Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Annotations / Storage / StoreContentChangedEventArgs.cs / 1 / StoreContentChangedEventArgs.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The AnnotationStore.StoreContentChanged event is generated when any // changes are made to an annotation in an AnnotationStore. // // File contains the StoreContentChangedEventArgs class, the // AnnotationStoreEnum and the StoreContentChangedEventHandler delegate. // Spec: http://team/sites/ag/Specifications/CAF%20Storage%20Spec.doc // // History: // 07/10/2003: rruiz: Created (split from AnnotationStore.cs file). // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Xml; namespace System.Windows.Annotations.Storage { ////// Event handler delegate for AnnotationUpdated event. Listeners for /// this event must supply a delegate with this signature. /// /// AnnotationStore in which the change took place /// the event data public delegate void StoreContentChangedEventHandler(object sender, StoreContentChangedEventArgs e); ////// Possible actions performed on an IAnnotation in an AnnotationStore. /// public enum StoreContentAction { ////// Annotation was added to the store /// Added, ////// Annotation was deleted from the store /// Deleted } ////// The AnnotationUpdated event is generated when any changes are made /// to an annotation in an AnnotationStore. An instance of this class /// specifies the action that was taken and the IAnnotation that was /// acted upon. /// public class StoreContentChangedEventArgs : System.EventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of AnnotationUpdatedEventArgs with the /// specified action and annotation. /// /// the action that was performed on an annotation /// the annotation that was updated public StoreContentChangedEventArgs(StoreContentAction action, Annotation annotation) { if (annotation == null) throw new ArgumentNullException("annotation"); _action = action; _annotation = annotation; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Returns the IAnnotation that was updated. /// public Annotation Annotation { get { return _annotation; } } ////// Returns the action that was performed on the annotation. /// public StoreContentAction Action { get { return _action; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //----------------------------------------------------- //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private StoreContentAction _action; // action taken on the annotation private Annotation _annotation; // annotation that was updated #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The AnnotationStore.StoreContentChanged event is generated when any // changes are made to an annotation in an AnnotationStore. // // File contains the StoreContentChangedEventArgs class, the // AnnotationStoreEnum and the StoreContentChangedEventHandler delegate. // Spec: http://team/sites/ag/Specifications/CAF%20Storage%20Spec.doc // // History: // 07/10/2003: rruiz: Created (split from AnnotationStore.cs file). // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Xml; namespace System.Windows.Annotations.Storage { ////// Event handler delegate for AnnotationUpdated event. Listeners for /// this event must supply a delegate with this signature. /// /// AnnotationStore in which the change took place /// the event data public delegate void StoreContentChangedEventHandler(object sender, StoreContentChangedEventArgs e); ////// Possible actions performed on an IAnnotation in an AnnotationStore. /// public enum StoreContentAction { ////// Annotation was added to the store /// Added, ////// Annotation was deleted from the store /// Deleted } ////// The AnnotationUpdated event is generated when any changes are made /// to an annotation in an AnnotationStore. An instance of this class /// specifies the action that was taken and the IAnnotation that was /// acted upon. /// public class StoreContentChangedEventArgs : System.EventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of AnnotationUpdatedEventArgs with the /// specified action and annotation. /// /// the action that was performed on an annotation /// the annotation that was updated public StoreContentChangedEventArgs(StoreContentAction action, Annotation annotation) { if (annotation == null) throw new ArgumentNullException("annotation"); _action = action; _annotation = annotation; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Returns the IAnnotation that was updated. /// public Annotation Annotation { get { return _annotation; } } ////// Returns the action that was performed on the annotation. /// public StoreContentAction Action { get { return _action; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //----------------------------------------------------- //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private StoreContentAction _action; // action taken on the annotation private Annotation _annotation; // annotation that was updated #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
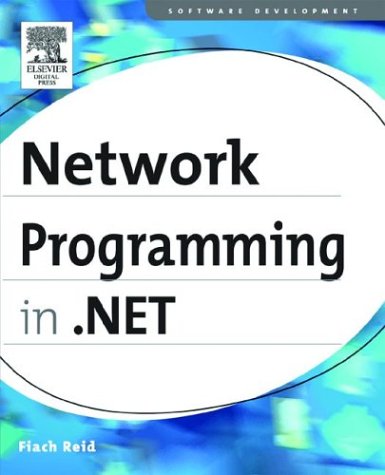
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewAccessibleObject.cs
- IntranetCredentialPolicy.cs
- DataGridViewCellEventArgs.cs
- FormsAuthenticationCredentials.cs
- NameValueConfigurationElement.cs
- DataGridViewCellMouseEventArgs.cs
- CodeArrayIndexerExpression.cs
- MessageSmuggler.cs
- DesignerActionItem.cs
- HttpHeaderCollection.cs
- SslStreamSecurityBindingElement.cs
- ListViewItem.cs
- UdpDuplexChannel.cs
- RoleManagerModule.cs
- RenameRuleObjectDialog.Designer.cs
- Vector3DCollectionConverter.cs
- URIFormatException.cs
- GuidConverter.cs
- TableLayoutCellPaintEventArgs.cs
- RpcResponse.cs
- DataControlCommands.cs
- Privilege.cs
- TextTreeTextElementNode.cs
- ActivityTypeDesigner.xaml.cs
- XamlTypeMapper.cs
- EventLogTraceListener.cs
- Transform.cs
- SettingsContext.cs
- ReservationCollection.cs
- Material.cs
- EntityProviderFactory.cs
- PolicyStatement.cs
- WebPartConnectionsDisconnectVerb.cs
- ExpandSegmentCollection.cs
- DataColumnMappingCollection.cs
- SupportsPreviewControlAttribute.cs
- Funcletizer.cs
- EntityViewGenerationConstants.cs
- KeyedHashAlgorithm.cs
- ObjectSpanRewriter.cs
- TextSegment.cs
- UserMapPath.cs
- DataBoundControlHelper.cs
- DataSourceView.cs
- TemplateControlBuildProvider.cs
- ECDiffieHellmanPublicKey.cs
- DesignerWidgets.cs
- ToolStripManager.cs
- HintTextConverter.cs
- SignatureToken.cs
- RouteData.cs
- AttributeCollection.cs
- ToolStripPanelRow.cs
- StackOverflowException.cs
- HttpHandlerActionCollection.cs
- MatrixIndependentAnimationStorage.cs
- XmlStringTable.cs
- ByeOperationAsyncResult.cs
- LinkLabelLinkClickedEvent.cs
- SafeNativeMethods.cs
- ConditionCollection.cs
- xdrvalidator.cs
- PersonalizationAdministration.cs
- BlockingCollection.cs
- SiteMapNodeCollection.cs
- DLinqDataModelProvider.cs
- SplitterEvent.cs
- PrintController.cs
- TemplateControlCodeDomTreeGenerator.cs
- EtwTrace.cs
- TextServicesPropertyRanges.cs
- XNodeNavigator.cs
- BaseCollection.cs
- UdpSocket.cs
- HttpFileCollection.cs
- CalendarSelectionChangedEventArgs.cs
- InspectionWorker.cs
- TransformerInfoCollection.cs
- MethodRental.cs
- TextBoxDesigner.cs
- MetaData.cs
- XmlSchemaObject.cs
- sqlcontext.cs
- Stack.cs
- FileChangesMonitor.cs
- HostExecutionContextManager.cs
- RemotingAttributes.cs
- CheckBoxStandardAdapter.cs
- CodeVariableDeclarationStatement.cs
- Timeline.cs
- WeakReadOnlyCollection.cs
- PropertyTabAttribute.cs
- Panel.cs
- ClientData.cs
- RectangleGeometry.cs
- ResolveNameEventArgs.cs
- PageSetupDialog.cs
- RSAPKCS1KeyExchangeFormatter.cs
- DependencyObjectValidator.cs
- Cell.cs