Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataStreamFromComStream.cs / 1 / DataStreamFromComStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.IO; ////// /// /// internal class DataStreamFromComStream : Stream { private UnsafeNativeMethods.IStream comStream; public DataStreamFromComStream(UnsafeNativeMethods.IStream comStream) : base() { this.comStream = comStream; } public override long Position { get { return Seek(0, SeekOrigin.Current); } set { Seek(value, SeekOrigin.Begin); } } public override bool CanWrite { get { return true; } } public override bool CanSeek { get { return true; } } public override bool CanRead { get { return true; } } public override long Length { get { long curPos = this.Position; long endPos = Seek(0, SeekOrigin.End); this.Position = curPos; return endPos - curPos; } } /* private void _NotImpl(string message) { NotSupportedException ex = new NotSupportedException(message, new ExternalException(SR.GetString(SR.ExternalException), NativeMethods.E_NOTIMPL)); throw ex; } */ private unsafe int _Read(void* handle, int bytes) { return comStream.Read((IntPtr)handle, bytes); } private unsafe int _Write(void* handle, int bytes) { return comStream.Write((IntPtr)handle, bytes); } public override void Flush() { } public unsafe override int Read(byte[] buffer, int index, int count) { int bytesRead = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { fixed (byte* ch = buffer) { bytesRead = _Read((void*)(ch + index), count); } } return bytesRead; } public override void SetLength(long value) { comStream.SetSize(value); } public override long Seek(long offset, SeekOrigin origin) { return comStream.Seek(offset, (int)origin); } public unsafe override void Write(byte[] buffer, int index, int count) { int bytesWritten = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { try { fixed (byte* b = buffer) { bytesWritten = _Write((void*)(b + index), count); } } catch { } } if (bytesWritten < count) { throw new IOException(SR.GetString(SR.DataStreamWrite)); } } protected override void Dispose(bool disposing) { try { if (disposing && comStream != null) { try { comStream.Commit(NativeMethods.STGC_DEFAULT); } catch(Exception) { } } // Can't release a COM stream from the finalizer thread. comStream = null; } finally { base.Dispose(disposing); } } ~DataStreamFromComStream() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
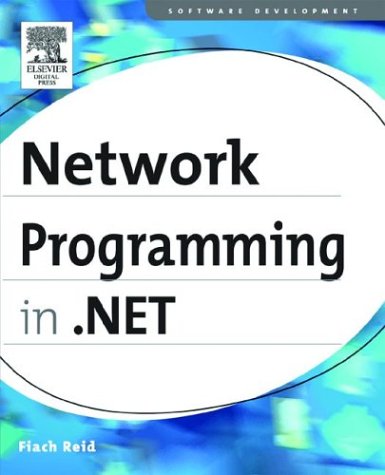
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MouseButtonEventArgs.cs
- WizardPanelChangingEventArgs.cs
- RoleService.cs
- SQLChars.cs
- rsa.cs
- BitmapMetadataEnumerator.cs
- ElementUtil.cs
- MetafileHeader.cs
- StrokeRenderer.cs
- BrowserDefinition.cs
- TimeBoundedCache.cs
- QuaternionValueSerializer.cs
- validation.cs
- ClientRolePrincipal.cs
- OptimalBreakSession.cs
- ObjectSecurity.cs
- MouseWheelEventArgs.cs
- ApplicationSettingsBase.cs
- DesignerWithHeader.cs
- ToolBarButtonClickEvent.cs
- MetadataExchangeBindings.cs
- Rect3DConverter.cs
- COM2TypeInfoProcessor.cs
- InteropAutomationProvider.cs
- AppModelKnownContentFactory.cs
- WebBrowserContainer.cs
- ContourSegment.cs
- XmlSchemaAttributeGroup.cs
- QilStrConcatenator.cs
- EntityParameter.cs
- JoinTreeSlot.cs
- ConstraintEnumerator.cs
- MouseActionConverter.cs
- ConcurrentQueue.cs
- ButtonStandardAdapter.cs
- coordinator.cs
- SiteMapNode.cs
- DataList.cs
- EventLogInformation.cs
- DbModificationCommandTree.cs
- DataIdProcessor.cs
- ResourceContainer.cs
- DeferredElementTreeState.cs
- AttributeUsageAttribute.cs
- Matrix.cs
- Permission.cs
- SmtpClient.cs
- ClientTargetSection.cs
- MessageBox.cs
- DataSourceDescriptorCollection.cs
- ServiceContractGenerator.cs
- DataSetUtil.cs
- SqlBooleanizer.cs
- EventProxy.cs
- TransformPatternIdentifiers.cs
- HebrewNumber.cs
- HitTestFilterBehavior.cs
- RadioButton.cs
- LabelLiteral.cs
- RegexReplacement.cs
- XmlSchemaSimpleType.cs
- QueueTransferProtocol.cs
- BulletedListDesigner.cs
- SendKeys.cs
- Camera.cs
- WebPartsPersonalization.cs
- oledbmetadatacollectionnames.cs
- XmlSchema.cs
- CollectionViewSource.cs
- DataComponentMethodGenerator.cs
- ServiceEndpointElementCollection.cs
- TableRow.cs
- MultipleViewPattern.cs
- CodeRemoveEventStatement.cs
- ListBoxItemAutomationPeer.cs
- ObjectNavigationPropertyMapping.cs
- PeerDuplexChannel.cs
- ToolboxComponentsCreatedEventArgs.cs
- ContentTypeSettingClientMessageFormatter.cs
- InkCanvasSelectionAdorner.cs
- CustomAttribute.cs
- XmlQueryType.cs
- XsdCachingReader.cs
- SizeF.cs
- PrintController.cs
- TreeNodeStyleCollection.cs
- X509Utils.cs
- NumericExpr.cs
- TextSelectionHighlightLayer.cs
- XmlSchemaSimpleTypeList.cs
- ConsoleKeyInfo.cs
- SqlXml.cs
- EntityDataSourceContainerNameConverter.cs
- uribuilder.cs
- DataView.cs
- SegmentInfo.cs
- validationstate.cs
- ActivityDesignerHelper.cs
- CodeSnippetTypeMember.cs
- RegexReplacement.cs