Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / MetadataItem.cs / 1305376 / MetadataItem.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Text; using System.Xml.Serialization; using System.Xml.Schema; using System.Xml; using System.Globalization; namespace System.Data.Metadata.Edm { ////// Represents the base item class for all the metadata /// public abstract partial class MetadataItem { #region Constructors ////// Implementing this internal constructor so that this class can't be derived /// outside this assembly /// internal MetadataItem() { } internal MetadataItem(MetadataFlags flags) { _flags = flags; } #endregion #region Fields [Flags] internal enum MetadataFlags { // GlobalItem None = 0, // DataSpace flags are off by one so that zero can be the uninitialized state CSpace = 1, // (1 << 0) OSpace = 2, // (1 << 1) OCSpace = 3, // CSpace | OSpace SSpace = 4, // (1 << 2) CSSpace = 5, // CSpace | SSpace DataSpace = OSpace | CSpace | SSpace | OCSpace | CSSpace, // MetadataItem Readonly = (1 << 3), // EdmType IsAbstract = (1 << 4), // FunctionParameter In = (1 << 9), Out = (1 << 10), InOut = In | Out, ReturnValue = (1 << 11), ParameterMode = (In | Out | InOut | ReturnValue), } private MetadataFlags _flags; private object _flagsLock = new object(); private MetadataCollection_itemAttributes; private Documentation _documentation; #endregion #region Properties /// /// Returns the kind of the type /// public abstract BuiltInTypeKind BuiltInTypeKind { get; } ////// List of item attributes on this type /// [MetadataProperty(BuiltInTypeKind.MetadataProperty, true)] public ReadOnlyMetadataCollectionMetadataProperties { get { if (null == _itemAttributes) { MetadataPropertyCollection itemAttributes = new MetadataPropertyCollection(this); if (IsReadOnly) { itemAttributes.SetReadOnly(); } System.Threading.Interlocked.CompareExchange >( ref _itemAttributes, itemAttributes, null); } return _itemAttributes.AsReadOnlyMetadataCollection(); } } /// /// List of item attributes on this type /// internal MetadataCollectionRawMetadataProperties { get { return _itemAttributes; } } /// /// List of item attributes on this type /// public Documentation Documentation { get { return _documentation; } set { _documentation = value; } } ////// Identity of the item /// internal abstract String Identity { get; } ////// Just checks for identities to be equal /// /// ///internal virtual bool EdmEquals(MetadataItem item) { return ((null != item) && ((this == item) || // same reference (this.BuiltInTypeKind == item.BuiltInTypeKind && this.Identity == item.Identity))); } /// /// Returns true if this item is not-changeable. Otherwise returns false. /// internal bool IsReadOnly { get { return GetFlag(MetadataFlags.Readonly); } } #endregion #region Methods ////// Validates the types and sets the readOnly property to true. Once the type is set to readOnly, /// it can never be changed. /// internal virtual void SetReadOnly() { if (!IsReadOnly) { if (null != _itemAttributes) { _itemAttributes.SetReadOnly(); } SetFlag(MetadataFlags.Readonly, true); } } ////// Builds identity string for this item. By default, the method calls the identity property. /// /// internal virtual void BuildIdentity(StringBuilder builder) { builder.Append(this.Identity); } ////// Adds the given metadata property to the metadata property collection /// /// internal void AddMetadataProperties(ListmetadataProperties) { this.MetadataProperties.Source.AtomicAddRange(metadataProperties); } #endregion #region MetadataFlags internal