Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Input / ProcessInputEventArgs.cs / 1 / ProcessInputEventArgs.cs
using System; using System.Security.Permissions; using System.Security; using MS.Internal; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// Provides access to the input manager's staging area. /// ////// An instance of this class, or a derived class, is passed to the /// handlers of the following events: /// public class ProcessInputEventArgs : NotifyInputEventArgs { // Only we can make these. Note that we cache and resuse instances. internal ProcessInputEventArgs() {} //////
///- ///
////// - ///
////// /// Critical - calls a critical method base.Reset /// [SecurityCritical] internal override void Reset(StagingAreaInputItem input, InputManager inputManager) { _allowAccessToStagingArea = true; base.Reset(input, inputManager); } ////// Pushes an input event onto the top of the staging area. /// /// /// The input event to place on the staging area. This may not /// be null, and may not already exist in the staging area. /// /// /// An existing staging area item to promote the state from. /// ////// The staging area input item that wraps the specified input. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls a critical method ( PushInput) /// PublicOK - there is a link demand for public callers. /// [SecurityCritical ] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] public StagingAreaInputItem PushInput(InputEventArgs input, StagingAreaInputItem promote) // Note: this should be a bool, and always use the InputItem available on these args. { if(!_allowAccessToStagingArea) { throw new InvalidOperationException(SR.Get(SRID.NotAllowedToAccessStagingArea)); } return this.UnsecureInputManager.PushInput(input, promote); } ////// Pushes an input event onto the top of the staging area. /// /// /// The input event to place on the staging area. This may not /// be null, and may not already exist in the staging area. /// ////// The specified staging area input item. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls a critical method ( PushInput) /// PublicOK - there is a link demand for public callers. /// [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] public StagingAreaInputItem PushInput(StagingAreaInputItem input) { if(!_allowAccessToStagingArea) { throw new InvalidOperationException(SR.Get(SRID.NotAllowedToAccessStagingArea)); } return this.UnsecureInputManager.PushInput(input); } ////// Pops off the input event on the top of the staging area. /// ////// The input event that was on the top of the staging area. /// This can be null, if the staging area was empty. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls a critical function ( InputManager.PopInput) /// PublicOK - there is a demand. /// [SecurityCritical] public StagingAreaInputItem PopInput() { SecurityHelper.DemandUnrestrictedUIPermission(); if(!_allowAccessToStagingArea) { throw new InvalidOperationException(SR.Get(SRID.NotAllowedToAccessStagingArea)); } return this.UnsecureInputManager.PopInput(); } ////// Returns the input event on the top of the staging area. /// ////// The input event that is on the top of the staging area. /// This can be null, if the staging area is empty. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - accesses UnsecureInputManager /// PublicOK - there is a demand. /// [SecurityCritical] public StagingAreaInputItem PeekInput() { SecurityHelper.DemandUnrestrictedUIPermission(); if(!_allowAccessToStagingArea) { throw new InvalidOperationException(SR.Get(SRID.NotAllowedToAccessStagingArea)); } return this.UnsecureInputManager.PeekInput(); } private bool _allowAccessToStagingArea; } ////// Delegate type for handles of events that use /// public delegate void ProcessInputEventHandler(object sender, ProcessInputEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Security.Permissions; using System.Security; using MS.Internal; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ///. /// /// Provides access to the input manager's staging area. /// ////// An instance of this class, or a derived class, is passed to the /// handlers of the following events: /// public class ProcessInputEventArgs : NotifyInputEventArgs { // Only we can make these. Note that we cache and resuse instances. internal ProcessInputEventArgs() {} //////
///- ///
////// - ///
