping { ////// Represents the Mapping metadata for an association type map in CS space. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ComplexTypeMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EndPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --EndPropertyMap /// --ScalarPropertyMap /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// --TableMappingFragment /// --ParentEntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ComplexTypeMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// This class represents the metadata for all association Type map elements in the /// above example. Users can access the table mapping fragments under the /// association type mapping through this class. /// internal class StorageAssociationTypeMapping : StorageTypeMapping { #region Constructors ////// Construct the new AssociationTypeMapping object. /// /// Represents the Association Type metadata object /// Set Mapping that contains this Type mapping internal StorageAssociationTypeMapping(AssociationType relation, StorageSetMapping setMapping) : base(setMapping) { this.m_relation = relation; } #endregion #region Fields AssociationType m_relation; //Type for which the mapping is represented #endregion #region Properties ////// The AssociationTypeType Metadata object for which the mapping is represented. /// internal AssociationType AssociationType { get { return this.m_relation; } } ////// a list of TypeMetadata that this mapping holds true for. /// Since Association types dont participate in Inheritance, This can only /// be one type. /// internal override ReadOnlyCollectionTypes { get { return new ReadOnlyCollection (new AssociationType[] { m_relation }); } } /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// Since Association types dont participate in Inheritance, an Empty list /// is returned here. /// internal override ReadOnlyCollectionIsOfTypes { get { return new List ().AsReadOnly(); } } #endregion #region Methods /// /// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal override void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("AssociationTypeMapping"); sb.Append(" "); sb.Append("Type Name:"); sb.Append(this.m_relation.Name); sb.Append(" "); Console.WriteLine(sb.ToString()); foreach (StorageMappingFragment fragment in MappingFragments) { fragment.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
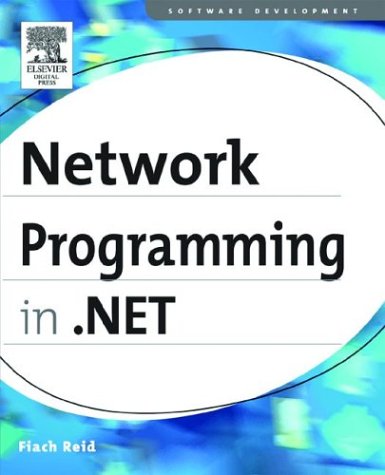
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DispatcherEventArgs.cs
- ProjectedSlot.cs
- IOException.cs
- ScriptingRoleServiceSection.cs
- ArrayItemReference.cs
- WebPartConnectionsCancelEventArgs.cs
- MemberProjectedSlot.cs
- BaseDataBoundControl.cs
- FunctionDetailsReader.cs
- MailBnfHelper.cs
- EventProviderWriter.cs
- DefaultAuthorizationContext.cs
- PanelStyle.cs
- TableRow.cs
- HTTPNotFoundHandler.cs
- DesignerVerb.cs
- UdpDiscoveryMessageFilter.cs
- ParamArrayAttribute.cs
- StringTraceRecord.cs
- TdsParserHelperClasses.cs
- ButtonBaseAutomationPeer.cs
- Publisher.cs
- TextSelectionProcessor.cs
- relpropertyhelper.cs
- DbMetaDataCollectionNames.cs
- ByteAnimationBase.cs
- BindingCompleteEventArgs.cs
- CompilationUnit.cs
- LookupBindingPropertiesAttribute.cs
- PrivilegedConfigurationManager.cs
- DocumentXPathNavigator.cs
- DecoratedNameAttribute.cs
- HTTPNotFoundHandler.cs
- PageThemeBuildProvider.cs
- webeventbuffer.cs
- ConnectorRouter.cs
- DataListItemEventArgs.cs
- WebPartDisplayModeCollection.cs
- SafeTokenHandle.cs
- SqlCaseSimplifier.cs
- BitmapInitialize.cs
- CancellationToken.cs
- WebServicesInteroperability.cs
- AnnotationObservableCollection.cs
- DetailsViewUpdatedEventArgs.cs
- SizeConverter.cs
- GiveFeedbackEventArgs.cs
- XmlQualifiedName.cs
- VisualStyleInformation.cs
- TailCallAnalyzer.cs
- XmlAnyAttributeAttribute.cs
- QueuePropertyVariants.cs
- EventlogProvider.cs
- Vector3D.cs
- SynchronizedKeyedCollection.cs
- DataGrid.cs
- ErrorFormatterPage.cs
- MailBnfHelper.cs
- DocumentGridPage.cs
- DesignObjectWrapper.cs
- AdapterDictionary.cs
- NavigationEventArgs.cs
- ApplicationCommands.cs
- EncoderReplacementFallback.cs
- GroupStyle.cs
- ObjectPersistData.cs
- IQueryable.cs
- QilParameter.cs
- Currency.cs
- DateTimeOffset.cs
- PropertyDescriptor.cs
- FlowchartSizeFeature.cs
- PropertyManager.cs
- DesignerSerializationOptionsAttribute.cs
- Transform3DCollection.cs
- WaitHandleCannotBeOpenedException.cs
- BaseTreeIterator.cs
- SignatureDescription.cs
- DoubleMinMaxAggregationOperator.cs
- PassportAuthentication.cs
- ExpandableObjectConverter.cs
- StringBuilder.cs
- WmfPlaceableFileHeader.cs
- Filter.cs
- HttpsHostedTransportConfiguration.cs
- ExceptionValidationRule.cs
- PrintPageEvent.cs
- ProfileSection.cs
- WebBaseEventKeyComparer.cs
- InternalBufferManager.cs
- SecurityContext.cs
- ForwardPositionQuery.cs
- NotificationContext.cs
- ConsumerConnectionPoint.cs
- IsolatedStorage.cs
- TextCompositionManager.cs
- LocalizationParserHooks.cs
- SafeWaitHandle.cs
- StylusLogic.cs
- WindowsGraphics2.cs