DataSpace GetDataSpace() { switch (_flags & MetadataFlags.DataSpace) { default: return (DataSpace)(-1); case MetadataFlags.CSpace: return DataSpace.CSpace; case MetadataFlags.OSpace: return DataSpace.OSpace; case MetadataFlags.SSpace: return DataSpace.SSpace; case MetadataFlags.OCSpace: return DataSpace.OCSpace; case MetadataFlags.CSSpace: return DataSpace.CSSpace; } } internal void SetDataSpace(DataSpace space) { _flags = (_flags & ~MetadataFlags.DataSpace) | (MetadataFlags.DataSpace & Convert(space)); } private static MetadataFlags Convert(DataSpace space) { switch (space) { default: return MetadataFlags.None; // invalid case DataSpace.CSpace: return MetadataFlags.CSpace; case DataSpace.OSpace: return MetadataFlags.OSpace; case DataSpace.SSpace: return MetadataFlags.SSpace; case DataSpace.OCSpace: return MetadataFlags.OCSpace; case DataSpace.CSSpace: return MetadataFlags.CSSpace; } } internal ParameterMode GetParameterMode() { switch (_flags & MetadataFlags.ParameterMode) { default: return (ParameterMode)(-1); // invalid case MetadataFlags.In: return ParameterMode.In; case MetadataFlags.Out: return ParameterMode.Out; case MetadataFlags.InOut: return ParameterMode.InOut; case MetadataFlags.ReturnValue: return ParameterMode.ReturnValue; } } internal void SetParameterMode(ParameterMode mode) { _flags = (_flags & ~MetadataFlags.ParameterMode) | (MetadataFlags.ParameterMode & Convert(mode)); } private static MetadataFlags Convert(ParameterMode mode) { switch (mode) { default: return MetadataFlags.ParameterMode; // invalid case ParameterMode.In: return MetadataFlags.In; case ParameterMode.Out: return MetadataFlags.Out; case ParameterMode.InOut: return MetadataFlags.InOut; case ParameterMode.ReturnValue: return MetadataFlags.ReturnValue; } } internal bool GetFlag(MetadataFlags flag) { return (flag == (_flags & flag)); } internal void SetFlag(MetadataFlags flag, bool value) { if ((flag & MetadataFlags.Readonly) == MetadataFlags.Readonly) { Debug.Assert(System.Convert.ToInt32(flag & ~MetadataFlags.Readonly,CultureInfo.InvariantCulture) == 0, "SetFlag() invoked with Readonly and additional flags."); } lock (_flagsLock) { // an attempt to set the ReadOnly flag on a MetadataItem that is already read-only // is a no-op // if (IsReadOnly && ((flag & MetadataFlags.Readonly) == MetadataFlags.Readonly)) { return; } Util.ThrowIfReadOnly(this); if (value) { _flags |= flag; } else { _flags &= ~flag; } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Text; using System.Xml.Serialization; using System.Xml.Schema; using System.Xml; using System.Globalization; namespace System.Data.Metadata.Edm { ////// Represents the base item class for all the metadata /// public abstract partial class MetadataItem { #region Constructors ////// Implementing this internal constructor so that this class can't be derived /// outside this assembly /// internal MetadataItem() { } internal MetadataItem(MetadataFlags flags) { _flags = flags; } #endregion #region Fields [Flags] internal enum MetadataFlags { // GlobalItem None = 0, // DataSpace flags are off by one so that zero can be the uninitialized state CSpace = 1, // (1 << 0) OSpace = 2, // (1 << 1) OCSpace = 3, // CSpace | OSpace SSpace = 4, // (1 << 2) CSSpace = 5, // CSpace | SSpace DataSpace = OSpace | CSpace | SSpace | OCSpace | CSSpace, // MetadataItem Readonly = (1 << 3), // EdmType IsAbstract = (1 << 4), // FunctionParameter In = (1 << 9), Out = (1 << 10), InOut = In | Out, ReturnValue = (1 << 11), ParameterMode = (In | Out | InOut | ReturnValue), } private