////// /// Critical - calls a critical method base.Reset /// [SecurityCritical] internal override void Reset(StagingAreaInputItem input, InputManager inputManager) { _allowAccessToStagingArea = true; base.Reset(input, inputManager); } ////// Pushes an input event onto the top of the staging area. /// /// /// The input event to place on the staging area. This may not /// be null, and may not already exist in the staging area. /// /// /// An existing staging area item to promote the state from. /// ////// The staging area input item that wraps the specified input. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls a critical method ( PushInput) /// PublicOK - there is a link demand for public callers. /// [SecurityCritical ] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] public StagingAreaInputItem PushInput(InputEventArgs input, StagingAreaInputItem promote) // Note: this should be a bool, and always use the InputItem available on these args. { if(!_allowAccessToStagingArea) { throw new InvalidOperationException(SR.Get(SRID.NotAllowedToAccessStagingArea)); } return this.UnsecureInputManager.PushInput(input, promote); } ////// Pushes an input event onto the top of the staging area. /// /// /// The input event to place on the staging area. This may not /// be null, and may not already exist in the staging area. /// ////// The specified staging area input item. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls a critical method ( PushInput) /// PublicOK - there is a link demand for public callers. /// [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] public StagingAreaInputItem PushInput(StagingAreaInputItem input) { if(!_allowAccessToStagingArea) { throw new InvalidOperationException(SR.Get(SRID.NotAllowedToAccessStagingArea)); } return this.UnsecureInputManager.PushInput(input); } ////// Pops off the input event on the top of the staging area. /// ////// The input event that was on the top of the staging area. /// This can be null, if the staging area was empty. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - calls a critical function ( InputManager.PopInput) /// PublicOK - there is a demand. /// [SecurityCritical] public StagingAreaInputItem PopInput() { SecurityHelper.DemandUnrestrictedUIPermission(); if(!_allowAccessToStagingArea) { throw new InvalidOperationException(SR.Get(SRID.NotAllowedToAccessStagingArea)); } return this.UnsecureInputManager.PopInput(); } ////// Returns the input event on the top of the staging area. /// ////// The input event that is on the top of the staging area. /// This can be null, if the staging area is empty. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical - accesses UnsecureInputManager /// PublicOK - there is a demand. /// [SecurityCritical] public StagingAreaInputItem PeekInput() { SecurityHelper.DemandUnrestrictedUIPermission(); if(!_allowAccessToStagingArea) { throw new InvalidOperationException(SR.Get(SRID.NotAllowedToAccessStagingArea)); } return this.UnsecureInputManager.PeekInput(); } private bool _allowAccessToStagingArea; } ////// Delegate type for handles of events that use /// public delegate void ProcessInputEventHandler(object sender, ProcessInputEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.. ///
Link Menu
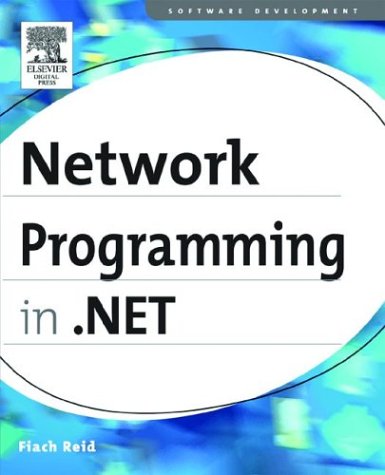
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializationSectionGroup.cs
- PropertyStore.cs
- SafeLocalMemHandle.cs
- ThumbAutomationPeer.cs
- PipelineModuleStepContainer.cs
- FixedFlowMap.cs
- ImmutableObjectAttribute.cs
- EntityDataSourceContainerNameItem.cs
- DomainConstraint.cs
- GroupJoinQueryOperator.cs
- DataGridRow.cs
- ServiceKnownTypeAttribute.cs
- UIElement.cs
- StringUtil.cs
- SelectedDatesCollection.cs
- WsdlHelpGeneratorElement.cs
- Keywords.cs
- UpdateManifestForBrowserApplication.cs
- ImageSourceConverter.cs
- GridViewEditEventArgs.cs
- ClientUtils.cs
- XsltQilFactory.cs
- FacetValues.cs
- FormsAuthenticationUserCollection.cs
- ConnectionStringsExpressionBuilder.cs
- HandlerWithFactory.cs
- BrushMappingModeValidation.cs
- PolicyStatement.cs
- PreviewKeyDownEventArgs.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- NegotiateStream.cs
- ByteAnimation.cs
- StatusBarPanel.cs
- ContentTypeSettingDispatchMessageFormatter.cs
- FontStyleConverter.cs
- CellRelation.cs
- TypeElement.cs
- HtmlTableRowCollection.cs
- OdbcStatementHandle.cs
- GiveFeedbackEvent.cs
- DrawListViewColumnHeaderEventArgs.cs
- CheckBoxFlatAdapter.cs
- ThemeInfoAttribute.cs
- OutputCacheSettingsSection.cs
- StylusShape.cs
- TreeNode.cs
- ListenerElementsCollection.cs
- FrameworkElement.cs
- MissingSatelliteAssemblyException.cs
- SingleAnimationUsingKeyFrames.cs
- TargetFrameworkUtil.cs
- TagPrefixInfo.cs
- CodeMethodMap.cs
- UrlMappingsModule.cs
- LZCodec.cs
- HttpException.cs
- EventBuilder.cs
- WindowsGraphics2.cs
- Decimal.cs
- RegexCompilationInfo.cs
- DataControlField.cs
- TableDetailsRow.cs
- InstanceData.cs
- DecodeHelper.cs
- UserControlAutomationPeer.cs
- DataObject.cs
- BindingManagerDataErrorEventArgs.cs
- MatrixCamera.cs
- SizeConverter.cs
- __ConsoleStream.cs
- TagPrefixAttribute.cs
- CustomErrorsSection.cs
- NavigationExpr.cs
- InvalidOleVariantTypeException.cs
- XmlSerializerNamespaces.cs
- PrivilegedConfigurationManager.cs
- ReadOnlyCollection.cs
- ToolStrip.cs
- SqlClientMetaDataCollectionNames.cs
- XmlObjectSerializerReadContext.cs
- Matrix3D.cs
- DuplicateWaitObjectException.cs
- ReferenceTypeElement.cs
- CustomAttributeSerializer.cs
- ToolStripContainerActionList.cs
- OleDbFactory.cs
- ActivationServices.cs
- ToolStripManager.cs
- AccessKeyManager.cs
- EncryptedKey.cs
- TableLayoutPanelBehavior.cs
- MenuRenderer.cs
- DataGridViewEditingControlShowingEventArgs.cs
- FixedHighlight.cs
- DocumentXmlWriter.cs
- EventEntry.cs
- WebHttpBinding.cs
- WeakHashtable.cs
- SmiContextFactory.cs
- WpfWebRequestHelper.cs