Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Compiler / TypeSystem / TypeProvider.cs / 1305376 / TypeProvider.cs
#pragma warning disable 1634, 1691 namespace System.Workflow.ComponentModel.Compiler { using System; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.Diagnostics; using System.Resources; using System.Reflection; using System.Globalization; using System.IO; using System.Text.RegularExpressions; public sealed class TypeProvider : ITypeProvider, IServiceProvider, IDisposable { internal static readonly char[] nameSeparators = new char[]{'.', '+'}; private IServiceProvider serviceProvider = null; private Hashtable designTimeTypes = new Hashtable(); private Hashtable assemblyLoaders = new Hashtable(); private Hashtable rawAssemblyLoaders = new Hashtable(); private Hashtable compileUnitLoaders = new Hashtable(); private Hashtable hashOfRTTypes = new Hashtable(); private Hashtable hashOfDTTypes = new Hashtable(); // these variables will cache all the information which is passed to private ListaddedAssemblies = null; private List addedCompileUnits = null; private Dictionary needRefreshCompileUnits = null; private bool executingEnsureCurrentTypes = false; private Hashtable typeLoadErrors = new Hashtable(); private Assembly localAssembly = null; public TypeProvider(IServiceProvider serviceProvider) { this.serviceProvider = serviceProvider; } #region Public methods public void SetLocalAssembly(Assembly assembly) { this.localAssembly = assembly; if (this.TypesChanged != null) FireEventsNoThrow(this.TypesChanged, new object[] { this, EventArgs.Empty }); } public void AddAssembly(Assembly assembly) { if (assembly == null) throw new ArgumentNullException("assembly"); if (!rawAssemblyLoaders.Contains(assembly)) { try { rawAssemblyLoaders[assembly] = new AssemblyLoader(this, assembly, this.localAssembly == assembly); if (this.TypesChanged != null) FireEventsNoThrow(this.TypesChanged, new object[] { this, EventArgs.Empty }); } catch(Exception e) { this.typeLoadErrors[assembly.FullName] = e; if (this.TypeLoadErrorsChanged != null) FireEventsNoThrow(this.TypeLoadErrorsChanged, new object[] { this, EventArgs.Empty }); } } } public void RemoveAssembly(Assembly assembly) { if (assembly == null) throw new ArgumentNullException("assembly"); AssemblyLoader assemblyLoader = (AssemblyLoader)this.rawAssemblyLoaders[assembly]; if (assemblyLoader != null) { this.rawAssemblyLoaders.Remove(assembly); RemoveCachedAssemblyWrappedTypes(assembly); if (this.TypesChanged != null) FireEventsNoThrow(this.TypesChanged, new object[] { this, EventArgs.Empty }); } } public void AddAssemblyReference(string path) { if (path == null) throw new ArgumentNullException("path"); if (File.Exists(path) && !this.assemblyLoaders.ContainsKey(path) && (this.addedAssemblies == null || !this.addedAssemblies.Contains(path))) { // lets put these changes into our cache if (this.addedAssemblies == null) this.addedAssemblies = new List (); this.addedAssemblies.Add(path); if (this.TypesChanged != null) FireEventsNoThrow(this.TypesChanged, new object[] { this, EventArgs.Empty }); } } public void RemoveAssemblyReference(string path) { if (path == null) throw new ArgumentNullException("path"); AssemblyLoader assemblyLoader = this.assemblyLoaders[path] as AssemblyLoader; if (assemblyLoader != null) { this.assemblyLoaders.Remove(path); RemoveCachedAssemblyWrappedTypes(assemblyLoader.Assembly); } if (this.addedAssemblies != null && this.addedAssemblies.Contains(path)) this.addedAssemblies.Remove(path); if (this.typeLoadErrors.ContainsKey(path)) { this.typeLoadErrors.Remove(path); if (this.TypeLoadErrorsChanged != null) FireEventsNoThrow(this.TypeLoadErrorsChanged, new object[] { this, EventArgs.Empty }); } if (this.TypesChanged != null) FireEventsNoThrow(this.TypesChanged, new object[] { this, EventArgs.Empty }); } public void AddCodeCompileUnit(CodeCompileUnit codeCompileUnit) { if (codeCompileUnit == null) throw new ArgumentNullException("codeCompileUnit"); if (this.compileUnitLoaders.ContainsKey(codeCompileUnit) || (this.addedCompileUnits != null && this.addedCompileUnits.Contains(codeCompileUnit))) throw new ArgumentException(TypeSystemSR.GetString("Error_DuplicateCodeCompileUnit"), "codeCompileUnit"); // lets put these changes into our cache if (this.addedCompileUnits == null) this.addedCompileUnits = new List (); this.addedCompileUnits.Add(codeCompileUnit); if (this.needRefreshCompileUnits != null && this.needRefreshCompileUnits.ContainsKey(codeCompileUnit)) this.needRefreshCompileUnits.Remove(codeCompileUnit); if (this.TypesChanged != null) FireEventsNoThrow(this.TypesChanged, new object[] { this, EventArgs.Empty }); } public void RemoveCodeCompileUnit(CodeCompileUnit codeCompileUnit) { if (codeCompileUnit == null) throw new ArgumentNullException("codeCompileUnit"); // lets put these changes into our cache CodeDomLoader codeDomLoader = this.compileUnitLoaders[codeCompileUnit] as CodeDomLoader; if (codeDomLoader != null) { codeDomLoader.Dispose(); this.compileUnitLoaders.Remove(codeCompileUnit); } if (this.addedCompileUnits != null && this.addedCompileUnits.Contains(codeCompileUnit)) this.addedCompileUnits.Remove(codeCompileUnit); if (this.needRefreshCompileUnits != null && this.needRefreshCompileUnits.ContainsKey(codeCompileUnit)) this.needRefreshCompileUnits.Remove(codeCompileUnit); if (this.typeLoadErrors.ContainsKey(codeCompileUnit)) { this.typeLoadErrors.Remove(codeCompileUnit); if (this.TypeLoadErrorsChanged != null) FireEventsNoThrow(this.TypeLoadErrorsChanged, new object[] { this, EventArgs.Empty }); } if (this.TypesChanged != null) FireEventsNoThrow(this.TypesChanged, new object[] { this, EventArgs.Empty }); } public void RefreshCodeCompileUnit(CodeCompileUnit codeCompileUnit, EventHandler refresher) { if (codeCompileUnit == null) throw new ArgumentNullException("codeCompileUnit"); if (!this.compileUnitLoaders.Contains(codeCompileUnit) && (this.addedCompileUnits != null && !this.addedCompileUnits.Contains(codeCompileUnit))) throw new ArgumentException(TypeSystemSR.GetString("Error_NoCodeCompileUnit"), "codeCompileUnit"); if (this.needRefreshCompileUnits == null) this.needRefreshCompileUnits = new Dictionary (); this.needRefreshCompileUnits[codeCompileUnit] = refresher; if (this.TypesChanged != null) FireEventsNoThrow(this.TypesChanged, new object[] { this, EventArgs.Empty }); } #endregion #region TargetFrameworkProvider Support // // In general this func can be used by anyone that wants to configure TypeProvder // to do non standard type to assembly name mapping // Specifically this func is set by Microsoft.Workflow.VSDesigner when running within VS // The func encapsulates VS multi-targeting functionality so that System.Workflow.ComponentModel // does not need to take a dependency on VS bits. public Func AssemblyNameResolver { get; set; } public Func IsSupportedPropertyResolver { get; set; } // // VS multi-targeting uses LMR which, unlike reflection, does not cache // Caching in the caller (here) is critical for performance // GetAssemblyName, IsSupportedProperty provide both default behavior // if *Resolver is null and a cache over the LMR methods behind the Resolvers // Caches rely on a single Type universe and object equality however due to issues in reflection it is // possible to get redundant items in the cache. This is because reflection may return different instances // of PropertyInfo depending