MetadataFlags _flags; private object _flagsLock = new object(); private MetadataCollection_itemAttributes; private Documentation _documentation; #endregion #region Properties /// /// Returns the kind of the type /// public abstract BuiltInTypeKind BuiltInTypeKind { get; } ////// List of item attributes on this type /// [MetadataProperty(BuiltInTypeKind.MetadataProperty, true)] public ReadOnlyMetadataCollectionMetadataProperties { get { if (null == _itemAttributes) { MetadataPropertyCollection itemAttributes = new MetadataPropertyCollection(this); if (IsReadOnly) { itemAttributes.SetReadOnly(); } System.Threading.Interlocked.CompareExchange >( ref _itemAttributes, itemAttributes, null); } return _itemAttributes.AsReadOnlyMetadataCollection(); } } /// /// List of item attributes on this type /// internal MetadataCollectionRawMetadataProperties { get { return _itemAttributes; } } /// /// List of item attributes on this type /// public Documentation Documentation { get { return _documentation; } set { _documentation = value; } } ////// Identity of the item /// internal abstract String Identity { get; } ////// Just checks for identities to be equal /// /// ///internal virtual bool EdmEquals(MetadataItem item) { return ((null != item) && ((this == item) || // same reference (this.BuiltInTypeKind == item.BuiltInTypeKind && this.Identity == item.Identity))); } /// /// Returns true if this item is not-changeable. Otherwise returns false. /// internal bool IsReadOnly { get { return GetFlag(MetadataFlags.Readonly); } } #endregion #region Methods ////// Validates the types and sets the readOnly property to true. Once the type is set to readOnly, /// it can never be changed. /// internal virtual void SetReadOnly() { if (!IsReadOnly) { if (null != _itemAttributes) { _itemAttributes.SetReadOnly(); } SetFlag(MetadataFlags.Readonly, true); } } ////// Builds identity string for this item. By default, the method calls the identity property. /// /// internal virtual void BuildIdentity(StringBuilder builder) { builder.Append(this.Identity); } ////// Adds the given metadata property to the metadata property collection /// /// internal void AddMetadataProperties(ListmetadataProperties) { this.MetadataProperties.Source.AtomicAddRange(metadataProperties); } #endregion #region MetadataFlags internal DataSpace GetDataSpace() { switch (_flags & MetadataFlags.DataSpace) { default: return (DataSpace)(-1); case MetadataFlags.CSpace: return DataSpace.CSpace; case MetadataFlags.OSpace: return DataSpace.OSpace; case MetadataFlags.SSpace: return DataSpace.SSpace; case MetadataFlags.OCSpace: return DataSpace.OCSpace; case MetadataFlags.CSSpace: return DataSpace.CSSpace; } } internal void SetDataSpace(DataSpace space) { _flags = (_flags & ~MetadataFlags.DataSpace) | (MetadataFlags.DataSpace & Convert(space)); } private static MetadataFlags Convert(DataSpace space) { switch (space) { default: return MetadataFlags.None; // invalid case DataSpace.CSpace: return MetadataFlags.CSpace; case DataSpace.OSpace: return MetadataFlags.OSpace; case DataSpace.SSpace: return MetadataFlags.SSpace; case DataSpace.OCSpace: return MetadataFlags.OCSpace; case DataSpace.CSSpace: return MetadataFlags.CSSpace; } } internal ParameterMode GetParameterMode() { switch (_flags & MetadataFlags.ParameterMode) { default: return (ParameterMode)(-1); // invalid case MetadataFlags.In: return ParameterMode.In; case MetadataFlags.Out: return ParameterMode.Out; case MetadataFlags.InOut: return ParameterMode.InOut; case