Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Configuration / ConfigurationHelpers.cs / 1 / ConfigurationHelpers.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Configuration { using System; using System.Configuration; using System.Globalization; using System.Reflection; using System.Runtime.CompilerServices; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Diagnostics; using System.ServiceModel.Dispatcher; using System.ServiceModel.Description; using System.Web.Configuration; using System.Security.Permissions; using System.Security; internal static class ConfigurationHelpers { /// Be sure to update UnsafeGetAssociatedBindingCollectionElement if you modify this method internal static BindingCollectionElement GetAssociatedBindingCollectionElement(ContextInformation evaluationContext, string bindingCollectionName) { BindingCollectionElement retVal = null; BindingsSection bindingsSection = (BindingsSection)ConfigurationHelpers.GetAssociatedSection(evaluationContext, ConfigurationStrings.BindingsSectionGroupPath); if (null != bindingsSection) { try { retVal = bindingsSection[bindingCollectionName]; } catch (System.Collections.Generic.KeyNotFoundException) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationErrorsException(SR.GetString(SR.ConfigBindingExtensionNotFound, ConfigurationHelpers.GetBindingsSectionPath(bindingCollectionName)))); } catch (NullReferenceException) // System.Configuration.ConfigurationElement bug { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationErrorsException(SR.GetString(SR.ConfigBindingExtensionNotFound, ConfigurationHelpers.GetBindingsSectionPath(bindingCollectionName)))); } } return retVal; } ////// Critical - uses SecurityCritical method UnsafeGetAssociatedSection which elevates /// /// Be sure to update GetAssociatedBindingCollectionElement if you modify this method [SecurityCritical] internal static BindingCollectionElement UnsafeGetAssociatedBindingCollectionElement(ContextInformation evaluationContext, string bindingCollectionName) { BindingCollectionElement retVal = null; BindingsSection bindingsSection = (BindingsSection)ConfigurationHelpers.UnsafeGetAssociatedSection(evaluationContext, ConfigurationStrings.BindingsSectionGroupPath); if (null != bindingsSection) { try { retVal = bindingsSection[bindingCollectionName]; } catch (System.Collections.Generic.KeyNotFoundException) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationErrorsException(SR.GetString(SR.ConfigBindingExtensionNotFound, ConfigurationHelpers.GetBindingsSectionPath(bindingCollectionName)))); } catch (NullReferenceException) // System.Configuration.ConfigurationElement bug { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationErrorsException(SR.GetString(SR.ConfigBindingExtensionNotFound, ConfigurationHelpers.GetBindingsSectionPath(bindingCollectionName)))); } } return retVal; } /// Be sure to update UnsafeGetAssociatedSection if you modify this method internal static object GetAssociatedSection(ContextInformation evalContext, string sectionPath) { object retval = null; if (evalContext != null) { retval = evalContext.GetSection(sectionPath); } else { if (ServiceHostingEnvironment.IsHosted) { retval = GetSectionFromWebConfigurationManager(sectionPath); } else { retval = ConfigurationManager.GetSection(sectionPath); } // Trace after call to underlying configuration system to // insure that configuration system is initialized if (DiagnosticUtility.ShouldTraceVerbose) { TraceUtility.TraceEvent(System.Diagnostics.TraceEventType.Verbose, TraceCode.GetConfigurationSection, new StringTraceRecord("ConfigurationSection", sectionPath), null, null); } } if (retval == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationErrorsException(SR.GetString(SR.ConfigSectionNotFound, sectionPath))); } return retval; } ////// Critical - uses SecurityCritical methods which elevate /// /// Be sure to update GetAssociatedSection if you modify this method [SecurityCritical] internal static object UnsafeGetAssociatedSection(ContextInformation evalContext, string sectionPath) { object retval = null; if (evalContext != null) { retval = UnsafeGetSectionFromContext(evalContext, sectionPath); } else { if (ServiceHostingEnvironment.IsHosted) { if (ServiceHostingEnvironment.CurrentVirtualPath != null) { retval = UnsafeGetSectionFromWebConfigurationManager(sectionPath, ServiceHostingEnvironment.CurrentVirtualPath); } else { retval = UnsafeGetSectionFromWebConfigurationManager(sectionPath); } } else { retval = UnsafeGetSectionFromConfigurationManager(sectionPath); } // Trace after call to underlying configuration system to // insure that configuration system is initialized if (DiagnosticUtility.ShouldTraceVerbose) { TraceUtility.TraceEvent(System.Diagnostics.TraceEventType.Verbose, TraceCode.GetConfigurationSection, new StringTraceRecord("ConfigurationSection", sectionPath), null, null); } } if (retval == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationErrorsException(SR.GetString(SR.ConfigSectionNotFound, sectionPath))); } return retval; } /// Be sure to update UnsafeGetBindingCollectionElement if you modify this method internal static BindingCollectionElement GetBindingCollectionElement(string bindingCollectionName) { return GetAssociatedBindingCollectionElement(null, bindingCollectionName); } ////// Critical - uses SecurityCritical method UnsafeGetAssociatedBindingCollectionElement which elevates /// /// Be sure to update GetBindingCollectionElement if you modify this method [SecurityCritical] internal static BindingCollectionElement UnsafeGetBindingCollectionElement(string bindingCollectionName) { return UnsafeGetAssociatedBindingCollectionElement(null, bindingCollectionName); } internal static string GetBindingsSectionPath(string sectionName) { return string.Concat(ConfigurationStrings.BindingsSectionGroupPath, "/", sectionName); } /// Be sure to update UnsafeGetSection if you modify this method internal static object GetSection(string sectionPath) { return GetAssociatedSection(null, sectionPath); } ////// Critical - uses SecurityCritical method UnsafeGetAssociatedSection which elevates /// /// Be sure to update GetSection if you modify this method [SecurityCritical] internal static object UnsafeGetSection(string sectionPath) { return UnsafeGetAssociatedSection(null, sectionPath); } /// Be sure to update UnsafeGetSectionFromWebConfigurationManager if you modify this method [MethodImpl(MethodImplOptions.NoInlining)] static object GetSectionFromWebConfigurationManager(string sectionPath) { if (ServiceHostingEnvironment.CurrentVirtualPath != null) { return WebConfigurationManager.GetSection(sectionPath, ServiceHostingEnvironment.CurrentVirtualPath); } return WebConfigurationManager.GetSection(sectionPath); } ////// Critical - Asserts ConfigurationPermission in order to fetch config from WebConfigurationManager /// caller must guard return value /// /// Be sure to update GetSectionFromWebConfigurationManager if you modify this method [SecurityCritical] [ConfigurationPermission(SecurityAction.Assert, Unrestricted = true)] [MethodImpl(MethodImplOptions.NoInlining)] internal static object UnsafeGetSectionFromWebConfigurationManager(string sectionPath) { return WebConfigurationManager.GetSection(sectionPath); } ////// Critical - Asserts ConfigurationPermission in order to fetch config from ConfigurationManager /// caller must guard return value /// [SecurityCritical] [ConfigurationPermission(SecurityAction.Assert, Unrestricted = true)] static object UnsafeGetSectionFromConfigurationManager(string sectionPath) { return ConfigurationManager.GetSection(sectionPath); } ////// Critical - Asserts ConfigurationPermission in order to fetch config from ContextInformation /// caller must guard return value /// [SecurityCritical] [ConfigurationPermission(SecurityAction.Assert, Unrestricted = true)] static object UnsafeGetSectionFromContext(ContextInformation evalContext, string sectionPath) { return evalContext.GetSection(sectionPath); } ////// Critical - Asserts ConfigurationPermission in order to fetch config from WebConfigurationManager /// caller must guard return value /// [SecurityCritical] [ConfigurationPermission(SecurityAction.Assert, Unrestricted = true)] [MethodImpl(MethodImplOptions.NoInlining)] static object UnsafeGetSectionFromWebConfigurationManager(string sectionPath, string virtualPath) { return WebConfigurationManager.GetSection(sectionPath, virtualPath); } internal static string GetSectionPath(string sectionName) { return string.Concat(ConfigurationStrings.SectionGroupName, "/", sectionName); } ////// Critical - calls SetIsPresentWithAssert which elevates in order to set a property /// caller must guard ConfigurationElement parameter, ie only pass 'this' /// [SecurityCritical] internal static void SetIsPresent(ConfigurationElement element) { // Work around for VSW 578830: ConfigurationElements that override DeserializeElement cannot set ElementInformation.IsPresent PropertyInfo elementPresent = element.GetType().GetProperty("ElementPresent", BindingFlags.Instance | BindingFlags.NonPublic); SetIsPresentWithAssert(elementPresent, element, true); } ////// Critical - Asserts ReflectionPermission(MemberAccess) in order to set a private member in the ConfigurationElement /// caller must guard parameters /// [SecurityCritical] [ReflectionPermission(SecurityAction.Assert, MemberAccess=true)] static void SetIsPresentWithAssert(PropertyInfo elementPresent, ConfigurationElement element, bool value) { elementPresent.SetValue(element, value, null); } internal static ContextInformation GetEvaluationContext(IConfigurationContextProviderInternal provider) { if (provider != null) { try { return provider.GetEvaluationContext(); } catch (ConfigurationErrorsException) { } } return null; } ////// RequiresReview - the return value will be used for a security decision. if in doubt about the return value, it /// is safe to return null (caller will assume the worst case from a security perspective). /// internal static ContextInformation GetOriginalEvaluationContext(IConfigurationContextProviderInternal provider) { if (provider != null) { // provider may not need this try/catch, but it doesn't hurt to do it try { return provider.GetOriginalEvaluationContext(); } catch (ConfigurationErrorsException) { } } return null; } } interface IConfigurationContextProviderInternal { ////// return the current ContextInformation (the protected property ConfigurationElement.EvaluationContext) /// this may throw ConfigurationErrorsException, caller should guard (see ConfigurationHelpers.GetEvaluationContext) /// ///result of ConfigurationElement.EvaluationContext ContextInformation GetEvaluationContext(); ////// return the ContextInformation that was present when the ConfigurationElement was first deserialized. /// if Reset was called, this will be the value of parent.GetOriginalEvaluationContext() /// if Reset was not called, this will be the value of this.GetEvaluationContext() /// ///result of parent's ConfigurationElement.EvaluationContext ////// RequiresReview - the return value will be used for a security decision. if in doubt about the return value, it /// is safe (from a security perspective) to return null (caller will assume the worst case). /// ContextInformation GetOriginalEvaluationContext(); } ////// Critical -- stores information used in a security decision /// [SecurityCritical(SecurityCriticalScope.Everything)] struct EvaluationContextHelper { bool reset; ContextInformation inheritedContext; internal void OnReset(ConfigurationElement parent) { this.reset = true; this.inheritedContext = ConfigurationHelpers.GetOriginalEvaluationContext(parent as IConfigurationContextProviderInternal); } internal ContextInformation GetOriginalContext(IConfigurationContextProviderInternal owner) { if (this.reset) { // if reset, inherited context is authoritative, even if null return this.inheritedContext; } else { // otherwise use current return ConfigurationHelpers.GetEvaluationContext(owner); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
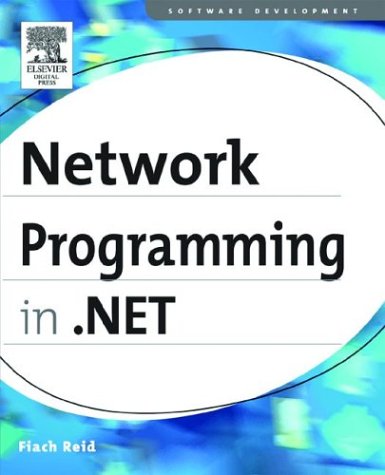
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIElement.cs
- ElementNotEnabledException.cs
- CryptoStream.cs
- TypeLoadException.cs
- Encoder.cs
- PathTooLongException.cs
- HttpModuleActionCollection.cs
- UserPreferenceChangedEventArgs.cs
- LoginViewDesigner.cs
- FromRequest.cs
- Size3DConverter.cs
- SqlColumnizer.cs
- CodeSnippetStatement.cs
- FileDialogCustomPlace.cs
- MethodCallTranslator.cs
- UInt16Converter.cs
- SelectionEditor.cs
- UserPersonalizationStateInfo.cs
- CodeCatchClause.cs
- SizeConverter.cs
- WrappedKeySecurityTokenParameters.cs
- PrefixQName.cs
- BaseDataList.cs
- XmlNodeChangedEventManager.cs
- OdbcPermission.cs
- DataServices.cs
- PanelStyle.cs
- QueryContext.cs
- BaseCollection.cs
- WindowsGraphics2.cs
- WizardForm.cs
- SelectorItemAutomationPeer.cs
- ParseNumbers.cs
- SyndicationDeserializer.cs
- EditorPartChrome.cs
- XamlUtilities.cs
- IDReferencePropertyAttribute.cs
- ComplexPropertyEntry.cs
- ThemeInfoAttribute.cs
- DoubleAnimationBase.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- CompositeCollection.cs
- TableColumnCollection.cs
- QilReplaceVisitor.cs
- TypeExtensions.cs
- ListControlConvertEventArgs.cs
- EntityDataSourceContainerNameItem.cs
- ReferenceConverter.cs
- GenericTransactionFlowAttribute.cs
- SqlComparer.cs
- FixedSOMPageElement.cs
- TextViewSelectionProcessor.cs
- TextElementCollectionHelper.cs
- RemotingConfigParser.cs
- XmlSchemaComplexContentRestriction.cs
- DynamicDocumentPaginator.cs
- DataGridViewTextBoxEditingControl.cs
- MetadataItem.cs
- Graphics.cs
- UriExt.cs
- _ConnectionGroup.cs
- util.cs
- TableHeaderCell.cs
- SapiAttributeParser.cs
- SettingsProviderCollection.cs
- BulletedListEventArgs.cs
- DataGridViewRowConverter.cs
- PathTooLongException.cs
- SqlRecordBuffer.cs
- GridEntry.cs
- HtmlTextArea.cs
- ICspAsymmetricAlgorithm.cs
- HijriCalendar.cs
- CuspData.cs
- CellTreeSimplifier.cs
- PasswordRecovery.cs
- InstalledFontCollection.cs
- DataGridViewComboBoxColumnDesigner.cs
- DataViewManagerListItemTypeDescriptor.cs
- SQLBytes.cs
- ModelServiceImpl.cs
- VerticalAlignConverter.cs
- AuthenticationModuleElementCollection.cs
- SurrogateSelector.cs
- GcSettings.cs
- SubordinateTransaction.cs
- DetailsViewDeleteEventArgs.cs
- Unit.cs
- ConvertTextFrag.cs
- storepermission.cs
- xdrvalidator.cs
- DispatcherOperation.cs
- dsa.cs
- SizeAnimationClockResource.cs
- Missing.cs
- XamlSerializer.cs
- ValueOfAction.cs
- CryptoConfig.cs
- FormClosedEvent.cs
- OdbcRowUpdatingEvent.cs