on what API is called. This is rare but it can happen. Worst case is // redundant entries in the cache; this will not cause incorrect behavior. Dictionary typeToAssemblyName = null; Dictionary supportedProperties = null; public string GetAssemblyName(Type type) { if (type == null) { throw new ArgumentNullException("type"); } if (typeToAssemblyName == null) { typeToAssemblyName = new Dictionary (); } string assemblyName = null; if (!typeToAssemblyName.TryGetValue(type, out assemblyName)) { // // DesignTimeType will not have an assembly if (type.Assembly != null) { if (this.AssemblyNameResolver != null) { assemblyName = this.AssemblyNameResolver(type); } else { assemblyName = type.Assembly.FullName; } typeToAssemblyName.Add(type, assemblyName); } } if (assemblyName == null) { assemblyName = string.Empty; } return assemblyName; } public bool IsSupportedProperty(PropertyInfo property, object declaringInstance) { if (property == null) { throw new ArgumentNullException("property"); } if (declaringInstance == null) { throw new ArgumentNullException("declaringInstance"); } // // If we don't have a resolver to determine if a property is supported // just return true if (IsSupportedPropertyResolver == null) { return true; } if (supportedProperties == null) { supportedProperties = new Dictionary (); } bool supported = false; if (!supportedProperties.TryGetValue(property, out supported)) { supported = IsSupportedPropertyResolver(property, declaringInstance); supportedProperties.Add(property, supported); } return supported; } #endregion #region ITypeProvider Members public Type GetType(string name) { if (name == null) throw new ArgumentNullException("name"); return GetType(name, false); } public Type GetType(string name, bool throwOnError) { if (name == null) throw new ArgumentNullException("name"); EnsureCurrentTypes(); bool hasTypeLoadErrors = false; Type returnType = null; string typeName = string.Empty; string[] parameters = null; string elementDecorator = string.Empty; if (ParseHelpers.ParseTypeName(name, ParseHelpers.ParseTypeNameLanguage.NetFramework, out typeName, out parameters, out elementDecorator)) { if ((parameters != null) && (parameters.Length > 0)) { //Generic type Type templateType = GetType(typeName, throwOnError); if ((templateType == null) || (!templateType.IsGenericTypeDefinition)) return null; Type[] templateParamTypes = new Type[parameters.Length]; for(int index = 0; index < parameters.Length; index++) { Type templateParameter = GetType(parameters[index], throwOnError); if (templateParameter == null) return null; templateParamTypes[index] = templateParameter; } return templateType.MakeGenericType(templateParamTypes); } else if (elementDecorator != string.Empty) { //type with element (Array, ByRef, Pointer) Type elementType = this.GetType(typeName); if (elementType != null) { // first we verify the name is formated well (AssemblyQualifiedName for generic // parameters + no spaces in array brackets) System.Text.StringBuilder nameBuilder = new System.Text.StringBuilder(elementType.FullName); for (int loop = 0; loop < elementDecorator.Length; loop++) if (elementDecorator[loop] != ' ') nameBuilder.Append(elementDecorator[loop]); name = nameBuilder.ToString(); // let tha assembly of the element type a chance to find a type (will fail only // if element contains parameter from external assembly if (elementType.Assembly != null) returnType = elementType.Assembly.GetType(name, false); if (returnType == null) { // now we can fetch or create the type if (this.hashOfDTTypes.Contains(name)) { returnType = this.hashOfDTTypes[name] as Type; } else { returnType = new DesignTimeType(null, name, this); this.hashOfDTTypes.Add(name, returnType); } return