MetadataFlags.ReturnValue: return ParameterMode.ReturnValue; } } internal void SetParameterMode(ParameterMode mode) { _flags = (_flags & ~MetadataFlags.ParameterMode) | (MetadataFlags.ParameterMode & Convert(mode)); } private static MetadataFlags Convert(ParameterMode mode) { switch (mode) { default: return MetadataFlags.ParameterMode; // invalid case ParameterMode.In: return MetadataFlags.In; case ParameterMode.Out: return MetadataFlags.Out; case ParameterMode.InOut: return MetadataFlags.InOut; case ParameterMode.ReturnValue: return MetadataFlags.ReturnValue; } } internal bool GetFlag(MetadataFlags flag) { return (flag == (_flags & flag)); } internal void SetFlag(MetadataFlags flag, bool value) { if ((flag & MetadataFlags.Readonly) == MetadataFlags.Readonly) { Debug.Assert(System.Convert.ToInt32(flag & ~MetadataFlags.Readonly,CultureInfo.InvariantCulture) == 0, "SetFlag() invoked with Readonly and additional flags."); } lock (_flagsLock) { // an attempt to set the ReadOnly flag on a MetadataItem that is already read-only // is a no-op // if (IsReadOnly && ((flag & MetadataFlags.Readonly) == MetadataFlags.Readonly)) { return; } Util.ThrowIfReadOnly(this); if (value) { _flags |= flag; } else { _flags &= ~flag; } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
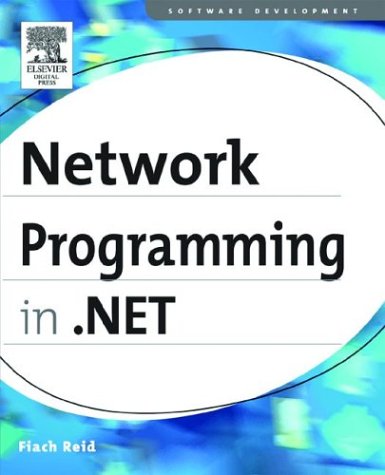
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InfiniteIntConverter.cs
- ContractMapping.cs
- StructuralCache.cs
- PassportAuthenticationModule.cs
- EventLogger.cs
- TraceListener.cs
- AssemblyName.cs
- UrlPath.cs
- ImportCatalogPart.cs
- SqlBuilder.cs
- PersonalizationProviderCollection.cs
- InternalConfigHost.cs
- ListSurrogate.cs
- LayoutEvent.cs
- ScriptReferenceBase.cs
- EntityClientCacheEntry.cs
- LogRecordSequence.cs
- WCFBuildProvider.cs
- EntityDataSourceDesigner.cs
- ValidationResult.cs
- SafePEFileHandle.cs
- OleDbError.cs
- TreeBuilderBamlTranslator.cs
- TableItemStyle.cs
- returneventsaver.cs
- Effect.cs
- SevenBitStream.cs
- ObjectListField.cs
- ExceptionHandler.cs
- XpsFont.cs
- FontUnit.cs
- LayoutEngine.cs
- MultiTrigger.cs
- CacheEntry.cs
- SafePEFileHandle.cs
- SchemaLookupTable.cs
- RepeaterCommandEventArgs.cs
- EditorResources.cs
- OutputScopeManager.cs
- Int32.cs
- EventTrigger.cs
- RuntimeVariableList.cs
- MenuItemStyle.cs
- MethodBuilder.cs
- MSAAEventDispatcher.cs
- Parameter.cs
- Translator.cs
- ProcessInputEventArgs.cs
- DataViewManagerListItemTypeDescriptor.cs
- ScriptReferenceEventArgs.cs
- SoapEnvelopeProcessingElement.cs
- AttachmentService.cs
- OperationContext.cs
- CoreSwitches.cs
- XmlDataDocument.cs
- ImageMap.cs
- _BaseOverlappedAsyncResult.cs
- MessageQueueKey.cs
- HorizontalAlignConverter.cs
- SR.cs
- DataTableExtensions.cs
- ContentElement.cs
- SplitterEvent.cs
- SrgsDocument.cs
- IPCCacheManager.cs
- Button.cs
- DBSchemaRow.cs
- RegularExpressionValidator.cs
- SourceFileBuildProvider.cs
- IntSecurity.cs
- itemelement.cs
- ProxyWebPartManager.cs
- BridgeDataReader.cs
- ConfigXmlCDataSection.cs
- SessionParameter.cs
- NativeMethods.cs
- MailSettingsSection.cs
- ProcessModelInfo.cs
- ClientSponsor.cs
- IntSecurity.cs
- DrawingGroup.cs
- LocalizedNameDescriptionPair.cs
- ActivityLocationReferenceEnvironment.cs
- MetadataException.cs
- ClearTypeHintValidation.cs
- NopReturnReader.cs
- SystemIcons.cs
- ImageDrawing.cs
- Lasso.cs
- BamlTreeMap.cs
- ContentFilePart.cs
- PagedControl.cs
- Encoder.cs
- WindowsComboBox.cs
- SignatureHelper.cs
- ValidationSettings.cs
- RegisteredExpandoAttribute.cs
- LinearKeyFrames.cs
- Properties.cs
- NTAccount.cs