Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / RequestCachePolicyConverter.cs / 1 / RequestCachePolicyConverter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2007 // // File: RequesetCachePolicyConverter.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using System.Net.Cache; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// RequestCachePolicyConverter Parses a RequestCachePolicy. /// public sealed class RequestCachePolicyConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } ////// ConvertFrom - attempt to convert to a RequestCachePolicy from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a RequestCachePolicy. /// public override object ConvertFrom(ITypeDescriptorContext td, System.Globalization.CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } string s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } HttpRequestCacheLevel level = (HttpRequestCacheLevel)Enum.Parse(typeof(HttpRequestCacheLevel), s, true); return new HttpRequestCachePolicy(level); } ////// ConvertTo - Attempt to convert to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the object is not null, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The policy to convert. /// The type to which to convert the policy. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for RequestCachePolicy/HttpRequestCachePolicy, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } HttpRequestCachePolicy httpPolicy = value as HttpRequestCachePolicy; if(httpPolicy != null) { if (destinationType == typeof(string)) { return httpPolicy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(HttpRequestCachePolicy).GetConstructor(new Type[] { typeof(HttpRequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { httpPolicy.Level }); } } //if it's not an HttpRequestCachePolicy, try a regular RequestCachePolicy RequestCachePolicy policy = value as RequestCachePolicy; if (policy != null) { if (destinationType == typeof(string)) { return policy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(RequestCachePolicy).GetConstructor(new Type[] { typeof(RequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { policy.Level }); } } throw GetConvertToException(value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2007 // // File: RequesetCachePolicyConverter.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Windows.Media; using System.Text; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using System.Net.Cache; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// RequestCachePolicyConverter Parses a RequestCachePolicy. /// public sealed class RequestCachePolicyConverter : TypeConverter { ////// CanConvertFrom /// public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } ////// ConvertFrom - attempt to convert to a RequestCachePolicy from the given object /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a RequestCachePolicy. /// public override object ConvertFrom(ITypeDescriptorContext td, System.Globalization.CultureInfo ci, object value) { if (null == value) { throw GetConvertFromException(value); } string s = value as string; if (null == s) { throw new ArgumentException(SR.Get(SRID.General_BadType, "ConvertFrom"), "value"); } HttpRequestCacheLevel level = (HttpRequestCacheLevel)Enum.Parse(typeof(HttpRequestCacheLevel), s, true); return new HttpRequestCachePolicy(level); } ////// ConvertTo - Attempt to convert to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the object is not null, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The policy to convert. /// The type to which to convert the policy. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for RequestCachePolicy/HttpRequestCachePolicy, not an arbitrary class /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } HttpRequestCachePolicy httpPolicy = value as HttpRequestCachePolicy; if(httpPolicy != null) { if (destinationType == typeof(string)) { return httpPolicy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(HttpRequestCachePolicy).GetConstructor(new Type[] { typeof(HttpRequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { httpPolicy.Level }); } } //if it's not an HttpRequestCachePolicy, try a regular RequestCachePolicy RequestCachePolicy policy = value as RequestCachePolicy; if (policy != null) { if (destinationType == typeof(string)) { return policy.Level.ToString(); } else if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(RequestCachePolicy).GetConstructor(new Type[] { typeof(RequestCachePolicy) }); return new InstanceDescriptor(ci, new object[] { policy.Level }); } } throw GetConvertToException(value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
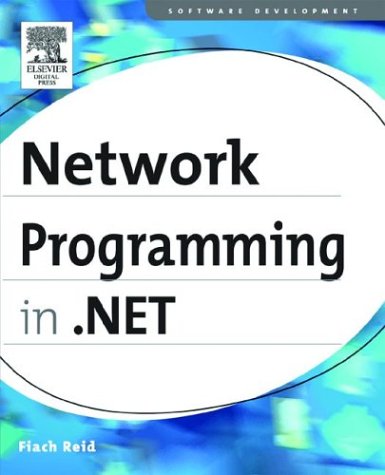
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Track.cs
- ListControl.cs
- DocumentXmlWriter.cs
- ImmutableCollection.cs
- WindowShowOrOpenTracker.cs
- WindowsScroll.cs
- AppDomainProtocolHandler.cs
- TypeTypeConverter.cs
- ProfileProvider.cs
- ViewValidator.cs
- ReverseInheritProperty.cs
- XmlQueryRuntime.cs
- ListBoxItem.cs
- ArrayList.cs
- MenuItemBinding.cs
- WindowsToolbarAsMenu.cs
- WebPartHelpVerb.cs
- AncestorChangedEventArgs.cs
- Helpers.cs
- ConfigurationElementProperty.cs
- Win32KeyboardDevice.cs
- StoreContentChangedEventArgs.cs
- ApplicationSecurityManager.cs
- RegisteredHiddenField.cs
- ModuleBuilder.cs
- DescendentsWalkerBase.cs
- SafeNativeMethods.cs
- TreeChangeInfo.cs
- DoubleAverageAggregationOperator.cs
- SqlInternalConnectionTds.cs
- NonBatchDirectoryCompiler.cs
- AuthenticationService.cs
- HttpListenerResponse.cs
- UniqueConstraint.cs
- mactripleDES.cs
- ToolStripArrowRenderEventArgs.cs
- MetadataArtifactLoader.cs
- DemultiplexingClientMessageFormatter.cs
- ChannelServices.cs
- StandardToolWindows.cs
- PrintingPermission.cs
- PlanCompilerUtil.cs
- CultureTableRecord.cs
- StatusBarItemAutomationPeer.cs
- XmlWhitespace.cs
- MenuItem.cs
- ToolStripSystemRenderer.cs
- WindowsContainer.cs
- RoleGroup.cs
- Membership.cs
- DataContractSerializerSection.cs
- Config.cs
- GZipUtils.cs
- ReferenceSchema.cs
- EnumValidator.cs
- RegularExpressionValidator.cs
- LateBoundBitmapDecoder.cs
- ExecutedRoutedEventArgs.cs
- QilFactory.cs
- OdbcDataReader.cs
- StringWriter.cs
- Int16Converter.cs
- SecurityPermission.cs
- RC2.cs
- Transform3DGroup.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- FullTrustAssembly.cs
- KeyGestureConverter.cs
- TreeView.cs
- ModulesEntry.cs
- BamlLocalizationDictionary.cs
- PersonalizationEntry.cs
- GeometryValueSerializer.cs
- DuplicateWaitObjectException.cs
- NodeLabelEditEvent.cs
- DoubleLinkListEnumerator.cs
- InputScope.cs
- IdentifierService.cs
- ConfigurationPermission.cs
- SafeThemeHandle.cs
- NamedPermissionSet.cs
- x509utils.cs
- TileBrush.cs
- AutomationInteropProvider.cs
- WasAdminWrapper.cs
- TypeDelegator.cs
- WS2007HttpBindingElement.cs
- NGCPageContentSerializerAsync.cs
- DataBinding.cs
- QilNode.cs
- PartialArray.cs
- ColorEditor.cs
- SecurityAlgorithmSuiteConverter.cs
- TypedReference.cs
- Color.cs
- HtmlControl.cs
- SystemException.cs
- BuildProviderCollection.cs
- DependencySource.cs
- AttachmentService.cs