returnType; } } } else { // regular type, get the type name string assemblyName = string.Empty; int indexOfComma = name.IndexOf(','); if (indexOfComma != -1) { typeName = name.Substring(0, indexOfComma); assemblyName = name.Substring(indexOfComma + 1).Trim(); } typeName = typeName.Trim(); if (typeName.Length > 0) { returnType = this.designTimeTypes[typeName] as Type; if (returnType == null) { foreach (DictionaryEntry dictionaryEntry in this.rawAssemblyLoaders) { AssemblyLoader assemblyLoader = dictionaryEntry.Value as AssemblyLoader; if ((assemblyName.Length == 0) || (ParseHelpers.AssemblyNameEquals(assemblyLoader.AssemblyName, assemblyName))) { try { returnType = assemblyLoader.GetType(typeName); } catch (Exception e) { if (!this.typeLoadErrors.Contains(dictionaryEntry.Key)) { this.typeLoadErrors[dictionaryEntry.Key] = e; hasTypeLoadErrors = true; } // bubble up exceptions only when appropiate if (throwOnError) throw e; } if (returnType != null) break; } } } if (returnType == null) { foreach (DictionaryEntry dictionaryEntry in this.assemblyLoaders) { AssemblyLoader assemblyLoader = dictionaryEntry.Value as AssemblyLoader; if ((assemblyName.Length == 0) || (ParseHelpers.AssemblyNameEquals(assemblyLoader.AssemblyName, assemblyName))) { try { returnType = assemblyLoader.GetType(typeName); } catch (Exception e) { if (!this.typeLoadErrors.Contains(dictionaryEntry.Key)) { this.typeLoadErrors[dictionaryEntry.Key] = e; hasTypeLoadErrors = true; } // bubble up exceptions only when appropiate if (throwOnError) throw e; } if (returnType != null) break; } } } if (hasTypeLoadErrors) { if (this.TypeLoadErrorsChanged != null) FireEventsNoThrow(this.TypeLoadErrorsChanged, new object[] { this, EventArgs.Empty }); } if (returnType == null && this.localAssembly != null && assemblyName == this.localAssembly.FullName) returnType = this.localAssembly.GetType(typeName); } } } if (returnType == null) { if(throwOnError) throw new Exception(TypeSystemSR.GetString(CultureInfo.CurrentCulture, "Error_TypeResolution", name)); else return null; } // replace the System.Type with RTTypeWrapper for generic types. // WinOE Bug 16560: The type provider may be used at runtime. No RTTypeWrapper should ever be returned // at runtime. // At design time, we need to wrap all generic types even if the parameter types are not // design time types. This is because our parsing function creates a base generic type before it binds // all the parameters. The RTTypeWrapper.MakeGenericType override will then take care of binding to // design time types. if (this.designTimeTypes != null && this.designTimeTypes.Count > 0 && returnType.Assembly != null && returnType.IsGenericTypeDefinition) { if (this.hashOfRTTypes.Contains(returnType)) { returnType = (Type)this.hashOfRTTypes[returnType]; } else { Type returnType2 = new RTTypeWrapper(this, returnType); this.hashOfRTTypes.Add(returnType, returnType2); returnType = returnType2; } } return returnType; } public Type[] GetTypes() { EnsureCurrentTypes(); bool hasTypeLoadErrors = false; this.typeLoadErrors.Clear();//clear all old errors List typeList = new List (); // Design time types foreach (Type type in this.designTimeTypes.Values) typeList.Add(type); foreach (DictionaryEntry dictionaryEntry in this.assemblyLoaders) { AssemblyLoader assemblyLoader = dictionaryEntry.Value as AssemblyLoader; try { typeList.AddRange(assemblyLoader.GetTypes()); } catch (Exception e) { ReflectionTypeLoadException typeLoadException = e as ReflectionTypeLoadException; if (typeLoadException != null) { //we should at least add the types that did get loaded foreach (Type type in typeLoadException.Types) { if (type != null) typeList.Add(type); } } //we should