Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / XmlQualifiedName.cs / 1 / XmlQualifiedName.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System.Collections; using System.Diagnostics; ////// /// [Serializable] public class XmlQualifiedName { string name; string ns; [NonSerialized] Int32 hash; ///[To be supplied.] ////// /// public static readonly XmlQualifiedName Empty = new XmlQualifiedName(string.Empty); ///[To be supplied.] ////// /// public XmlQualifiedName() : this(string.Empty, string.Empty) {} ///[To be supplied.] ////// /// public XmlQualifiedName(string name) : this(name, string.Empty) {} ///[To be supplied.] ////// /// public XmlQualifiedName(string name, string ns) { this.ns = ns == null ? string.Empty : ns; this.name = name == null ? string.Empty : name; } ///[To be supplied.] ////// /// public string Namespace { get { return ns; } } ///[To be supplied.] ////// /// public string Name { get { return name; } } ///[To be supplied.] ////// /// public override int GetHashCode() { if(hash == 0) { hash = Name.GetHashCode() /*+ Namespace.GetHashCode()*/; // for perf reasons we are not taking ns's hashcode. } return hash; } ///[To be supplied.] ////// /// public bool IsEmpty { get { return Name.Length == 0 && Namespace.Length == 0; } } ///[To be supplied.] ////// /// public override string ToString() { return Namespace.Length == 0 ? Name : string.Concat(Namespace, ":" , Name); } ///[To be supplied.] ////// /// public override bool Equals(object other) { XmlQualifiedName qname; if ((object) this == other) { return true; } qname = other as XmlQualifiedName; if (qname != null) { return (Name == qname.Name && Namespace == qname.Namespace); } return false; } ///[To be supplied.] ////// /// public static bool operator ==(XmlQualifiedName a, XmlQualifiedName b) { if ((object) a == (object) b) return true; if ((object) a == null || (object) b == null) return false; return (a.Name == b.Name && a.Namespace == b.Namespace); } ///[To be supplied.] ////// /// public static bool operator !=(XmlQualifiedName a, XmlQualifiedName b) { return !(a == b); } ///[To be supplied.] ////// /// public static string ToString(string name, string ns) { return ns == null || ns.Length == 0 ? name : ns + ":" + name; } // --------- Some useful internal stuff ----------------- internal void Init(string name, string ns) { Debug.Assert(name != null && ns != null); this.name = name; this.ns = ns; this.hash = 0; } internal void SetNamespace(string ns) { Debug.Assert(ns != null); this.ns = ns; //Not changing hash since ns is not used to compute hashcode } internal void Verify() { XmlConvert.VerifyNCName(name); if (ns.Length != 0) { XmlConvert.ToUri(ns); } } internal void Atomize(XmlNameTable nameTable) { Debug.Assert(name != null); name = nameTable.Add(name); ns = nameTable.Add(ns); } internal static XmlQualifiedName Parse(string s, IXmlNamespaceResolver nsmgr, out string prefix) { string localName; ValidateNames.ParseQNameThrow(s, out prefix, out localName); string uri = nsmgr.LookupNamespace(prefix); if (uri == null) { if (prefix.Length != 0) { throw new XmlException(Res.Xml_UnknownNs, prefix); } else { //Re-map namespace of empty prefix to string.Empty when there is no default namespace declared uri = string.Empty; } } return new XmlQualifiedName(localName, uri); } internal XmlQualifiedName Clone() { return (XmlQualifiedName)MemberwiseClone(); } internal static int Compare(XmlQualifiedName a, XmlQualifiedName b) { if (null == a) { return (null == b) ? 