Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Monitoring / system / Diagnosticts / EventLogTraceListener.cs / 1305376 / EventLogTraceListener.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Diagnostics { using System; using System.Security; using System.Security.Permissions; using System.IO; using System.Text; using System.Globalization; using System.Runtime.InteropServices; ////// [HostProtection(SecurityAction.LinkDemand, Synchronization=true)] public sealed class EventLogTraceListener : TraceListener { private EventLog eventLog; private bool nameSet; ///Provides a simple listener for directing tracing or /// debugging output to a ///or to a , such as or /// . /// public EventLogTraceListener() { } ///Initializes a new instance of the ///class without a trace /// listener. /// public EventLogTraceListener(EventLog eventLog) : base((eventLog != null) ? eventLog.Source : string.Empty) { this.eventLog = eventLog; } ///Initializes a new instance of the ///class using the /// specified event log. /// public EventLogTraceListener(string source) { eventLog = new EventLog(); eventLog.Source = source; } ///Initializes a new instance of the ///class using the /// specified source. /// public EventLog EventLog { get { return eventLog; } set { eventLog = value; } } ///Gets or sets the event log to write to. ////// public override string Name { get { if (nameSet == false && eventLog != null) { nameSet = true; base.Name = eventLog.Source; } return base.Name; } set { nameSet = true; base.Name = value; } } ///Gets or sets the /// name of this trace listener. ////// public override void Close() { if (eventLog != null) eventLog.Close(); } ///Closes the text writer so that it no longer receives tracing or /// debugging output. ////// /// protected override void Dispose(bool disposing) { try { if (disposing) { this.Close(); } else { // clean up resources if (eventLog != null) eventLog.Close(); eventLog = null; } } finally { base.Dispose(disposing); } } ////// public override void Write(string message) { if (eventLog != null) eventLog.WriteEntry(message); } ///Writes a message to this instance's event log. ////// public override void WriteLine(string message) { Write(message); } [ ComVisible(false) ] public override void TraceEvent(TraceEventCache eventCache, string source, TraceEventType severity, int id, string format, params object[] args) { if (Filter != null && !Filter.ShouldTrace(eventCache, source, severity, id, format, args)) return; EventInstance data = CreateEventInstance(severity, id); if (args == null) { eventLog.WriteEvent(data, format); } else if(String.IsNullOrEmpty(format)) { string[] strings = new string[args.Length]; for (int i=0; iWrites a message to this instance's event log followed by a line terminator. /// The default line terminator is a carriage return followed by a line feed /// (\r\n). ///ushort.MaxValue) id = ushort.MaxValue; // Ideally we need to pick a value other than '0' as zero is // a commonly used EventId by most applications if (id < ushort.MinValue) id = ushort.MinValue; EventInstance data = new EventInstance(id, 0); if (severity == TraceEventType.Error || severity == TraceEventType.Critical) data.EntryType = EventLogEntryType.Error; else if (severity == TraceEventType.Warning) data.EntryType = EventLogEntryType.Warning; else data.EntryType = EventLogEntryType.Information; return data; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
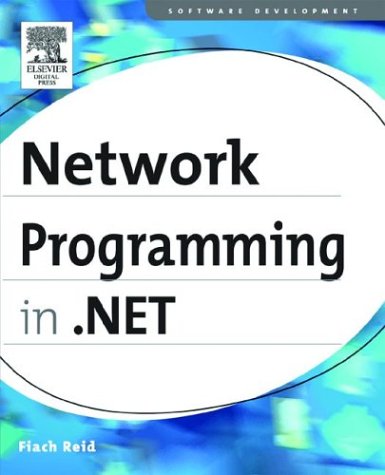
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MeshGeometry3D.cs
- InvokeProviderWrapper.cs
- DependencyObjectCodeDomSerializer.cs
- VectorKeyFrameCollection.cs
- SelectingProviderEventArgs.cs
- EventProviderWriter.cs
- CodeFieldReferenceExpression.cs
- XmlSchemaGroup.cs
- HttpProfileBase.cs
- IndexingContentUnit.cs
- RawStylusInput.cs
- TextTreeRootNode.cs
- Win32Native.cs
- DropShadowBitmapEffect.cs
- ProgressChangedEventArgs.cs
- MenuItem.cs
- TemplateBamlTreeBuilder.cs
- ScriptComponentDescriptor.cs
- ToolstripProfessionalRenderer.cs
- NamespaceDecl.cs
- AssemblyBuilder.cs
- WebPartMinimizeVerb.cs
- cookiecollection.cs
- Metafile.cs
- FileDialog.cs
- SmiRequestExecutor.cs
- MediaElement.cs
- AdvancedBindingEditor.cs
- ToolboxItemWrapper.cs
- XamlReader.cs
- GlyphTypeface.cs
- controlskin.cs
- CachedPathData.cs
- DataGridViewTextBoxEditingControl.cs
- ToolStripRenderEventArgs.cs
- DataTemplateSelector.cs
- SafeEventLogWriteHandle.cs
- X509UI.cs
- HasCopySemanticsAttribute.cs
- DataFormat.cs
- HierarchicalDataSourceIDConverter.cs
- ToolStripItemTextRenderEventArgs.cs
- VideoDrawing.cs
- WebPartDisplayModeCollection.cs
- SapiInterop.cs
- UpWmlMobileTextWriter.cs
- Registration.cs
- RegexRunnerFactory.cs
- ResourceAssociationSetEnd.cs
- HierarchicalDataBoundControl.cs
- CheckBoxStandardAdapter.cs
- MatrixStack.cs
- DocumentSequenceHighlightLayer.cs
- MouseEvent.cs
- ListChangedEventArgs.cs
- StringAnimationUsingKeyFrames.cs
- BuildManagerHost.cs
- LineSegment.cs
- ListBindingConverter.cs
- FormViewPagerRow.cs
- QueryStringConverter.cs
- IIS7UserPrincipal.cs
- AppDomainUnloadedException.cs
- EmptyEnumerator.cs
- UnsupportedPolicyOptionsException.cs
- Imaging.cs
- _LazyAsyncResult.cs
- ImageMap.cs
- DummyDataSource.cs
- ObjectPersistData.cs
- FlagsAttribute.cs
- DataGridViewColumnStateChangedEventArgs.cs
- MembershipPasswordException.cs
- PointAnimationUsingPath.cs
- messageonlyhwndwrapper.cs
- basevalidator.cs
- X509CertificateCollection.cs
- MenuAutomationPeer.cs
- TriggerAction.cs
- DataGridHeaderBorder.cs
- SystemWebSectionGroup.cs
- AnimationException.cs
- RMEnrollmentPage2.cs
- DataGridViewSelectedRowCollection.cs
- UInt32Converter.cs
- LassoSelectionBehavior.cs
- ConfigurationPropertyCollection.cs
- OdbcDataReader.cs
- XPathDocumentBuilder.cs
- XmlDataSourceNodeDescriptor.cs
- PlanCompilerUtil.cs
- _BufferOffsetSize.cs
- ElementUtil.cs
- GraphicsPathIterator.cs
- UIntPtr.cs
- StylusButtonEventArgs.cs
- SubMenuStyleCollectionEditor.cs
- XmlBaseReader.cs
- ControlBuilderAttribute.cs
- HandledMouseEvent.cs