have the latest exception for every assembly (user might have copied required dlls over) if (this.typeLoadErrors.Contains(dictionaryEntry.Key)) this.typeLoadErrors.Remove(dictionaryEntry.Key); this.typeLoadErrors[dictionaryEntry.Key] = e; hasTypeLoadErrors = true; } } foreach (DictionaryEntry dictionaryEntry in this.rawAssemblyLoaders) { AssemblyLoader assemblyLoader = dictionaryEntry.Value as AssemblyLoader; try { typeList.AddRange(assemblyLoader.GetTypes()); } catch (Exception e) { ReflectionTypeLoadException typeLoadException = e as ReflectionTypeLoadException; if (typeLoadException != null) { //we should at least add the types that did get loaded foreach (Type type in typeLoadException.Types) { if (type != null) typeList.Add(type); } } //we should have the latest exception for every assembly (user might have copied required dlls over) if (this.typeLoadErrors.Contains(dictionaryEntry.Key)) this.typeLoadErrors.Remove(dictionaryEntry.Key); this.typeLoadErrors[dictionaryEntry.Key] = e; hasTypeLoadErrors = true; } } if (hasTypeLoadErrors) { if (this.TypeLoadErrorsChanged != null) FireEventsNoThrow(this.TypeLoadErrorsChanged, new object[] { this, EventArgs.Empty }); } return typeList.ToArray(); } public IDictionary
Link Menu
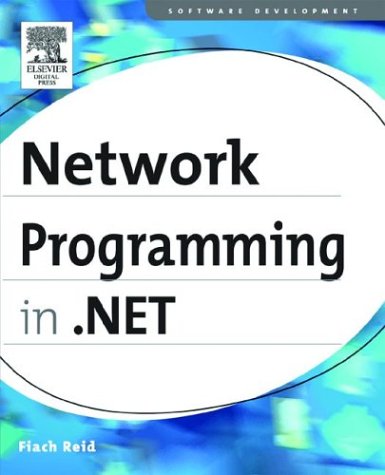
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataExpression.cs
- TransactionBridgeSection.cs
- GridViewColumn.cs
- ButtonFlatAdapter.cs
- SystemIcmpV6Statistics.cs
- __Filters.cs
- ErrorEventArgs.cs
- BookmarkCallbackWrapper.cs
- ToolStripDesignerAvailabilityAttribute.cs
- FixedLineResult.cs
- XmlExceptionHelper.cs
- UserPreferenceChangingEventArgs.cs
- ExtendedProperty.cs
- ChoiceConverter.cs
- LexicalChunk.cs
- InputReport.cs
- CompositeActivityDesigner.cs
- GridErrorDlg.cs
- RepeatBehaviorConverter.cs
- PrePostDescendentsWalker.cs
- ExpressionBuilder.cs
- SafeHandle.cs
- EmptyCollection.cs
- NumericUpDown.cs
- InputLanguage.cs
- StringCollectionEditor.cs
- ImageField.cs
- ServiceDescriptionSerializer.cs
- SafeHandles.cs
- SqlCacheDependency.cs
- BindingsCollection.cs
- ToolStripPanel.cs
- FieldInfo.cs
- VirtualDirectoryMappingCollection.cs
- TablePattern.cs
- WebPartCloseVerb.cs
- ValueQuery.cs
- XmlSubtreeReader.cs
- FrameworkContentElement.cs
- MSG.cs
- PageAsyncTask.cs
- Utils.cs
- XmlQuerySequence.cs
- EntityTransaction.cs
- WebServiceHandlerFactory.cs
- XPathMultyIterator.cs
- NavigationPropertyEmitter.cs
- CharStorage.cs
- ContractHandle.cs
- DbConnectionStringCommon.cs
- Matrix.cs
- ProgressBarAutomationPeer.cs
- SchemaImporter.cs
- ValidateNames.cs
- ScrollItemPatternIdentifiers.cs
- CqlIdentifiers.cs
- OdbcConnectionString.cs
- TextServicesProperty.cs
- ConfigurationLockCollection.cs
- Stack.cs
- VisualStyleTypesAndProperties.cs
- TerminatorSinks.cs
- BehaviorEditorPart.cs
- SmtpLoginAuthenticationModule.cs
- CmsInterop.cs
- XPathParser.cs
- InputLanguageManager.cs
- BitmapEffectOutputConnector.cs
- RuntimeIdentifierPropertyAttribute.cs
- AcceleratedTokenProviderState.cs
- RepeaterItemCollection.cs
- BamlLocalizableResource.cs
- DescendentsWalkerBase.cs
- MultipleViewPatternIdentifiers.cs
- ImageButton.cs
- TrustSection.cs
- SqlDelegatedTransaction.cs
- GridViewEditEventArgs.cs
- EmptyQuery.cs
- EdmToObjectNamespaceMap.cs
- MILUtilities.cs
- Point4D.cs
- ActivityDesigner.cs
- NavigationProperty.cs
- HandleExceptionArgs.cs
- XsltOutput.cs
- Grid.cs
- PropertyValueUIItem.cs
- MgmtResManager.cs
- IntegerValidator.cs
- SelectionItemProviderWrapper.cs
- ControlPaint.cs
- AppDomainResourcePerfCounters.cs
- FileDialog.cs
- DbConnectionPoolIdentity.cs
- GeneratedCodeAttribute.cs
- PermissionSetEnumerator.cs
- SelectedDatesCollection.cs
- FragmentQuery.cs
- PaintEvent.cs