0 : -1; } if (null == b) { return 1; } int i = String.CompareOrdinal(a.Namespace, b.Namespace); if (i == 0) { i = String.CompareOrdinal(a.Name, b.Name); } return i; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System.Collections; using System.Diagnostics; ////// /// [Serializable] public class XmlQualifiedName { string name; string ns; [NonSerialized] Int32 hash; ///[To be supplied.] ////// /// public static readonly XmlQualifiedName Empty = new XmlQualifiedName(string.Empty); ///[To be supplied.] ////// /// public XmlQualifiedName() : this(string.Empty, string.Empty) {} ///[To be supplied.] ////// /// public XmlQualifiedName(string name) : this(name, string.Empty) {} ///[To be supplied.] ////// /// public XmlQualifiedName(string name, string ns) { this.ns = ns == null ? string.Empty : ns; this.name = name == null ? string.Empty : name; } ///[To be supplied.] ////// /// public string Namespace { get { return ns; } } ///[To be supplied.] ////// /// public string Name { get { return name; } } ///[To be supplied.] ////// /// public override int GetHashCode() { if(hash == 0) { hash = Name.GetHashCode() /*+ Namespace.GetHashCode()*/; // for perf reasons we are not taking ns's hashcode. } return hash; } ///[To be supplied.] ////// /// public bool IsEmpty { get { return Name.Length == 0 && Namespace.Length == 0; } } ///[To be supplied.] ////// /// public override string ToString() { return Namespace.Length == 0 ? Name : string.Concat(Namespace, ":" , Name); } ///[To be supplied.] ////// /// public override bool Equals(object other) { XmlQualifiedName qname; if ((object) this == other) { return true; } qname = other as XmlQualifiedName; if (qname != null) { return (Name == qname.Name && Namespace == qname.Namespace); } return false; } ///[To be supplied.] ////// /// public static bool operator ==(XmlQualifiedName a, XmlQualifiedName b) { if ((object) a == (object) b) return true; if ((object) a == null || (object) b == null) return false; return (a.Name == b.Name && a.Namespace == b.Namespace); } ///[To be supplied.] ////// /// public static bool operator !=(XmlQualifiedName a, XmlQualifiedName b) { return !(a == b); } ///[To be supplied.] ////// /// public static string ToString(string name, string ns) { return ns == null || ns.Length == 0 ? name : ns + ":" + name; } // --------- Some useful internal stuff ----------------- internal void Init(string name, string ns) { Debug.Assert(name != null && ns != null); this.name = name; this.ns = ns; this.hash = 0; } internal void SetNamespace(string ns) { Debug.Assert(ns != null); this.ns = ns; //Not changing hash since ns is not used to compute hashcode } internal void Verify() { XmlConvert.VerifyNCName(name); if (ns.Length != 0) { XmlConvert.ToUri(ns); } } internal void Atomize(XmlNameTable nameTable) { Debug.Assert(name != null); name = nameTable.Add(name); ns = nameTable.Add(ns); } internal static XmlQualifiedName Parse(string s, IXmlNamespaceResolver nsmgr, out string prefix) { string localName; ValidateNames.ParseQNameThrow(s, out prefix, out localName); string uri = nsmgr.LookupNamespace(prefix); if (uri == null) { if (prefix.Length != 0) { throw new XmlException(Res.Xml_UnknownNs, prefix); } else { //Re-map namespace of empty prefix to string.Empty when there is no default namespace declared uri = string.Empty; } } return new XmlQualifiedName(localName, uri); } internal XmlQualifiedName Clone() { return (XmlQualifiedName)MemberwiseClone(); } internal static int Compare(XmlQualifiedName a, XmlQualifiedName b) { if (null == a) { return (null == b) ? 0 : -1; } if (null == b) { return 1; } int i = String.CompareOrdinal(a.Namespace, b.Namespace); if (i == 0) { i = String.CompareOrdinal(a.Name, b.Name); } return i; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
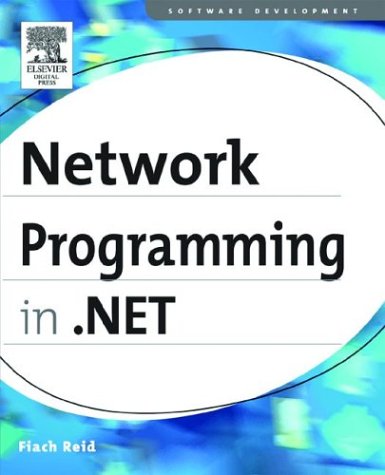
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SendMailErrorEventArgs.cs
- DrawingDrawingContext.cs
- TreeWalker.cs
- OleDbParameterCollection.cs
- LogicalMethodInfo.cs
- SizeValueSerializer.cs
- PageFunction.cs
- ArrayExtension.cs
- uribuilder.cs
- FixedSOMSemanticBox.cs
- CacheDict.cs
- IDispatchConstantAttribute.cs
- TextBox.cs
- Button.cs
- autovalidator.cs
- GeometryHitTestParameters.cs
- NodeFunctions.cs
- CommonGetThemePartSize.cs
- Pens.cs
- VisualStyleTypesAndProperties.cs
- TextSerializer.cs
- FamilyTypeface.cs
- RandomNumberGenerator.cs
- FirstMatchCodeGroup.cs
- TemplatedWizardStep.cs
- WebPartConnectionsCancelVerb.cs
- X509ChainPolicy.cs
- TypePresenter.xaml.cs
- TargetConverter.cs
- FixedTextBuilder.cs
- WaitHandleCannotBeOpenedException.cs
- ISAPIApplicationHost.cs
- DragAssistanceManager.cs
- UnsafeNativeMethodsPenimc.cs
- PropertyGeneratedEventArgs.cs
- CfgSemanticTag.cs
- Paragraph.cs
- XmlHierarchyData.cs
- CryptoHandle.cs
- Reference.cs
- StyleSheetRefUrlEditor.cs
- XmlNodeComparer.cs
- TypeGeneratedEventArgs.cs
- ObjectViewFactory.cs
- XPathDescendantIterator.cs
- TimeSpanValidator.cs
- IssuedTokensHeader.cs
- AppDomainAttributes.cs
- columnmapkeybuilder.cs
- WebPartEditorApplyVerb.cs
- SystemIcmpV4Statistics.cs
- CapiSafeHandles.cs
- _AuthenticationState.cs
- DetailsViewRowCollection.cs
- SimpleWorkerRequest.cs
- InternalSendMessage.cs
- WindowsRegion.cs
- XmlDownloadManager.cs
- Mapping.cs
- GradientStopCollection.cs
- StateMachineWorkflow.cs
- ModelPerspective.cs
- WindowsSolidBrush.cs
- FigureParagraph.cs
- ProgressBar.cs
- ValidatorCompatibilityHelper.cs
- GridToolTip.cs
- TdsRecordBufferSetter.cs
- QilCloneVisitor.cs
- HostProtectionException.cs
- DataStreamFromComStream.cs
- ContainerAction.cs
- NavigationService.cs
- DefaultBindingPropertyAttribute.cs
- ThrowHelper.cs
- FormsAuthenticationTicket.cs
- TableItemPatternIdentifiers.cs
- CounterCreationData.cs
- XmlChildEnumerator.cs
- GridViewRow.cs
- COM2ExtendedBrowsingHandler.cs
- BitmapScalingModeValidation.cs
- XmlSignatureManifest.cs
- GradientSpreadMethodValidation.cs
- SQLSingle.cs
- GacUtil.cs
- HandlerFactoryWrapper.cs
- X509CertificateStore.cs
- ToolZone.cs
- TreeView.cs
- PhysicalAddress.cs
- DictionaryBase.cs
- HttpListenerElement.cs
- PenContext.cs
- XmlCompatibilityReader.cs
- AnonymousIdentificationModule.cs
- Cursor.cs
- HelpEvent.cs
- StringCollectionEditor.cs
- SerializationBinder.cs